PyQt Guide | Learn Python GUI Development
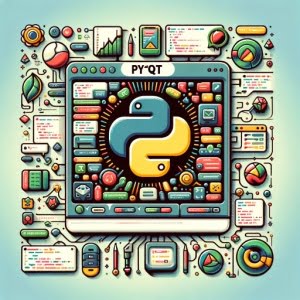
Are you looking to create GUI applications in Python? You’re not alone. Many developers find themselves puzzled when it comes to building desktop applications in Python. But, think of PyQt as a Swiss army knife – versatile and handy for various tasks.
Whether you’re creating simple forms, designing complex layouts, or even integrating database functionality, understanding how to use PyQt can significantly streamline your coding process.
In this guide, we’ll walk you through the process of using PyQt in Python, from the basics to more advanced techniques. We’ll cover everything from installing PyQt, creating your first window, to developing complex GUI applications.
Let’s get started!
TL;DR: What is PyQt and How Do I Use It?
PyQt is a set of Python bindings for The Qt Company’s Qt application framework. It allows you to create GUI applications in Python. Here’s a simple example of creating a basic window:
from PyQt5.QtWidgets import QApplication, QWidget
app = QApplication([])
window = QWidget()
window.show()
app.exec_()
# Output:
# A basic window will appear on your screen
In this example, we import the necessary modules from PyQt5 and create an instance of QApplication and QWidget. The window.show()
command makes the window visible, and app.exec_()
starts the application’s main loop.
This is just the tip of the iceberg when it comes to PyQt. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Getting Started with PyQt: Installation and Basic Use
- Crafting Complex PyQt Applications
- Exploring Alternatives: Tkinter and Kivy
- Addressing PyQt Challenges: Troubleshooting and Considerations
- Unveiling PyQt: The Qt Framework and Python Interface
- Exploring PyQt’s Relevance in Professional Development
- Wrapping Up: Mastering PyQt for Efficient GUI Development
Getting Started with PyQt: Installation and Basic Use
Before we can create GUI applications with PyQt, we first need to install it. The installation process is straightforward and can be done using pip, Python’s package manager. Here’s how:
pip install pyqt5
Once PyQt is installed, we can start building our first basic GUI application. Let’s create a simple window.
from PyQt5.QtWidgets import QApplication, QWidget
app = QApplication([])
window = QWidget()
window.setWindowTitle('My First PyQt App')
window.show()
app.exec_()
# Output:
# A window titled 'My First PyQt App' will appear on your screen
In the above code, we first import the necessary modules from PyQt5. We then create an instance of QApplication, which is the foundation of any PyQt application. A QWidget object represents the window, and we set its title using window.setWindowTitle()
. The window.show()
command makes the window visible, and app.exec_()
starts the application’s main loop, waiting for user interaction.
This basic PyQt application is straightforward and easy to understand, making PyQt a great choice for beginners in GUI development. However, as with any tool, there can be potential pitfalls. It’s important to remember that PyQt applications are event-driven. This means the flow of the program is determined by user actions such as mouse clicks or key presses, which can be a new concept for those used to procedural programming.
Crafting Complex PyQt Applications
As you become more comfortable with PyQt, you can start to explore its more advanced features. Let’s take a look at how to create a more complex GUI application, featuring multiple windows, menus, and buttons.
from PyQt5.QtWidgets import QApplication, QMainWindow, QAction, QTextEdit, QPushButton
def window():
app = QApplication([])
win = QMainWindow()
win.setWindowTitle('Advanced PyQt App')
# Adding a text edit field
textEdit = QTextEdit()
win.setCentralWidget(textEdit)
# Adding a button
button = QPushButton('Click me')
textEdit.setCentralWidget(button)
# Adding a menu
mainMenu = win.menuBar()
fileMenu = mainMenu.addMenu('File')
# Adding an action to the menu
exitButton = QAction('Exit', win)
exitButton.triggered.connect(win.close)
fileMenu.addAction(exitButton)
win.show()
app.exec_()
# Output:
# An advanced window titled 'Advanced PyQt App' with a text edit field, a button, and a menu will appear on your screen
In this code, we introduce several new PyQt concepts. A QMainWindow object is used instead of QWidget, as it provides a framework for building the main user interface of a typical desktop application. We add a QTextEdit widget as the central widget of the QMainWindow, and a QPushButton inside the QTextEdit field.
