NPM Explained | What It Is & Why It Matters
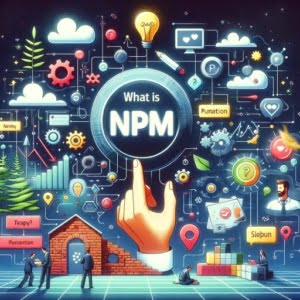
At IOFLOOD, we’ve often encountered questions about npm and its role in JavaScript development. To provide clarity on this tool, we’ve created this guide to explain what npm is and how it benefits developers. By reading our concise explanation, you’ll gain a better understanding of npm’s purpose and its significance in managing dependencies for your projects.
This guide will introduce you to npm, explaining its purpose, how it works, and why it’s indispensable for modern web development. By the end of this guide, you’ll have a solid understanding of npm and how to leverage it in your projects to manage packages efficiently.
Let’s demystify npm together and empower you to navigate the JavaScript ecosystem with confidence!
TL;DR: What Is npm?
npm, a package manager for JavaScript, comes bundled with Node.js and simplifies JavaScript development by managing project dependencies. With commands like
npm install
to add packages andnpm update
to keep them current, it streamlines code sharing and collaboration.
Here’s a quick example of installing a package with npm:
npm install lodash
In this example, we use npm to install the lodash library, a popular utility library that provides modular methods to enhance JavaScript programming. The command npm install lodash
fetches the lodash package from the npm registry and adds it to the project, making its functions available for use.
This guide will delve deeper into npm’s functionalities and best practices, offering insights into how it streamlines development workflows and fosters code sharing and reuse. Keep reading to unlock the full potential of npm in your projects.
Table of Contents
Getting Started with npm
Embarking on your journey with npm begins with two foundational steps: installing Node.js and initializing your project using npm. This process lays the groundwork for managing your project’s dependencies effectively.
Installing Node.js and npm
Node.js comes with npm installed, so when you install Node.js, you automatically get npm. To check if you have them installed, open your terminal or command prompt and type:
node -v
npm -v
# Output:
# v14.17.0
# 6.14.13
This output tells you the version of Node.js and npm installed on your system. It’s crucial to have both installed to utilize npm for your projects.
Initializing Your Project
The next step is to create a new directory for your project and initialize it with npm init
. This command starts a questionnaire that helps you create a package.json
file, which is essential for managing your project’s dependencies.
cd your_project_directory
npm init
# Output:
# This utility will walk you through creating a package.json file.
After answering the questions, a package.json
file is created in your project directory. This file is crucial as it keeps track of all the dependencies your project needs, as well as other important information.
Installing Dependencies
Now that your project is initialized, you can start adding dependencies with npm install
. For instance, if you want to add Express, a fast, unopinionated, minimalist web framework for Node.js, you would run:
npm install express
# Output:
# + [email protected]
# added 1 package in 2.265s
This command downloads Express and adds it to your project’s package.json
file, marking it as a dependency. It demonstrates npm’s role in managing and tracking project dependencies, ensuring that your project has access to the libraries it needs to run successfully.
By following these steps, you’ve taken your first strides in leveraging npm for project management. These actions highlight the simplicity and efficiency of npm, making it an indispensable tool for modern web development.
As you grow more comfortable with npm, you’ll discover its capabilities extend far beyond simple package installation. It plays a crucial role in version control, package updates, and distinguishing between global and local packages, facilitating a more collaborative and controlled development environment.
Version Control with npm
One of npm’s powerful features is its ability to manage different versions of packages. This ensures that your project remains stable even as new versions of packages are released. To install a specific version of a package, use:
npm install [email protected]
# Output:
# + [email protected]
# added 1 package in 1.524s
This command installs version 4.17.21 of lodash, allowing you to control exactly which version of a package your project uses. This is especially useful in maintaining project stability and compatibility.
Understanding package-lock.json
The package-lock.json
file is a snapshot of your project’s dependencies at a given time, recording exact versions of each package. This ensures that all team members and deployment environments use the same package versions, avoiding the “it works on my machine” problem.
{
"name": "your-project",
"version": "1.0.0",
"lockfileVersion": 1,
"requires": true,
"packages": {
"": {
"version": "1.0.0",
"dependencies": {
"lodash": "^4.17.21"
}
}
}
}
The presence of package-lock.json
in your project directory signifies a commitment to consistency, crucial for collaborative projects.
