JDBC Connections in Java: Best Practices and Optimization
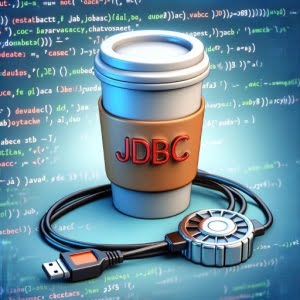
Are you finding it hard to establish a JDBC connection in Java? You’re not alone. Many developers find themselves in a similar situation, but there’s a solution.
Think of JDBC as a bridge that connects your Java application to a database. It’s a crucial link that enables your application to interact with the data stored in a database.
In this guide, we’ll walk you through the process of establishing a JDBC connection in Java, from the basic steps to more advanced techniques. We’ll cover everything from loading the database driver, defining the connection URL, to calling the DriverManager.getConnection()
method and beyond.
So, let’s dive in and start mastering JDBC connections in Java!
TL;DR: How Do I Establish a JDBC Connection in Java?
To establish a JDBC connection in Java, you need to load the database driver with
Class.forName("com.mysql.jdbc.Driver");
, define the connection URL with a string, such asString url = "jdbc:mysql://localhost:3306/mydatabase";
, and call theDriverManager.getConnection()
method.
Here’s a simple example:
import java.sql.*;
public class Main {
public static void main(String[] args) {
try {
Class.forName("com.mysql.jdbc.Driver");
Connection con = DriverManager.getConnection(
"jdbc:mysql://localhost:3306/mydatabase", "username", "password"
);
System.out.println("Connection established successfully");
} catch(Exception e) {
System.out.println(e);
}
}
}
# Output:
# Connection established successfully
In this example, we first load the MySQL JDBC driver using Class.forName()
. Then, we establish a connection to the database ‘mydatabase’ running on localhost using DriverManager.getConnection()
. The connection details include the database URL, username, and password. If the connection is successful, it prints ‘Connection established successfully’.
This is a basic way to establish a JDBC connection in Java, but there’s much more to learn about handling database transactions, using different JDBC drivers, and connecting to different types of databases. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Establishing a JDBC Connection: The Basics
- Diving Deeper: JDBC Drivers and Database Types
- JDBC Alternatives: JPA and Hibernate
- Solving Common JDBC Connection Issues
- Best Practices and Optimization
- Understanding the JDBC API
- JDBC Connection: The Bigger Picture
- Wrapping Up: Mastering JDBC Connections in Java
Establishing a JDBC Connection: The Basics
Establishing a JDBC connection in Java is a straightforward process. It involves three main steps: loading the database driver, defining the connection URL, and calling the DriverManager.getConnection()
method. Let’s break down each step.
Loading the JDBC Driver
The first step is to load the JDBC driver. The driver acts as an interface between the Java application and the database, allowing the two to communicate. Here’s how you load the JDBC driver:
Class.forName("com.mysql.jdbc.Driver");
In this line of code, Class.forName()
loads the JDBC driver class. The string "com.mysql.jdbc.Driver"
is the class name of the MySQL JDBC driver. Loading the driver class automatically registers it with DriverManager
, a service that handles loading drivers and connecting to databases.
Defining the Connection URL
Next, we define the connection URL. The URL specifies the location of the database we want to connect to. Here’s an example:
String url = "jdbc:mysql://localhost:3306/mydatabase";
In this code, "jdbc:mysql://localhost:3306/mydatabase"
is the connection URL. It tells the JDBC driver that we want to connect to a MySQL database located at localhost
on port 3306
. The database name is mydatabase
.
Establishing the Connection
Finally, we call DriverManager.getConnection()
to establish the connection. Here’s how:
Connection con = DriverManager.getConnection(url, "username", "password");
In this line of code, DriverManager.getConnection()
attempts to establish a connection to the database. It takes three arguments: the connection URL, the database username, and the database password. If the connection is successful, it returns a Connection
object.
Here’s the complete code:
import java.sql.*;
public class Main {
public static void main(String[] args) {
try {
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/mydatabase";
Connection con = DriverManager.getConnection(url, "username", "password");
System.out.println("Connection established successfully");
} catch(Exception e) {
System.out.println(e);
}
}
}
# Output:
# Connection established successfully
In this example, we first load the MySQL JDBC driver, define the connection URL, and then establish the connection. If the connection is successful, it prints ‘Connection established successfully’.
Diving Deeper: JDBC Drivers and Database Types
As you become more comfortable with JDBC, you’ll find that different scenarios may require different JDBC drivers or connecting to various types of databases. Let’s dive into these advanced topics.
Exploring Different JDBC Drivers
There are four types of JDBC drivers: JDBC-ODBC bridge driver, Native-API driver, Network Protocol driver, and Thin driver. Each has its strengths and weaknesses, and the choice depends on your specific needs. For example, the Thin driver is a pure Java driver that can communicate directly with the database. This makes it a versatile choice for any Java application.
