Java Interface: Implementing Methods and Constants
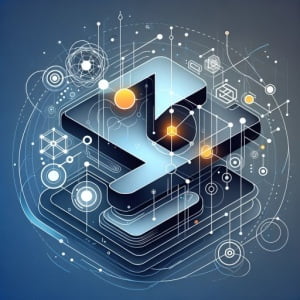
Are Java interfaces giving you a hard time? You’re not alone. Many developers find themselves puzzled when it comes to understanding and implementing interfaces in Java. Think of Java interfaces as a contract for your classes – a contract that ensures certain methods are implemented.
Interfaces are a powerful way to achieve abstraction in Java, making them an essential part of any Java developer’s toolkit.
In this guide, we’ll walk you through the concept of interfaces in Java, from basic usage to advanced techniques. We’ll cover everything from defining and implementing an interface, to more complex uses such as default methods, static methods, and private methods in interfaces. We’ll also discuss alternative approaches, common issues, and their solutions.
So, let’s dive in and start mastering Java interfaces!
TL;DR: What is a Java Interface?
A Java interface is a blueprint of a class, or a contract, that can be used to achieve full abstraction. It can be created by using the interface data type:
interface interfaceName
and it can only contain abstract methods and constants. Here’s a simple example:
interface Animal {
void eat();
void sleep();
}
In this example, we’ve defined a simple Java interface named ‘Animal’. This interface declares two methods: eat()
and sleep()
. Any class that implements this interface will have to provide the implementation for these two methods.
This is just a basic introduction to Java interfaces. There’s much more to learn about them, including advanced usage, alternative approaches, and troubleshooting common issues. Keep reading for a comprehensive guide on mastering Java interfaces.
Table of Contents
- Defining and Implementing Java Interfaces
- Advanced Usage: Default, Static, and Private Methods
- Abstract Classes vs Interfaces: A Comparison
- Troubleshooting Common Issues with Java Interfaces
- Best Practices and Optimization
- Unpacking Abstraction in Java
- Interfaces in Larger Projects: Organization and Manageability
- Wrapping Up: Mastering Java Interfaces
Defining and Implementing Java Interfaces
Java interfaces are a fundamental part of Java programming. They provide a way to ensure that a class adheres to a certain contract. If you’re new to Java or need a refresher, here’s a simple way to define an interface:
interface Drawable {
void draw();
}
In this example, we’ve created an interface named Drawable
. This interface has one method draw()
. It’s important to note that methods in an interface are implicitly public and abstract. They don’t have a body – they are meant to be implemented by the classes that inherit the interface.
Now, let’s see how to implement this interface in a class:
class Circle implements Drawable {
public void draw() {
System.out.println("Drawing a circle");
}
}
Here, we’ve created a class Circle
that implements the Drawable
interface. We’ve provided the implementation for the draw()
method. When this method is called, it prints ‘Drawing a circle’.
To use this class and its method, you can do something like this:
public class Main {
public static void main(String[] args) {
Circle circle = new Circle();
circle.draw();
}
}
// Output:
// Drawing a circle
In this example, we created an instance of the Circle
class and called the draw()
method. The output is ‘Drawing a circle’.
Interfaces are used because they allow for a form of abstraction. They can specify what a class must do, but not how it does it. This is a powerful concept in Java programming.
Advanced Usage: Default, Static, and Private Methods
As you journey deeper into Java interfaces, you’ll encounter more complex uses. Java 8 introduced three new capabilities to interfaces: default methods, static methods, and private methods. Let’s explore each of them.
Default Methods in Interfaces
Default methods allow you to add new methods to an interface without breaking the existing implementation of these interfaces. They include a default implementation that can be overridden by the implementing class. Here’s an example:
interface Drawable {
void draw();
default void print() {
System.out.println("Printing...");
}
}
class Circle implements Drawable {
public void draw() {
System.out.println("Drawing a circle");
}
}
public class Main {
public static void main(String[] args) {
Circle circle = new Circle();
circle.draw();
circle.print();
}
}
// Output:
// Drawing a circle
// Printing...
In this example, we’ve added a default method print()
to the Drawable
interface. The Circle
class implements this interface and inherits the print()
method without providing its implementation.
Static Methods in Interfaces
Static methods in interfaces are similar to static methods in classes. They belong to the interface rather than to the instances of the interface. Here’s an example:
interface Drawable {
void draw();
static int getArea() {
return 100; // Just a placeholder value
}
}
public class Main {
public static void main(String[] args) {
int area = Drawable.getArea();
System.out.println("Area: " + area);
}
}
// Output:
// Area: 100
In this example, we’ve added a static method getArea()
to the Drawable
interface. We can call this method directly on the interface, without creating an instance of it.
