Returning Values from Functions in Bash Shell Scripting
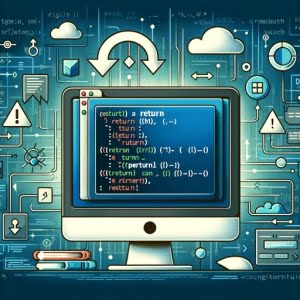
Ever found yourself puzzled over how to return a value from a Bash function? You’re not alone. Many developers find this concept a bit tricky. Think of Bash functions as a black box – you put something in, and you expect something out. But how do you get that ‘something out’? That’s where the return value comes in.
Bash functions are like the gears in a machine, each performing a specific task and often producing a result. The ability to return a value from a function allows us to capture that result, enhancing the flexibility and usefulness of our scripts.
In this guide, we’ll walk you through the process of returning values from Bash functions, from the basics to more advanced techniques. We’ll cover everything from using the ‘return’ command, handling multiple return values, to alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering Bash function return values!
TL;DR: How Do I Return a Value from a Bash Function?
In Bash, you can use the
'return'
command to return a value from a function with the syntax,function returnVal(){return val}
. The returned value can be captured using the special variable$?
.
Here’s a simple example:
function my_func() {
return 10
}
my_func
echo $?
# Output:
# 10
In this example, we define a function my_func
that returns the value 10. After calling my_func
, we use echo $?
to print the return value of the function, which is 10.
This is a basic way to return a value from a Bash function, but there’s much more to learn about Bash functions and their return values. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
The Basics of Returning Values in Bash
In Bash, the ‘return’ command is used to end a function and return the exit status to the caller function. This exit status, an integer number, can be used as the ‘return value’. Let’s look at how this works with a simple example:
function add_numbers() {
local sum=$(( $1 + $2 ))
return $sum
}
add_numbers 5 10
echo $? # prints the return value
# Output:
# 15
In this example, we define a function add_numbers
that takes two arguments and returns their sum. The return
command ends the function and sets the exit status to the sum of the two arguments. After calling add_numbers
with 5 and 10 as arguments, we use echo $?
to print the return value of the function, which is 15.
This is the basic use of the return
command in Bash. It’s simple and straightforward, but it has some limitations. For example, the return value must be an integer between 0 and 255, and only one value can be returned. Therefore, while it’s useful for simple scripts, it might not be sufficient for more complex scenarios.
Advanced Techniques for Returning Values
As we’ve seen, the ‘return’ command in Bash has certain limitations. It can only return an integer between 0 and 255, and it can only return one value. But what if we need to return multiple values, or if we need to return a string? Let’s explore some more advanced techniques.
Returning Multiple Values
One way to return multiple values from a Bash function is by using an array. Here’s an example:
function return_multiple() {
local array=("Hello" "World")
echo ${array[@]}
}
result=(`return_multiple`)
echo ${result[0]} # prints the first value
echo ${result[1]} # prints the second value
# Output:
# Hello
# World
In this example, the function return_multiple
returns two values. It does this by creating an array and then using echo
to print the entire array. The backticks around return_multiple
in the calling code capture the output of the function, which is then stored in another array result
.
Returning Strings
Bash doesn’t support returning strings from functions directly. However, we can use the echo
command to print a string, and then capture this output when the function is called. Here’s an example:
function return_string() {
local str="Hello, World!"
echo $str
}
result=`return_string`
echo $result
# Output:
# Hello, World!
In this example, the function return_string
uses echo
to print a string. The backticks around return_string
in the calling code capture this output, which is then stored in the variable result
.
These techniques allow us to overcome the limitations of the ‘return’ command, making our Bash scripts more flexible and powerful.
Exploring Alternative Approaches
While the ‘return’ command and ‘echo’ are commonly used to return values from Bash functions, there are other methods that offer more flexibility and control. Let’s explore two of these alternative approaches: using global variables and command substitution.
Utilizing Global Variables
One way to return values from a Bash function is by using global variables. A global variable is accessible from anywhere in the script, which means you can set it inside a function and then use it after the function has been called. Here’s an example:
function global_return() {
global_result="Hello, World!"
