SQL Update | How to use the Update Statement in SQL
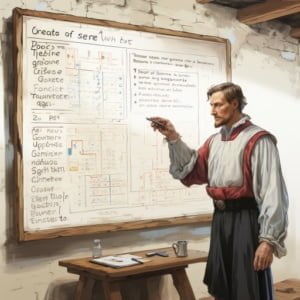
Imagine a world where data is static, unchangeable, and stuck in time. It’s a world that’s hard to envision, right? That’s because we live in a dynamic world where information is constantly changing, and our databases need to reflect that. This is where the SQL Update command comes into play. It’s a vital tool in the SQL arsenal, allowing you to modify records in your databases to keep up with the ever-changing world.
Whether you’re adjusting prices in an online store, updating contact information, or correcting a typo, the SQL Update command is there to make it happen. Think of it as the ‘edit’ button of your database, always ready to make necessary changes with precision and efficiency.
In this blog post, we aim to turn you into an SQL Update command pro. We’ll dive deep into its syntax, use cases, and some unique insights that will help you use the SQL Update command effectively and efficiently. So, if you’re ready to take your SQL skills to the next level, let’s get started!
TL;DR: What is the SQL Update command?
The SQL Update command is a SQL statement that allows you to modify records in your databases, keeping up with the dynamic nature of information. It’s like the ‘edit’ button of your database, enabling changes from adjusting prices in an online store to updating contact information. See example:
UPDATE Customers
SET ContactName = 'Alfred Schmidt', City= 'Frankfurt'
WHERE CustomerID = 1;
In this example, the UPDATE
SQL statement changes the ContactName
and City
for the customer with CustomerID
1.
Table of Contents
- Common Use Cases for the SQL Update Command
- The Impact of Efficient Updates on Database Performance
- The Syntax of the SQL Update Command
- The WHERE Clause in the Update Command
- An Example of a Simple SQL Update Command
- The Risks of Omitting the WHERE Clause
- Understanding the WHERE Clause
- Updating Multiple Columns with the SQL Update Command
- Example of Updating Multiple Columns in SQL
- Ensuring Accuracy When Updating Multiple Columns
- Benefits of Updating Multiple Columns Simultaneously
- Updating Multiple Rows with the SQL Update Command
- An Example of Updating Multiple Rows in SQL
- The Role of the CASE Statement in Updating Multiple Rows
- The Power of the CASE Statement for Conditional Updates
- SQL Update’s Row Locking Mechanism
- The Importance of the Row Locking Mechanism
- Illustrating the SQL Update Command’s Row Locking Mechanism
- Gaining Proficiency in the Row Locking Mechanism
- Wrapping Up
Common Use Cases for the SQL Update Command
The SQL Update command is commonly used in various scenarios. For instance, you might need to update a customer’s address after they move, change the price of a product, or update a user’s email after they get a new job. The SQL Update command is also used to modify multiple columns and select specific rows using the WHERE
clause. The WHERE
clause is like a filter that specifies which record or records should be updated.
The Impact of Efficient Updates on Database Performance
Efficient updating isn’t just about accuracy – it’s also about performance. When you update records efficiently, you can significantly improve your database’s performance. This is because databases are optimized to handle changes in data efficiently. So, the better your SQL Update command skills, the faster and more responsive your database can be. It’s like tuning a car engine – the better the tuning, the better the performance.
The Syntax of the SQL Update Command
Understanding the syntax of the SQL Update command is akin to learning the rules of a board game. Once you have grasped them, you can play effectively. The basic syntax of the SQL Update command is as follows:
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
In this syntax, table_name
refers to the name of the table where you want to update the data. column1
, column2
… are the names of the columns you want to update, and value1
, value2
… are the new values you want to insert. The WHERE
clause is used to specify the records that will be updated.
The WHERE Clause in the Update Command
The WHERE
clause in the SQL Update command acts as the targeting system of a guided missile, ensuring that the update operation hits the right target. If you omit the WHERE
clause, the SQL Update command will update all records in the table, leading to potential disastrous implications.
