PySimpleGUI: Guide to Python GUI Development
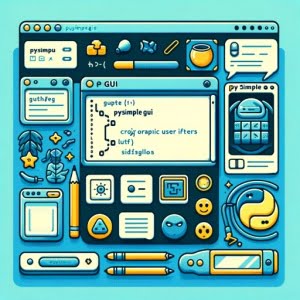
Are you finding it challenging to create a graphical user interface (GUI) in Python? You’re not alone. Many developers find themselves overwhelmed when it comes to GUI development in Python.
But, there’s a tool that can make this process a breeze. Like a master key, PySimpleGUI can unlock the world of GUI development for you.
In this guide, we’ll walk you through the process of using PySimpleGUI, from the basics to more advanced techniques. We’ll cover everything from creating a simple window, adding widgets, handling events, to discussing more advanced features such as creating custom widgets and using themes.
Let’s get started!
TL;DR: What is PySimpleGUI and How Do I Use It?
PySimpleGUI is a Python library that simplifies the process of creating GUIs. With PySimpleGUI, you can create a window with a button using just a few lines of code.
Here’s a simple example:
import PySimpleGUI as sg
layout = [[sg.Button('Hello')]]
window = sg.Window('Demo', layout)
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSED:
break
if event == 'Hello':
print('Hello, World!')
window.close()
# Output:
# 'Hello, World!'
In this example, we import the PySimpleGUI module as sg
. We then define a layout for our window, which includes a single button labeled ‘Hello’. We create a window with this layout, and then enter a loop where we wait for events. If the ‘Hello’ button is clicked, we print ‘Hello, World!’. The loop continues until the window is closed.
This is just a basic introduction to PySimpleGUI. There’s so much more to learn about this powerful library, including how to add different types of widgets, handle events, and create complex layouts. So, stay tuned for a more detailed guide on using PySimpleGUI.
Table of Contents
Getting Started with PySimpleGUI
Before we can start creating GUIs with PySimpleGUI, we first need to install the library. This can be done using pip, the Python package installer. Here’s how you can install PySimpleGUI on your system:
pip install PySimpleGUI
Once installed, we can start using PySimpleGUI to create our GUI.
Creating a Basic Window
The first step in creating a GUI with PySimpleGUI is to create a window. This is done using the sg.Window()
function. Here’s a simple example:
import PySimpleGUI as sg
window = sg.Window('My First Window')
# Output:
# A new window titled 'My First Window' is displayed on the screen
In this example, we import the PySimpleGUI module as sg
and then create a new window titled ‘My First Window’.
Adding Widgets
Widgets are the building blocks of a GUI. They include things like buttons, text inputs, labels, and more. In PySimpleGUI, you add widgets to a window by defining a layout. Here’s an example of how to add a button to a window:
import PySimpleGUI as sg
layout = [[sg.Button('Click Me')]]
window = sg.Window('My First Window', layout)
# Output:
# A new window titled 'My First Window' is displayed on the screen with a button labeled 'Click Me'
In this example, we define a layout that includes a single button labeled ‘Click Me’. We then create a window with this layout.
Handling Events
Events are things like button clicks or key presses. In PySimpleGUI, you handle events using a loop. Here’s an example of how to handle a button click event:
import PySimpleGUI as sg
layout = [[sg.Button('Click Me')]]
window = sg.Window('My First Window', layout)
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSED:
break
if event == 'Click Me':
print('Button clicked!')
window.close()
# Output:
# 'Button clicked!' is printed to the console when the 'Click Me' button is clicked
In this example, we enter a loop where we wait for events. If the ‘Click Me’ button is clicked, we print ‘Button clicked!’ to the console. The loop continues until the window is closed.
This is just the basics of using PySimpleGUI. There’s much more you can do with this powerful library, including creating custom widgets, using themes, and handling complex events. Stay tuned for more advanced topics!
Advanced Features of PySimpleGUI
As you gain more experience with PySimpleGUI, you’ll likely want to start leveraging its more advanced features. These include creating custom widgets, using themes, and handling complex events.
