FastAPI: Complete Guide to Python Web Development
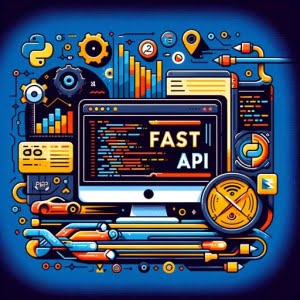
Are you on the hunt for a Python web framework that offers speed, simplicity, and flexibility? Look no further than FastAPI. Often compared to a sports car in the world of Python web frameworks, FastAPI is a modern, high-performance framework that’s perfect for building APIs.
This guide will introduce you to FastAPI, its features, and how to get started with it.
FastAPI is not just about speed, though that’s certainly one of its standout features. It’s also about making your life as a developer easier, with features like automatic interactive API documentation, easy-to-use serialization, and Python 3.6+ type hints support.
Whether you’re a seasoned Python developer or just getting started, FastAPI has something to offer you.
TL;DR: What is FastAPI?
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints. It’s designed to provide a simple and intuitive interface for building robust APIs, while also offering high performance and extensibility.
Here’s a simple example of a FastAPI application:
from fastapi import FastAPI
app = FastAPI()
@app.get('/')
def read_root():
return {'Hello': 'World'}
# Output:
# {'Hello': 'World'}
In this example, we’ve created a basic FastAPI application. We’ve defined a single route (/
) that, when accessed via a GET request, returns a JSON response with the greeting ‘Hello, World’.
This is just a taste of what FastAPI can do. Stay tuned for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with FastAPI
FastAPI is a breeze to start with, even if you’re new to Python or web development. Let’s see how to set up a basic FastAPI application.
First, you’ll need to install FastAPI. You can do this using pip, Python’s package manager:
pip install fastapi
You’ll also need an ASGI server, such as uvicorn, to serve your FastAPI application:
pip install uvicorn
Now, let’s create a new FastAPI app. Create a new Python file (let’s call it main.py
), and add the following code:
from fastapi import FastAPI
app = FastAPI()
@app.get('/')
def read_root():
return {'Hello': 'FastAPI'}
In this code, we’re importing FastAPI, creating a new FastAPI application, and defining a route (the root route, /
). When a GET request is made to this route, the function read_root
is called, and it returns a JSON response with the greeting ‘Hello, FastAPI’.
To start the application, run the following command in your terminal:
uvicorn main:app --reload
The --reload
flag enables hot reloading, which means the server will automatically update whenever you make changes to your code.
Now, if you navigate to http://localhost:8000/
in your web browser, you should see the message ‘Hello, FastAPI’ displayed.
FastAPI’s simplicity is one of its biggest advantages, but that doesn’t mean it’s not powerful. As we’ll see in the next sections, FastAPI has a wealth of features that make it a great choice for building robust, high-performance web APIs.
FastAPI: Dive into Advanced Features
FastAPI is not just about simplicity and speed. It also provides a wealth of advanced features that can help you build more complex and robust web APIs. Let’s take a look at some of these features.
Dependency Injection
One of FastAPI’s most powerful features is its built-in support for dependency injection. This feature allows you to manage your code’s dependencies in a clean and efficient manner. Let’s see how this works:
from fastapi import FastAPI, Depends
def get_db():
db = 'connect to db'
return db
@app.get('/items/')
def read_items(db = Depends(get_db)):
return {'db': db}
In this example, we have a function get_db
that simulates a database connection. We then have a route that depends on this function, as indicated by the Depends
keyword. When a request is made to this route, FastAPI will first call the get_db
function and pass its return value as an argument to read_items
.
Validation
FastAPI also provides automatic request validation using Python’s type hints. Here’s an example:
from fastapi import FastAPI, Query
app = FastAPI()
@app.get('/items/')
def read_items(q: str = Query(..., min_length=3, max_length=50)):
return {'q': q}
In this code, we’re specifying that the q
query parameter must be a string of at least 3 and at most 50 characters. If a request is made that doesn’t meet these requirements, FastAPI will automatically return a helpful and descriptive error response.