Global vs. Local Packages
npm allows you to install packages either globally (available to all projects on your system) or locally (only available to the project at hand). For tools and utilities you use across projects, a global installation is appropriate. However, project-specific dependencies should be installed locally. To install a package globally, use the -g
flag:
npm install -g nodemon
# Output:
# + [email protected]
# added 1 package in 2.765s
This command installs nodemon globally, making it accessible from any project on your system. Understanding when to use global vs. local installations is key to managing your development environment efficiently.
By mastering these advanced npm features, you elevate your project management skills, ensuring a more streamlined and stable development process.
Exploring npm Alternatives
While npm reigns as the default package manager for Node.js, it’s not the only player in the field. Alternatives like Yarn have emerged, offering unique features and benefits that cater to different project needs. Understanding these alternatives can empower you to make informed decisions tailored to your development workflow.
Yarn: A Viable npm Alternative
Introduced by Facebook, Yarn has gained popularity for its speed and reliability. One of Yarn’s standout features is its efficient caching mechanism, which significantly speeds up the installation process by caching every package it downloads. This means that once a package has been downloaded, Yarn won’t need to re-download it for future projects, saving time and bandwidth.
To install a package using Yarn, the command is slightly different from npm:
yarn add lodash
# Output:
# success Saved 1 new dependency.
# info Direct dependencies
# └─ [email protected]
# Done in 0.42s.
This command adds the lodash package to your project, similar to npm install lodash
, but notice the output difference. Yarn provides a concise summary of the action, including the version installed and the time taken, showcasing its focus on efficiency.
Choosing Between npm and Yarn
Deciding whether to use npm or Yarn boils down to your project requirements and personal preferences. npm has made significant improvements in speed and security with recent updates, narrowing the gap between the two. However, Yarn’s offline mode and deterministic installation process can be deciding factors for teams looking for those specific advantages.
Both npm and Yarn are excellent tools with their own sets of features and benefits. By understanding the nuances of each, you can choose the most suitable package manager for your Node.js projects, ensuring a smooth and efficient development process.
Even the most seasoned developers encounter bumps in the road when managing packages with npm. Recognizing common issues and understanding how to address them can significantly enhance your development experience. This section delves into troubleshooting strategies for frequent npm hurdles.
Resolving Dependency Conflicts
One common challenge is dealing with dependency conflicts. For instance, two packages might require different versions of the same dependency. npm tries to resolve these automatically, but sometimes manual intervention is necessary.
To identify dependency conflicts, you can use:
npm list
# Output:
# project-name@version
# ├─┬ [email protected]
# │ └── [email protected]
# └─┬ [email protected]
# └── [email protected]
This command lists your project’s dependencies and their versions, highlighting any conflicts. Resolving these conflicts might involve updating package versions or choosing a specific version that satisfies all dependencies.
Handling Network Issues
Network problems can interfere with npm’s ability to fetch packages. If you’re experiencing timeouts or slow downloads, adjusting the npm registry URL to a mirror closer to your location might help.
To change the npm registry URL, use:
npm config set registry http://registry.npmjs.eu/
# Output:
# 'registry' = 'http://registry.npmjs.eu/'
This alters the registry URL, potentially improving download speeds and reliability. Remember to choose a trustworthy and up-to-date registry mirror.
Solving Global Installation Errors
Global package installations can sometimes fail due to permission issues, especially on Linux systems. Using sudo
for global installs is a common practice, but it’s safer to configure npm to use a directory you own.
To avoid permission issues with global installations, you can configure npm to use a different directory:
mkdir ~/npm-global
npm config set prefix '~/npm-global'
# Output:
# 'prefix' = '~/npm-global'
This setup directs npm to use a directory in your home folder for global installations, circumventing permission problems and keeping your system more secure.
By familiarizing yourself with these troubleshooting techniques, you can navigate npm’s most common challenges more effectively, ensuring a smoother development process.
Background Info on npm
Understanding the fundamentals of npm (Node Package Manager) and the concept of package management is crucial for grasping its significance in the JavaScript ecosystem and web development. npm not only revolutionized how developers share and utilize code but also played a pivotal role in the evolution of JavaScript as a language and its community.
The Genesis of npm
npm was introduced in 2010, a time when JavaScript was rapidly gaining popularity, yet lacked a standardized way for developers to share their code and libraries. The introduction of npm provided a centralized repository for JavaScript packages, facilitating code sharing and reuse among developers worldwide.
Why Package Management?
