Python GUI Development: A Step-by-Step Guide
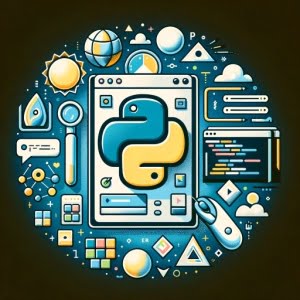
Are you finding it challenging to create a graphical user interface (GUI) in Python? You’re not alone. Many developers grapple with this task, but Python, like a skilled architect, offers tools to construct intuitive and user-friendly GUIs.
This guide will walk you through the process of creating a GUI in Python, from basic to advanced techniques. We’ll cover everything from using libraries like tkinter, PyQt, and wxPython to handling common issues and their solutions.
So, whether you’re a beginner just starting out or an experienced developer looking to brush up your skills, there’s something in this guide for you. Let’s get started!
TL;DR: How Do I Create a GUI in Python?
To create a GUI in Python, you can use libraries like tkinter, PyQt, or wxPython. These libraries provide the tools and functions needed to build interactive user interfaces.
Here’s a simple example using tkinter:
from tkinter import Tk, Label
window = Tk()
label = Label(window, text='Hello, Python GUI!')
label.pack()
window.mainloop()
# Output:
# A window pops up with the text 'Hello, Python GUI!'
In this example, we import the Tk and Label classes from the tkinter library. We then create a window and a label with the text ‘Hello, Python GUI!’. The pack()
method is used to position the label in the window, and mainloop()
is called to display the window.
This is just a basic introduction to creating GUIs in Python. Stay tuned for a more detailed guide, where we’ll dive deeper into tkinter and explore other libraries like PyQt and wxPython.
Table of Contents
Getting Started with tkinter: A Beginner’s Guide
Let’s start with the basics. To create a GUI in Python, one of the most straightforward libraries to use is tkinter. This library provides a fast and easy way to create windows, labels, buttons, and other GUI elements.
Here’s a simple code example that shows how to create a basic window using tkinter:
from tkinter import Tk
window = Tk()
window.title('My First Python GUI')
window.geometry('300x200')
window.mainloop()
# Output:
# A 300x200 window pops up with the title 'My First Python GUI'
In this code, we first import the Tk
class from the tkinter library. We then create a new window, set its title to ‘My First Python GUI’, and define its size to be 300×200 pixels. The mainloop()
method is called to display the window.
One of the advantages of tkinter is its simplicity and ease of use. With just a few lines of code, you can create a functional GUI. However, tkinter also has its limitations. For instance, it may not be the best choice for creating highly complex or custom GUIs. But for beginners, it’s a great place to start.
Using tkinter for Complex GUIs
As you gain confidence with tkinter, you can start to explore more complex GUI elements. This includes adding buttons, text input fields, and handling events. Let’s dive deeper into each of these topics.
Adding Buttons with tkinter
Buttons are a staple in GUI design. They allow users to interact with the software through simple clicks. Here’s an example of how to add a button in tkinter:
from tkinter import Tk, Button
def say_hello():
print('Hello, Python GUI!')
window = Tk()
button = Button(window, text='Click Me', command=say_hello)
button.pack()
window.mainloop()
# Output:
# When the 'Click Me' button is clicked, 'Hello, Python GUI!' is printed to the console.
In this code, we first define a function say_hello()
that prints a message to the console. We then create a button with the text ‘Click Me’ and set its command to the say_hello()
function. This means that when the button is clicked, the say_hello()
function is executed.
Creating Text Input Fields with tkinter
Text input fields are another common GUI element. They allow users to enter text, which can then be processed by the software. Here’s an example of how to create a text input field in tkinter:
from tkinter import Tk, Entry
window = Tk()
entry = Entry(window)
entry.pack()
window.mainloop()
# Output:
# A window pops up with a text input field.
In this code, we create an Entry
widget, which is tkinter’s text input field. We then pack it into the window.
Handling Events with tkinter
Event handling is a key aspect of GUI programming. It allows your software to respond to user actions, such as button clicks or key presses. Here’s an example of how to handle a button click event in tkinter:
from tkinter import Tk, Button
def on_click(event):
print('Button clicked!')
window = Tk()
button = Button(window, text='Click Me')
button.bind('<Button-1>', on_click)
button.pack()
window.mainloop()
# Output:
# When the 'Click Me' button is clicked, 'Button clicked!' is printed to the console.
In this code, we define an on_click()
function that prints a message to the console. We then create a button and bind the <Button-1>
event (which represents a left mouse button click) to the on_click()
function. This means that when the button is clicked, the on_click()
function is executed.
