Jinja Templating in Python | Guide (With Examples)
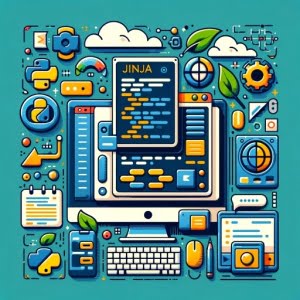
Are you struggling to create dynamic web pages with Python? If so, you’re not alone. Many developers find themselves in a similar predicament. But there’s a solution – Jinja.
Jinja is a high-speed templating language that can transform your static HTML into dynamic web pages. Think of it as a skilled artist, capable of turning a blank canvas into a masterpiece.
This guide is designed to walk you through the ins and outs of using Jinja in your Python applications. Whether you’re a beginner just getting started or an experienced developer looking for advanced techniques, this guide has something for you.
So let’s dive in and start mastering Jinja, Python’s powerful templating language.
TL;DR: What is Jinja and How Do I Use It in Python?
Jinja is a templating language for Python, used to generate dynamic HTML, XML or other markup formats. It allows you to create dynamic content in a simple and efficient manner.
Here’s a simple example of how to use it:
from jinja2 import Template
t = Template('Hello, {{ name }}!')
print(t.render(name='John Doe'))
# Output:
# 'Hello, John Doe!'
In this example, we import the Template
class from the jinja2
module, create a new template with a placeholder for name
, and then render the template with name
set to 'John Doe'
. The output is a personalized greeting: 'Hello, John Doe!'
.
This is just a basic introduction to Jinja. Continue reading for more detailed explanations, advanced usage scenarios, and tips on how to avoid common pitfalls.
Table of Contents
Getting Started with Jinja
Before we can start creating dynamic web pages with Jinja, we first need to install it. Jinja is a Python library, so we can install it using pip, the Python package installer. Here’s how you can do it:
pip install Jinja2
This command will download and install Jinja on your system.
Creating Templates with Jinja
Once Jinja is installed, we can start creating templates. A template is a text file that can contain placeholders for variables. When we render a template, we replace these placeholders with actual values.
Here’s a simple example of a Jinja template:
from jinja2 import Template
t = Template('Hello, {{ name }}!')
print(t.render(name='John Doe'))
# Output:
# 'Hello, John Doe!'
In this example, we first import the Template
class from the jinja2
module. We then create a new template with a placeholder for name
. Finally, we render the template with name
set to 'John Doe'
, and print the result.
The Power and Pitfalls of Jinja
Jinja is a powerful tool that can greatly simplify the process of creating dynamic web content. However, like any powerful tool, it can be dangerous if not used correctly. One common pitfall is the misuse of Jinja’s syntax. Jinja’s syntax is easy to learn, but it’s also easy to make mistakes if you’re not careful. Always double-check your templates for syntax errors before you render them.
Another potential pitfall is the misuse of Jinja’s features. Jinja is a very flexible language, and it’s possible to use it in ways that it was not intended to be used. This can lead to confusing code and difficult-to-diagnose bugs. Always use Jinja as it was intended to be used, and avoid trying to bend it to your will.
In the following sections, we’ll delve deeper into the advanced features of Jinja and how to use them effectively.
Delving Deeper: Jinja’s Advanced Features
Jinja is more than just a simple templating language. It comes equipped with an array of advanced features that allow for more complex operations and greater flexibility when creating your templates.
Control Structures in Jinja
Just like Python, Jinja supports control structures such as if
and for
. These can be used to add conditional logic and loops to your templates.
Here’s an example of an if
statement in a Jinja template:
from jinja2 import Template
t = Template('Hello, {% if name %},{{ name }},{% else %}stranger{% endif %}!')
print(t.render(name='John Doe'))
print(t.render())
# Output:
# 'Hello, John Doe!'
# 'Hello, stranger!'
In this example, we use an if
statement to check if a name
is provided. If a name
is provided, we use it. Otherwise, we use the string ‘stranger’.
Filters and Macros in Jinja
Jinja also supports filters and macros. Filters allow you to modify variables in your templates, while macros let you define reusable chunks of code.