Custom Widgets with PySimpleGUI
Custom widgets allow you to create interactive elements that are uniquely tailored to your application’s needs. Here’s an example of how you can create a custom input box that only accepts numeric input:
import PySimpleGUI as sg
layout = [[sg.InputText(do_not_clear=True, key='-IN-', enable_events=True)]]
window = sg.Window('Custom Input Box', layout)
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSED:
break
if event == '-IN-':
if not values['-IN-'].isdigit():
window['-IN-'].update(values['-IN-'][:-1])
window.close()
# Output:
# A new window titled 'Custom Input Box' is displayed on the screen with an input box that only accepts numeric input
In this example, we create a custom input box that only accepts numeric input. We do this by enabling events for the input box and then checking if the input is a digit each time an event occurs. If the input is not a digit, we update the input box to remove the last character.
Theming with PySimpleGUI
PySimpleGUI also allows you to easily change the look and feel of your GUI using themes. Here’s an example of how to apply a theme to a window:
import PySimpleGUI as sg
sg.theme('DarkAmber') # Add some color to the window
layout = [[sg.Button('Click Me')]]
window = sg.Window('Themed Window', layout)
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSED:
break
window.close()
# Output:
# A new window titled 'Themed Window' is displayed on the screen with a 'DarkAmber' theme
In this example, we apply the ‘DarkAmber’ theme to our window, changing the color of the window and the button.
Handling Complex Events
As your applications become more complex, you’ll likely need to handle more complex events. PySimpleGUI allows you to do this using the window.read()
function. Here’s an example of how to handle a button click event and a key press event at the same time:
import PySimpleGUI as sg
layout = [[sg.Button('Click Me'), sg.InputText(key='-IN-', enable_events=True)]]
window = sg.Window('Complex Events', layout)
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSED:
break
if event == 'Click Me':
print('Button clicked!')
if event == '-IN-':
print('Key pressed!')
window.close()
# Output:
# 'Button clicked!' is printed to the console when the 'Click Me' button is clicked
# 'Key pressed!' is printed to the console when a key is pressed in the input box
In this example, we handle both a button click event and a key press event. We do this by checking the event returned by window.read()
. If the ‘Click Me’ button is clicked, we print ‘Button clicked!’ to the console. If a key is pressed in the input box, we print ‘Key pressed!’ to the console.
These are just a few examples of the advanced features available in PySimpleGUI. By leveraging these features, you can create powerful and interactive GUIs in Python.
Exploring Alternatives to PySimpleGUI
While PySimpleGUI is a powerful tool for creating GUIs in Python, it’s not the only option available. There are several other libraries you can use to create GUIs in Python, including tkinter, PyQt, and wxPython.
tkinter: The Standard GUI Toolkit for Python
tkinter is the standard GUI toolkit for Python. It’s included with Python, which means you don’t need to install anything to use it. However, tkinter’s interface can be a bit more low-level compared to PySimpleGUI, which can make it more challenging for beginners.
PyQt: A Set of Python Bindings for Qt Libraries
PyQt is a set of Python bindings for the Qt libraries, which are used to create cross-platform applications. PyQt is powerful and flexible, but it can be overkill for simple GUI applications.
wxPython: A Wrapper for the wxWidgets C++ Library
wxPython is a wrapper for the wxWidgets C++ library, which is used to create native-looking GUI applications. Like PyQt, wxPython is powerful and flexible, but it can be more challenging to use than PySimpleGUI.
Library | Difficulty Level | Flexibility | Native Look |
---|---|---|---|
PySimpleGUI | Beginner to Intermediate | Moderate | Yes |
tkinter | Intermediate | Moderate | Yes |
PyQt | Intermediate to Advanced | High | Yes |
wxPython | Intermediate to Advanced | High | Yes |
In conclusion, while PySimpleGUI is a fantastic tool for creating GUIs in Python, especially for beginners and intermediate developers, there are other options available depending on your needs. If you need more flexibility or control over your GUI, you might want to consider using PyQt or wxPython. If you’re working on a simple project and don’t want to install any additional libraries, tkinter might be the right choice for you.
As with any tool, there can be challenges and common issues when using PySimpleGUI. In this section, we’ll discuss some of these problems, including layout problems, event handling issues, and cross-platform considerations, and provide solutions and workarounds.