We also create a menu bar with a ‘File’ menu, inside which we add an ‘Exit’ action. The exitButton.triggered.connect(win.close)
line connects the ‘Exit’ action’s triggered signal to the QMainWindow’s close slot, meaning that selecting ‘Exit’ from the menu will close the application.
Creating complex PyQt applications like this one requires a good understanding of PyQt’s signal and slot mechanism, which is key to developing interactive applications.
Exploring Alternatives: Tkinter and Kivy
While PyQt is a powerful tool for creating GUI applications in Python, it’s not the only game in town. Other libraries, like Tkinter and Kivy, offer alternative approaches. Let’s briefly explore these two alternatives.
Tkinter: Python’s Built-in GUI Package
Tkinter is Python’s standard GUI package and is included with most Python installations. It’s a great option for creating simple applications, and its built-in nature makes it an easy choice for beginners.
Here’s a simple example of creating a basic window with Tkinter:
import tkinter as tk
root = tk.Tk()
root.title('My First Tkinter App')
root.mainloop()
# Output:
# A window titled 'My First Tkinter App' will appear on your screen
In this example, we create a Tk root widget, which is the window for our application. The root.mainloop()
starts the event loop, which waits for user interaction.
Kivy: For Cross-platform Applications
Kivy is an open-source Python library for developing multitouch applications. It’s cross-platform (Linux/OS X/Windows/Android/iOS) and released under the MIT license. It is particularly good for applications that require multi-touch, gestures, and other modern touch features.
Here’s a simple example of creating a basic window with Kivy:
from kivy.app import App
from kivy.uix.button import Button
class MyApp(App):
def build(self):
return Button(text='Hello Kivy')
MyApp().run()
# Output:
# A window with a button labeled 'Hello Kivy' will appear on your screen
In the above Kivy application, we create a Button widget and use the App class to start our application.
While PyQt is a robust and versatile tool, Tkinter and Kivy have their own strengths. Tkinter’s simplicity makes it perfect for beginners, while Kivy’s focus on touch features makes it stand out for certain types of applications. Depending on your project’s requirements, you might find one of these alternatives to be a better fit.
Addressing PyQt Challenges: Troubleshooting and Considerations
Like any software development tool, PyQt comes with its own set of challenges. Whether it’s installation issues, compatibility problems, or understanding error messages, it’s essential to know how to troubleshoot these common problems. Let’s go through some of these issues and their solutions.
Installation Troubles
One of the first hurdles you might encounter is during the installation of PyQt. If you’re having trouble installing PyQt, make sure your pip, the Python package installer, is up-to-date. You can update pip using the following command:
pip install --upgrade pip
If you’re still having trouble, it might be a compatibility issue with your Python version. PyQt5 requires Python 3.5 or later. You can check your Python version using the following command:
python --version
# Output:
# Python 3.x.x
Compatibility Issues
Another common issue is compatibility problems between PyQt and your operating system. PyQt is cross-platform and should work on Linux, macOS, and Windows. However, there might be slight differences in behavior or appearance due to the underlying differences in these operating systems. It’s always a good idea to test your PyQt applications on all target platforms.
Understanding PyQt Error Messages
PyQt error messages can sometimes be cryptic, especially if you’re new to the library. Understanding these messages often requires a good grasp of PyQt’s concepts, like signals and slots, event handling, and the Qt object model. When you encounter an error, take the time to understand what the message is saying. Google is your friend here, and chances are someone else has faced the same issue.
Remember, mastering PyQt or any other programming tool is a journey filled with challenges. But with patience, persistence, and a lot of practice, you’ll find that PyQt is a powerful tool in your Python GUI development toolkit.
Unveiling PyQt: The Qt Framework and Python Interface
To truly understand PyQt, it’s important to learn about its foundation – the Qt framework. Qt is a free and open-source widget toolkit for creating graphical user interfaces as well as cross-platform applications that run on various software and hardware platforms.