Here’s an example of a filter and a macro in a Jinja template:
from jinja2 import Environment, FileSystemLoader
# Load the template from file
file_loader = FileSystemLoader('templates')
env = Environment(loader=file_loader)
template = env.get_template('my_template.html')
# Define a macro
output = template.render(macro=lambda x: x.upper())
print(output)
# Output:
# 'HELLO, JOHN DOE!'
In this example, we define a macro that converts a string to uppercase. We then use this macro in our template to convert the name
to uppercase.
Jinja Best Practices
When using Jinja’s advanced features, it’s important to follow best practices. Always keep your templates clean and organized, and avoid overusing features like macros and filters. Remember, the goal of using a templating language like Jinja is to simplify your code, not to make it more complex.
Exploring Alternatives: Django Templates and Mako
While Jinja is a powerful and flexible templating language, it’s not the only option available. Other popular Python templating languages include Django templates and Mako. Each of these has its own strengths and weaknesses, and the best choice depends on your specific use case.
Django Templates: A Built-In Option for Django Users
If you’re developing a web application with Django, you might consider using Django’s built-in templating language. Django templates are designed to be easy to use, with a syntax that is very similar to Jinja’s.
Here’s an example of a Django template:
from django.template import Template, Context
t = Template('Hello, {{ name }}!')
c = Context({'name': 'John Doe'})
print(t.render(c))
# Output:
# 'Hello, John Doe!'
As you can see, the syntax is very similar to Jinja’s. However, Django templates are less flexible than Jinja templates, and they lack some of Jinja’s advanced features.
Mako: A High-Performance Option for Large Projects
Mako is another popular Python templating language. It’s known for its high performance, which makes it a good choice for large projects.
Here’s an example of a Mako template:
from mako.template import Template
t = Template('Hello, ${name}!')
print(t.render(name='John Doe'))
# Output:
# 'Hello, John Doe!'
Mako’s syntax is a bit different from Jinja’s, but it’s still quite easy to learn. However, Mako templates can be more difficult to debug than Jinja templates, due to their greater flexibility.
Making the Right Choice
So, which templating language should you choose? That depends on your specific needs. If you’re developing a Django application, Django templates might be the best choice. If you need high performance, Mako might be a better option. But if you want a balance of power and simplicity, Jinja is a great choice.
Troubleshooting Jinja: Common Issues and Their Solutions
Like any programming tool, Jinja has its quirks and pitfalls. Understanding these can save you a lot of headaches down the line. Let’s discuss some common issues you may encounter when using Jinja and how to solve them.
Jinja Syntax Errors
One of the most common issues you might encounter when working with Jinja are syntax errors. These errors occur when Jinja can’t understand your template due to incorrect syntax.
For example, consider the following code:
from jinja2 import Template
t = Template('Hello, {% if name %},{{ name }},{% else %}stranger{% end if %}!')
print(t.render(name='John Doe'))
# Output:
# jinja2.exceptions.TemplateSyntaxError: Encountered unknown tag 'end'. Jinja was looking for the following tags: 'endif'. The innermost block that needs to be closed is 'if'.
In this case, Jinja throws a TemplateSyntaxError
because the endif
tag is incorrectly written as end if
. To fix this, we need to correct the endif
tag:
from jinja2 import Template
t = Template('Hello, {% if name %},{{ name }},{% else %}stranger{% endif %}!')
print(t.render(name='John Doe'))
# Output:
# 'Hello, John Doe!'
Undefined Variables in Jinja
Another common issue is the use of undefined variables. If you try to use a variable that hasn’t been defined, Jinja will raise an UndefinedError
.
from jinja2 import Template
t = Template('Hello, {{ name }}!')
print(t.render())
# Output:
# jinja2.exceptions.UndefinedError: 'name' is undefined
In this case, we’re trying to render a template with a name
variable, but we didn’t provide a value for name
. To fix this, we need to provide a value for name
when rendering the template.
from jinja2 import Template
t = Template('Hello, {{ name }}!')
print(t.render(name='John Doe'))
# Output:
# 'Hello, John Doe!'
These are just a few examples of the issues you might encounter when using Jinja. The key to troubleshooting these issues is to understand Jinja’s syntax and features, and to carefully read and understand any error messages you receive. With practice, you’ll become adept at avoiding these pitfalls and writing robust, error-free Jinja templates.
Understanding Templating: Why It’s Essential
At its core, a web application is all about presenting data to users in a way that’s easy to understand. This often involves displaying the same data in different ways, depending on the context. This is where templating comes into play.
