Python Socket Programming Guide (With Examples)
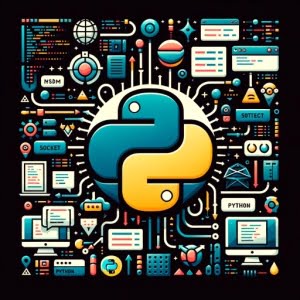
Are you finding it challenging to get a grip on Python sockets? Think of Python sockets like a virtual postman. They are the key to enabling communication between two nodes on a network.
Whether you are a beginner just dipping your toes into the world of Python sockets or an advanced user looking to refine your skills, this guide is designed for you.
We will walk you through everything you need to know about Python sockets, from the basic to the advanced usage. By the end of this guide, you will have a solid understanding of Python sockets and how to use them effectively in your projects.
TL;DR: What is a Python Socket?
A Python socket is a module in Python that provides a way for two computers to communicate. It’s like a virtual postman, delivering messages between two nodes on a network.
Here’s a simple example of a Python socket:
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect(('localhost', 12345))
# Output:
# Establishes a connection to the server at localhost on port 12345
In this example, we first import the socket module. We then create a socket object s
using the socket()
function. The arguments socket.AF_INET
and socket.SOCK_STREAM
specify the address family and socket type. Finally, we use the connect()
method to establish a connection to the server at ‘localhost’ on port 12345.
This is a basic way to use a Python socket, but there’s much more to learn about network communication in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Python Sockets: Basic Usage
- Advanced Python Sockets: Server Creation and Multi-Connections
- Exploring Alternative Approaches: Twisted and asyncio
- Troubleshooting Python Sockets: Common Issues and Solutions
- Understanding Python Sockets: TCP/IP, UDP, Ports, and IP Addresses
- Python Sockets: Real-World Applications and Further Exploration
- Wrapping Up: Python Socket Mastery
Python Sockets: Basic Usage
Python sockets are a powerful tool for network communication. Let’s start with the basics: creating a socket, connecting to a server, and sending and receiving data.
Creating a Socket
The first step is to import the socket module and create a socket object. Here’s how you do it:
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
In this code, socket.AF_INET
specifies that we are using IPv4, and socket.SOCK_STREAM
indicates that we are using TCP. The socket()
function returns a socket object s
.
Connecting to a Server
Next, we need to connect to a server. We do this using the connect()
method of the socket object, as shown in this example:
s.connect(('localhost', 12345))
Here, ‘localhost’ is the server name, and 12345 is the port number. The connect()
method establishes a TCP client-server connection.
Sending and Receiving Data
Once the connection is established, we can send and receive data using the send()
and recv()
methods. Here’s an example:
s.send(b'Hello, Server!')
message = s.recv(1024)
print('Received from server: ', message)
# Output:
# Received from server: b'Hello, Client!'
In this code, s.send(b'Hello, Server!')
sends a message to the server. The b
before the string converts it to bytes, because the send()
method requires data in bytes. The recv(1024)
method receives data from the server. The argument 1024 is the maximum amount of data to be received at once.
Python sockets are relatively easy to use and offer a high degree of control over network communication. However, they can be tricky for beginners. Error handling is essential, especially for network issues such as connection errors and timeouts. Also, remember to always close the socket when you’re done using it to free up resources.
s.close()
This command closes the socket and releases the resources.
Advanced Python Sockets: Server Creation and Multi-Connections
As you become more comfortable with Python sockets, you can start exploring more complex uses. This includes creating a server, handling multiple connections, and working with non-blocking sockets.
Creating a Server
Let’s start by creating a basic server that can accept connections from clients. Here’s an example:
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind(('localhost', 12345))
s.listen(5)
print('Server is listening')
while True:
c, addr = s.accept()
print('Got connection from', addr)
c.send(b'Thank you for connecting')
c.close()
# Output:
# Server is listening
# Got connection from ('127.0.0.1', 52617)
# ...
In this code, the bind()
method binds the server to the specified host and port. The listen()
function enables the server to accept connections. The argument 5 is the maximum number of queued connections.
The accept()
method waits until a client connects to the server. It returns a new socket object c
and the address addr
of the client. The server then sends a message to the client and closes the connection.
