Python split() | String Manipulation Guide (With Examples)
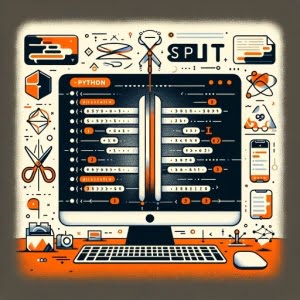
Ever found yourself wrestling with the task of breaking down strings in Python? Consider the Python’s split function your very own culinary expert, adept at chopping up strings into digestible bits. This comprehensive guide is your pathway to understanding the nuances of Python’s split function, unraveling its usage from the most basic level to advanced techniques.
In the realm of Python, the split()
function is a powerful tool that helps you dissect a string and convert it into a list of substrates. Whether you’re a Python newbie or an experienced coder looking to brush up your skills, this guide will serve as your roadmap to mastering Python’s split function.
So, are you ready to dive in and learn how to split strings in Python like a pro? Let’s get started!
TL;DR: How Do I Use Python’s Split Function?
Python’s split function is used to divide a string into a list of substrates. It’s as simple as using the
split()
method. Let’s look at an example:
text = 'Hello World'
words = text.split()
print(words)
# Output:
# ['Hello', 'World']
In this example, we have a string ‘Hello World’. Using the split()
method, we break it down into a list of words, ['Hello', 'World']
. This is the basic usage of the split function in Python. But there’s much more to it! Read on to explore the split function in more depth, including advanced usage scenarios and alternative approaches.
Table of Contents
- Python Split Function: Basic Use
- Dealing with Different Delimiters
- Exploring Alternative Methods for String Splitting
- Troubleshooting Common Issues with Python’s Split Function
- Understanding Python’s String and List Data Types
- The Power of Python Split in Data Processing
- Exploring Related Concepts
- Further Learning and Related Topics
- Python Split Function: A Recap
Python Split Function: Basic Use
The Python split()
function is an inbuilt method that breaks down a string into a list of substrates. It primarily works by identifying spaces (or any specified delimiter) and slicing the string accordingly. The result is a list of ‘words’ that were initially separated by the delimiter in the original string.
Let’s look at a basic example:
sentence = 'Python is fun'
words = sentence.split()
print(words)
# Output:
# ['Python', 'is', 'fun']
In this scenario, we have a string ‘Python is fun’. The split()
method is used to break down this string into a list of words ['Python', 'is', 'fun']
. This is achieved by identifying the spaces in the string and slicing it at those points.
This method is particularly advantageous when you need to parse a sentence or larger block of text into individual words for further processing. However, a potential pitfall to keep in mind is that the default delimiter is a space. Therefore, if your string has words separated by a different delimiter (like a comma or a hyphen), the split()
method won’t work as expected.
In the next section, we’ll explore how to handle different delimiters and more advanced uses of the Python split function.
Dealing with Different Delimiters
The split()
function in Python is versatile and can handle different delimiters. A delimiter is a character or a set of characters that separates words in a string. By default, the split()
function uses a space as a delimiter. However, you can specify a different delimiter according to your needs.
Here’s an example where we use a comma as a delimiter:
data = 'Python,Java,C++'
languages = data.split(',')
print(languages)
# Output:
# ['Python', 'Java', 'C++']
In this example, our string ‘Python,Java,C++’ has words separated by commas. By passing the comma ‘,’ as an argument to the split()
method, we tell Python to use the comma as a delimiter. The output is a list of languages ['Python', 'Java', 'C++']
.
Splitting at Specific Indices
The split()
function also allows you to specify the number of splits to perform, using the second parameter. This can be particularly useful when you want to split a string at specific indices.
Let’s look at an example:
data = 'Python,Java,C++,JavaScript'
languages = data.split(',', 2)
print(languages)
# Output:
# ['Python', 'Java', 'C++,JavaScript']
In this scenario, we have a string ‘Python,Java,C++,JavaScript’ and we want to split it into three substrates. By passing 2 as the second argument to the split()
method, we tell Python to perform only two splits. The output is a list with three substrates ['Python', 'Java', 'C++,JavaScript']
.