Consider the following SQL Update statement as an example:
UPDATE Customers
SET ContactName = 'Alfred Schmidt';
In this case, the WHERE
clause is missing. As a result, the ContactName
for all customers in the Customers
table will be updated to ‘Alfred Schmidt’. This is equivalent to changing everyone’s name in a city to ‘Alfred Schmidt’ – a situation you certainly want to avoid!
An Example of a Simple SQL Update Command
Let’s look at a simple example of an SQL Update command. Suppose you want to change the ContactName
of the customer with CustomerID
1 to ‘Alfred Schmidt’. You would use the following SQL Update command:
UPDATE Customers
SET ContactName = 'Alfred Schmidt'
WHERE CustomerID = 1;
In this case, only the ContactName
of the customer with CustomerID
1 is updated to ‘Alfred Schmidt’. The WHERE
clause ensures that the update operation is targeted and precise.
The Risks of Omitting the WHERE Clause
Not using the WHERE
clause in an SQL Update command is like swinging a sword blindly – you’re likely to cause unintended damage. As mentioned earlier, if you omit the WHERE
clause, the SQL Update command will update all records in the table. This could lead to massive data corruption, especially in large databases. Hence, it is crucial to always include the WHERE
clause in your SQL Update commands to avoid unintentional modifications.
Understanding the WHERE Clause
Understanding the WHERE
clause is vital for precision in SQL commands. It’s like knowing how to aim in archery – without it, you’re likely to miss your target. By using the WHERE
clause effectively, you can ensure that your SQL Update commands modify only the intended records, thereby maintaining the integrity and accuracy of your database.
Updating Multiple Columns with the SQL Update Command
The SQL Update command is not just capable of updating a single column, but it can also update multiple columns simultaneously. This feature is akin to multitasking, allowing you to make several changes to your data at once. The syntax for updating multiple columns is as follows:
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
In this syntax, column1
, column2
… are the names of the columns you want to update, and value1
, value2
… are the new values you want to insert. You can list as many column-value pairs as you need, separated by commas.
Example of Updating Multiple Columns in SQL
Let’s consider an example. Suppose you want to update the ContactName
and City
of the customer with CustomerID
1. You would use the following SQL Update command:
UPDATE Customers
SET ContactName = 'Alfred Schmidt', City = 'Frankfurt'
WHERE CustomerID = 1;
In this case, both the ContactName
and City
of the customer with CustomerID
1 are updated simultaneously. The WHERE
clause ensures that the update operation is targeted and precise.
Ensuring Accuracy When Updating Multiple Columns
When updating multiple columns, accuracy is of paramount importance. It’s similar to juggling – one wrong move can lead to a mess. If you make a mistake while updating multiple columns, you could end up with incorrect data in your database. Therefore, always double-check your SQL Update commands before running them to ensure they’re correct.
Benefits of Updating Multiple Columns Simultaneously
Updating multiple columns simultaneously can save time and ensure data consistency. It’s akin to cooking a meal – you can prepare several dishes at once to save time and ensure all the dishes are ready together. Similarly, updating multiple columns at once can save you from having to run multiple SQL Update commands. Moreover, it ensures that all the changes are made together, maintaining the consistency of your data.
Updating Multiple Rows with the SQL Update Command
The SQL Update command isn’t limited to updating just one row at a time. It also has the capability to update multiple rows simultaneously, a feature that can prove to be a real time-saver when dealing with large databases. You might be wondering, ‘How is this possible?’ The answer lies in the WHERE
clause. By specifying a condition that matches multiple rows, you can update all those rows at once.
Here’s the basic syntax:
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
In this case, the condition
in the WHERE
clause matches multiple rows. As a result, the SQL Update command updates all those rows simultaneously.
An Example of Updating Multiple Rows in SQL
Let’s consider an example. Suppose you have a Products
table, and you want to increase the Price
of all products in the ‘Electronics’ category by 10%. Here’s how you could do it with the SQL Update command:
UPDATE Products
SET Price = Price * 1.10
WHERE Category = 'Electronics';
In this case, the WHERE
clause matches all products in the ‘Electronics’ category. As a result, the SQL Update command increases the Price
of all those products by 10%.