Qt is written in C++, and it’s this base that makes PyQt so powerful. PyQt is essentially a set of Python bindings for the Qt libraries, which means that a PyQt application is a blend of Python and Qt’s native C++.
The Architecture of PyQt Applications
When you’re building a PyQt application, you’re building a Qt application. The difference is that you’re using Python, which is easier to learn and use than C++, especially for beginners.
Here’s a simple PyQt application and its breakdown:
from PyQt5.QtWidgets import QApplication, QLabel
app = QApplication([])
label = QLabel('Hello PyQt!')
label.show()
app.exec_()
# Output:
# A window with 'Hello PyQt!' will appear on your screen
In this PyQt application, we begin by importing the necessary modules from PyQt5. We then create an instance of QApplication
. This is a crucial line as every PyQt application must have one QApplication
object. This object is responsible for initialization, finalization, and providing the event loop.
Next, we create a QLabel
object. QLabel is a widget that displays a short piece of text or an image, or nothing at all. We set its text to ‘Hello PyQt!’.
The label.show()
command makes the QLabel visible. Without this, the QLabel would be hidden.
Finally, app.exec_()
starts the application’s event loop. The event loop is an infinite loop that waits for events from the user and sends them to the application’s objects. It ends when we close the QLabel window.
Understanding the architecture of PyQt applications is key to leveraging the full power of PyQt and Qt. It’s this architecture that allows PyQt to provide such a robust toolset for GUI development in Python.
Exploring PyQt’s Relevance in Professional Development
PyQt is not just for hobbyists or beginners. It’s a powerful tool that’s widely used in the industry to create professional desktop applications. From music players to scientific applications, PyQt’s versatility makes it a popular choice for a wide range of software.
Diving Deeper: QML and Qt Designer
As you grow more comfortable with PyQt, you might want to explore related technologies like QML and Qt Designer. QML is a markup language that allows you to design and create responsive, fluid user interfaces. Qt Designer, on the other hand, is a tool for designing and building graphical user interfaces (GUIs) from Qt components.
Further Resources for PyQt Proficiency
To deepen your understanding of PyQt and related technologies, here are some resources that you might find helpful:
- Python GUI Techniques and Tips – Learn how to handle events and user interactions in Python GUIs.
Building GUI Applications in Python with Tkinter – Learn how to design GUI applications with tkinter.
Simplifying GUI Development with PySimpleGUI – Dive into PySimpleGUI’s simplicity and versatility.
Official PyQt5 Documentation – A comprehensive resource covering all aspects of PyQt5.
Qt for Python – The official set of Python bindings for Qt, with tutorials and guides for both beginners and advanced users.
Learn PyQt – A collection of tutorials and ‘cookbook’ recipes for solving specific widget problems.
Remember, mastering PyQt or any programming language or library takes time and practice. Don’t rush the process. Happy coding!
Wrapping Up: Mastering PyQt for Efficient GUI Development
In this comprehensive guide, we’ve explored PyQt, a robust tool for creating GUI applications in Python. From installation to creating complex applications, we’ve covered every step of the journey to mastering PyQt.
We began with the basics, learning how to install PyQt and create a simple window. We then delved into more advanced territory, crafting complex applications with multiple windows, menus, and buttons. Along the way, we tackled common challenges you might encounter when using PyQt, such as installation problems and compatibility issues, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to GUI development in Python, comparing PyQt with other libraries like Tkinter and Kivy. Here’s a quick comparison of these libraries:
Library | Versatility | Ease of Use | Special Features |
---|---|---|---|
PyQt | High | Moderate | Robust toolset, Qt framework |
Tkinter | Moderate | High | Built-in Python library |
Kivy | High | Low | Touch features |
Whether you’re a beginner just starting out with PyQt or an experienced Python developer looking to level up your GUI development skills, we hope this guide has given you a deeper understanding of PyQt and its capabilities.
With its balance of versatility, ease of use, and powerful toolset, PyQt is a valuable tool for GUI development in Python. Happy coding!