Java Recursion Techniques: A Step-by-Step Guide
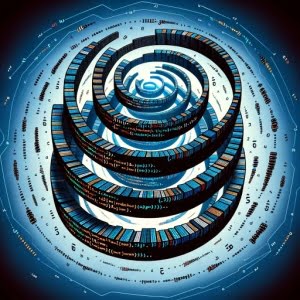
Are you finding it challenging to understand recursion in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling recursion in Java, but we’re here to help.
Think of Java recursion as a Russian doll – a method that contains a smaller version of itself, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of understanding recursion in Java, from the basics to more advanced techniques. We’ll cover everything from the simple recursive methods to more complex uses, as well as alternative approaches.
Let’s get started!
TL;DR: What is Recursion in Java?
Recursion in Java is a process where a method calls itself to solve a problem, for example: calling
return n * factorial(n - 1);
inside a function calledfactorial
. A method in Java might call itself during its own execution to solve a problem in smaller, more manageable parts.
Here’s a simple example of recursion in Java:
public static int factorial(int n) {
if (n == 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
# Output:
# If you call factorial(5), the output will be 120
In this example, we define a method factorial
that calculates the factorial of a number using recursion. The method calls itself, reducing the problem size with each call. When the problem size (in this case, the number n
) reaches zero, the method returns a base case (in this case, 1
). This base case stops the recursion and allows the stacked calls to resolve.
This is just a basic example of recursion in Java. There’s much more to learn about recursion, including more complex recursive methods and alternatives to recursion. Continue reading for a deeper understanding and more complex examples.
Table of Contents
- Understanding Java Recursion: A Beginner’s Perspective
- Advanced Recursion: Multiple Recursive Cases
- Exploring Alternatives: Recursion vs Iteration
- Troubleshooting Recursion: Common Pitfalls and Best Practices
- Understanding Recursion: The Fundamental Concepts
- Recursion in Real-World Applications: Sorting Algorithms and Tree Traversal
- Wrapping Up: Mastering Recursion in Java
Understanding Java Recursion: A Beginner’s Perspective
Recursion in Java, as in any other programming language, is a technique where a method calls itself to solve a problem. The idea is to break down a complex problem into smaller, manageable parts that can be solved individually and then combined to find the overall solution.
Let’s start with a simple example of a recursive method in Java:
public class Main {
public static void countDown(int n) {
if (n > 0) {
System.out.println(n);
countDown(n - 1);
}
}
public static void main(String[] args) {
countDown(5);
}
}
# Output:
# 5 4 3 2 1
In this example, this countDown
function outputs a given number, and then calls itself with that number minus one, until it reaches 0. This is a great beginner’s example as it clearly illustrates the concept of recursion – a function calling itself until it reaches a “base case”, at which point it stops. In this case, the base case is when n
is no longer greater than 0.
When and Why to Use Recursion
Recursion is a powerful tool in a programmer’s toolkit. It’s particularly useful when you need to solve problems that can be broken down into similar sub-problems. Recursive algorithms are often simpler and more intuitive to understand than their iterative counterparts.
However, recursion should be used judiciously. It can lead to problems such as stack overflow errors if the depth of recursion is too high. It can also be less efficient than iterative solutions for large inputs due to the overhead of function calls.
Understanding when and why to use recursion, as well as being aware of its potential pitfalls, is an important part of mastering Java recursion.
Advanced Recursion: Multiple Recursive Cases
As you become more comfortable with basic recursion, you’ll find that some problems require a more complex approach. These problems often involve multiple recursive cases, where a method calls itself more than once during its execution.
Let’s consider an example: The Fibonacci sequence. Each number in this sequence is the sum of the two preceding ones. Here’s how you can generate the Fibonacci sequence using recursion in Java:
public static int fibonacci(int n) {
if (n <= 1) {
return n;
} else {
return fibonacci(n - 1) + fibonacci(n - 2);
}
}
# Output:
# If you call fibonacci(7), the output will be 13
In this example, the fibonacci
method calls itself twice within its execution. The base case is when n
is less than or equal to 1
, where it returns n
. For other cases, it calls itself with n-1
and n-2
and returns the sum of these two calls. This process continues until n
becomes 1
or 0
, at which point the recursion stops, and the method returns the calculated Fibonacci number.