The Role of the CASE Statement in Updating Multiple Rows
In some scenarios, you might want to update multiple rows with different values. This is where the CASE
statement comes in handy. The CASE
statement allows you to perform conditional updates in SQL, which means you can update different rows with different values based on certain conditions.
Here’s the basic syntax of the CASE
statement in an SQL Update command:
UPDATE table_name
SET column_name = CASE
WHEN condition1 THEN value1
WHEN condition2 THEN value2
...
ELSE value
END;
In this case, condition1
, condition2
… are the conditions you specify, and value1
, value2
… are the values you want to insert when those conditions are true. The ELSE
clause specifies the value to be inserted if none of the conditions are true.
The Power of the CASE Statement for Conditional Updates
The CASE
statement is a powerful tool for conditional updates in SQL. It’s like a Swiss Army knife – it has a tool for every situation. By using the CASE
statement effectively, you can perform complex update operations with ease, making your database management tasks a breeze.
SQL Update’s Row Locking Mechanism
The SQL Update command comes with a built-in safety feature that might not be immediately apparent: a row locking mechanism. This mechanism functions like a traffic controller at a busy intersection – it ensures that only one transaction can modify a row at a time, preventing conflicts and ensuring data integrity.
Here’s how it works: When a transaction initiates an update on a row, the SQL Update command ‘locks’ that row. This means that other transactions are unable to modify that row until the first transaction has completed. Once the first transaction is complete, the lock is released, and other transactions can modify the row.
The Importance of the Row Locking Mechanism
The row locking mechanism plays a crucial role in maintaining database integrity. It’s much like a security guard at a museum – it prevents ‘collisions’ where two transactions attempt to modify the same row simultaneously, which could lead to inconsistent data.
Consider, for example, a situation where two transactions try to update the same row at the same time. Without a row locking mechanism, both transactions could read the original data, make their modifications, and write back their results, overwriting each other’s changes. This could lead to data loss and inconsistencies.
Illustrating the SQL Update Command’s Row Locking Mechanism
Let’s consider an example. Suppose two transactions – Transaction A and Transaction B – try to update the same row in a Customers
table. Here’s what transpires:
- Transaction A initiates the update on the row. The SQL Update command locks the row.
- Transaction B attempts to update the same row but is unable to because the row is locked.
- Transaction A completes updating the row and the SQL Update command releases the lock.
- Transaction B can now update the row.
In this scenario, the row locking mechanism ensures that both transactions can update the row without interfering with each other, maintaining the integrity of the data.
Gaining Proficiency in the Row Locking Mechanism
Gaining proficiency in the row locking mechanism can aid you in managing database concurrency and enhancing performance during updates. It’s akin to learning to drive a manual car – once you’ve mastered it, you can handle any driving situation. By understanding how the row locking mechanism works, you can write more efficient SQL Update commands, avoid conflicts, and maintain the integrity of your data.
Wrapping Up
The SQL Update command is a powerful tool in the world of database management, akin to a Swiss Army knife with its versatility and capability to perform a variety of tasks. From updating a single column in a single row to updating multiple columns in multiple rows, the abilities of the SQL Update command are truly impressive.
Throughout this article, we’ve delved into the syntax of the SQL Update command and highlighted the crucial role of the WHERE clause in targeting updates. We’ve also explored how the SQL Update command can update multiple columns and rows simultaneously, and how the CASE statement can be used for conditional updates.
An additional layer of complexity was added when we delved into the row locking mechanism of the SQL Update command. This built-in safety feature, much like a traffic controller at a busy intersection, ensures data integrity by preventing conflicts, ensuring that each transaction gets its turn to update a row.
By mastering the SQL Update command, you’re not only keeping your databases up-to-date and accurate, but also improving their performance and handling complex update operations with ease. Keep practicing, keep learning, and you’ll soon find that you’ve become an SQL Update command pro! So, remember, the SQL Update command is not just a tool, it’s your key to efficient and effective database management.