Python Multiline String | Quick Tips for Python Print String
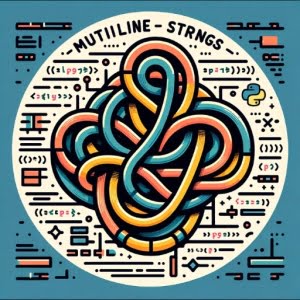
We often discuss maintaining clean code in Python scripting projects at IOFLOOD, for the sake of our colleagues’ sanity. To this end, formatting large blocks of text both with a python multiline string can help with readable output. As we strongly believe in the usefulness of a python multi line string for script clarity, we’ve formulated this article to assist our customers manage text data on their own bare metal cloud projects.
In this guide, we’ll walk you through the process of multiline string Python methods, from the basics to more advanced techniques. We’ll cover everything from the basic use of triple quotes, advanced use of escape sequences and string concatenation, as well as alternative approaches for when a python line is too long for readability.
Let’s get started!
TL;DR: How Do I Create a Python Multiline String?
To create a multiline Python print string, you can use triple quotes (
'''multi line message'''
or"""multi line message"""
). This allows you to write strings that span multiple lines, making your code cleaner and easier to read.
Here’s a simple example:
text = '''This is a
multiline
string'''
print(text)
# Output:
# This is a
# multiline
# string
In this example, we’ve created a multiline string using triple quotes. The string spans three lines, and when printed, it retains the line breaks that were included in the triple quotes. This is a basic way to create multiline strings in Python, but there’s much more to learn about string manipulation in this versatile language.
Stay tuned for more detailed explanations and advanced usage scenarios. Whether you’re a beginner or an experienced developer, you’ll find valuable insights in the sections to come.
Table of Contents
Basics of a Python Multiline String
Python makes it simple to create multiline strings. The most basic way to create a multiline string in Python is by using triple quotes (”’ or “””). This method is straightforward, and it’s perfect for beginners who are just starting to learn Python.
Let’s take a look at an example:
text = """This is a
multiline
string"""
print(text)
# Output:
# This is a
# multiline
# string
In this code block, we’ve defined a string that spans three lines. We’ve used triple quotes to denote the start and end of the string. When we execute the python print string command, it retains the line breaks that were included within the triple quotes.
One of the advantages of this method is its simplicity and readability. It’s easy to see where the string starts and ends, and the line breaks are preserved exactly as they’re written. This is particularly useful when you’re working with large blocks of text or when you need to include line breaks in your string.
However, there are a few potential pitfalls to keep in mind. One is that the indentation of your multiline string is determined by the triple quotes. If you indent your triple quotes, the entire string will be indented. This can lead to unexpected results if you’re not careful.
Advanced Multine String Python
As you become more comfortable with Python, you might find yourself needing more control over your multiline strings. This is where escape sequences and string concatenation come into play.
Escape Sequences in a Python Print String
Escape sequences allow you to include special characters in your strings that would otherwise be difficult or impossible to include. For example, the newline character (\n
) allows you to add a line break to your string.
Here’s an example of how you might use escape sequences to create a multiline string:
multiline_string = 'This is the first line.\nAnd this is the second line.'
print(multiline_string)
# Output:
# This is the first line.
# And this is the second line.
In this example, the \n
escape sequence creates a newline in the string, effectively creating a multiline string.
Multiline String Python Concatenation
String concatenation is another powerful tool for creating multiline strings. You can use the +
operator to combine multiple strings into one, allowing you to create a multiline string from several smaller strings.
Here’s an example of how you might use string concatenation to create a multiline string:
first_line = 'This is the first line.'
second_line = 'And this is the second line.'
multiline_string = first_line + '\n' + second_line
print(multiline_string)
# Output:
# This is the first line.
# And this is the second line.
In this example, we’ve used the +
operator to combine two strings and a newline character (\n
), creating a multiline string.
Both escape sequences and string concatenation offer more control over your multiline strings, but they can also be more complex to use. It’s important to understand the differences between these methods and the basic use of triple quotes, and to choose the method that best suits your needs.
Alternatives: Python Multiline String
While using triple quotes, escape sequences, and string concatenation are the most common methods to create multiline strings in Python, there are other alternatives that can offer more flexibility and control, especially for more complex tasks.
The Join() Function
One such alternative is using the join()
function with a list of strings. This approach can be particularly useful when you’re dealing with a large number of strings or when the strings are being generated dynamically.
Here’s an example of how you might use the join()
function to create a multiline string:
lines = ['This is the first line.', 'And this is the second line.']
multiline_string = '\n'.join(lines)
print(multiline_string)
# Output:
# This is the first line.
# And this is the second line.
In this example, we’ve created a list of strings and then used the join()
function to combine them into a single string, separated by newline characters (\n
). This creates a multiline string.
One of the main advantages of this method is its flexibility. You can easily add, remove, or modify lines in your multiline string by simply updating the list. This can be a powerful tool when you’re dealing with dynamic data.
In conclusion, while the join()
function is a powerful tool for creating multiline strings in Python, it’s best used in situations where its advantages outweigh its complexity and potential performance issues.
To can find out more details on the uses of the Python join() function for strings, lists, and tuples, you can view our free guide here!
Issues in Multiline String Python
While creating multiline strings in Python is generally straightforward, you may encounter some common issues. Let’s discuss these potential pitfalls and how to navigate around them.