As you can see, tkinter provides a variety of tools for creating complex GUIs. However, it’s important to note that while tkinter is powerful, it might not be the best choice for every project. In the next section, we’ll explore some alternative libraries for creating GUIs in Python.
Exploring Alternative Libraries: PyQt and wxPython
While tkinter is a powerful tool for creating GUIs in Python, it’s not the only option. There are several other libraries available that offer more features and flexibility. Two of the most popular alternatives are PyQt and wxPython.
Dive into PyQt
PyQt is a set of Python bindings for the Qt application framework. It is known for its flexibility and has a wide range of capabilities.
Here’s a basic example of how to create a window using PyQt:
from PyQt5.QtWidgets import QApplication, QWidget
app = QApplication([])
window = QWidget()
window.setWindowTitle('My First PyQt GUI')
window.show()
app.exec_()
# Output:
# A window pops up with the title 'My First PyQt GUI'
In this example, we first create a QApplication
object, which is needed for any PyQt application. We then create a QWidget
object, which serves as a window. We set its title to ‘My First PyQt GUI’ and display it using the show()
method. Finally, we start the application’s event loop with app.exec_()
.
One of the advantages of PyQt is its extensive functionality. It supports a wide range of widgets, layouts, and other GUI elements. However, PyQt is also more complex than tkinter, which can make it more challenging for beginners.
Getting Started with wxPython
wxPython is another popular library for creating GUIs in Python. It’s a set of Python bindings for the wxWidgets C++ library, which provides native look and feel for different operating systems.
Here’s a basic example of how to create a window using wxPython:
import wx
app = wx.App()
window = wx.Frame(None, title='My First wxPython GUI')
window.Show()
app.MainLoop()
# Output:
# A window pops up with the title 'My First wxPython GUI'
In this example, we first create a wx.App
object, which is needed for any wxPython application. We then create a wx.Frame
object, which serves as a window. We set its title to ‘My First wxPython GUI’ and display it using the Show()
method. Finally, we start the application’s event loop with app.MainLoop()
.
One of the advantages of wxPython is its native look and feel. It uses the native widgets of the operating system, which makes your GUIs look like they belong on the platform they’re running on. However, like PyQt, wxPython is also more complex than tkinter.
Which Library Should You Choose?
The choice between tkinter, PyQt, and wxPython depends on your specific needs. If you’re a beginner or need to create a simple GUI quickly, tkinter is a great choice. If you need more advanced features or want a native look and feel, PyQt or wxPython might be a better option.
Regardless of the library you choose, the important thing is to understand the basics of GUI programming in Python. Once you have a solid foundation, you can explore different libraries and find the one that works best for you.
Troubleshooting Common Python GUI Issues
Creating a GUI in Python can sometimes come with its own set of challenges. This section will cover some common issues you may encounter and provide solutions and workarounds for each one.
Handling User Input
One common issue in GUI programming is handling user input, especially when it comes to validating and processing this input. Here’s an example of how to handle user input in tkinter:
from tkinter import Tk, Entry, StringVar
def on_submit(event):
print('User input:', user_input.get())
window = Tk()
user_input = StringVar()
entry = Entry(window, textvariable=user_input)
entry.bind('<Return>', on_submit)
entry.pack()
window.mainloop()
# Output:
# When the user presses the Return key, their input is printed to the console.
In this code, we bind the <Return>
event (which represents the Return key being pressed) to the on_submit()
function. This function prints the user’s input to the console.
Updating the GUI
Another common issue is updating the GUI, especially in response to user actions. Here’s an example of how to update a label in tkinter:
from tkinter import Tk, Label, StringVar
window = Tk()
text = StringVar()
text.set('Hello, Python GUI!')
label = Label(window, textvariable=text)
label.pack()
# Later in your code...
text.set('Goodbye, Python GUI!')
# Output:
# The label's text is updated from 'Hello, Python GUI!' to 'Goodbye, Python GUI!'.
In this code, we use a StringVar
to hold the label’s text. We can then update this text by calling text.set()
.
