Python Inheritance Explained: Complete Guide
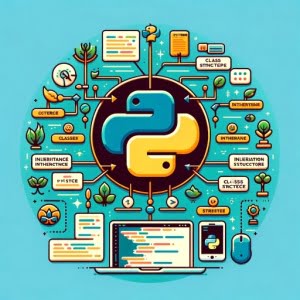
Ever found yourself puzzled by the concept of inheritance in Python? You’re not alone. Many developers, especially those new to object-oriented programming, find this concept a bit challenging.
Think of Python’s inheritance like a family tree – it allows classes to inherit attributes and methods from each other, creating a hierarchy of classes that share common features.
This guide will provide a clear understanding of Python inheritance, from basic use to advanced techniques. We’ll cover everything from the syntax for creating parent and child classes, different types of inheritance in Python, to alternative approaches and common issues.
Let’s get started!
TL;DR: What is Inheritance in Python?
Inheritance is a fundamental concept in Python’s object-oriented programming. It allows a class, known as the child class, to inherit the attributes and methods of another class, referred to as the parent class. This mechanism promotes code reusability and logical organization of code.
Here’s a basic example of how inheritance works in Python:
class Parent:
pass
class Child(Parent):
pass
# In this example, Child is a subclass of Parent and inherits its attributes and methods.
In the above code, we define a Parent
class and a Child
class. The Child
class is defined as a subclass of the Parent
class, meaning it inherits all of its attributes and methods. In this case, since the Parent
class doesn’t have any defined attributes or methods, the Child
class doesn’t inherit anything. But if there were any, the Child
class would have access to them.
This is just the tip of the iceberg when it comes to Python inheritance. There’s a lot more to learn, including different types of inheritance and how to use them effectively. So, keep reading for a more in-depth explanation and advanced usage scenarios.
Table of Contents
- Python Inheritance: The Basics
- Digging Deeper: Types of Python Inheritance
- Exploring Alternatives to Python Inheritance
- Troubleshooting Python Inheritance: Common Issues and Solutions
- Understanding Python’s Object-Oriented Programming
- Python Inheritance in Real-World Applications
- Wrapping Up: Mastering Python Inheritance
Python Inheritance: The Basics
In Python, inheritance is a mechanism that allows one class to inherit the properties and methods of another class. This is a powerful feature of object-oriented programming that promotes code reusability and logical organization.
Let’s start with the basic syntax for creating parent and child classes in Python.
Syntax for Creating Parent and Child Classes
In Python, you create a parent (or base) class just like any other class. However, when creating a child (or derived) class, you need to pass the parent class as a parameter during the declaration of the child class. Here’s how you can do it:
class Parent: # Creating the parent class
def greet(self):
print('Hello from the Parent class!')
class Child(Parent): # Creating the child class
pass
# Creating an instance of the Child class
child = Child()
child.greet()
# Output:
# 'Hello from the Parent class!'
In this example, we created a Parent
class with a greet
method. We then created a Child
class that inherits from the Parent
class. As a result, the Child
class has access to the greet
method of the Parent
class.
This is the simplest form of inheritance in Python. However, Python’s inheritance model allows for more complex scenarios, which we’ll explore in the next sections.
Digging Deeper: Types of Python Inheritance
As you gain more experience with Python and object-oriented programming, you’ll encounter different types of inheritance. Each type has its own use case and can be used to solve different kinds of problems. Let’s take a closer look at these types and understand them with some code examples.
Single Inheritance
Single inheritance is the simplest type of inheritance where a class inherits from a single superclass. We’ve already seen an example of this in the previous section. Here’s another example:
class Parent:
def greet(self):
print('Hello from the Parent class!')
class Child(Parent):
def greet(self):
super().greet()
print('Hello from the Child class!')
# Creating an instance of the Child class
child = Child()
child.greet()
# Output:
# 'Hello from the Parent class!'
# 'Hello from the Child class!'
In this code, the Child
class inherits from the Parent
class and overrides the greet
method. However, it still calls the greet
method of the Parent
class using the super()
function.
Multiple Inheritance
Multiple inheritance is when a class can inherit from more than one parent class. This is a powerful feature but can lead to a lot of confusion if not used carefully.
class Father:
def skills(self):
print('Programming and Cooking')
class Mother:
def skills(self):
print('Art and Teaching')
class Child(Father, Mother):
pass
# Creating an instance of the Child class
child = Child()
child.skills()
# Output:
# 'Programming and Cooking'
In this example, the Child
class inherits from both the Father
and Mother
classes. However, since the Father
class is listed first in the inheritance list, its skills
method is the one that gets called.
Multilevel Inheritance
Multilevel inheritance refers to a scenario where a subclass is derived from a derived class.
class Grandparent:
def greet(self):
print('Hello from the Grandparent class!')
class Parent(Grandparent):
pass
class Child(Parent):
pass
# Creating an instance of the Child class
child = Child()
child.greet()
# Output:
# 'Hello from the Grandparent class!'
In this example, the Child
class inherits from the Parent
class, which in turn inherits from the Grandparent
class. This is an example of multilevel inheritance.
