Using Python Heapq Module for Heaps and Priority Queues
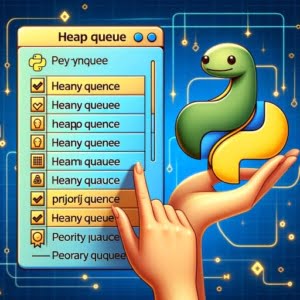
Welcome to the fascinating world of Python’s heapq module. This powerful tool is not just a simple module; it’s a versatile asset that introduces the concepts of priority queues and heaps into your Python programs.
Priority queues and heaps might seem like complex concepts, but consider this. Imagine you have a to-do list. You could tackle your tasks in the order you wrote them down, but what if some tasks are more urgent or important than others? You’d want to prioritize those tasks, right? That’s where the concept of a priority queue comes in. It’s like a refined version of your to-do list that serves tasks based on their priority.
Now, imagine you could organize this priority-based to-do list in a tree-like structure where each task (node) has a value greater than or equal to its subtasks (children). This would make it easier to manage and sort your tasks. This is what we call a Heap.
Python’s heapq module is an efficient tool that brings these concepts to life using the binary heap data structure. In this blog post, we’ll dive deep into heapq, exploring its functions, understanding its efficiency, and seeing it in action. Ready to uncover the power and versatility of heapq? Let’s get started!
TL;DR: What is Python’s heapq module?
Python’s heapq module is a powerful tool that implements the heap queue algorithm (priority queue algorithm) using the binary heap data structure. It provides functions to create a heap, add/remove elements, and perform heap operations efficiently. For a more in-depth understanding and practical usage of heapq, continue reading the article.
import heapq
# Create a heap
numbers = [3, 2, 1, 5, 6, 4]
heapq.heapify(numbers)
print(numbers) # prints: [1, 2, 3, 5, 6, 4]
Priority Queue and Heap work hand in hand to solve complex programming problems. They provide an efficient way to manage data in programs where priority matters. But implementing a priority queue using a heap can be challenging.
That’s where Python’s heapq module comes into play. It addresses these challenges by storing entries as a 3-element list. This list includes the priority of the entry, an entry count, and the task itself. This ingenious approach allows Python’s heapq module to efficiently implement Priority Queues.
Table of Contents
Understanding Priority Queues and Heaps
Let’s delve deeper into the concepts of Priority Queues and Heaps, which are fundamental to the working of Python’s heapq module.
Priority Queues
Imagine a regular queue – a line at the grocery store, for instance. You join the line, and you wait your turn. This is a simple first-in, first-out (FIFO) concept. But what if we could refine this? What if we could decide who gets served next based on their ‘priority’? That’s exactly what a Priority Queue does. It’s a refined version of a queue that serves elements based on their priority.
Example of a Priority Queue using heapq:
import heapq
# Create a priority queue
pq = []
heapq.heappush(pq, (2, 'code'))
heapq.heappush(pq, (1, 'eat'))
heapq.heappush(pq, (3, 'sleep'))
while pq:
next_item = heapq.heappop(pq)
print(next_item)
This will output the tasks in the order of their priority.
Heaps
Now, let’s turn our attention to a Heap. A Heap is a special tree-based data structure that satisfies the heap property. If we visualize it, a Heap is like a binary tree. But what makes it special? It’s all about the parent-child relationship.
In a Heap, for any given node I, the value of I is greater than or equal to the values of its children. This property holds true for every single node in the Heap.
Heaps can be of two types: max-heap and min-heap. In a max-heap, the parent node is always larger than or equal to its child nodes. Conversely, in a min-heap, the parent node is less than or equal to its child nodes.
Heap Type | Parent-Child Relationship |
---|---|
Max-Heap | Parent >= Children |
Min-Heap | Parent <= Children |
This table shows the difference between a max-heap and a min-heap.
Binary Heap
You might be wondering, what’s a Binary Heap? A Binary Heap is a complete binary tree that maintains the heap property. It’s a crucial concept when it comes to implementing Priority Queues.
Binary Heap and heapq
How does heapq use the Binary Heap to solve programming problems? The heapq module provides several functions that utilize the Binary Heap to perform various operations.
These functions allow us to easily create a heap, add elements to it, remove elements from it, and even change the heap elements.
Creating a heap using the heapq module is straightforward. You just need to call the heapify
function on a list. Here’s how you can do it:
import heapq
numbers = [3, 2, 1, 5, 6, 4]
heapq.heapify(numbers)
print(numbers)
This will output: [1, 2, 3, 5, 6, 4]
, which is a Min heap.
In the output, the first element is the smallest which shows it’s a Min heap.
heapq Functions
The real power of Python’s heapq module lies in its functions. It provides seven core functions that allow us to create, manipulate, and use heaps efficiently. Let’s take a closer look at each of these functions.
heapify(iterable)
This function transforms a regular list into a heap. In other words, it rearranges the list in-place into a Min heap.
Example:
import heapq
numbers = [3, 2, 1, 5, 6, 4]
heapq.heapify(numbers)
print(numbers)
In the output, the first element is the smallest which shows it’s a Min heap.
heappush(heap, ele)
This function inserts an element into the heap while maintaining the heap property.
Example:
import heapq
heap = []
heapq.heappush(heap, 3)
heapq.heappush(heap, 2)
heapq.heappush(heap, 5)
print(heap)
In the output, the first element is the smallest which shows it’s a Min heap.
heappop(heap)
This function removes and returns the smallest element from the heap, preserving the heap property.
