Python Counter Quick Reference Guide
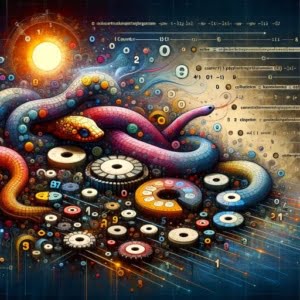
Navigating the world of Python programming can often feel like a daunting task, particularly when dealing with large datasets and complex data structures. But what if there was a way to simplify these tasks?
Meet Python’s Counter class – a hidden gem in Python’s collections module. It’s a powerful tool that can help you manage and count your data efficiently. Whether you’re a Python newbie or an experienced programmer looking for a refresher, this guide is for you. Let’s dive in and master the Python Counter!
TL;DR: What is Python’s Counter class?
Python’s Counter class is a dict subclass in the collections module designed for counting hashable objects. It simplifies the task of counting data, making your code cleaner and more efficient. Here’s a simple example:
from collections import Counter
myList = ['apple', 'banana', 'apple', 'banana', 'apple', 'orange']
counter = Counter(myList)
print(counter)
# Output: `Counter({'apple': 3, 'banana': 2, 'orange': 1})`
This code will show the count of each item in the list. Read on for more advanced methods, background, tips, and tricks.
Table of Contents
Basics of Python Counter
In the vast universe of Python, the Counter class is a hidden gem that often goes unnoticed by many programmers. But what exactly is this Python Counter class? It’s a dict subclass nestled within Python’s collections module, specifically designed for counting hashable objects.
The Counter class is equipped with several built-in functions that make it a handy tool for Python programmers. Here are some of the core functions:
- count(): This function allows you to count the number of occurrences of each item in a list, tuple, or string. Let’s see this function in action with an example:
from collections import Counter
myList = ['apple', 'banana', 'apple', 'banana', 'apple', 'orange']
counter = Counter(myList)
print(counter)
# Output: Counter({'apple': 3, 'banana': 2, 'orange': 1})
This shows that ‘apple’ appears three times, ‘banana’ twice, and ‘orange’ once in the list.
- elements(): This function returns an iterator that produces all the elements in the Counter object that have counts greater than zero.
Here’s an example of how you can use the elements() function with a Counter object in Python:
from collections import Counter
# Define a Counter
counter = Counter({'red': 3, 'blue': 2, 'green': 1})
# Get an iterator over the elements
iterator = counter.elements()
for element in iterator:
print(element)
When you run this code, the output will be:
red
red
red
blue
blue
green
Take into account that the order of elements can vary as they are returned in the order they are encountered in the original input. For collections with equal counts, the order is arbitrary.
- most_common([n]): This function returns a list of the n most common elements and their counts from the most common to the least. If n is omitted or None, it returns all elements.
Example of most_common() function:
from collections import Counter
myList = ['apple', 'banana', 'apple', 'banana', 'apple', 'orange']
counter = Counter(myList)
print(counter.most_common(2))
# Output: [('apple', 3), ('banana', 2)]
This code shows the two most common items in the list.
Why Use Python Counter
Why should you consider using the Counter class? The Counter class provides an efficient and straightforward way to count hashable objects in Python. Instead of writing several lines of code to count objects, you can achieve the same result with just a few lines using the Counter class. This not only saves you time but also makes your code cleaner and easier to read.
Moreover, the Counter class shines brightly when handling large datasets. This makes it an indispensable tool for data analysis tasks where you need to count and manage vast amounts of data. So, whether you’re working on a small project or grappling with a large dataset, the Counter class can be a game-changer in your Python programming journey.
Advanced Methods of Python Counter
While the basics of Python Counter are undoubtedly useful, this powerful class has much more to offer. It’s time to delve deeper and explore some of the more advanced functions of the Counter class.
Two of the most useful advanced methods in the Counter class are subtract()
and update()
. Let’s take a closer look at these methods.
- subtract([iterable-or-mapping]): This method subtracts count, but keeps only results with positive counts. Here’s an example:
from collections import Counter
c1 = Counter(a=3, b=2, c=1)
c2 = Counter(a=1, b=2, c=3)
c1.subtract(c2)
print(c1)
When you run this code, it will output: Counter({'a': 2, 'b': 0, 'c': -2})
. This shows that the counts of ‘b’ are equal in both counters, the count of ‘a’ in c1 is 2 more than in c2, and the count of ‘c’ in c2 is 2 more than in c1.
- update([iterable-or-mapping]): This method is used to add counts from an iterable or from another mapping (or counter). Rather than replacing the count, it adds to the count.
Example of update() function:
from collections import Counter
c1 = Counter(a=3, b=2, c=1)
c2 = Counter(a=1, b=2, c=3)
c1.update(c2)
print(c1)
# Output: Counter({'a': 4, 'b': 4, 'c': 4})
This shows that the counts of ‘a’, ‘b’, and ‘c’ in c1 have been updated with the counts from c2.