Here’s how you might load a Thin driver for Oracle database:
Class.forName("oracle.jdbc.driver.OracleDriver");
In this code, we’re loading the Oracle Thin driver instead of the MySQL driver we used in the basic example.
Connecting to Different Databases
JDBC allows you to connect to virtually any relational database. The process is similar to what we’ve already covered; you just need to adjust the driver and connection URL for the specific database. For instance, here’s how you might connect to a PostgreSQL database:
Class.forName("org.postgresql.Driver");
String url = "jdbc:postgresql://localhost:5432/mydatabase";
Connection con = DriverManager.getConnection(url, "username", "password");
In this example, we’re loading the PostgreSQL driver and connecting to a PostgreSQL database running on localhost on port 5432.
Delving into Connection Pools
Connection pools can significantly improve the performance of your Java applications. A connection pool is a cache of database connections that can be reused, eliminating the overhead of opening a new connection every time the database is accessed.
Here’s a simple example of using a connection pool with the HikariCP library:
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase");
config.setUsername("username");
config.setPassword("password");
HikariDataSource ds = new HikariDataSource(config);
Connection con = ds.getConnection();
In this example, we’re creating a HikariCP connection pool and getting a connection from it. The connection pool handles opening and closing connections, allowing your application to run more efficiently.
Handling Database Transactions
Transactions are a fundamental part of working with databases. They ensure data integrity by treating a sequence of database operations as a single unit. In JDBC, you can control transactions with the commit()
and rollback()
methods of the Connection
class.
Here’s a simple example of a transaction:
con.setAutoCommit(false);
Statement stmt = con.createStatement();
stmt.executeUpdate("INSERT INTO students (name) VALUES ('John')");
stmt.executeUpdate("INSERT INTO students (name) VALUES ('Jane')");
con.commit();
In this example, we’re turning off auto-commit mode, executing two INSERT
statements, and then committing the transaction. If an error occurred during any of the INSERT
statements, we could call con.rollback()
to undo all changes made in the current transaction.
JDBC Alternatives: JPA and Hibernate
While JDBC is a powerful tool for interacting with databases in Java, it’s not the only option. Other technologies like Java Persistence API (JPA) and Hibernate provide additional features and can simplify database operations. Let’s explore these alternatives.
Java Persistence API (JPA)
JPA is a specification that standardizes the way Java objects are mapped to database tables. It simplifies database operations by allowing you to work with Java objects instead of SQL statements.
Here’s an example of using JPA to insert a new record:
EntityManagerFactory emf = Persistence.createEntityManagerFactory("my-pu");
EntityManager em = emf.createEntityManager();
em.getTransaction().begin();
Student student = new Student();
student.setName("John");
em.persist(student);
em.getTransaction().commit();
em.close();
emf.close();
In this example, we’re creating an EntityManager
, which is the primary interface for JPA operations. We then start a transaction, create a new Student
object, and persist it to the database with em.persist()
. Finally, we commit the transaction and close the EntityManager
.
Hibernate
Hibernate is an implementation of the JPA specification that also provides additional features. It offers a powerful query language, caching, and performance tuning options.
Here’s an example of using Hibernate to retrieve a list of students:
SessionFactory sf = new Configuration().configure().buildSessionFactory();
Session session = sf.openSession();
List<Student> students = session.createQuery("FROM Student").list();
for (Student student : students) {
System.out.println(student.getName());
}
session.close();
In this example, we’re creating a Session
, which is the primary interface for Hibernate operations. We then use a Hibernate Query Language (HQL) query to retrieve a list of students and print their names.
Decision-Making Considerations
When deciding between JDBC, JPA, and Hibernate, consider the complexity of your project and your team’s familiarity with these technologies. JDBC gives you full control and is great for complex or performance-critical operations. JPA and Hibernate can simplify development and are excellent for standard CRUD operations. However, they have a steeper learning curve and may be overkill for simple projects.
Solving Common JDBC Connection Issues
While working with JDBC, you may encounter some common issues. Let’s discuss these problems and their solutions.
Issue: Driver Not Found
One common error is java.lang.ClassNotFoundException
. This occurs when the JDBC driver class is not found.
try {
Class.forName("com.mysql.jdbc.Driver");
} catch(ClassNotFoundException e) {
System.out.println("Driver not found");
}
# Output:
# Driver not found
In this example, the system cannot find the MySQL JDBC driver class, resulting in the ClassNotFoundException
.
Solution: Make sure the JDBC driver is in your classpath. If you’re using a build tool like Maven or Gradle, ensure the driver is included in your dependencies.
Issue: Connection Failure
Another common issue is a failure to establish a connection to the database. This can be caused by various factors, including incorrect connection URL, username, or password, or the database server not running.
try {
Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "wrong-username", "wrong-password");
} catch(SQLException e) {
System.out.println("Connection failed");
}
# Output:
# Connection failed
In this example, the connection fails because the username and password are incorrect.