Hierarchical Inheritance
Hierarchical inheritance is when one class serves as a superclass (base class) for more than one subclass.
class Parent:
def greet(self):
print('Hello from the Parent class!')
class Child1(Parent):
pass
class Child2(Parent):
pass
# Creating instances of the Child classes
child1 = Child1()
child2 = Child2()
child1.greet()
child2.greet()
# Output:
# 'Hello from the Parent class!'
# 'Hello from the Parent class!'
In this example, both Child1
and Child2
classes inherit from the Parent
class. This is an example of hierarchical inheritance.
These are some of the advanced uses of inheritance in Python. However, inheritance isn’t always the best solution. There are alternative approaches that might be more suitable in certain situations, which we’ll explore in the next sections.
Exploring Alternatives to Python Inheritance
While inheritance is a powerful concept in Python, it’s not the only way to reuse code and create relationships between classes. There are alternative approaches such as composition and aggregation that can sometimes be more appropriate and flexible. Let’s explore these alternatives and understand when to use them.
Composition: Building Complex Objects
Composition is a concept where a class is composed of one or more objects of other classes. In other words, a class can have an object of another class as a member. This allows you to build complex objects by combining simpler ones.
class Engine:
def start(self):
print('Engine starts')
class Car:
def __init__(self):
self.engine = Engine()
def start(self):
self.engine.start()
# Creating an instance of the Car class
car = Car()
car.start()
# Output:
# 'Engine starts'
In this example, the Car
class has an Engine
object as its member. When the start
method of the Car
class is called, it calls the start
method of the Engine
object. This is an example of composition.
Aggregation: A Relationship Between Classes
Aggregation is a concept where one class can have a relationship with another class, but the two classes are still independent of each other.
class Engine:
def start(self):
print('Engine starts')
class Car:
def __init__(self, engine):
self.engine = engine
def start(self):
self.engine.start()
# Creating an instance of the Engine class
engine = Engine()
# Creating an instance of the Car class
# and passing the engine object to it
# Output:
# 'Engine starts'
In this example, the Car
class has a relationship with the Engine
class through aggregation. The Car
class has an Engine
object as its member, but the Engine
object can exist independently of the Car
object.
When to Use Inheritance vs. Alternatives
The choice between using inheritance or alternatives like composition and aggregation depends on the specific requirements of your program. Inheritance is a good choice when there is a clear ‘is-a’ relationship between classes. On the other hand, composition and aggregation are better choices when there is a ‘has-a’ relationship between classes, or when you want to reuse code but there isn’t a clear ‘is-a’ relationship.
Understanding these concepts and knowing when to use each one is a key skill in Python and object-oriented programming. As you gain more experience and work on more complex projects, you’ll develop a better sense of when to use inheritance and when to use alternatives.
Troubleshooting Python Inheritance: Common Issues and Solutions
Python’s inheritance model is powerful but can also lead to several issues if not used carefully. One such issue is the infamous ‘diamond problem’ in multiple inheritance. Let’s explore this issue and discuss how to resolve it.
The Diamond Problem in Python Inheritance
The diamond problem occurs in multiple inheritance when a class inherits from two or more classes that have a common superclass. This can lead to ambiguity because it’s not clear which parent class’s method should be inherited when the child class calls it.
class Grandparent:
def greet(self):
print('Hello from the Grandparent class!')
class Parent1(Grandparent):
def greet(self):
print('Hello from the Parent1 class!')
class Parent2(Grandparent):
def greet(self):
print('Hello from the Parent2 class!')
class Child(Parent1, Parent2):
pass
# Creating an instance of the Child class
child = Child()
child.greet()
# Output:
# 'Hello from the Parent1 class!'
In this example, the Child
class inherits from both Parent1
and Parent2
classes, which in turn inherit from the Grandparent
class. This leads to the diamond problem because it’s not clear which greet
method should be inherited by the Child
class.
Resolving the Diamond Problem
Python resolves the diamond problem using the Method Resolution Order (MRO). The MRO determines the order in which the base classes are searched when executing a method. In Python, the MRO follows the C3 linearization or just simply ‘C3’.
In the above example, the MRO is [Child, Parent1, Parent2, Grandparent]
. This means that when the greet
method is called on a Child
object, Python first looks for the method in the Child
class. If it doesn’t find it, it looks in the Parent1
class, then in the Parent2
class, and finally in the Grandparent
class.
Knowing about these common issues and how to resolve them can save you a lot of time and frustration when working with Python inheritance. As with any tool, the key is to understand how it works and use it appropriately.
Understanding Python’s Object-Oriented Programming
Python is an object-oriented programming (OOP) language, which means it allows for the creation and manipulation of complex data structures known as objects. These objects are instances of classes, which can be thought of as blueprints for creating objects.