}
global_return
echo $global_result
# Output:
# Hello, World!
In this example, the function global_return
sets a global variable global_result
. After the function is called, we can use echo $global_result
to print the value of the global variable.
While this method is powerful and flexible, it can lead to issues if you’re not careful. Since global variables are accessible from anywhere in the script, they can be accidentally modified, leading to unexpected behavior.
Leveraging Command Substitution
Command substitution allows you to use the output of a command as an argument to another command. In the context of Bash functions, you can use command substitution to capture the output of a function. Here’s an example:
function command_substitution() {
local result="Hello, World!"
echo $result
}
result=$(command_substitution)
echo $result
# Output:
# Hello, World!
In this example, the function command_substitution
uses echo
to print a string. The command substitution $(command_substitution)
captures this output, which is then stored in the variable result
.
Command substitution is a powerful feature of Bash, but it should be used judiciously. Capturing the output of a function can be resource-intensive if the function produces a lot of output, and it can lead to issues if the output contains special characters or whitespace.
As with all programming techniques, the best approach depends on your specific needs and constraints. Understanding the various methods to return values from Bash functions and their trade-offs will help you make informed decisions and write more effective scripts.
Troubleshooting Common Issues with Bash Return Values
Bash scripting can be tricky, and returning values from functions is no exception. Let’s look at some common issues you might encounter and how to solve them.
Exceeding the Return Value Limit
As mentioned earlier, the ‘return’ command in Bash can only return an integer between 0 and 255. If you try to return a value outside this range, Bash will not throw an error, but the result will not be what you expect. Here’s an example:
function return_large_number() {
return 300
}
return_large_number
echo $?
# Output:
# 44
In this example, the function return_large_number
tries to return 300, which is outside the valid range. The actual return value ends up being 44, which is the remainder of 300 divided by 256.
To avoid this issue, make sure to only return values within the valid range, or consider using another method to return values, such as ‘echo’ or global variables.
Forgetting to Capture the Return Value
The return value of a function is stored in the special variable $?
, but it’s only available immediately after the function is called. If you call another command before capturing the return value, $?
will be overwritten. Here’s an example:
function return_value() {
return 10
}
return_value
echo "Hello, World!"
echo $?
# Output:
# Hello, World!
# 0
In this example, the function return_value
returns 10, but we don’t capture the return value immediately. Instead, we call echo "Hello, World!"
, which overwrites $?
. When we finally print $?
, it’s 0, which is the exit status of the echo
command, not the return value of return_value
.
To avoid this issue, always capture the return value immediately after calling the function.
Using Unset or Uninitialized Variables
In Bash, using an unset or uninitialized variable will not throw an error, but it can lead to unexpected results. Here’s an example:
function return_uninitialized() {
local result
echo $result
}
result=$(return_uninitialized)
echo "Result: $result"
# Output:
# Result:
In this example, the function return_uninitialized
tries to print the value of the local variable result
, which has not been initialized. As a result, the function output is empty, and the variable result
in the calling code is also empty.
To avoid this issue, always initialize your variables before using them, even if you’re just setting them to an empty string.
Understanding these common issues and their solutions will help you write more robust Bash scripts and avoid some of the pitfalls associated with returning values from functions.
Understanding Bash Functions and Exit Status
To fully comprehend the concept of returning values from Bash functions, it’s important to understand the basics of how functions work in Bash and the role of exit status.
Bash Functions: The Building Blocks
In Bash, a function is a named block of code that can be reused anywhere in your script. It’s like a mini-script within your script. Functions can take input in the form of parameters and return a value to the caller function using the ‘return’ command.
Here’s a simple example of a Bash function:
function greet() {
echo "Hello, $1!"
}
greet "World"
# Output:
# Hello, World!
In this example, greet
is a function that takes one parameter (represented by $1
) and prints a greeting message. The function is called with “World” as the argument, and it prints “Hello, World!”.