Handling Multiple Connections
To handle multiple connections simultaneously, we can use threading. Here’s an example of a multithreaded server:
import socket
import threading
def handle_client(c):
message = c.recv(1024)
print('Received:', message)
c.send(b'Echo:' + message)
c.close()
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind(('localhost', 12345))
s.listen(5)
while True:
c, addr = s.accept()
print('Got connection from', addr)
threading.Thread(target=handle_client, args=(c,)).start()
# Output:
# Got connection from ('127.0.0.1', 52617)
# Received: b'Hello, Server!'
# ...
In this code, we create a new thread for each connection. The handle_client()
function receives a message from the client, prints it, sends an echo back to the client, and then closes the connection.
Non-Blocking Sockets
Python also supports non-blocking sockets. These sockets don’t wait for a send()
or recv()
operation to complete before continuing to execute the program. Here’s an example:
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.setblocking(0)
try:
s.connect(('localhost', 12345))
except BlockingIOError:
pass
# ...
In this code, the setblocking(0)
method makes the socket non-blocking. The connect()
method will now return immediately, and any subsequent send()
or recv()
calls will raise an BlockingIOError
if the operation would block.
Advanced usage of Python sockets can open up a wide range of possibilities for network communication. However, it also requires careful error handling and understanding of networking concepts.
Exploring Alternative Approaches: Twisted and asyncio
While Python sockets are a powerful tool for network communication, there are also third-party libraries that offer alternative approaches. Two such libraries are Twisted and asyncio.
Twisted: An Event-Driven Networking Engine
Twisted is an event-driven networking engine for Python. It supports a multitude of protocols, and includes a web server, an email server, and more. Here’s an example of a simple echo server using Twisted:
from twisted.internet import protocol, reactor
class Echo(protocol.Protocol):
def dataReceived(self, data):
self.transport.write(data)
class EchoFactory(protocol.Factory):
def buildProtocol(self, addr):
return Echo()
reactor.listenTCP(12345, EchoFactory())
reactor.run()
# Output:
# Echo server is running on port 12345
In this code, Echo
is a protocol that echoes any data it receives. EchoFactory
is a factory that produces Echo
instances. The reactor
handles the event loop, listens on TCP port 12345, and runs the server.
One advantage of Twisted is its extensive support for various protocols. However, its learning curve is steep, and its code can be difficult to understand for beginners.
asyncio: Asynchronous I/O, Event Loop, Coroutines and Tasks
asyncio is a library for writing single-threaded concurrent code using coroutines, multiplexing I/O access over sockets and other resources, running network clients and servers, and other related primitives. Here’s an example of a simple echo server using asyncio:
import asyncio
async def handle_echo(reader, writer):
data = await reader.read(100)
message = data.decode()
addr = writer.get_extra_info('peername')
print(f'Received {message} from {addr}')
print(f'Send: {message}')
writer.write(data)
await writer.drain()
writer.close()
async def main():
server = await asyncio.start_server(
handle_echo, '127.0.0.1', 12345)
addr = server.sockets[0].getsockname()
print(f'Serving on {addr}')
async with server:
await server.serve_forever()
asyncio.run(main())
# Output:
# Serving on ('127.0.0.1', 12345)
# Received Hello, Server! from ('127.0.0.1', 52617)
# Send: Hello, Server!
In this code, handle_echo
is a coroutine that reads from a client, echoes back the message, and then closes the connection. The main
coroutine starts the server and runs it forever.
One advantage of asyncio is its native support for asynchronous I/O, which can lead to more efficient use of resources. However, it requires careful handling of coroutines and tasks, and can be complex to use correctly.
Both Twisted and asyncio offer powerful alternatives to Python sockets for network communication. Your choice between them, and Python sockets, will depend on your specific needs and your comfort with each library’s style and requirements.
Troubleshooting Python Sockets: Common Issues and Solutions
Working with Python sockets can sometimes lead to unexpected issues. Let’s discuss some common problems you might encounter, along with their solutions and workarounds.
Handling Connection Errors
One common issue is handling connection errors. This can happen if the server is not running, or if the client and server are not properly configured to connect to each other. Here’s how you can handle such errors:
import socket
try:
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect(('localhost', 12345))
except socket.error as e:
print('Got an error:', e)
# Output:
# Got an error: [Errno 111] Connection refused
In this code, we use a try/except block to catch any socket errors that occur when trying to connect to the server. If a connection error occurs, the error message is printed to the console.
Dealing with Timeouts
Another common issue is dealing with timeouts. This can happen if the server takes too long to respond. Here’s how you can set a timeout for a socket:
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.settimeout(5)
try:
s.connect(('localhost', 12345))
except socket.timeout:
print('Connection timed out')
# Output:
# Connection timed out
In this code, the settimeout(5)
method sets a timeout of 5 seconds for the socket. If the connect()
method takes longer than 5 seconds, a socket.timeout
error is raised.