The Counter Class and Other Python Data Structures
The Counter class is a subclass of the dict class, which means it inherits all the methods of the dict class. This relationship allows you to use the Counter class seamlessly with other Python data structures. For example, you can use the Counter class with lists, tuples, and strings to count the number of occurrences of each element.
The Counter class is incredibly versatile and can be used in a wide range of programming scenarios. Whether you’re analyzing text data, counting the frequency of items in a list, or working with large datasets, the Counter class can be a powerful tool in your Python toolkit.
Troubleshooting Python Counter: Common Errors
Like any tool, the Python Counter class can present its fair share of challenges. However, with some knowledge and handy tips, you can overcome these obstacles and use the Counter class more efficiently.
Unhashable Objects
One common error encountered when using the Counter class is attempting to count unhashable objects. Remember, the Counter class only works with hashable objects, like strings, integers, and tuples.
If you try to count unhashable objects, like lists or dictionaries, Python will throw a TypeError. The solution? Always ensure you’re using hashable objects with the Counter class.
Here’s a code example where we try to count unhashable objects (that throws an error), and then a corrected version using hashable objects:
from collections import Counter
# Trying to count a list of lists.
try:
list_of_lists = [['apple', 'banana'], ['apple', 'banana'], ['grape', 'apple']]
counter = Counter(list_of_lists)
except TypeError as e:
print("Error: ", e)
# Output: Error: unhashable type: 'list'
Here is the corrected code:
# Correct way: Count a list of tuples. Tuples are hashable
list_of_tuples = [('apple', 'banana'), ('apple', 'banana'), ('grape', 'apple')]
counter_corrected = Counter(list_of_tuples)
for item, frequency in counter_corrected.items():
print(f"Item: {item}, Frequency: {frequency}")
# Output:
# Item: ('apple', 'banana'), Frequency: 2
# Item: ('grape', 'apple'), Frequency: 1
TypeError: ‘Counter’ object is not callable
This error occurs when you try to call the Counter class as a function. To fix this, remember that the Counter class needs to be instantiated with a list, tuple, or string.
Below is a code example where a Counter
object is mistakenly used as a function, resulting in an error, and then the corrected version:
from collections import Counter
# Trying to use a Counter object as a function
try:
counter = Counter(['apple', 'banana', 'apple', 'banana', 'grape', 'apple'])
counter('apple') # This will raise an error
except TypeError as e:
print("Error: ", e)
# Output: Error: 'Counter' object is not callable
Here’s the corrected code:
# Correct way: Use the Counter object as a dictionary
counter_corrected = Counter(['apple', 'banana', 'apple', 'banana', 'grape', 'apple'])
print(counter_corrected['apple']) # This will give you the count of 'apple'
# Output: 3
AttributeError: ‘Counter’ object has no attribute ‘x’
This error occurs when you try to access an attribute or method that doesn’t exist in the Counter class. To fix this, ensure you’re using only the methods and attributes provided by the Counter class.
Here’s an example where an attempt is made to access a nonexistent attribute in a Counter
object, resulting in an error, and then a corrected version:
from collections import Counter
# Trying to access a nonexistent attribute
try:
counter = Counter(['apple', 'banana', 'apple', 'banana', 'grape', 'apple'])
counter.nonexistent_attribute # This will raise an error
except AttributeError as e:
print("Error: ", e)
# Output: Error: 'Counter' object has no attribute 'nonexistent_attribute'
This code will throw an AttributeError because ‘nonexistent_attribute’ is not a valid attribute of the Counter class. To fix this, you should only access valid attributes and methods of the Counter class.
Here’s the corrected example:
# Correct way: Access an attribute that exists
counter_corrected = Counter(['apple', 'banana', 'apple', 'banana', 'grape', 'apple'])
print(counter_corrected.most_common(1)) # This will give you the most common element
# Output: [('apple', 3)]
Tips for Efficient Usage of the Counter Class
The Counter class is a subclass of the dict class in Python. This means it inherits all the methods and properties of the dict class. Understanding this relationship can help you use the Counter class more effectively. For example, you can use dict methods, like keys(), values(), and items(), with a Counter object.
Here are some tips to help you use the Counter class more efficiently:
Use the right data structure
The Counter class works best with hashable objects. If you’re working with unhashable objects, consider converting them to a hashable type before using the Counter class.
Leverage built-in methods
The Counter class comes with several built-in methods, like most_common() and subtract(). Make the most of these methods to simplify your code and improve efficiency.
Understand the output
The Counter class returns a dictionary. Make sure you’re comfortable working with dictionaries and understand how to access the elements you need.
Other Classes in the Python Collections Module
While the Counter class is a powerful tool in its own right, it’s part of a larger context – Python’s collections module. This module is a treasure trove of high-performance container datatypes that can make your Python programming tasks easier and more efficient.
The collections module is a built-in Python module that implements specialized container datatypes providing alternatives to Python’s general-purpose built-in containers like dict, list, set, and tuple. Some of the most commonly used classes in the collections module, besides Counter, include OrderedDict, defaultdict, namedtuple, and deque.
