Java Functions Explained: Your Ultimate Guide
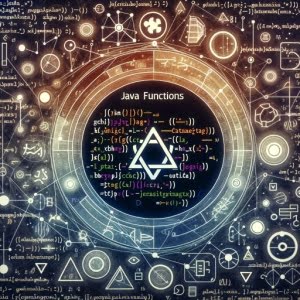
Are you finding it challenging to structure your code in Java? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a chef’s recipe, functions in Java are the building blocks of any Java program. They help you organize and reuse your code, making your programming life a whole lot easier.
This guide will walk you through everything you need to know about Java functions, from basic use to advanced techniques. We’ll explore Java functions’ core functionality, delve into their advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Java functions!
TL;DR: What is a Function in Java?
A function in Java is a block of code that performs a specific task:
void functionName() { //task to perform when called; }
It is declared with a name, a return type, and optionally, parameters. Functions are essential for structuring and reusing code in Java.
Here’s a simple example:
void greet() {
System.out.println('Hello, World!');
}
// Output:
// 'Hello, World!'
In this example, we’ve declared a function named ‘greet’. This function prints ‘Hello, World!’ when called. The ‘void’ keyword indicates that this function does not return any value.
This is just a basic introduction to Java functions. There’s a lot more to learn about how to use them effectively, including advanced techniques and common pitfalls. Continue reading for a comprehensive guide on mastering Java functions.
Table of Contents
- Declaring and Calling Functions in Java: The Basics
- Advantages of Using Functions
- Common Pitfalls with Functions
- Diving Deeper: Function Overloading and Recursion
- Exploring Alternatives: Loops and Third-Party Libraries
- Troubleshooting Java Functions: Common Issues and Solutions
- Unpacking the Concept of Functions
- The Importance of Functions in Code Organization and Reusability
- Applying Java Functions in Larger Projects
- Wrapping Up: Mastering Java Functions
Declaring and Calling Functions in Java: The Basics
Java functions, also known as methods, are declared within a class. A function declaration includes its name, return type, and optionally, parameters. The function body, enclosed between braces, contains the code that will be executed when the function is called.
Let’s look at a simple example of declaring and calling a function in Java:
public class HelloWorld {
// declaring the function
static void greet() {
System.out.println('Hello, World!');
}
public static void main(String[] args) {
// calling the function
greet();
}
}
// Output:
// 'Hello, World!'
In this example, we declare a function named greet
inside the HelloWorld
class. The void
keyword indicates that this function does not return any value. Inside the main
function, we call the greet
function with greet();
. When the program runs, it calls the main
function, which in turn calls the greet
function, and ‘Hello, World!’ is printed to the console.
Advantages of Using Functions
Functions provide several benefits in Java programming:
- Code Reusability: Once a function is defined, it can be used multiple times throughout your code, preventing the need for code duplication.
Code Organization: Functions allow you to break down complex code into manageable pieces, making your code easier to read and maintain.
Modularity: Functions enable you to create modular code, where each function performs a specific task. This makes debugging and testing easier.
Common Pitfalls with Functions
While functions are extremely useful, there are potential pitfalls to watch out for:
- Incorrect Function Call: If you call a function before it’s been declared, you’ll get a compile-time error. Always make sure your functions are declared before you call them.
Parameter Mismatch: If the number or type of parameters in the function call does not match the function declaration, you’ll get a compile-time error. Ensure the parameters match in both the function call and declaration.
Infinite Recursion: If a function calls itself (either directly or indirectly) without a terminating condition, it can lead to infinite recursion, causing the program to crash. Always have a terminating condition when using recursion.
Diving Deeper: Function Overloading and Recursion
As you become more comfortable with Java functions, you can start to explore more advanced techniques, such as function overloading and recursion.
Function Overloading in Java
Function overloading allows you to define multiple functions with the same name but different parameters. It’s a way of giving a single function multiple ways to perform a task.
Here’s an example of function overloading in Java:
public class Main {
static void display(int num) {
System.out.println('Displaying an integer: ' + num);
}
static void display(double num) {
System.out.println('Displaying a double: ' + num);
}
public static void main(String[] args) {
display(5);
display(15.6);
}
}
// Output:
// 'Displaying an integer: 5'
// 'Displaying a double: 15.6'
In this code, we have two display
functions. The first one takes an integer as a parameter, while the second one takes a double. When we call display(5);
in the main
function, the first display
function is executed. When we call display(15.6);
, the second display
function is executed.
Recursion in Java
Recursion is a process where a function calls itself as a subroutine. This allows the function to be repeated several times, as it can call itself during its execution.