Package management is akin to organizing a vast library. Just as a librarian categorizes and manages books to make them easily accessible, npm organizes code packages. This organization allows developers to easily find, share, and use code written by others, significantly speeding up development processes and encouraging collaboration.
npm’s Impact on JavaScript
npm’s introduction marked a turning point for JavaScript development. It enabled the creation of complex applications by simplifying dependency management, allowing developers to easily add, update, or remove code packages as needed. This flexibility is demonstrated in the following example:
npm update lodash
# Output:
# + [email protected]
# updated 1 package in 0.76s
In this example, npm update lodash
updates the lodash library to the latest version available in the npm registry. This command is crucial for maintaining the security and efficiency of your project, as it ensures you’re using the most current versions of your dependencies.
The ability to manage dependencies effectively is a cornerstone of modern web development, and npm has been at the forefront of this transformation. By understanding npm’s history and its role in package management, developers can appreciate the tool’s impact on making JavaScript a powerful language for web development.
Practical Usage Cases of npm
npm is more than just a package manager; it’s a vital tool in modern development workflows. Its interaction with build tools, continuous integration, and deployment strategies significantly streamlines development and deployment processes, making it a cornerstone of the JavaScript ecosystem.
Integrating with Build Tools
npm seamlessly integrates with various build tools like Webpack and Gulp, automating tasks such as minification, compilation, and bundling of assets. For example, installing Gulp in your project can be achieved through npm:
npm install --save-dev gulp
# Output:
# + [email protected]
# added 40 packages in 4.512s
This command adds Gulp as a development dependency, enabling you to automate and enhance your build process. The efficiency gained through such integrations is invaluable for modern web development, ensuring projects are not only built faster but also adhere to best practices.
Facilitating Continuous Integration
npm also plays a key role in continuous integration (CI) environments. By using npm scripts, developers can automate testing and deployment tasks, ensuring code quality and consistency across development teams. For instance, integrating npm with a CI tool like Jenkins allows for automated testing and deployment, exemplified by the npm command to run tests:
npm test
# Output:
# > [email protected] test
# > echo "Error: no test specified"
# Error: no test specified
This placeholder output indicates that no tests have been specified yet, highlighting the importance of setting up automated tests in your CI/CD pipeline.
Streamlining Deployment Strategies
npm is integral to deployment strategies, especially in containerized environments like Docker. It ensures that all necessary packages are installed within containers, maintaining consistency across development, testing, and production environments. An example command to install dependencies in a Dockerfile might look like:
RUN npm install
This ensures that all dependencies are correctly installed in the container, facilitating a smoother deployment process.
Further Resources for Mastering npm
To deepen your understanding of npm and its vast capabilities, consider exploring the following resources:
- The official npm Documentation provides comprehensive guides on npm usage, commands, and best practices.
Node.js Guides offer insights into using Node.js and npm in various scenarios.
The npm Blog: For the latest news, updates, and insights into npm, the npm blog is an invaluable resource.
By leveraging npm in conjunction with these resources, developers can enhance their workflow, contributing to more efficient and effective project development.
Recap: npm Usage Guide
In this comprehensive guide, we’ve explored the essence of npm (Node Package Manager), starting from its basic usage to its advanced features, and not overlooking the troubleshooting strategies that ensure your development journey is as smooth as possible. npm stands as a fundamental tool in the JavaScript ecosystem, empowering developers to manage packages with unparalleled efficiency.
We began with the basics, learning how to install Node.js and initialize our projects with npm init
. This foundational knowledge sets the stage for managing project dependencies effectively. We then delved into more complex operations, such as installing specific package versions and understanding the significance of the package-lock.json
file, which ensures consistency across environments.
Moving forward, we explored npm’s advanced features, including handling global vs. local package installations and leveraging npm in continuous integration and deployment strategies. These practices not only streamline the development process but also enhance collaboration and code quality.
Feature | Benefit |
---|---|
Package Version Management | Ensures project stability |
package-lock.json | Guarantees consistency |
Global vs. Local Installations | Optimizes environment setup |
CI/CD Integration | Automates testing and deployment |
By now, you should have a well-rounded understanding of npm and its pivotal role in modern web development. Whether you’re initiating a new project, managing dependencies, or streamlining your development workflow, npm provides the tools necessary to achieve your goals with efficiency and precision.
As we wrap up, remember that mastering npm not only enhances your development capabilities but also opens up new avenues for collaboration and innovation in the JavaScript community. Embrace the power of npm to build more efficient, scalable, and robust projects. Happy coding!