OrderedDict
This is a dictionary subclass that remembers the order in which its contents are added. This is useful in situations where the order of insertion matters.
from collections import OrderedDict
# 1. OrderedDict
print("1. OrderedDict:")
ordered_dict = OrderedDict()
ordered_dict['one'] = 1
ordered_dict['two'] = 2
ordered_dict['three'] = 3
for key, value in ordered_dict.items():
print(key, value)
# Output:
# one 1
# two 2
# three 3
defaultdict
This is a dictionary subclass that calls a factory function to supply missing values. This can be helpful when you’re working with keys that might not exist in the dictionary.
from collections import defaultdict
# 2. defaultdict
print("\n2. defaultdict:")
default_dict = defaultdict(int)
default_dict['one']
default_dict['two'] = 2
for key, value in default_dict.items():
print(key, value)
# Output:
# one 0
# two 2
namedtuple
This factory function creates tuple subclasses with named fields. This can be useful when you’re working with large tuples and want to make your code more readable.
from collections import namedtuple
# 3. namedtuple
print("\n3. namedtuple:")
Point = namedtuple('Point', ['x', 'y'])
p = Point(11, y=22) # instantiate with positional or keyword arguments
print(p.x, p.y)
# Output:
# 11 22
deque
This is a list-like container with fast appends and pops on either end. This is useful when you’re implementing queues or breadth-first tree searches.
from collections import deque
# 4. deque
print("\n4. deque:")
de = deque([1,2,3])
de.append(4) # add to the right
de.appendleft(0) # add to the left
print(de)
# Output:
# deque([0, 1, 2, 3, 4])
Practical Applications of Python Counter
The Python Counter class isn’t just a theoretical concept; it has numerous practical applications in the real world. From data analysis and machine learning to web development and beyond, the Counter class is a versatile tool that can be used in a variety of fields.
Python Counter in Data Analysis and Machine Learning
In the realm of data analysis and machine learning, the Counter class is often used to count the frequency of elements in a dataset. For example, you might use it to count the number of times each word appears in a text document, or to count the number of occurrences of each category in a categorical variable.
Let’s consider a more specific example of how you might use the Counter class in data analysis. Imagine you’re working on a project where you need to analyze customer reviews for a product. You could use the Counter class to count the frequency of positive and negative words in the reviews, helping you determine the overall sentiment towards the product.
Here’s a simplified example of how you might do this:
from collections import Counter
# List of words from customer reviews
words = ['excellent', 'bad', 'excellent', 'great', 'great', 'bad', 'excellent', 'great']
# Use Counter to count the frequency of each word
counter = Counter(words)
# Print the frequency of each word
print(counter)
When you run this code, it will output: Counter({'excellent': 3, 'great': 3, 'bad': 2})
. This shows that ‘excellent’ and ‘great’ each appear three times, while ‘bad’ appears twice.
Python Counter in Web Development
In web development, the Counter class can be used in a variety of ways. For example, you might use it to count the number of hits on a website, or to count the number of occurrences of certain keywords in a web page. You could also use it to analyze user behavior, such as tracking the most visited pages on a website or the most clicked buttons.
The Importance of Mastering Python’s Built-in Classes
Mastering Python’s built-in classes like Counter is crucial for any aspiring Python programmer. These classes provide powerful tools that can simplify complex tasks and make your code more efficient.
By mastering these classes, you can take your Python programming skills to the next level and open up a world of opportunities in fields like data analysis, machine learning, web development, and more.
Further Info for Python Classes
For more insights on Python OOP and how to define and use classes in Python for creating blueprints of objects, Click Here.
And for more resources on working with Python Classes, consider the following:
- Understanding Classes in Python – Learn how to define and instantiate classes in Python for object-oriented programming.
Class Hierarchy with Inheritance – Learn how to implement inheritance in Python for building flexible and scalable code.
Python Classes YouTube Tutorial – Stream this video tutorial for a detailed guide to Python classes.
Dataquest’s Guide on Using Classes in Python – Learn how to use classes with hands-on examples.
Codecademy’s Python Classes Documentation – Comprehensive reference & guide to Python classes.
Concluding Thoughts:
Mastering the Counter class presents numerous benefits. It simplifies the task of counting hashable objects, making your code cleaner and more efficient. It’s versatile, with a wide range of applications in fields like data analysis, machine learning, and web development. However, like any tool, it’s not without its pitfalls. Understanding its limitations, such as its inability to count unhashable objects, is key to using it effectively.
The Counter class is not an isolated entity. It’s a part of Python’s robust collections module, a treasure trove of high-performance container datatypes. By understanding the Counter class in this broader context, you can leverage the full power of the collections module to handle complex data structures with ease.
In conclusion, the Python Counter class is a powerful, versatile tool that every Python programmer should have in their toolkit. Whether you’re a Python newbie or a seasoned pro, mastering the Counter class can take your programming skills to the next level. So, why wait? Dive in and start exploring the power of Python’s Counter class today!