These advanced techniques allow you to use the Python split function more effectively. Understanding how to handle different delimiters and split at specific indices can be very useful when dealing with complex strings.
Exploring Alternative Methods for String Splitting
While Python’s split()
function is incredibly handy, it’s not the only tool available for string splitting in Python. Let’s delve into some alternative methods that can be used to split strings, such as the splitlines()
method, the re.split()
function, and even some third-party libraries.
Splitting Lines with splitlines()
The splitlines()
method is a built-in Python function that breaks up a string at line boundaries. This method is particularly useful when dealing with multi-line strings.
Here’s an example:
multiline_string = 'Python
Java
C++'
lines = multiline_string.splitlines()
print(lines)
# Output:
# ['Python', 'Java', 'C++']
In this example, we have a multi-line string ‘Python
Java
C++’. The splitlines()
method breaks it down into a list of lines ['Python', 'Java', 'C++']
.
Regular Expressions with re.split()
The re.split()
function is a part of Python’s re
module, which deals with regular expressions. This function is powerful as it allows you to split a string based on a regular expression, providing much more flexibility.
Consider the following example:
import re
data = 'Python,Java;C++ JavaScript'
words = re.split('[,;\s]', data)
print(words)
# Output:
# ['Python', 'Java', 'C++', 'JavaScript']
In this scenario, we have a string ‘Python,Java;C++ JavaScript’ with words separated by different delimiters – a comma, a semicolon, and a space. Using the re.split()
function with the regular expression ‘[,;\s]’, we’re able to split the string at any of these delimiters. The output is a list of words ['Python', 'Java', 'C++', 'JavaScript']
.
Third-Party Libraries
There are also several third-party libraries available that offer more sophisticated methods for string splitting, such as pandas
and numpy
. These libraries can be particularly useful when dealing with large datasets or complex string manipulation tasks.
In conclusion, while the split()
function is a powerful tool for string splitting in Python, these alternative methods offer additional flexibility and functionality. Depending on your specific use case, one of these methods might be more suitable. Therefore, it’s beneficial to familiarize yourself with these alternatives and understand their advantages and disadvantages.
Troubleshooting Common Issues with Python’s Split Function
While Python’s split()
function is straightforward, you may encounter some common issues when using it. Let’s discuss these potential pitfalls and their solutions.
Dealing with Empty Strings
When splitting a string, you might end up with empty strings in your output list. This usually happens when there are multiple delimiters in a row. Here’s an example:
data = 'Python,,Java'
words = data.split(',')
print(words)
# Output:
# ['Python', '', 'Java']
In this example, there are two commas in a row. The split function treats the area between the two commas as an empty string, resulting in an empty string in the output list. To avoid this, you can use a list comprehension to remove empty strings from the list:
words = [word for word in words if word]
print(words)
# Output:
# ['Python', 'Java']
Splitting on Whitespace
By default, the split()
function splits on spaces. However, it will also split on other types of whitespace, such as tabs and newlines. If you only want to split on spaces, you need to pass a space ‘ ‘ as the delimiter:
data = 'Python Java
C++'
words = data.split(' ')
print(words)
# Output:
# ['Python Java
C++']
In this example, the string ‘Python Java
C++’ contains a tab and a newline. By passing a space ‘ ‘ as the delimiter to the split()
function, we ensure that the string is not split at the tab or newline.
These are just a couple of the issues you might encounter when using Python’s split function. By understanding these pitfalls and their solutions, you can use the split function more effectively.
Understanding Python’s String and List Data Types
Before delving deeper into the Python’s split()
function, it’s crucial to understand the fundamental data types involved – the string and list data types.
String Data Type
In Python, a string is a sequence of characters enclosed in single quotes, double quotes, or triple quotes. It’s an immutable sequence data type, meaning once defined, you can’t change its content. Here’s an example of a string:
str1 = 'Hello, Python!'
print(str1)
# Output:
# Hello, Python!
In this example, ‘Hello, Python!’ is a string.