Security Features
FastAPI has robust security features built-in, including support for OAuth2 authentication, JWT tokens, HTTP Basic auth, and more. For example, here’s how you can secure a route with HTTP Basic auth:
from fastapi import FastAPI, Depends, HTTPException, status
from fastapi.security import HTTPBasic, HTTPBasicCredentials
app = FastAPI()
security = HTTPBasic()
def get_current_username(credentials: HTTPBasicCredentials = Depends(security)):
if credentials.username != 'foo' or credentials.password != 'bar':
raise HTTPException(status_code=status.HTTP_401_UNAUTHORIZED)
return credentials.username
@app.get('/users/me')
def read_current_user(username: str = Depends(get_current_username)):
return {'username': username}
In this example, we’re using the HTTPBasic
class to require HTTP Basic auth for the /users/me
route. If the provided username and password don’t match ‘foo’ and ‘bar’, respectively, FastAPI will return a 401 Unauthorized response.
These advanced features make FastAPI a flexible and powerful tool for building web APIs, whether you’re working on a small side project or a large-scale production application.
FastAPI vs Flask vs Django: A Python Framework Showdown
While FastAPI is a powerful tool for building web APIs, it’s not the only game in town. Two other popular Python web frameworks are Flask and Django. Let’s take a look at how they compare to FastAPI.
FastAPI vs Flask
Flask is a lightweight and flexible framework that’s great for small to medium-sized applications. It’s easy to learn and use, but it doesn’t come with many of the features that FastAPI and Django do out of the box. For example, Flask doesn’t have built-in support for data validation or serialization. However, Flask’s simplicity and flexibility make it a good choice for projects where you need full control over the application’s behavior.
FastAPI, on the other hand, is more feature-rich and is designed with speed in mind. It has built-in support for data validation, serialization, and asynchronous programming, among other things. However, these features come at the cost of a steeper learning curve compared to Flask.
FastAPI vs Django
Django is a high-level framework that’s designed for building complex web applications. It comes with a lot of features out of the box, such as an ORM, authentication support, and an admin interface. However, Django’s ‘batteries included’ approach can make it overkill for small, simple applications, and its monolithic design can be a downside for some use cases.
FastAPI, like Flask, is more flexible than Django and is a better choice for applications that require high performance or real-time capabilities. However, Django’s built-in features can save a lot of development time for complex applications.
In summary, while FastAPI is a great choice for building high-performance web APIs, Flask and Django are also worth considering depending on your specific needs. Flask is a good choice for small to medium-sized applications where you need full control over the application’s behavior, while Django is a good choice for complex applications that can benefit from its many built-in features.
FastAPI Troubleshooting and Best Practices
Like any other framework, you might encounter some challenges when working with FastAPI. Let’s discuss some common issues and their solutions. We’ll also touch on some best practices for optimizing your FastAPI applications.
Handling Errors
FastAPI provides a standard way to handle errors using HTTPException. Let’s say you want to validate a path parameter and return a 400 error if it’s not valid. Here’s how you can do it:
from fastapi import FastAPI, HTTPException
app = FastAPI()
@app.get('/items/{item_id}')
def read_item(item_id: int):
if item_id < 1:
raise HTTPException(status_code=400, detail='Invalid item ID')
return {'item_id': item_id}
In this code, if the item_id
is less than 1, FastAPI will return a 400 error with the detail ‘Invalid item ID’.
Using Asynchronous Functions
One of FastAPI’s standout features is its support for asynchronous programming. However, it’s important to remember that you should only use async functions if the libraries you’re using also support it. If they don’t, using async functions can actually make your application slower.
Optimizing FastAPI Applications
FastAPI is designed for speed, but there are still things you can do to make your applications even faster. For example, you can use Pydantic’s @validator
decorator to validate request data instead of doing it manually in your route functions. This can result in cleaner code and better performance.
FastAPI is a powerful tool, but like any tool, it’s most effective when used correctly. By understanding and anticipating common issues, and by following best practices, you can get the most out of FastAPI.
Understanding FastAPI’s Core Concepts
FastAPI is built on several key concepts that make it a powerful and flexible tool for web development. Let’s delve into these fundamental concepts to better understand how FastAPI works.
Asynchronous Programming
FastAPI is built with asynchronous programming in mind. But what does that mean? Simply put, asynchronous programming is a way of writing software that’s designed to perform tasks concurrently, rather than sequentially. This can result in significant performance improvements for certain types of tasks, like handling HTTP requests.
Here’s a simple example of an asynchronous route handler in FastAPI:
from fastapi import FastAPI
import asyncio
app = FastAPI()
@app.get('/async')
async def read_items():
await asyncio.sleep(1)
return {'Hello': 'World'}
In this code, we’re using the async
keyword to define an asynchronous function, and the await
keyword to pause the function execution until asyncio.sleep(1)
completes. This allows FastAPI to handle other requests while this function is waiting, improving the overall performance of the application.
