Python Pathlib Module | File Path Usage Guide
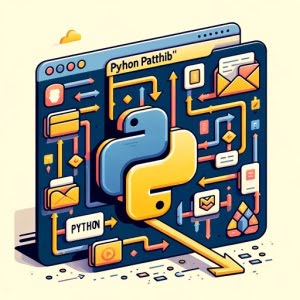
Are you finding it challenging to manage file paths in Python? You’re not alone. Many developers find themselves tangled in the web of file system paths. But, think of Python’s pathlib module as your GPS – versatile and handy for various tasks.
Whether you’re navigating directories, manipulating files, or even debugging, understanding how to use pathlib in Python can significantly streamline your coding process.
In this guide, we’ll walk you through the process of mastering file paths in Python with pathlib, from the basics to more advanced techniques. We’ll cover everything from creating Path objects, navigating the file system, to file operations and even alternative approaches.
Let’s get started!
TL;DR: How Do I Use the pathlib Module in Python?
The
pathlib
module in Python provides an object-oriented interface for dealing with file system paths. It simplifies the process of working with files and directories, making your code more readable and easier to understand.
Here’s a simple example:
from pathlib import Path
p = Path('.')
print(p.absolute())
# Output:
# '/Users/username/Current/Directory'
In this example, we import the Path class from the pathlib module. We then create a Path object representing the current directory (‘.’). Finally, we print the absolute path of the current directory using the absolute()
method.
This is just the tip of the iceberg when it comes to the pathlib module in Python. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Python Pathlib Basics: A Beginner’s Guide
- Advanced Python Pathlib: File Operations and More
- Exploring Alternatives to Python’s Pathlib
- Troubleshooting Common Issues with Python’s Pathlib
- Understanding File System Paths in Python
- From os.path to pathlib: A Shift in Paradigm
- Exploring the Impact of Python’s Pathlib Module
- Wrapping Up: Mastering Python’s Pathlib Module
Python Pathlib Basics: A Beginner’s Guide
The pathlib module in Python introduces classes for handling filesystem paths with semantics appropriate for different operating systems. Let’s start by understanding how to create Path objects and navigate the file system.
Creating Path Objects
In pathlib, everything starts by creating a Path
object. You can create a Path object for the current directory, a specific directory, or even a specific file.
from pathlib import Path
# Path representing the current directory
p1 = Path('.')
print(p1)
# Path representing a specific directory
p2 = Path('/usr/local/bin')
print(p2)
# Path representing a specific file
p3 = Path('/usr/local/bin/python3')
print(p3)
# Output:
# .
# /usr/local/bin
# /usr/local/bin/python3
In the above code, we created three Path objects – one for the current directory, one for a specific directory, and one for a specific file. The Path
class automatically handles the differences between different operating systems.
Navigating the File System
Once you have a Path object, you can use various methods to navigate the file system. Let’s see how we can get the current directory and navigate to other directories.
from pathlib import Path
# Get the current directory
p = Path('.')
print(p.absolute())
# Navigate to the parent directory
parent = p.parent
print(parent.absolute())
# Output:
# /Users/username/Current/Directory
# /Users/username
In the above code, we first print the absolute path of the current directory. Then, we navigate to the parent directory using the parent
property and print its absolute path.
The pathlib module provides a more intuitive and high-level interface for dealing with file paths in Python. It’s a powerful tool that can make your code cleaner and easier to understand.
Advanced Python Pathlib: File Operations and More
After mastering the basics of Python’s pathlib module, it’s time to dive into some of its more advanced features. In this section, we’ll explore file operations like creating, deleting, and renaming files, checking file attributes, and using glob patterns.
File Operations with Pathlib
Pathlib provides several methods to perform file operations. Let’s see how to create, delete, and rename files.
from pathlib import Path
# Create a new file
p = Path('new_file.txt')
p.touch()
# Rename the file
p.rename('renamed_file.txt')
# Delete the file
p.unlink()
# Output:
# No output, but 'new_file.txt' will be created, renamed to 'renamed_file.txt', and then deleted.
In the above code, we first create a new file using the touch()
method. Then, we rename the file using the rename()
method. Finally, we delete the file using the unlink()
method. Pathlib handles all these operations in a simple and intuitive way.
Checking File Attributes
Pathlib also allows you to check various file attributes. Let’s see how to check if a path exists, if it’s a file or a directory, and get its size.
from pathlib import Path
p = Path('/usr/local/bin/python3')
# Check if the path exists
print(p.exists())
# Check if it's a file
print(p.is_file())
# Check if it's a directory
print(p.is_dir())
# Get the file size
print(p.stat().st_size)
# Output:
# True
# True
# False
# 10289152
In the above code, we first check if the path exists using the exists()
method. Then, we check if it’s a file using the is_file()
method and if it’s a directory using the is_dir()
method. Finally, we get the file size using the stat().st_size
attribute.