Python Classes and Objects
In Python, a class is defined using the class
keyword. A class can have attributes (variables) and methods (functions).
class MyClass:
attribute = 'This is an attribute'
def my_method(self):
print('This is a method')
# Creating an instance of MyClass
my_object = MyClass()
print(my_object.attribute)
my_object.my_method()
# Output:
# 'This is an attribute'
# 'This is a method'
In this example, MyClass
is a class with an attribute attribute
and a method my_method
. We then create an object my_object
of this class and access its attribute and method.
Python Methods and Attributes
Methods are functions defined inside a class. They are used to define the behaviors of an object. Attributes, on the other hand, are variables that store data that belongs to an instance of a class.
Inheritance in Python’s OOP Paradigm
Inheritance is a key concept in Python’s OOP paradigm. It allows a class (child class) to inherit the attributes and methods of another class (parent class). This promotes code reusability and logical organization of code.
Inheritance in Python is implemented by defining a new class, along with the parent class as an argument.
class Parent:
def greet(self):
print('Hello from the Parent class!')
class Child(Parent):
pass
# Creating an instance of Child
c = Child()
c.greet()
# Output:
# 'Hello from the Parent class!'
In this example, the Child
class inherits from the Parent
class, and thus it has access to its greet
method.
Understanding these fundamentals of Python’s OOP paradigm is essential to mastering Python inheritance. With this background, let’s delve deeper into how inheritance is used in real-world Python applications.
Python Inheritance in Real-World Applications
Inheritance is not just a theoretical concept in Python, but it’s widely used in real-world applications. One such example is Django, a high-level Python web framework that encourages rapid development and clean, pragmatic design.
Inheritance in Django Models
In Django, models are a single, definitive source of information about your data. They contain the essential fields and behaviors of the data you’re storing. Django follows the DRY Principle (Don’t Repeat Yourself). The idea is to put all the common attributes and methods into a base class and then have all the models inherit from this base class.
from django.db import models
class Person(models.Model):
first_name = models.CharField(max_length=30)
last_name = models.CharField(max_length=30)
class Employee(Person):
employee_id = models.CharField(max_length=10)
In the above example, Employee
is a model that inherits from the Person
model. This means that the Employee
model has all the fields of the Person
model, plus the employee_id
field.
Polymorphism and Encapsulation in Python
Inheritance is just one of the many concepts of object-oriented programming. Other important concepts include polymorphism and encapsulation.
- Polymorphism allows us to use a single interface with different underlying forms. In Python, polymorphism is used in many ways, including method overloading, method overriding, and operator overloading.
Encapsulation is the process of hiding the real implementation of an application and only exposing the methods and properties that the users need.
Understanding these concepts will give you a deeper understanding of Python’s object-oriented programming paradigm.
Further Resources for Mastering Python Inheritance
Ready to dive deeper into Python inheritance? Here are some resources to help you further your understanding:
- IOFlood’s Python OOP Article – Learn about abstraction in Python OOP to focus on essential details and hide complexity.
Exploring Counter in Python – Master Python Counter techniques for data manipulation and analysis tasks.
The super() Function in Python – Master the Python super() function for elegant method delegation in class hierarchies.
Python’s Official Documentation on Classes – Deep dive into Python’s classes from the official Python documentation.
Real Python’s Guide on Inheritance and Composition – Comprehensive guide on Python inheritance and composition.
Python Course’s Tutorial on Inheritance – Learn about Python inheritance in an easy manner.
These resources provide in-depth explanations and examples that can help you become more proficient in using Python inheritance, whether you’re working on a small project or a large-scale application.
Wrapping Up: Mastering Python Inheritance
In this comprehensive guide, we’ve delved into the world of Python inheritance, a fundamental concept in Python’s object-oriented programming paradigm. We’ve explored how classes inherit attributes and methods from each other, similar to how characteristics are passed down in a family tree.
We began with the basics, learning how to create parent and child classes and understanding the syntax and mechanics of Python inheritance. We then ventured into more advanced territory, exploring different types of inheritance such as single, multiple, multilevel, and hierarchical inheritance, and understanding them with practical code examples.
We tackled common challenges you might face when using Python inheritance, such as the diamond problem in multiple inheritance, providing solutions and workarounds for each issue. We also looked at alternative approaches to inheritance, such as composition and aggregation, and discussed when to use each approach.
Here’s a quick comparison of the different types of inheritance we’ve discussed:
Type of Inheritance | Description | Use Case |
---|---|---|
Single Inheritance | A class inherits from a single superclass | When there is a simple ‘is-a’ relationship |
Multiple Inheritance | A class inherits from more than one parent class | When a class shares features with multiple superclasses |
Multilevel Inheritance | A subclass is derived from a derived class | When there is a chain of ‘is-a’ relationships |
Hierarchical Inheritance | One class serves as a superclass for more than one subclass | When multiple classes share features with a single superclass |
Whether you’re a beginner just starting out with Python inheritance or an experienced developer looking to solidify your understanding, we hope this guide has given you a deeper understanding of Python inheritance and its practical applications. Keep exploring, keep learning, and happy coding!