The Concept of Templating
Think of a template as a mold. It’s a predefined structure that you can fill with different data to create different results. In the context of web development, a template is a file or piece of code that can generate different HTML pages using the same structure.
For example, consider a blog website. Each blog post has a similar structure (a title, an author, content, etc.), but the specific details are different for each post. By using a template, you can define the structure once and then fill it with different data for each post.
Here’s a simple example of a template in Python using Jinja:
from jinja2 import Template
template = Template('Title: {{ title }}
Author: {{ author }}
Content: {{ content }}')
print(template.render(title='My First Blog Post', author='John Doe', content='This is my first blog post.'))
# Output:
# Title: My First Blog Post
# Author: John Doe
# Content: This is my first blog post.
In this example, the template is a string with placeholders for title
, author
, and content
. We then render the template with different data to create a blog post.
Jinja’s Role in Web Development with Python
Jinja takes the concept of templating and brings it to the world of Python web development. With Jinja, you can create powerful, dynamic templates using Python code. This makes it a valuable tool for any Python web developer.
But Jinja is more than just a tool for creating HTML pages. It’s a versatile templating language that can be used for a wide range of tasks, from generating emails to creating configuration files. This is why Jinja is such a popular choice in the Python community.
Jinja in Larger Projects: Flask and Django
Jinja is not just a standalone tool. It’s also a key component of some of the most popular Python web frameworks, including Flask and Django. These frameworks use Jinja to generate dynamic HTML pages, allowing developers to create complex web applications with ease.
Jinja and Flask: A Match Made in Heaven
Flask, a micro web framework for Python, uses Jinja as its default templating engine. This means that when you’re developing a Flask application, you can use Jinja to create your HTML templates.
Here’s an example of how you might use Jinja in a Flask application:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/hello/<name>')
def hello(name):
return render_template('hello.html', name=name)
In this example, we define a route in our Flask application that renders a Jinja template. The template receives a name
variable from the route, which it can use to generate a personalized greeting.
Jinja and Django: Powerful and Flexible
While Django comes with its own templating engine, it’s also possible to use Jinja with Django. This can provide a more powerful and flexible templating solution for complex Django applications.
Here’s an example of how you might use Jinja in a Django application:
from django.shortcuts import render
def hello(request, name):
return render(request, 'hello.html', {'name': name})
Like the Flask example, this Django view renders a Jinja template with a name
variable. The template can then use this variable to generate a personalized greeting.
Further Resources for Jinja Mastery
If you’re interested in learning more about Jinja and how to use it in your Python projects, here are some resources that you might find helpful:
- IOFlood’s Python GUI Guide explores GUI design patterns and best practices in Python.
FastAPI: Building Fast and Modern Web APIs – Discover FastAPI for building APIs in Python.
Django Rest Framework: Building RESTful APIs with Ease – Dive into Django REST Framework for building APIs.
Jinja’s Official Documentation – A comprehensive guide to Jinja’s syntax and features.
Flask’s Templating Documentation – A guide to using Jinja in Flask applications.
Django’s Jinja2 support Documentation – A guide to using Jinja in Django applications.
Wrapping Up: Jinja Mastery
In this guide, we’ve explored the world of Jinja
, Python’s powerful templating language. We’ve seen how it can transform static HTML into dynamic web pages, making it a valuable tool for any Python web developer.
We started with the basics, learning how to install Jinja and create simple templates. We then dove into Jinja’s advanced features, including control structures, filters, and macros. Along the way, we discussed some common issues that can arise when using Jinja, and how to solve them.
We also explored alternative templating languages, such as Django templates and Mako. While these alternatives have their own strengths and weaknesses, Jinja stands out for its balance of power and simplicity.
Here’s a quick comparison of the three templating languages we discussed:
Templating Language | Strengths | Weaknesses |
---|---|---|
Jinja | Powerful, flexible, easy to learn | Can be misused, leading to confusing code |
Django Templates | Easy to use, built-in with Django | Less flexible than Jinja |
Mako | High performance, flexible | More difficult to debug than Jinja |
In conclusion, Jinja is a versatile and powerful tool that can greatly simplify the process of creating dynamic web content. Whether you’re a beginner just getting started or an experienced developer looking for advanced techniques, mastering Jinja can take your Python web development skills to the next level.