Private Methods in Interfaces
Java 9 introduced private methods in interfaces. They can be used to share common code between non-abstract methods. Here’s an example:
interface Drawable {
void draw();
default void print() {
log("Printing...");
}
private void log(String message) {
System.out.println(message);
}
}
class Circle implements Drawable {
public void draw() {
System.out.println("Drawing a circle");
}
}
public class Main {
public static void main(String[] args) {
Circle circle = new Circle();
circle.draw();
circle.print();
}
}
// Output:
// Drawing a circle
// Printing...
In this example, we’ve added a private method log()
to the Drawable
interface. This method is used by the default method print()
. The Circle
class implements this interface and inherits the print()
method.
These advanced features of Java interfaces offer more flexibility and promote code reusability and abstraction.
Abstract Classes vs Interfaces: A Comparison
In Java, besides interfaces, abstract classes are another way to achieve abstraction. Understanding the difference between these two can be crucial for making the right design decisions.
Understanding Abstract Classes
An abstract class in Java is a class that contains one or more abstract methods, which are declared but not implemented. Here’s an example:
abstract class Animal {
abstract void makeSound();
}
class Dog extends Animal {
public void makeSound() {
System.out.println("Woof");
}
}
public class Main {
public static void main(String[] args) {
Dog dog = new Dog();
dog.makeSound();
}
}
// Output:
// Woof
In this example, Animal
is an abstract class with an abstract method makeSound()
. The Dog
class extends Animal
and provides an implementation for makeSound()
.
Comparing Interfaces and Abstract Classes
While both interfaces and abstract classes can be used to achieve abstraction in Java, there are some key differences:
- Default Methods: Both interfaces and abstract classes can have default methods. However, an abstract class can provide a default implementation for all its methods, while an interface can’t (it can only provide default implementations for non-abstract methods).
Multiple Inheritance: A class can implement multiple interfaces but can only extend one abstract class.
State: Abstract classes can have instance variables, while interfaces can only have constants.
Access Modifiers: Methods in an abstract class can have public, private, or protected access modifiers, while methods in an interface are implicitly public.
Choosing between an interface and an abstract class depends on the design requirements of your program. If you need to define a contract for classes without worrying about the state of objects, use an interface. If you need to provide common behavior across classes and care about the state of objects, use an abstract class.
Troubleshooting Common Issues with Java Interfaces
Working with interfaces in Java can sometimes lead to errors or obstacles. Let’s look at some common issues and their solutions.
Attempting to Instantiate an Interface
A common mistake is trying to instantiate an interface. Remember, an interface is a blueprint, not a concrete class. Here’s an example of this mistake:
interface Drawable {
void draw();
}
public class Main {
public static void main(String[] args) {
Drawable d = new Drawable(); // This will cause a compile error
}
}
This code will cause a compile error because you cannot instantiate an interface. The solution is to implement the interface in a class and instantiate that class instead.
Not Implementing All Methods
When a class implements an interface, it must provide implementations for all of the interface’s methods. If it doesn’t, you’ll get a compile error. Here’s an example:
interface Drawable {
void draw();
void erase();
}
class Circle implements Drawable {
public void draw() {
System.out.println("Drawing a circle");
}
// Missing implementation for erase()
}
This code will cause a compile error because the Circle
class doesn’t provide an implementation for the erase()
method. The solution is to implement all methods from the interface.
Best Practices and Optimization
When working with interfaces, here are some tips to follow:
- Use descriptive names: The name of the interface should describe what the classes implementing the interface will do.
Don’t add too many methods: An interface with too many methods can be hard to implement. Try to keep your interfaces small and focused.
Use default methods judiciously: While default methods can be useful, they can also lead to confusion if overused. Use them sparingly and only when necessary.
Remember, interfaces are a powerful feature of Java. With a good understanding and proper usage, they can greatly enhance the design of your code.
Unpacking Abstraction in Java
Abstraction is one of the four fundamental principles of object-oriented programming, along with encapsulation, inheritance, and polymorphism. It’s a process of hiding the implementation details and showing only the functionality to the user.
In Java, abstraction is achieved using either abstract classes or interfaces. As we’ve been discussing, interfaces play a crucial role in this. But why is abstraction so important?
The Power of Abstraction
Abstraction allows you to focus on what an object does instead of how it does it. It provides a simple way to represent complex systems. By hiding the complexity, it makes the code more manageable and reduces the potential for errors.