The Role of Exit Status
In Bash, every command that is executed returns an exit status (also known as a return status or exit code). This is a numeric value that represents the success or failure of the command. By convention, an exit status of 0 indicates success, while a non-zero exit status indicates an error.
The exit status of the last executed command is stored in a special variable $?
. This can be used to capture the return value of a function. For example:
function check_directory() {
cd $1
}
check_directory /nonexistent_directory
echo $?
# Output:
# 1
In this example, check_directory
is a function that tries to change the current directory to the one specified by the argument. If the directory does not exist, the cd
command fails and returns a non-zero exit status, which is captured by $?
and printed by echo $?
.
Understanding the concept of functions and exit status in Bash is fundamental to mastering the art of returning values from Bash functions.
Expanding Your Bash Scripting Skills
Returning values from Bash functions is a fundamental concept that plays a significant role when working with larger scripts or projects. As you gain proficiency in Bash scripting, you’ll find that the ‘return’ command often goes hand in hand with other key commands and functions.
Leveraging Return Values in Larger Scripts
In larger scripts, return values can serve as the cornerstone for decision-making processes. For instance, you might have a function that checks the availability of a certain resource, and based on its return value, your script might decide to proceed or abort the operation.
function check_file() {
if [[ -f $1 ]]; then
return 0 # file exists
else
return 1 # file does not exist
fi
}
check_file /path/to/myfile.txt
if [[ $? -eq 0 ]]; then
echo "Proceeding..."
else
echo "Aborting..."
fi
# Output (if file does not exist):
# Aborting...
In this example, the check_file
function checks if a certain file exists. Depending on the return value of this function, the script decides whether to proceed or abort the operation.
Complementary Commands and Functions
Commands such as ‘echo’, ‘printf’, and ‘read’, as well as constructs like arrays and loops, often accompany the ‘return’ command in typical use cases. Mastery of these commands and constructs, along with a good understanding of Bash function return values, will significantly enhance your Bash scripting prowess.
Further Resources for Bash Scripting Mastery
To continue your learning journey in Bash scripting, here are a few resources you might find helpful:
- GNU Bash Manual: The official manual for Bash, the GNU Project’s shell. It’s a comprehensive resource that covers all aspects of Bash scripting.
Return Values in Bash Functions: This Linux Journal article discusses the concept of return values in Bash functions.
Advanced Bash-Scripting Guide: An in-depth exploration of the art of scripting, including detailed descriptions of Bash’s command-line and scripting interfaces.
Remember, mastering Bash scripting is a journey. Keep experimenting, keep learning, and most importantly, have fun while doing it!
Wrapping Up: Mastering Bash Function Return Values
Throughout this comprehensive guide, we’ve delved into the intricacies of returning values from Bash functions. We’ve explored the fundamentals, the use of ‘return’ command, and the limitations it might present. Along the way, we’ve also navigated through advanced techniques like returning multiple values and strings, and alternative approaches including the use of global variables and command substitution.
We began with the basics, learning how to return a simple integer value using the ‘return’ command. Then, we ventured into more complex scenarios, understanding how to return multiple values and strings using arrays and the ‘echo’ command. We also looked at alternative approaches, leveraging global variables and command substitution to return values from functions.
Along the way, we tackled common issues you might encounter when returning values from Bash functions, such as exceeding the return value limit, forgetting to capture the return value, and using unset or uninitialized variables. We provided solutions and workarounds for each of these challenges, equipping you with the knowledge to write robust and effective Bash scripts.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity |
---|---|---|
‘return’ Command | Low (only returns integers 0-255) | Low |
‘echo’ Command | High (can return strings and multiple values) | Medium |
Global Variables | High (can return any value) | High (risk of accidental modification) |
Command Substitution | High (can return any output) | High (potential issues with large output) |
Whether you’re just starting out with Bash scripting or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of Bash function return values and how to handle them effectively.
The ability to return values from Bash functions is a powerful tool in your scripting arsenal, opening up new possibilities for creating complex, interactive scripts. Now, you’re well-equipped to harness this power. Happy scripting!