Using Glob Patterns
Pathlib supports glob patterns, which can be extremely useful when you need to list files that match a certain pattern.
from pathlib import Path
p = Path('.')
# List all Python files in the current directory
for file in p.glob('*.py'):
print(file)
# Output:
# sample1.py
# sample2.py
# ...
In the above code, we use the glob()
method to list all Python files in the current directory. The glob()
method returns a generator, which we can iterate over to get the matching files.
The advanced features of pathlib provide a powerful and flexible toolset for handling file paths in Python. By understanding these features, you can write more efficient and cleaner code.
Exploring Alternatives to Python’s Pathlib
While the pathlib module offers a modern, object-oriented approach to handling file paths in Python, it’s not the only option available. Let’s take a look at some alternative methods, including the traditional os.path module and third-party libraries.
Traditional Approach: os.path Module
Before pathlib was introduced in Python 3.4, the os.path module was the go-to option for handling file paths. It provides functions to manipulate file paths and check properties of files and directories.
import os
# Get the current directory
print(os.getcwd())
# Get the absolute path of a file
print(os.path.abspath('file.txt'))
# Output:
# /Users/username/Current/Directory
# /Users/username/Current/Directory/file.txt
In the above code, we use os.getcwd()
to get the current directory and os.path.abspath()
to get the absolute path of a file. While os.path is powerful and flexible, its function-based interface can be less intuitive than pathlib’s object-oriented approach.
Third-Party Libraries: PyFilesystem2
PyFilesystem2 is a third-party library that provides a unified interface to different types of filesystems. It supports local filesystems, network filesystems, and even in-memory filesystems.
from fs.osfs import OSFS
# Create a filesystem object for the current directory
fs = OSFS('.')
# List all files in the current directory
print(fs.listdir('.'))
# Output:
# ['file1.txt', 'file2.txt', ...]
In the above code, we create a filesystem object for the current directory using OSFS()
. We then list all files in the current directory using listdir()
. PyFilesystem2 offers a high level of abstraction, but it may be overkill for simple file path operations.
Choosing the right tool for handling file paths in Python depends on your specific needs. If you prefer an object-oriented approach and are using Python 3.4 or later, pathlib is a great choice. If you’re working with older code or prefer a function-based interface, os.path is a reliable option. And if you need a high level of abstraction and don’t mind installing a third-party library, PyFilesystem2 is worth considering.
Troubleshooting Common Issues with Python’s Pathlib
While the pathlib module is a powerful tool for handling file paths in Python, it’s not without its quirks. In this section, we’ll discuss some common issues you may encounter when using pathlib, such as handling different file systems and dealing with symbolic links.
Handling Different File Systems
One of the challenges of working with file paths is that different operating systems use different conventions. For example, Windows uses backslashes (\
) while Unix-based systems like Linux and macOS use forward slashes (/
). Fortunately, pathlib automatically handles these differences for you.
However, when you need to create a Path object for a specific file system, you can use the PurePosixPath
or PureWindowsPath
classes.
from pathlib import PurePosixPath, PureWindowsPath
# Create a POSIX path
p1 = PurePosixPath('/usr/local/bin')
print(p1)
# Create a Windows path
p2 = PureWindowsPath('C:\Windows\System32')
print(p2)
# Output:
# /usr/local/bin
# C:\Windows\System32
In the above code, we create a POSIX path and a Windows path using the PurePosixPath
and PureWindowsPath
classes, respectively. These classes allow you to create paths that follow the conventions of a specific file system.
Dealing with Symbolic Links
Symbolic links, or symlinks, are a type of file that points to another file or directory. When you’re dealing with symlinks, you may want to know if a path is a symlink, get the target of a symlink, or resolve a symlink to its target.
from pathlib import Path
# Create a symlink
Path('target.txt').touch()
Path('symlink.txt').symlink_to('target.txt')
# Check if a path is a symlink
print(Path('symlink.txt').is_symlink())
# Get the target of a symlink
print(Path('symlink.txt').resolve())
# Output:
# True
# /Users/username/Current/Directory/target.txt
In the above code, we first create a symlink using the symlink_to()
method. We then check if a path is a symlink using the is_symlink()
method and get the target of a symlink using the resolve()
method.
Understanding these common issues and their solutions can help you use pathlib more effectively. Remember, the key to mastering any tool is practice and experience.
Understanding File System Paths in Python
Before we dive any deeper into the pathlib module, it’s important to understand what file system paths are and why they’re so crucial in Python programming.
File system paths are the addresses where data is stored in your computer. They can point to files, directories, or even devices. When you’re writing a Python program that reads from or writes to a file, you need to tell Python where that file is located. That’s where file system paths come in.
# Open a file in the current directory
f = open('file.txt', 'r')
# Open a file in a specific directory
f = open('/Users/username/Documents/file.txt', 'r')
# Output:
# No output, but the file is opened for reading.
In the above code, we open a file in the current directory and a file in a specific directory. The string we pass to the open()
function is the file system path.