Here’s a simple example to illustrate this:
interface Vehicle {
void startEngine();
void stopEngine();
}
class Car implements Vehicle {
public void startEngine() {
System.out.println("Starting car engine...");
}
public void stopEngine() {
System.out.println("Stopping car engine...");
}
}
public class Main {
public static void main(String[] args) {
Vehicle myCar = new Car();
myCar.startEngine();
myCar.stopEngine();
}
}
// Output:
// Starting car engine...
// Stopping car engine...
In this example, Vehicle
is an interface with two methods: startEngine()
and stopEngine()
. The Car
class implements this interface and provides the implementation for these methods. When you create a Car
object, you don’t need to know how the engine starts or stops. You only need to know that you can start and stop the engine.
The Benefits of Using Interfaces
Interfaces bring several benefits to Java programming:
- Abstraction: As we’ve seen, interfaces provide a high level of abstraction. They allow you to hide the complexity of the implementation while exposing the methods that can be used.
Loose coupling: Interfaces help reduce the dependency between objects, leading to a loosely coupled system. This makes your code more flexible and easier to maintain and scale.
Multiple inheritance: Java doesn’t support multiple inheritance in classes due to the “Diamond Problem”. However, by using interfaces, you can achieve a form of multiple inheritance.
Polymorphism: Interfaces are a key part of implementing polymorphism in Java. They allow an object to take on many different forms depending on the interface it implements.
In conclusion, mastering interfaces and understanding the concept of abstraction will greatly enhance your efficiency and effectiveness as a Java programmer.
Interfaces in Larger Projects: Organization and Manageability
As you venture into larger projects, the role of interfaces becomes more prominent. They provide a robust structure that makes your code more organized and manageable.
Interfaces and Code Organization
In a large project, organization is key. Interfaces can be used to define a set of methods that a class must implement, thereby establishing a common protocol for classes. This can be particularly useful when your project involves a team of developers, as it ensures everyone adheres to the same set of methods.
Interfaces and Code Manageability
Interfaces also contribute to code manageability. By using interfaces, you can change the implementation of a class without affecting other parts of your code. This makes your code more flexible and easier to maintain.
Related Concepts to Explore
While mastering interfaces is important, there are other related concepts in Java that you may want to explore further:
- Inheritance: This is a mechanism where a new class is derived from an existing class. In Java, classes can inherit fields and methods from one superclass.
Polymorphism: This is the ability of an object to take on many forms. The most common use of polymorphism in OOP occurs when a parent class reference is used to refer to a child class object.
By understanding these concepts, you’ll have a more comprehensive grasp of Java programming.
Further Resources for Mastering Java Interfaces
If you’re looking to deepen your understanding of Java interfaces, here are some resources that you might find useful:
- Essential Java Concepts – Build a solid understanding of core Java to kickstart your journey in Java development.
Predicate Usage in Java – Master Predicate usage for expressive and concise code in Java.
Understanding Java Serializable – Learn how to serialize and deserialize Java objects for data persistence.
Oracle’s Java Interfaces Tutorials provide a comprehensive guide on interfaces, including how to declare use them.
GeeksforGeeks’ Java Interface provides a deep dive into Java interfaces, including their properties advantages.
Baeldung’s A Guide to Java Interfaces explores Java interfaces, default methods, static methods, and private methods.
These resources should provide you with a solid foundation for mastering interfaces in Java.
Wrapping Up: Mastering Java Interfaces
In this comprehensive guide, we’ve delved deep into the world of Java interfaces, unraveling their complexities and showcasing their power in creating robust and flexible code.
We began with the basics, understanding what a Java interface is and how to define and implement it. We then journeyed into more advanced territory, exploring the additional capabilities introduced in Java 8 and 9, such as default methods, static methods, and private methods in interfaces.
We also navigated through the common issues that you might face when working with interfaces and provided practical solutions to overcome these challenges. Moreover, we discussed alternative approaches, comparing the use of interfaces with abstract classes, and provided a clear understanding of when to use each.
Here’s a quick comparison of interfaces and abstract classes:
Concept | Abstraction | Multiple Inheritance | Instance Variables | Default Implementation |
---|---|---|---|---|
Interface | Yes | Yes | No | Only for non-abstract methods |
Abstract Class | Yes | No | Yes | Yes |
As we conclude, whether you’re just starting out with Java interfaces or you’re looking to level up your skills, we hope this guide has provided you with a deeper understanding of Java interfaces and their capabilities.
With its balance of abstraction, loose coupling, and polymorphism, mastering interfaces is a powerful tool for any Java programmer. Now, you’re well-equipped to harness the power of interfaces in your Java programming journey. Happy coding!