Dealing with Different Screen Sizes
Finally, dealing with different screen sizes can be a challenge in GUI programming. Here’s a tip for creating a responsive layout in tkinter:
from tkinter import Tk, Button
window = Tk()
window.geometry('300x200')
button = Button(window, text='Click Me')
button.pack(fill='both', expand=True)
window.mainloop()
# Output:
# A button fills the entire window, and resizes when the window is resized.
In this code, we use the fill
and expand
options in the pack()
method to make the button fill the entire window and resize with it.
While these are just a few examples, they illustrate some of the common challenges you may face when creating a GUI in Python. With a little practice and patience, you’ll be able to overcome these challenges and create effective and user-friendly interfaces.
Understanding GUI: The Building Blocks
Before diving into the code, let’s take a moment to understand the concept of a Graphical User Interface (GUI) and how it works in Python.
A GUI is a type of user interface that allows users to interact with electronic devices through graphical icons and visual indicators, as opposed to text-based interfaces, typed command labels, or text navigation. GUIs were introduced in reaction to the perceived steep learning curve of command-line interfaces (CLIs).
Event-Driven Programming: The Heart of GUI
GUI programming in Python, like in most modern languages, is event-driven. Event-driven programming is a programming paradigm in which the flow of the program is determined by events such as user actions (mouse clicks, key presses), sensor outputs, or messages from other programs.
Here’s an example of a simple event-driven program in Python using tkinter:
from tkinter import Tk, Button
def on_click():
print('Button clicked!')
window = Tk()
button = Button(window, text='Click Me', command=on_click)
button.pack()
window.mainloop()
# Output:
# When the 'Click Me' button is clicked, 'Button clicked!' is printed to the console.
In this code, we first define an on_click()
function that prints a message to the console. We then create a button with the text ‘Click Me’ and set its command to the on_click()
function. This means that when the button is clicked, the on_click()
function is executed. This is a basic example of event-driven programming.
Understanding the concept of GUI and the fundamentals of event-driven programming is crucial to mastering GUI programming in Python. With these basics in mind, you’re ready to start creating your own GUIs.
Python GUI in Real-World Applications
GUI programming in Python is not just an academic exercise – it has a wide range of applications in the real world. Let’s explore a few of these.
Desktop Applications
One of the most common uses of GUI programming is in creating desktop applications. Python, with its rich set of libraries, allows you to build applications for a variety of tasks, from simple text editors to complex data analysis tools.
Game Development
Python is also a popular choice for game development, thanks to libraries like Pygame. With GUI programming, you can create interactive games with rich, engaging interfaces.
Exploring Related Concepts
As you continue your journey in GUI programming, you might want to explore related concepts like threading in GUI applications, which allows for multitasking, and 3D GUIs, which add a whole new dimension to your interfaces.
Further Resources for Python GUI Mastery
To deepen your understanding of Python GUI programming, check out these resources:
- Getting Started with Django: A Quick Tutorial – Dive into Django’s powerful features and tools.
Python GUI Programming with Tkinter by Real Python – A comprehensive tutorial on tkinter, Python’s standard GUI package.
PyQt5 tutorial by Learn PyQt – A detailed guide on PyQt, a Python binding for the Qt application framework.
wxPython tutorial by ZetCode – An in-depth tutorial on wxPython, a Python wrapper for the wxWidgets C++ library.
Remember, mastering Python GUI programming is a journey. Keep exploring, keep learning, and most importantly, keep coding!
Wrapping Up: Mastering Python GUI Creation
In this comprehensive guide, we’ve explored the vast landscape of creating graphical user interfaces (GUIs) in Python. We’ve delved into the process, the libraries used, and common issues you might encounter, along with their solutions.
We started with the basics, learning how to create a simple GUI using tkinter. We then ventured into more advanced territory, adding buttons, text input fields, and handling events with tkinter. Along the way, we tackled common challenges, such as handling user input, updating the GUI, and dealing with different screen sizes, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to GUI programming in Python, comparing tkinter with other libraries like PyQt and wxPython. Here’s a quick comparison of these libraries:
Library | Ease of Use | Advanced Features | Native Look and Feel |
---|---|---|---|
tkinter | High | Moderate | Low |
PyQt | Moderate | High | High |
wxPython | Moderate | High | High |
Whether you’re a beginner just starting out with Python GUI programming or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of how to create GUIs in Python and the power of this tool. Happy coding!