Layout Problems
One common issue is creating complex layouts. For example, you may encounter difficulties when trying to align widgets or create nested layouts. Here’s a common issue:
import PySimpleGUI as sg
layout = [[sg.Button('Button 1'), sg.Button('Button 2')], [sg.Button('Button 3')]]
window = sg.Window('Layout Problem', layout)
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSED:
break
window.close()
# Output:
# A new window titled 'Layout Problem' is displayed on the screen with 'Button 1' and 'Button 2' on the same line and 'Button 3' on a new line
In this example, ‘Button 1’ and ‘Button 2’ are on the same line, but ‘Button 3’ is on a new line. If you want all buttons to be on the same line, you can modify the layout like this:
import PySimpleGUI as sg
layout = [[sg.Button('Button 1'), sg.Button('Button 2'), sg.Button('Button 3')]]
window = sg.Window('Layout Solution', layout)
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSED:
break
window.close()
# Output:
# A new window titled 'Layout Solution' is displayed on the screen with 'Button 1', 'Button 2', and 'Button 3' all on the same line
Event Handling Issues
Another common issue is handling events. For example, you may encounter difficulties when trying to handle multiple events at the same time. Here’s a common issue:
import PySimpleGUI as sg
layout = [[sg.Button('Button 1'), sg.Button('Button 2')]]
window = sg.Window('Event Handling Problem', layout)
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSED:
break
if event == 'Button 1':
print('Button 1 clicked!')
elif event == 'Button 2':
print('Button 2 clicked!')
window.close()
# Output:
# 'Button 1 clicked!' is printed to the console when 'Button 1' is clicked
# 'Button 2 clicked!' is printed to the console when 'Button 2' is clicked
In this example, ‘Button 1’ and ‘Button 2’ can’t be clicked at the same time. If you want to handle multiple events at the same time, you can use threads or multiprocessing.
Cross-Platform Considerations
PySimpleGUI is designed to be cross-platform, but there can still be differences between platforms. For example, the look and feel of your GUI can vary between Windows, Mac, and Linux. To ensure your GUI looks and behaves consistently across platforms, it’s important to thoroughly test your application on all target platforms.
These are just a few of the common issues you might encounter when using PySimpleGUI. However, with a little bit of practice and patience, these challenges can be easily overcome. Remember, the best way to learn is by doing, so don’t be afraid to get your hands dirty and start coding!
Understanding GUI Development in Python
Creating a Graphical User Interface (GUI) is a fundamental part of developing desktop applications. In Python, there are several libraries available for this purpose, but PySimpleGUI stands out for its simplicity and flexibility.
The Role of PySimpleGUI
PySimpleGUI serves as a wrapper around other Python GUI libraries like tkinter, Qt, Remi, and WxPython. This means it simplifies the process of creating GUIs by providing a more Pythonic and straightforward API.
import PySimpleGUI as sg
layout = [[sg.Text('Hello from PySimpleGUI')], [sg.Button('OK')]]
window = sg.Window('Demo', layout)
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSED or event == 'OK':
break
window.close()
# Output:
# A new window titled 'Demo' is displayed on the screen with a text 'Hello from PySimpleGUI' and a button 'OK'
In this code block, we’re creating a simple GUI with a text element and a button. This is a straightforward task with PySimpleGUI, and it demonstrates how it abstracts away much of the complexity associated with GUI development.
Event-Driven Programming in PySimpleGUI
At its core, GUI development is based on an event-driven programming model. This means that the flow of the program is determined by events such as user actions (like mouse clicks or key presses), sensor outputs, or messages from other programs.
PySimpleGUI applies this model by providing an event loop where you can handle these events. Let’s take a look at an example:
import PySimpleGUI as sg
layout = [[sg.Button('Button')]]
window = sg.Window('Event Driven Programming', layout)
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSED:
break
if event == 'Button':
print('Button clicked!')
window.close()
# Output:
# 'Button clicked!' is printed to the console when the 'Button' is clicked
In this example, we create a window with a single button. In the event loop, we wait for two types of events: the window being closed and the button being clicked. When the button event occurs, we print ‘Button clicked!’ to the console. This demonstrates how you can use PySimpleGUI to respond to user interactions in an event-driven manner.
Understanding the fundamentals of GUI development and the event-driven programming model is crucial for effectively using PySimpleGUI. With this knowledge, you’ll be better equipped to create dynamic and interactive applications.
PySimpleGUI in Real-World Projects
While learning to use PySimpleGUI in isolation is great, it’s even more rewarding to see how it can be applied in real-world projects. Whether you’re developing desktop applications, games, or data visualization tools, PySimpleGUI has a lot to offer.