Here’s a simple example of a recursive function in Java:
public class Main {
static int factorial(int n) {
if (n == 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
public static void main(String[] args) {
System.out.println(factorial(5));
}
}
// Output:
// '120'
In this code, we define a function factorial
that calculates the factorial of a number. The function calls itself to calculate the factorial, leading to recursion. The recursion continues until the number is 1, at which point the function returns 1 and the recursion ends.
Pros and Cons of Advanced Techniques
While these advanced techniques can make your code more flexible and powerful, they also come with their own challenges.
Pros:
- Flexibility: Function overloading allows a single function to perform different tasks, making your code more flexible.
Simplicity: Recursion can simplify your code by breaking down complex tasks into simpler ones.
Cons:
- Complexity: Overloaded functions can make your code harder to read and debug, as it may not be immediately clear which function is being called.
Performance: Recursive functions can be less efficient and may cause a stack overflow if the recursion goes too deep.
Exploring Alternatives: Loops and Third-Party Libraries
While functions are a fundamental part of Java programming, there are alternative approaches to structuring your code. Let’s explore two of these: using loops and leveraging third-party libraries.
Structuring Code with Loops
Loops in Java allow you to repeatedly execute a block of code as long as a certain condition is met. They can be a useful alternative to functions for performing repetitive tasks.
Here’s a simple example of a loop in Java:
public class Main {
public static void main(String[] args) {
for (int i = 0; i < 5; i++) {
System.out.println('Loop iteration: ' + i);
}
}
}
// Output:
// 'Loop iteration: 0'
// 'Loop iteration: 1'
// 'Loop iteration: 2'
// 'Loop iteration: 3'
// 'Loop iteration: 4'
In this code, we use a for
loop to print the phrase ‘Loop iteration: ‘ followed by the current iteration number. The loop continues until i
is no longer less than 5.
Leveraging Third-Party Libraries
Third-party libraries can provide pre-written functions that perform specific tasks, saving you the time and effort of writing these functions yourself. For instance, Apache Commons Lang provides a host of utility functions that Java developers find useful.
Here’s an example of how to use a function from a third-party library:
// Add the library to your project
import org.apache.commons.lang3.StringUtils;
public class Main {
public static void main(String[] args) {
String reversed = StringUtils.reverse('Hello, World!');
System.out.println(reversed);
}
}
// Output:
// '!dlroW ,olleH'
In this code, we use the reverse
function from the StringUtils
class in Apache Commons Lang to reverse the string ‘Hello, World!’.
Pros and Cons of Alternative Approaches
Like everything in programming, these alternative approaches have their own benefits and drawbacks.
Pros:
- Simplicity: Loops can be a simpler alternative to functions for performing repetitive tasks.
Efficiency: Third-party libraries can save you time and effort by providing pre-written functions.
Cons:
- Limited Flexibility: Loops are less flexible than functions and can make your code more complex if the task is not inherently repetitive.
Dependency: Using third-party libraries makes your code dependent on external code. If the library is not well-maintained, it can lead to issues in your code.
As always, the best approach depends on your specific scenario. Consider the task at hand, the complexity of your code, and the resources available to you when deciding how to structure your code.
Troubleshooting Java Functions: Common Issues and Solutions
Working with functions in Java can sometimes lead to unexpected issues. Let’s discuss some common problems you might encounter and how to resolve them.
Syntax Errors
When declaring and calling functions, you might face syntax errors. These could be due to incorrect function names, mismatched parameters, or missing semicolons.
Here’s an example of a syntax error:
public class Main {
static void greet() {
System.out.println('Hello, World!') // missing semicolon
}
}
In the above code, the missing semicolon after the println
statement will cause a compile-time error. To fix this, simply add a semicolon at the end of the statement.
Scope Issues
Scope issues arise when you try to access a variable outside of its scope. For instance, a variable declared inside a function cannot be accessed outside of that function.
Here’s an example of a scope issue:
public class Main {
static void greet() {
String greeting = 'Hello, World!';
}
public static void main(String[] args) {
greet();
System.out.println(greeting); // scope issue
}
}
In this code, we’re trying to print the greeting
variable, which is declared inside the greet
function. This will cause a compile-time error because greeting
is out of scope in the main
function. To fix this, you can declare greeting
at the class level, making it accessible to all functions within the class.
Other Considerations
When working with functions, it’s also important to consider the function’s return type. If your function is declared with a return type other than void
, it must return a value of that type.