List Data Type
A list in Python is an ordered sequence of items. It can contain items of different data types. It’s a mutable data type, meaning you can add, remove, or change items after the list is created. Here’s an example of a list:
list1 = ['Python', 'Java', 'C++']
print(list1)
# Output:
# ['Python', 'Java', 'C++']
In this example, ['Python', 'Java', 'C++']
is a list.
The split()
function in Python converts a string into a list by breaking it up at specified delimiters.
Delimiters and Indices
A delimiter is a character or a set of characters that separates words in a string. By default, the split()
function uses a space as a delimiter, but you can specify a different delimiter.
An index refers to the position of an item in a list or a character in a string. In Python, indices start at 0 for the first element.
Understanding these concepts is key to mastering the Python’s split()
function and its application in string manipulation.
The Power of Python Split in Data Processing
The split()
function in Python is not just a string manipulation tool. Its utility extends far beyond, especially in the realms of data processing and text analysis.
The Role of Split in Data Processing
In data processing, the split()
function often serves as a critical first step. It’s used to break down raw data (usually in string format) into more manageable and analyzable pieces. For example, if you’re dealing with a log file where entries are separated by a specific character, the split()
function can help parse the log file into individual entries for further analysis.
Text Analysis and Python Split
In text analysis, the split()
function is indispensable. It’s frequently used to break down large pieces of text into individual words, which can then be analyzed for frequency, sentiment, etc.
text = 'The quick brown fox jumps over the lazy dog'
words = text.split()
print(words)
# Output:
# ['The', 'quick', 'brown', 'fox', 'jumps', 'over', 'the', 'lazy', 'dog']
In this example, we have a sentence ‘The quick brown fox jumps over the lazy dog’. By using the split()
function, we break it down into a list of words. This list can now be analyzed for word frequency, keyword extraction, etc.
Exploring Related Concepts
Once you’ve mastered Python’s split()
function, there are other related concepts worth exploring. Regular expressions offer a more powerful and flexible way to manipulate strings. String concatenation is another important concept that deals with joining strings together.
The Python split()
function is a powerful tool in your Python arsenal. Its applications in string manipulation, data processing, and text analysis make it a must-know for any Python programmer.
Further Learning and Related Topics
For those looking to delve deeper into Python’s string manipulation capabilities, consider the following resources:
- Beginner’s Guide to Python Print Statements – Dive into the various parameters and options available with the Python print() function.
Python Printing: No Newline – Learn how to use the end parameter in Python’s print() function to control output formatting.
To Lowercase in Python – Understand how to convert strings to lowercase in Python for standardizing text data.
Python String Split Method – More info on the split method in Python.
Python String Format Method – This guide from W3Schools illustrates how to use the ‘format’ method.
Mastering String Manipulation in Python – Get practical advice on various string manipulation techniques in Python.
Python Split Function: A Recap
In this guide, we’ve delved into the depths of Python’s split()
function, a powerful tool for string manipulation. We’ve explored its basic usage, where it breaks down a string into a list of substrates using spaces as the default delimiter.
sentence = 'Python is fun'
words = sentence.split()
print(words)
# Output:
# ['Python', 'is', 'fun']
We’ve also discussed common issues such as dealing with empty strings and splitting on whitespace, and offered solutions to handle these problems effectively.
Moreover, we’ve examined advanced usage scenarios, including handling different delimiters and splitting at specific indices. We’ve also looked at alternative methods for string splitting, such as the splitlines()
method, the re.split()
function, and third-party libraries.
While the split()
function is a powerful tool, these alternatives can offer additional flexibility and functionality, depending on your specific use case.
Method | Use Case | Example |
---|---|---|
split() | Basic string splitting | sentence.split() |
splitlines() | Splitting multi-line strings | multiline_string.splitlines() |
re.split() | Splitting based on regular expressions | re.split('[,;\s]', data) |
This table summarizes the different methods discussed in this guide.
Mastering Python’s split()
function and its alternatives can significantly enhance your string manipulation capabilities in Python. Whether you’re parsing data or analyzing text, these tools are essential in your Python toolkit.