Desktop Applications with PySimpleGUI
PySimpleGUI is a fantastic tool for creating desktop applications. Its simplicity and flexibility make it a great choice for both simple and complex applications.
import PySimpleGUI as sg
layout = [[sg.Text('Enter your name:'), sg.InputText()], [sg.Button('OK')]]
window = sg.Window('Desktop Application', layout)
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSED or event == 'OK':
print(f'Hello, {values[0]}!')
break
window.close()
# Output:
# 'Hello, [Your Name]!' is printed to the console when the 'OK' button is clicked
In this example, we create a simple desktop application that asks the user to enter their name and then greets them when they click the ‘OK’ button.
Game Development with PySimpleGUI
PySimpleGUI can also be used in game development. While it might not be the first choice for complex 3D games, it’s perfect for simpler games like tic-tac-toe or snake.
import PySimpleGUI as sg
layout = [[sg.Button('', key=(i,j)) for j in range(3)] for i in range(3)]
window = sg.Window('Tic-Tac-Toe', layout)
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSED:
break
if event:
window[event].update('X', disabled=True)
window.close()
# Output:
# A new window titled 'Tic-Tac-Toe' is displayed on the screen with a 3x3 grid of buttons
# When a button is clicked, it's label is changed to 'X' and it is disabled
In this example, we create a simple tic-tac-toe game. When a button is clicked, its label is changed to ‘X’ and it is disabled.
Data Visualization with PySimpleGUI
Another great use case for PySimpleGUI is data visualization. By integrating PySimpleGUI with libraries like Matplotlib, you can create interactive data visualizations with ease.
import PySimpleGUI as sg
import matplotlib.pyplot as plt
# Create a simple plot
plt.plot([0, 1, 2, 3], [0, 1, 4, 9])
plt.ylabel('y')
plt.xlabel('x')
# Save the plot to a file
plt.savefig('plot.png')
# Create a PySimpleGUI window with an Image element to display the plot
layout = [[sg.Image(filename='plot.png')]]
window = sg.Window('Data Visualization', layout)
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSED:
break
window.close()
# Output:
# A new window titled 'Data Visualization' is displayed on the screen with an image of the plot
In this example, we create a simple plot using Matplotlib, save it to a file, and then display it in a PySimpleGUI window.
Further Resources for PySimpleGUI Proficiency
If you’re interested in exploring more about PySimpleGUI and how it can be used in your projects, here are a few resources that you might find helpful:
- Python GUI: A Quick Overview – Dive into layout management and design principles in Python.
PyQt: Creating Cross-Platform GUI Applications – Discover PyQt for GUI development in Python.
Simplifying API Development with FastAPI – Learn how FastAPI simplifies API development.
The official PySimpleGUI Cookbook is a great place for practical examples of how to use PySimpleGUI.
Real Python’s guide to creating GUIs in Python with PySimpleGUI provides a comprehensive introduction to PySimpleGUI.
Create an advanced GUI app with PySimpleGUI – This YouTube video provides a visual tutorial on PySimpleGUI.
By leveraging these resources and practicing regularly, you can become proficient in using PySimpleGUI and create amazing GUIs for your Python applications.
Wrapping Up: Mastering PySimpleGUI for Python GUI Development
In this comprehensive guide, we’ve delved into the world of PySimpleGUI, a simple yet powerful tool for creating Graphical User Interfaces (GUIs) in Python.
We began with the basics, exploring how to install PySimpleGUI and create a basic window. We then dove into more advanced topics, such as creating custom widgets, using themes, and handling complex events. Along the way, we tackled common issues you might encounter when using PySimpleGUI, such as layout problems, event handling issues, and cross-platform considerations, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to Python GUI development, comparing PySimpleGUI with other libraries like tkinter, PyQt, and wxPython. Here’s a quick comparison of these libraries:
Library | Difficulty Level | Flexibility | Native Look |
---|---|---|---|
PySimpleGUI | Beginner to Intermediate | Moderate | Yes |
tkinter | Intermediate | Moderate | Yes |
PyQt | Intermediate to Advanced | High | Yes |
wxPython | Intermediate to Advanced | High | Yes |
Whether you’re a beginner just starting out with PySimpleGUI or an experienced Python developer looking to level up your GUI development skills, we hope this guide has given you a deeper understanding of PySimpleGUI and its capabilities.
With its balance of simplicity and flexibility, PySimpleGUI is a powerful tool for GUI development in Python. Happy coding!