While Loop Java: The Basics and Beyond
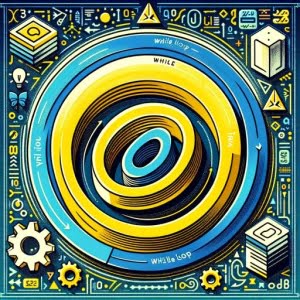
Are you finding it challenging to grasp the concept of while loops in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling while loops in Java, but we’re here to help.
Think of a while loop in Java as a repeating if statement. It executes a block of code as long as a certain condition holds true. It’s like a persistent machine, tirelessly performing its task until told to stop.
In this guide, we’ll walk you through the syntax, usage, and common pitfalls of while loops in Java. We’ll cover everything from the basics of while loops to more advanced techniques, as well as alternative approaches.
Let’s get started and master the while loop in Java!
TL;DR: How Do I Use a While Loop in Java?
A while loop in Java is used to repeatedly execute a block of code as long as a certain condition is true. You can create a while loop in Java using the following syntax:
while(condition) { // code to be executed }
.
Here’s a simple example:
int i = 0;
while(i < 5) {
System.out.println(i);
i++;
}
// Output:
// 0
// 1
// 2
// 3
// 4
In this example, we initialize a variable i
to 0. The while loop checks if i
is less than 5. If the condition is true, it executes the code block inside the loop, which prints the value of i
and then increments i
by 1. This continues until i
is no longer less than 5, at which point the loop stops executing.
This is a basic way to use a while loop in Java, but there’s much more to learn about controlling the flow of your programs with loops. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Syntax and Basic Use of While Loop in Java
The while
loop in Java is a control flow statement that allows code to be executed repeatedly based on a given condition. The condition expression must be a boolean expression. The loop executes the block of code as long as the condition is true
. Once the condition becomes false
, the loop terminates. Let’s break down the syntax:
while (condition) {
// Block of code to be executed
}
Here’s a simple example:
int count = 0;
while (count < 5) {
System.out.println("Count is: " + count);
count++;
}
// Output:
// Count is: 0
// Count is: 1
// Count is: 2
// Count is: 3
// Count is: 4
In this example, we have a variable count
initialized to 0. The while
loop checks if count
is less than 5. If the condition is true, it executes the code within the loop, which prints the current value of count
and then increments count
by 1. The loop continues until count
is no longer less than 5, at which point the loop stops executing.
Avoiding Infinite Loops
An important aspect to note while using while
loops is the updating of the condition within the loop. If the condition never becomes false
, the loop will continue indefinitely, creating what we call an infinite loop. This can cause your program to hang or crash. In the above example, we increment count
within the loop, ensuring that the condition eventually becomes false
(when count
reaches 5), thus avoiding an infinite loop.
Advanced Usage of While Loop in Java
As you become more comfortable with while loops in Java, you’ll encounter situations that require more complex uses of these loops. Let’s look at two such scenarios: nested while loops and while-true loops.
Nested While Loops
A nested while loop is a while loop within another while loop. The inner loop completes all its iterations for each single iteration of the outer loop. Here’s an example:
int i = 0;
while(i < 3) {
int j = 0;
while(j < 3) {
System.out.println("i: " + i + ", j: " + j);
j++;
}
i++;
}
// Output:
// i: 0, j: 0
// i: 0, j: 1
// i: 0, j: 2
// i: 1, j: 0
// i: 1, j: 1
// i: 1, j: 2
// i: 2, j: 0
// i: 2, j: 1
// i: 2, j: 2
In this example, for each iteration of the outer loop (i
loop), the inner loop (j
loop) runs completely, resulting in a total of 9 print statements.
While-True Loops
A while-true loop is a loop that continues indefinitely because its condition always remains true. It’s often used when the program needs to wait for a certain event to occur, or when it should run until the user decides to stop it. To stop a while-true loop, you can use the break
statement. Here’s an example:
while(true) {
System.out.println("This will print until you stop it.");
// Assuming some condition here
if (someCondition) {
break;
}
}
In this code, the message will print indefinitely until someCondition
becomes true. When that happens, the break
statement is executed, which immediately terminates the loop.