This example illustrates how recursion can be used to solve problems that involve multiple recursive cases. However, it’s important to note that this approach can lead to inefficiencies due to repeated computations. We’ll explore how to address this issue in the ‘Troubleshooting and Considerations’ section.
Exploring Alternatives: Recursion vs Iteration
While recursion is a powerful tool, it’s not always the best approach for every problem. One common alternative to recursion is iteration, where you use loops to repeat a set of instructions until a certain condition is met.
Iteration as an Alternative
Let’s consider the factorial function again, but this time using an iterative approach:
public static int factorial(int n) {
int result = 1;
for (int i = 2; i <= n; i++) {
result *= i;
}
return result;
}
# Output:
# If you call factorial(5), the output will be 120
In this example, we use a for
loop to calculate the factorial of a number. The loop starts from 2
and multiplies result
by each number up to n
. This approach gives the same result as the recursive method, but it does so iteratively.
Recursion vs Iteration: Which to Use?
Both recursion and iteration have their advantages and disadvantages. Recursion can make code easier to understand and can be more intuitive for problems that naturally lend themselves to recursive solutions. However, it can also lead to inefficiencies due to repeated computations and can cause stack overflow errors for deep recursion.
On the other hand, iteration can be more efficient for large inputs and doesn’t carry the risk of stack overflow. However, iterative solutions can be more complex and harder to understand for problems that naturally lend themselves to recursive solutions.
As a rule of thumb, consider the nature of the problem, the size of the input, and the potential for stack overflow when deciding whether to use recursion or iteration. In some cases, you might also consider hybrid approaches that combine elements of both recursion and iteration.
Troubleshooting Recursion: Common Pitfalls and Best Practices
While recursion in Java can be a powerful tool, it can also lead to some common issues if not handled correctly. Let’s discuss these issues and how to avoid them.
Stack Overflow Errors
One of the most common issues with recursion is the risk of a stack overflow error. This happens when the depth of recursion is too high, exceeding the limit of the call stack.
For example, calling a recursive method with a large input might cause a stack overflow error:
public static int factorial(int n) {
if (n == 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
# Output:
# If you call factorial(10000), you'll get a StackOverflowError
In this example, calling factorial(10000)
leads to a StackOverflowError
because the depth of recursion exceeds the limit of the call stack.
Avoiding Stack Overflow Errors
To avoid stack overflow errors, you can often refactor your recursive method to an iterative one, as we discussed in the ‘Alternative Approaches’ section. Alternatively, you can use techniques like tail recursion optimization, which Java unfortunately does not support natively but can be achieved with some workarounds.
Best Practices for Recursive Methods
When writing recursive methods in Java, there are a few best practices to keep in mind:
- Define a clear base case: The base case is what stops the recursion. Without a clear and reachable base case, your recursive method might run indefinitely.
Ensure progress towards the base case: Each recursive call should progress towards the base case. If the recursive calls do not progress towards the base case, you might end up with infinite recursion.
Be mindful of the call stack limit: Deep recursion can lead to stack overflow errors. If your problem requires deep recursion, consider using an iterative solution instead or implementing tail recursion optimization.
By keeping these considerations in mind, you can write effective and efficient recursive methods in Java.
Understanding Recursion: The Fundamental Concepts
Before we dive deeper into recursion in Java, let’s take a step back and discuss the fundamental concept of recursion in computer science and its related concepts such as the call stack and base case.
What is Recursion?
Recursion, in the realm of computer science, is a method of solving problems where the solution depends on solutions to smaller instances of the same problem. This involves a function calling itself while a certain condition is true. The process continues until a condition (known as the base case) is met.
The Call Stack and Recursion
To understand recursion, it’s necessary to understand the concept of the call stack. The call stack is a data structure that stores information about the active subroutines in a program. In the case of recursion, every time a recursive call is made, the current computation is pushed onto the call stack. When the base case is reached, the computations are popped from the stack and resolved in a last-in, first-out (LIFO) order.