Solution: Check your connection URL, username, and password. Also, ensure the database server is running and accessible.
Best Practices and Optimization
When using JDBC, keep these best practices in mind for optimal performance:
- Close resources: Always close your
ResultSet
,Statement
, andConnection
objects when you’re done with them. This helps to free up database resources. Use connection pools: Connection pools can significantly improve performance by reusing database connections.
Handle exceptions: Always handle SQL exceptions in your code. This can help you troubleshoot issues when they occur.
Use transactions: Use transactions to ensure data integrity. Remember to call
commit()
to save your changes to the database.
Understanding the JDBC API
The JDBC API plays a pivotal role in Java database connectivity. It provides a standard interface for interacting with relational databases, allowing Java applications to execute SQL statements and retrieve results.
The Role of the JDBC Driver
The JDBC driver is the heart of the JDBC architecture. It’s responsible for communicating with the database and translating the API calls into database-specific operations.
Class.forName("com.mysql.jdbc.Driver");
In this example, we’re loading the MySQL JDBC driver. This driver knows how to interact with MySQL databases and translates our JDBC API calls into MySQL-specific commands.
The loaded driver class registers itself with the DriverManager
class, which is responsible for managing a list of database drivers.
DriverManager: The Connection Factory
The DriverManager
class acts as a factory for connections to the database. It keeps track of the drivers that are available and handles establishing connections with the database.
Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
In this example, we’re asking the DriverManager
to establish a connection to the MySQL database at localhost:3306
. The DriverManager
selects an appropriate driver from the ones it manages and establishes the connection.
Understanding the roles of the JDBC API, JDBC driver, and DriverManager
class is crucial for effectively working with databases in Java. They form the foundation of Java database connectivity and enable you to interact with a wide range of databases using a consistent API.
JDBC Connection: The Bigger Picture
Working with JDBC connections is a fundamental skill in Java development, but it’s just one piece of the puzzle. When building larger Java applications, you’ll need to consider other related topics such as database design, SQL, and Object-Relational Mapping (ORM).
JDBC in Larger Applications
In a larger Java application, managing JDBC connections becomes even more critical. You’ll often find yourself dealing with multiple databases, complex transactions, and higher performance demands. This is where connection pools, advanced JDBC features, and alternative technologies like JPA and Hibernate can really shine.
Exploring Related Topics: SQL and ORM
Understanding SQL is crucial for working with JDBC as it is the language you’ll use to interact with the database. You’ll need to write SQL queries to create, read, update, and delete data.
ORM is another important concept. As we’ve discussed, technologies like JPA and Hibernate allow you to work with databases using Java objects, simplifying the development process and increasing productivity.
Database Design Considerations
Good database design is key to building efficient and scalable Java applications. This involves designing tables and relationships, choosing the right data types, and implementing indexes for performance.
Further Resources for Mastering JDBC
To continue your journey in mastering JDBC and related topics, here are some resources that provide more in-depth information:
- Java Interface Fundamentals Covered – Discover best practices for designing and using Java interfaces.
Exploring JPA in Java – Learn about entity classes, relationships, and JPQL (Java Persistence Query Language).
Java Predicate: Guide – Explore Java Predicate for functional-style conditional checks and filtering.
Oracle’s Official JDBC Documentation covers all aspects of JDBC, including advanced features and best practices.
Baeldung’s Guide on JDBC provides a practical introduction to JDBC, complete with code examples and explanations.
Java Persistence with Hibernate offers a deep dive into Hibernate, one of the most popular ORM frameworks for Java.
Wrapping Up: Mastering JDBC Connections in Java
In this comprehensive guide, we’ve journeyed through the process of establishing a JDBC connection in Java. We’ve covered the basics, from loading the JDBC driver and defining the connection URL, to calling the DriverManager.getConnection()
method and beyond.
We began with the basics, providing a step-by-step guide for beginners to establish a JDBC connection in Java. We then moved into more advanced territory, exploring different JDBC drivers, connecting to different types of databases, using connection pools, and handling database transactions.
Along the way, we tackled common challenges you might encounter when establishing a JDBC connection, such as driver not found and connection failure, providing you with solutions and best practices for each issue.
We also looked at alternative approaches to Java database connectivity, delving into the world of JPA and Hibernate. Here’s a quick comparison of these technologies:
Technology | Use Case | Complexity |
---|---|---|
JDBC | Full control, complex operations | High |
JPA | Simplified development, standard CRUD operations | Moderate |
Hibernate | Advanced features, complex applications | High |
Whether you’re just starting out with JDBC or you’re looking to level up your Java database connectivity skills, we hope this guide has given you a deeper understanding of JDBC and its capabilities.
With its balance of control and flexibility, JDBC is a powerful tool for interacting with databases in Java. Now, you’re well equipped to tackle any Java database connectivity challenge. Happy coding!