Comparing While Loops with Other Loop Structures in Java
While loops are just one of the ways to control the flow of your program in Java. Other looping structures such as for loops and do-while loops offer alternative ways to handle repeated tasks. Let’s compare these structures and discuss when to use each one.
For Loops
For loops are ideal when you know exactly how many times you want the loop to iterate. The syntax of a for loop includes initialization, condition, and increment/decrement in a single line, which can make your code more compact and readable. Here’s an example:
for(int i = 0; i < 5; i++) {
System.out.println(i);
}
// Output:
// 0
// 1
// 2
// 3
// 4
In this example, the loop will run exactly five times. The variable i
is initialized to 0, incremented by 1 after each iteration, and the loop continues as long as i
is less than 5.
Do-While Loops
Do-while loops are similar to while loops, but with a key difference: the do-while loop will execute the block of code at least once, even if the condition is false from the start. This is because the condition is checked after the first execution. Here’s an example:
int i = 5;
do {
System.out.println(i);
i++;
} while(i < 5);
// Output:
// 5
In this example, even though i
is not less than 5, the loop still executes once, printing the value of i
, before checking the condition and terminating the loop.
In summary, while loops are a powerful tool for controlling the flow of your Java programs, but they’re not always the best tool for the job. Depending on the specifics of your task, a for loop or a do-while loop might be a better choice.
Troubleshooting While Loops in Java
Like any aspect of programming, working with while loops in Java can present certain challenges. Let’s discuss some common issues you might encounter, such as infinite loops and off-by-one errors, and how to resolve them.
Avoiding Infinite Loops
An infinite loop occurs when the condition in your while loop never becomes false. This causes the loop to run indefinitely, which can cause your program to become unresponsive. Here’s an example of an infinite loop:
while(true) {
System.out.println("This will print forever!");
}
To avoid infinite loops, make sure that the condition in your while loop will eventually become false. This usually involves modifying a variable within the loop so that it changes the result of the condition.
Off-By-One Errors
An off-by-one error occurs when a loop iterates one time too many or one time too few. This can happen if you’re not careful with the condition in your while loop. For example:
int i = 0;
while(i <= 5) {
System.out.println(i);
i++;
}
// Output:
// 0
// 1
// 2
// 3
// 4
// 5
In this example, the loop runs six times, not five, because it also executes when i
is equal to 5. To avoid off-by-one errors, be sure to double-check your conditions and consider the initial and final values of your loop variable.
Best Practices
To ensure your while loops work as intended, follow these best practices:
- Always initialize your loop variables.
- Make sure the loop condition changes within the loop.
- Be mindful of off-by-one errors.
- Test your loops with different inputs to ensure they work correctly in all scenarios.
With these tips in mind, you’ll be well-equipped to tackle while loops in Java effectively and efficiently.
Understanding the Concept of Loops in Programming
Loops are fundamental constructs in any programming language, including Java. They allow us to execute a block of code multiple times, which is often necessary in programming tasks. Whether it’s processing items in a collection, repeating an operation until a condition is met, or simply waiting for an event to occur, loops are an indispensable part of a programmer’s toolkit.
Why Are Loops Important?
Imagine you need to print the numbers from 1 to 100. Without a loop, you’d have to write a print statement 100 times! With a loop, you can accomplish this task with just a few lines of code:
for(int i = 1; i <= 100; i++) {
System.out.println(i);
}
// Output:
// 1
// 2
// ...
// 100
This not only makes your code more concise and readable, but it also makes it more flexible and maintainable. If you later decide to print the numbers up to 200, you only need to change a single number in your code.
How Do Loops Work in Java?