Python Type Hints
FastAPI makes extensive use of Python’s type hints. Type hints are a way of indicating the expected type of a function’s arguments and return value. FastAPI uses these type hints to perform data validation, serialization, and to generate interactive API documentation.
Here’s an example of a FastAPI route that uses type hints:
from fastapi import FastAPI
from pydantic import BaseModel
class Item(BaseModel):
name: str
price: float
app = FastAPI()
@app.post('/items/')
def create_item(item: Item):
return item
In this code, we’re defining a Pydantic model Item
with two fields: name
and price
. We then use this model as a type hint for the item
parameter of our route function. FastAPI will automatically validate the request data against this model and return a 422 error if the data is invalid.
Understanding these concepts will help you better understand and utilize FastAPI’s features. Asynchronous programming and type hints are key to FastAPI’s performance and ease of use.
FastAPI in Larger Projects and Modern Web Development
FastAPI is not just for small applications or projects. Its features and capabilities make it a powerful tool for larger projects and modern web development.
FastAPI in Large-Scale Applications
FastAPI’s support for asynchronous programming, automatic validation, serialization, and interactive documentation make it a great choice for large-scale applications. Its high performance and flexibility allow it to handle complex requirements and high loads, while its intuitive design and clear syntax make it easy to maintain, even as your codebase grows.
FastAPI and Modern Web Development Trends
FastAPI aligns well with modern web development trends. Its support for asynchronous programming fits in with the increasing demand for real-time applications. Its built-in validation and serialization make it easy to work with modern front-end frameworks and libraries. And its automatic generation of interactive API documentation fits in with the trend towards API-first development.
FastAPI and Best Practices
FastAPI encourages best practices in Python and web development. It uses modern Python features like type hints and async/await, and it follows the SOLID principles of software design. Using FastAPI can help you write cleaner, more maintainable code.
Further Resources for FastAPI Mastery
If you’re interested in diving deeper into FastAPI, here are a few resources that can help:
- Python GUI: Your User Interface Solution – Learn how to create custom widgets and components in Python.
PySimpleGUI: Easy GUI Development in Python – Learn about PySimpleGUI for easy GUI development.
Jinja: Templating Engine for Python Web Development – Explore Jinja for templating in Python.
FastAPI’s official documentation – A comprehensive guide to FastAPI’s features and usage.
Awesome FastAPI – A curated list of awesome things related to FastAPI, including plugins, templates, and articles.
FastAPI Tutorial – Full Course for Beginners on YouTube.
These resources can help you gain a deeper understanding of FastAPI and how to use it effectively in your projects.
Wrapping Up: FastAPI’s Power and Versatility
FastAPI is a modern, fast, and feature-rich Python web framework that’s perfect for building APIs.
Its standout features like support for asynchronous programming, automatic validation, serialization, and interactive API documentation make it a powerful tool for both simple and complex applications.
FastAPI’s use of Python type hints not only provides automatic data validation but also leverages modern Python features to improve code readability and maintainability.
While FastAPI is packed with features, it’s also designed with simplicity in mind. Whether you’re building a small application or a large-scale project, FastAPI offers an intuitive and flexible approach to web development. Its clear syntax and comprehensive documentation make it accessible to developers of all skill levels.
However, like any tool, FastAPI comes with its own set of challenges. Understanding common issues, such as when to use asynchronous functions and how to handle errors, can help you get the most out of this framework. But with a little practice and the right resources, you can overcome these challenges and harness the full power of FastAPI.
When comparing FastAPI with other Python web frameworks like Flask and Django, it’s clear that each has its own strengths and use cases. FastAPI stands out for its speed and features, but Flask’s simplicity and flexibility or Django’s ‘batteries included’ approach may be more suitable for certain applications.
Framework | Speed | Flexibility | Built-in Features |
---|---|---|---|
FastAPI | High | High | High |
Flask | Medium | High | Low |
Django | Low | Medium | High |
FastAPI is more than just a web framework—it’s a powerful tool for modern web development. By understanding its core concepts and features, you can leverage FastAPI to build high-performance, robust, and maintainable web APIs. So, whether you’re a seasoned Python developer or just getting started, FastAPI
could be the perfect tool for your next project.