From os.path to pathlib: A Shift in Paradigm
The traditional way of working with file system paths in Python is using the os.path module. This module provides functions like os.path.join()
, os.path.split()
, and os.path.abspath()
to manipulate file paths.
However, the os.path module has a few shortcomings. It’s not object-oriented, which means you can’t use methods on the paths. It also doesn’t support the ‘/’ operator for joining paths, which can make your code less readable.
The pathlib module was introduced in Python 3.4 to address these issues. It provides an object-oriented interface for working with file paths. You can call methods on Path objects, use the ‘/’ operator to join paths, and do much more.
from pathlib import Path
# Join paths using the '/' operator
p = Path('/usr') / 'local' / 'bin'
print(p)
# Output:
# /usr/local/bin
In the above code, we create a Path object and join paths using the ‘/’ operator. This is more intuitive and readable than using os.path.join()
.
The shift from os.path to pathlib represents a broader trend in Python towards a more intuitive and high-level interface for common tasks. By understanding the fundamentals of file system paths and the benefits of pathlib, you’ll be well-equipped to write efficient and readable code.
Exploring the Impact of Python’s Pathlib Module
The pathlib module in Python is not just a tool for handling file paths. It’s a versatile library that plays a crucial role in various areas of Python programming, from file handling and data processing to web development and more.
Pathlib in File Handling
File handling is one of the most common tasks in Python programming. Whether you’re reading data from a CSV file, writing results to a log file, or managing a database, you’ll need to work with file paths. And that’s where pathlib comes in.
from pathlib import Path
# Write to a file
p = Path('file.txt')
p.write_text('Hello, World!')
# Read from a file
print(p.read_text())
# Output:
# 'Hello, World!'
In the above code, we use the write_text()
and read_text()
methods of a Path object to write to and read from a file. These methods make file handling in Python simple and intuitive.
Pathlib in Data Processing
In data processing, you often need to read data from multiple files, write results to different files, or even manage a directory of files. Pathlib provides powerful tools to handle these tasks.
from pathlib import Path
# List all CSV files in a directory
p = Path('.')
for file in p.glob('*.csv'):
# Read data from the file
data = file.read_text()
# Process the data...
In the above code, we use the glob()
method to list all CSV files in a directory and read data from each file. This makes it easy to process data from multiple files.
Pathlib in Web Development
In web development, you often need to manage static files like HTML, CSS, and JavaScript files. Pathlib can help you manage these files efficiently.
from pathlib import Path
# Get the path of a static file
p = Path('static') / 'css' / 'style.css'
# Serve the static file in a web application...
In the above code, we use the ‘/’ operator to get the path of a static file. This makes it easy to manage static files in a web application.
Further Resources for Mastering Python’s Pathlib Module
If you want to dive deeper into the pathlib module and related concepts, here are some resources you might find useful:
- Python OS Module Simplified – Discover essential functions for managing files, paths, and directories in Python.
Writing to Files in Python – A quick guide on Python’s file writing tools and how to store data in files.
File Management with Python os.listdir() – Discover how to explore file contents and streamline file management.
Python’s official pathlib documentation provides an overview of the pathlib module’s classes and methods.
This Python file and directory handling guide provides an introduction to Python file handling and the pathlib module.
Python’s official input and output tutorial covers the basics of file I/O in Python, which is a concept related to file paths.
By exploring these resources and practicing with real-world examples, you can master the pathlib module and improve your Python programming skills.
Wrapping Up: Mastering Python’s Pathlib Module
In this comprehensive guide, we’ve navigated the intricate world of Python’s pathlib module, an object-oriented library designed to handle file system paths. From understanding the basics to exploring more advanced functionalities, we’ve covered the ins and outs of how pathlib can simplify and streamline your code when dealing with file paths.
We began with the basics, learning how to create Path objects and navigate the file system, and then dived into more advanced usage scenarios, such as file operations, checking file attributes, and using glob patterns. We also tackled common issues you might encounter when using pathlib, like handling different file systems and dealing with symbolic links, providing you with solutions and workarounds for each issue.
In addition, we explored alternative approaches to handling file paths in Python, comparing the pathlib module with the traditional os.path module and the third-party library PyFilesystem2. Here’s a quick comparison of these methods:
Method | Object-Oriented | Ease of Use | Flexibility |
---|---|---|---|
Pathlib | Yes | High | High |
os.path | No | Moderate | High |
PyFilesystem2 | Yes | High | Very High |
Whether you’re a Python beginner just starting to grapple with file paths, or an experienced developer looking to level up your file handling skills, we hope this guide has given you a deeper understanding of Python’s pathlib module and its capabilities.
With its balance of intuitiveness, efficiency, and versatility, pathlib is an invaluable tool for any Python developer’s toolkit. Happy coding!