Here’s an example:
public class Main {
static int add(int a, int b) {
int sum = a + b;
// missing return statement
}
}
In the above code, the add
function is declared to return an int
, but there’s no return statement in the function. This will cause a compile-time error. To fix this, simply add a return statement that returns the sum.
public class Main {
static int add(int a, int b) {
int sum = a + b;
return sum;
}
}
By being aware of these common issues and knowing how to resolve them, you can work more effectively with functions in Java and avoid many common pitfalls.
Unpacking the Concept of Functions
In programming, a function is a self-contained module of code that carries out a specific task. You can think of a function as a machine: it takes in inputs (if any), performs an operation, and produces outputs (if any).
Here’s a simple function in Java:
public class Main {
static int add(int a, int b) {
int sum = a + b;
return sum;
}
public static void main(String[] args) {
int result = add(5, 3);
System.out.println(result);
}
}
// Output:
// '8'
In this code, we have a function named add
that takes two integers as input, adds them together, and returns the result. We then call this function in the main
function, passing in 5 and 3 as arguments, and print the result.
The Importance of Functions in Code Organization and Reusability
Functions play a crucial role in organizing and structuring code in programming. They allow you to break down complex problems into smaller, more manageable tasks. Each function performs a specific task, making your code easier to understand and debug.
Functions also promote code reusability. Once a function is defined, it can be used over and over again in your program. This saves you from having to write the same code multiple times. In our example, if we wanted to add two numbers in multiple places in our program, we could simply call the add
function each time, instead of writing the addition code again and again.
By understanding the fundamentals of functions and their importance in code organization and reusability, you can write more efficient and maintainable code in Java.
Applying Java Functions in Larger Projects
As you progress in your Java journey, you’ll find that functions become increasingly important in larger projects. A well-structured function can make the difference between a project that’s manageable and one that’s a tangled web of code.
Beyond the basic and advanced use of functions, you can explore related concepts that will further enhance your programming skills.
Diving into Object-Oriented Programming
Java is an object-oriented programming (OOP) language, and understanding OOP concepts is key to mastering Java. Functions in OOP, often called methods, are associated with objects and can manipulate the object’s data. This encapsulation of functions within objects allows for more organized and modular code.
public class Greeting {
String greeting;
Greeting(String g) {
this.greeting = g;
}
void greet() {
System.out.println(greeting);
}
}
public class Main {
public static void main(String[] args) {
Greeting hello = new Greeting('Hello, World!');
hello.greet();
}
}
// Output:
// 'Hello, World!'
In this example, we create a Greeting
class with a greet
method. We then create an instance of Greeting
and call its greet
method.
Exploring Data Structures
Data structures, such as arrays, linked lists, and trees, are another fundamental concept in Java programming. Functions play a key role in manipulating these data structures.
public class Main {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
System.out.println(number);
}
}
}
// Output:
// '1'
// '2'
// '3'
// '4'
// '5'
In this code, we use a function (the main
function) to iterate over an array of integers and print each one.
Further Resources for Mastering Java Functions
To deepen your understanding of Java functions and related concepts, here are some resources you might find useful:
- Tips and Techniques for Learning Java – Dive into Java development with this tutorial.
Java REPL Overview – Discover Java REPL (Read-Eval-Print Loop) environments for interactive Java programming.
Java Code Examples Showcase – A collection of Java code examples covering various concepts and techniques.
Oracle’s Java Documentation is a comprehensive resource for understanding Java programming, including functions.
Java Programming Masterclass covers everything from Java basics to advanced concepts.
GeeksforGeeks’ Java Library provides detailed guides and tutorials on various Java functions and data structures.
Wrapping Up: Mastering Java Functions
In this comprehensive guide, we’ve delved into the heart of Java programming – functions. We’ve explored how these building blocks of code can help you structure your programs, from simple tasks to complex operations.
We began with the basics, learning how to declare and call functions in Java. We then moved on to more advanced techniques, such as function overloading and recursion, providing you with tools to make your code more flexible and powerful.
Along the way, we tackled common issues that you might encounter when working with functions, such as syntax errors and scope issues, equipping you with the knowledge to troubleshoot these problems effectively. We also explored alternative approaches to structuring your code, such as using loops and third-party libraries, giving you a broader perspective on code organization in Java.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Functions | Code reusability, organization, and modularity | Can lead to complex code if not managed well |
Loops | Simplicity for repetitive tasks | Limited flexibility |
Third-Party Libraries | Pre-written functions, time-saving | Dependency on external code |
Whether you’re just starting out with Java or you’re looking to deepen your understanding of functions, we hope this guide has provided you with valuable insights and practical knowledge.
Mastering functions is a critical step in becoming a proficient Java programmer. With this guide, you’re well on your way to writing more efficient, organized, and reusable code. Happy coding!