Indentation Errors
One common issue when creating multiline strings is indentation errors. Remember, Python is sensitive to white spaces at the start of lines, and this extends to multiline strings as well.
multiline_string = '''
This is line one
This is line two
'''
print(multiline_string)
# Output:
# This is line one
# This is line two
In this example, the indentation in the multiline string is preserved, and when printed, the string includes the spaces at the beginning of each line. This might not be the desired outcome, especially when dealing with structured text or code.
Issues with Escape Sequences
Another common issue arises when you need to include escape sequences in your multiline string. For instance, if you want to include a quotation mark in your string, you need to escape it using a backslash (\
).
multiline_string = '''
\"This is line one\"
\"This is line two\"
'''
print(multiline_string)
# Output:
# "This is line one"
# "This is line two"
In this example, the escape sequence \"
is used to include quotation marks in the multiline string. If you forget to escape them, Python will interpret them as the end of the string, leading to a syntax error.
Understanding these common issues and how to avoid them will help you create multiline strings effectively and avoid unexpected results. As always, the best way to master these skills is through practice and experience.
Core of Python Print String
Before diving deeper into multiline strings, it’s essential to understand the basic concept of strings in Python. A string is a sequence of characters and is one of Python’s built-in data types. You can create a string by enclosing characters in either single quotes ('
), double quotes ("
), or triple quotes ('''
or """
).
single_quote_string = 'Hello, World!'
double_quote_string = "Hello, World!"
triple_quote_string = '''Hello, World!'''
print(single_quote_string)
print(double_quote_string)
print(triple_quote_string)
# Output:
# Hello, World!
# Hello, World!
# Hello, World!
In this example, we’ve created the same string using single quotes, double quotes, and triple quotes. When printed, all three strings produce the same output.
The choice between single quotes, double quotes, and triple quotes depends on the needs of your string. If your string contains a single quote, you might choose to use double quotes to avoid having to escape the single quote. Similarly, if your string spans multiple lines, you might choose to use triple quotes.
Understanding these fundamentals is crucial to mastering the creation of multiline strings in Python. With this knowledge in hand, you’re well-prepared to tackle more advanced topics like escape sequences, string concatenation, and alternative methods for creating multiline strings.
Use Cases of Python Multiline String
Multiline strings in Python are not just about situations when a python line too long for reading. They hold much more significance when it comes to file handling, data processing, and other advanced Python techniques.
Python Multiline Strings in File Handling
Python’s multiline strings are particularly useful when working with files. They allow you to read or write large blocks of text to a file in a format that preserves line breaks, which is essential for maintaining the structure and readability of the text.
Data Processing and Multiline Strings
In data processing, multiline strings can be a powerful tool for working with structured text data. They allow you to create complex data structures, parse text data, and even create dynamic SQL queries.
Concepts Related to Python Print Strings
Once you’ve mastered multiline strings, there are many related concepts in Python that you might find interesting. String formatting in Python, for example, allows you to create strings that include variables, which can be incredibly useful for generating dynamic output.
Regular expressions, on the other hand, are a powerful tool for working with strings. They allow you to perform complex pattern matching and manipulation on strings, making them a valuable tool for tasks like data validation, data extraction, and string transformation.
Further Resources for Python Print Strings
To deepen your understanding of Python strings and related concepts, consider exploring these resources:
- Python Strings Demystified for Beginners: Our beginner-friendly guide that demystifies Python strings, making them easy to understand and use in various programming contexts.
Guide to Python String Methods: IOFlood’s official Python documentation provides in-depth information about string manipulation in Python, including string literals, common string operations, string formatting, and more.
Tutorial on Appending to a String in Python: This tutorial by IOFlood demonstrates different approaches to append to a string in Python. It covers techniques such as using the
+=
operator, string concatenation, and thestr.join()
method.Documentation on Strings: The official Python documentation provides in-depth information about string manipulation in Python, including string literals, common string operations, string formatting, and more.
Guide to Python Multiline String Formatting: This guide covers Python string formatting techniques, such as using the
%
operator and the newerformat()
function.Python Regular Expressions Tutorial: This tutorial on W3Schools provides an introduction to regular expressions in Python. It covers the basics of regular expressions and metacharacters.
Remember, mastery comes with practice. So, don’t hesitate to experiment with multiline strings and related concepts, and explore the endless possibilities they offer in Python programming.
Wrapping Up: Multiline Strings
In this comprehensive guide, we’ve delved into the art of creating multiline strings in Python, a fundamental skill in Python programming that can significantly enhance the readability and structure of your code.
We began with the basics, learning how to create multiline strings using triple quotes. This simple and straightforward method is perfect for beginners and can handle most multiline string needs. We then ventured into more advanced territory, exploring the use of escape sequences and string concatenation for greater control over our multiline strings.
Throughout this journey, we’ve tackled common issues you might encounter when creating multiline strings in Python, such as indentation errors and issues with escape sequences, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to creating multiline strings, such as using the join()
function with a list of strings. This method offers more flexibility and control, particularly when dealing with a large number of strings or dynamic data.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Triple Quotes | Simple, Readable | Indentation, White Space |
Escape Sequences & Concatenation | More Control | Complexity |
Join() Function | Flexibility with Dynamic Data | Complexity, Performance with Large Lists |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of multiline strings in Python. With these skills in hand, you’re well-prepared to write more readable, organized, and efficient Python code. Happy coding!