In Java, a loop typically consists of three parts: initialization, condition, and update. Let’s look at these parts in the context of a while loop:
int i = 0; // Initialization
while(i < 5) { // Condition
System.out.println(i);
i++; // Update
}
// Output:
// 0
// 1
// 2
// 3
// 4
- Initialization: Before the loop starts, we initialize a variable (
i
in this case) that we’ll use in the loop condition. - Condition: This is a boolean expression that determines whether the loop should continue. If the condition is true, the loop executes. If it’s false, the loop terminates.
- Update: Inside the loop, we update the loop variable in some way. This is crucial for ensuring that the loop condition eventually becomes false, preventing infinite loops.
In the next sections, we’ll delve deeper into the specifics of while loops in Java, including their syntax, usage, and common pitfalls.
Expanding the Use of While Loops in Java
While loops in Java are not just limited to simple tasks. They can be a powerful tool when dealing with larger projects or scripts, allowing you to control the flow of your program in a dynamic way. Whether it’s processing large amounts of data, controlling user interactions, or managing the behavior of threads, while loops often play a crucial role.
For example, in a large project, you might use a while loop to continuously check for user input, to monitor the status of a database, or to keep a thread running until a certain condition is met.
while(!userHasQuit) {
// Check for user input
// Process data
// Update database
}
In this example, the loop continues running as long as the userHasQuit
variable is false
. Inside the loop, the program might check for user input, process data, and update a database. When the user decides to quit (setting userHasQuit
to true
), the loop stops, and the program can perform any necessary cleanup operations.
Exploring Related Topics: Recursion and Multi-Threading
As you get more comfortable with while loops, you might want to explore related topics such as recursion and multi-threading in Java. Recursion is a concept where a method calls itself to solve a problem, and it can often be used as an alternative to traditional looping structures. Multi-threading allows a program to perform multiple tasks concurrently, and controlling the flow of these threads often involves careful use of while loops and other control structures.
Further Resources for Mastering Java Loops
To continue your journey in mastering loops in Java, here are some additional resources that you might find helpful:
- Fundamentals Covered: Loops in Java – Understand loop scoping and variable visibility in Java.
Recursion in Java – Understand recursion in Java for solving problems through self-referential function calls.
Using For-Each Loop in Java – Master the for-each loop for concise and readable code in Java.
Oracle Java Tutorials: Control Flow Statements – Understand the procuring flow of statements in Java by Oracle.
GeeksforGeeks’ Loops in Java – Comprehensive guide on utilizing loops in Java programming.
W3Schools’ Java While Loop Tutorial – Learn the essentials of Java while loop with W3Schools tutorials.
These resources offer in-depth discussions, examples, and exercises on while loops and other control flow statements in Java, helping you further solidify your understanding and improve your coding skills.
Wrapping Up: Java While Loop
In this comprehensive guide, we’ve delved into the world of while loops in Java, starting from the basics and moving towards more advanced concepts.
We began with the fundamentals, understanding the importance of loops in programming and how they work in Java. We then explored the syntax and basic usage of while loops, including how to avoid common pitfalls like infinite loops and off-by-one errors. We also ventured into more advanced territory, discussing nested while loops and while-true loops, and how they can be used effectively in Java programming.
In addition to while loops, we also compared them with other looping structures in Java, such as for loops and do-while loops, providing you with a broader perspective on how to control the flow of your programs. Here’s a quick comparison of these loops:
Loop Type | Use Case | Pros | Cons |
---|---|---|---|
While Loop | When you don’t know the number of iterations in advance | Simple and easy to understand | Can lead to infinite loops if not handled properly |
For Loop | When you know the exact number of iterations | Compact syntax, easy to control the number of iterations | Not suitable when the number of iterations is unknown |
Do-While Loop | When the loop should execute at least once regardless of the condition | Guarantees at least one execution of the loop | Less commonly used, can be confusing |
Whether you’re just starting out with Java or you’re looking to level up your looping skills, we hope this guide has given you a deeper understanding of while loops and their usage in Java.
Mastering while loops is a crucial step in becoming proficient in Java. With this knowledge, you’re now well-equipped to tackle complex programming tasks with more efficiency and confidence. Happy coding!