Example:
import heapq
heap = [2, 3, 5, 7, 9, 4]
print(heapq.heappop(heap))
print(heap)
The heappop
function removes and returns the smallest element from the heap. The output shows the smallest element and the updated heap.
heappushpop(heap, ele)
This function combines the pushing and popping operations into one, enhancing operational efficiency. It pushes the element into the heap and then pops and returns the smallest element.
Example:
import heapq
heap = [2, 3, 5, 7, 9, 4]
print(heapq.heappushpop(heap, 1))
print(heap)
The heappushpop
function pushes the new element into the heap, then pops and returns the smallest element. The output shows the smallest element and the updated heap.
heapreplace(heap, ele)
Similar to heappushpop
, this function pops and returns the smallest element, and then pushes the new element into the heap.
Example:
import heapq
heap = [2, 3, 5, 7, 9, 4]
print(heapq.heapreplace(heap, 1))
print(heap)
The heapreplace
function pops and returns the smallest element, then pushes the new element into the heap. The output shows the smallest element and the updated heap.
nlargest(n, iterable, key = fun)
One of the main strengths of the heapq module is its efficiency. For instance, if you want to find the largest numbers from a list, you can use the nlargest
function.
Example of using the nlargest
function:
import heapq
numbers = [1, 3, 5, 7, 9, 2, 4, 6, 8, 0]
print(heapq.nlargest(3, numbers))
This will output the 3 largest numbers in the list. This function is much faster than sorting the entire list and then slicing the largest elements.
When it comes to dealing with large datasets, heapq truly shines. It’s significantly more efficient than Python’s sort function, especially when the data is huge.
This is because the heapq module performs operations directly on the heap, which optimizes memory usage and improves speed.
nsmallest(n, iterable, key = fun)
This function returns the ‘n’ smallest elements from the iterable, based on the key function.
Example:
import heapq
numbers = [1, 3, 5, 7, 9, 2, 4, 6, 8, 0]
print(heapq.nsmallest(3, numbers))
The nsmallest
function returns the 3 smallest numbers in the list.
Functions Summary
As you can see, each function provided by the heapq module has its unique purpose and utility. They are efficient and fast, making them ideal for manipulating and managing heaps.
Moreover, you can combine these functions to perform more complex operations. For instance, you can use heappushpop
or heapreplace
to efficiently manage a heap while performing simultaneous push and pop operations.
These functions perform heap operations directly on lists, optimizing memory usage and enhancing operational efficiency. With Python’s heapq module, managing heaps has never been easier!
Applying heapq Functions: Practical Exercises
Now that we’ve learned about the heapq module and its functions, it’s time to put our knowledge into practice. We’ll try out some practical exercises to demonstrate the use of heapq functions.
This hands-on approach will not only help us understand how these functions work but also showcase their efficiency in solving real-world problems. So, let’s roll up our sleeves and get coding!
Exercise 1: Creating a Heap
Let’s start by creating a heap. We’ll use a list of numbers and transform it into a heap using the heapify
function.
import heapq
numbers = [3, 2, 1, 5, 6, 4]
heapq.heapify(numbers)
print(numbers)
When you run this code, you’ll see that the list numbers
has been rearranged into a heap. The output will be [1, 2, 3, 5, 6, 4]
.
Exercise 2: Inserting Elements into the Heap
Next, let’s try inserting elements into the heap. We’ll use the heappush
function for this.
import heapq
heap = []
heapq.heappush(heap, 3)
heapq.heappush(heap, 2)
heapq.heappush(heap, 5)
print(heap)
After running this code, you’ll see that the elements have been inserted into the heap while maintaining the heap property. The output will be [2, 3, 5]
.
Exercise 3: Removing Elements from the Heap
Now, let’s try removing the smallest element from the heap using the heappop
function.
import heapq
heap = [2, 3, 5, 7, 9, 4]
print(heapq.heappop(heap))
print(heap)
This code will remove and return the smallest element from the heap. The output will be 2
and the updated heap will be [3, 4, 5, 7, 9]
.
These exercises should give you a good sense of how to use Python’s heapq functions.
Further Resources for Python Modules
If you are interested in exploring module installation and distribution with packaging tools, Click Here
To further enhance your proficiency in Python Modules, we suggest utilizing these other resources:
- Python Socket Programming: A Quick Guide on Python’s “socket” module for network communication.
Python ZIP File Handling Simplified – Dive into zip file creation, extraction, and modification in Python.
Python Queue and Stack Tutorial – A detailed Youtube video tutorial on implementing queues and stacks in Python.
Stacks and Queues in Python by GeeksforGeeks explains the implementation of stacks and queues in Python.
How to Implement a Queue in Python – An explanation by Educative on how to implement queues in Python effectively.
Final Thoughts
We’ve embarked on an enlightening journey exploring Python’s heapq module. We’ve dug into the basics of Priority Queues and Heaps, and unveiled how Python’s heapq module provides a dynamic and efficient tool to implement these concepts using the binary heap data structure.
We’ve also dissected the seven core functions provided by the heapq module, each with its unique purpose and utility, and applied them through practical exercises.
For a deeper dive, click here.
The next time you’re faced with a complex programming problem, don’t forget to consider if heapq might be the right tool for the job. It’s a tool that can simplify your tasks and make your life much easier, and that’s the power of Python’s heapq module.