Consider our factorial example:
public static int factorial(int n) {
if (n == 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
# Output:
# If you call factorial(3), the output will be 6
When you call factorial(3)
, three frames are pushed onto the call stack:
factorial(3)
factorial(2)
factorial(1)
When the base case factorial(0)
is reached, it starts popping the frames from the stack and resolving them, starting with factorial(1)
and ending with factorial(3)
.
The Base Case in Recursion
The base case in recursion is a condition that determines when the recursive calls should stop. It’s crucial to define a reachable base case to prevent infinite recursion. In our factorial example, the base case is n == 0
, which returns 1
and stops the recursion.
Understanding these fundamental concepts is key to mastering recursion in Java.
Recursion in Real-World Applications: Sorting Algorithms and Tree Traversal
Recursion isn’t just a theoretical concept. It’s a practical tool that’s used in various real-world applications, particularly in data structures and algorithms. Let’s look at a couple of examples: sorting algorithms and tree traversal.
Recursive Sorting Algorithms
Many sorting algorithms use recursion to break down the problem of sorting a list into smaller, more manageable problems. One such algorithm is QuickSort. In QuickSort, a pivot element is chosen from the array. The array is rearranged such that all elements smaller than the pivot come before the pivot and all elements greater than the pivot come after it. This process is repeated on the sub-arrays to the left and right of the pivot, using recursion.
Here’s a simple implementation of QuickSort in Java:
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivotIndex = partition(arr, low, high);
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
# Output:
# If you call quickSort on an unsorted array, it will sort the array in ascending order
Tree Traversal Using Recursion
Recursion is also commonly used in tree traversal algorithms. In a binary tree, for example, you can use recursion to visit each node in a specified order. The three common types of depth-first traversal – in-order, pre-order, and post-order – all use recursion.
Here’s a simple example of in-order traversal of a binary tree in Java:
public static void inOrderTraversal(TreeNode node) {
if (node != null) {
inOrderTraversal(node.left);
System.out.println(node.value);
inOrderTraversal(node.right);
}
}
# Output:
# If you call inOrderTraversal on a binary tree, it will print the values of the nodes in in-order traversal order
Further Resources for Mastering Java Recursion
If you’re interested in learning more about recursion in Java, here are a few resources that might be helpful:
- Beginner’s Guide to Loops in Java – Discover the while loop for iterative operations in Java.
Using While Loop in Java – Master the while loop for flexible and efficient iteration in Java programs.
Sleep Usage in Java – Learn how to use sleep for introducing delays in Java programs.
Java Recursion guide by JavaTpoint includes explanations, examples, and some commonly seen recursive patterns.
GeeksforGeeks’ Recursion in Java offers a variety of examples and problems to solve.
Baeldung’s Understanding Recursion in Java provides a deep dive into recursion and real-world applications.
Wrapping Up: Mastering Recursion in Java
In this comprehensive guide, we’ve delved deep into the concept of recursion in Java, exploring its uses, potential issues, and alternatives.
We began with the basics, breaking down the concept of recursion and illustrating its functionality with simple examples. We then journeyed into more advanced territory, discussing multiple recursive cases and how to handle them. Along the way, we tackled common challenges such as stack overflow errors, providing solutions and best practices to avoid these pitfalls.
We also examined alternative approaches to recursion, specifically iteration, and provided a comparison to help you decide which approach is best suited for your specific needs. Here’s a quick comparison of these methods:
Method | Ease of Understanding | Efficiency | Risk of Stack Overflow |
---|---|---|---|
Recursion | High for recursive problems | Can be less efficient due to function call overhead | High for deep recursion |
Iteration | High for iterative problems | More efficient for large inputs | No risk |
Whether you’re a beginner just starting with recursion in Java, or an experienced developer looking to refine your skills, we hope this guide has been a valuable resource for you. Now you’re equipped with the knowledge and skills to effectively use recursion in Java, as well as to understand when an alternative approach might be more suitable.
Recursion is a powerful tool in the programmer’s toolkit, and mastering it will open up new possibilities in your coding journey. Happy coding!