Resolving Data Encoding Issues
When sending and receiving data, you might encounter issues with data encoding. This can happen if you try to send a string over a socket, which expects data in bytes. Here’s how you can encode a string to bytes before sending it, and decode it back to a string after receiving it:
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect(('localhost', 12345))
message = 'Hello, Server!'
s.send(message.encode())
data = s.recv(1024)
print('Received:', data.decode())
# Output:
# Received: Hello, Client!
In this code, the encode()
method converts the string to bytes before sending it, and the decode()
method converts the received bytes back to a string.
By understanding these common issues and their solutions, you can avoid many pitfalls when working with Python sockets. Remember, error handling is a crucial part of network programming.
Understanding Python Sockets: TCP/IP, UDP, Ports, and IP Addresses
To fully grasp Python sockets, it’s essential to understand the underlying concepts, including TCP/IP, UDP, ports, and IP addresses.
Decoding TCP/IP
TCP/IP is the foundational communication protocol of the internet. It stands for Transmission Control Protocol/Internet Protocol. Python sockets use TCP/IP to establish reliable connections and exchange data between nodes.
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
In this example, socket.AF_INET
refers to the address family ipv4, and socket.SOCK_STREAM
means that it is a TCP socket.
TCP is a connection-oriented protocol, which means it ensures data is delivered successfully from sender to receiver. It provides error checking and error recovery, which makes it reliable for network communication.
Unveiling UDP
UDP, or User Datagram Protocol, is another protocol used for network communication. Unlike TCP, UDP is connectionless, meaning it doesn’t establish a connection before sending data.
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
In this example, socket.SOCK_DGRAM
means that it is a UDP socket. UDP is faster than TCP as it doesn’t provide the reliability checks that TCP does. It’s often used for live broadcasts and online games.
Ports and IP Addresses
An IP address is a unique address that identifies a device on a network. A port is a virtual point where network connections start and end. Ports act as communication endpoints for each specific process or service.
s.connect(('localhost', 12345))
In this example, ‘localhost’ is the host (an alias for the IP address 127.0.0.1, which refers to the current device), and 12345 is the port number.
Understanding these fundamental concepts will give you a solid foundation for working with Python sockets. It will also help you troubleshoot issues and better understand network communication.
Python Sockets: Real-World Applications and Further Exploration
Python sockets are more than just a programming concept; they have real-world applications that affect our daily life. They are used in various areas, from web servers to chat applications, and even IoT devices.
Python Sockets in Web Servers
Web servers use Python sockets to listen for incoming connections from clients (web browsers). When a client makes a request, the server processes it, sends a response back to the client, and then closes the connection.
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind(('localhost', 80))
s.listen(1)
while True:
client_connection, client_address = s.accept()
request = client_connection.recv(1024)
print(request.decode())
http_response = b"""\
HTTP/1.1 200 OK
Hello, World!\
"""
client_connection.sendall(http_response)
client_connection.close()
# Output:
# GET / HTTP/1.1
# Host: localhost
# ...
In this example, the server listens on port 80 (the standard port for HTTP), accepts incoming connections, prints the client’s request, sends a response, and then closes the connection.
Python Sockets in Chat Applications
Chat applications use Python sockets to facilitate real-time communication between users. The server listens for messages from clients and broadcasts them to all connected clients.
Exploring Related Concepts: Multithreading and Encryption
As you delve deeper into network programming with Python, you might want to explore related concepts such as multithreading and encryption.
Multithreading can be used to handle multiple connections simultaneously, which is essential for building scalable servers. Encryption, on the other hand, ensures secure communication by encrypting the data before sending it over the network.
Further Resources for Mastering Python Sockets
To continue your journey in mastering Python sockets, here are some additional resources that you might find helpful:
- Python Modules: A Comprehensive Overview – Explore the world of Python modules for code organization and reuse.
Simplifying File Decompression with Python Unzip – Discover techniques for extracting files from zip archives in Python.
Python heapq Module: Priority Queues and Heaps Explained – Reference guide for Python’s “heapq” module.
Python’s Official Documentation on Socket Programming dives into socket programming for networking applications.
Socket Programming in Python – An in-depth guide from Real Python on the basics and of socket programming.
Tutorial on Socket Programming in Python by GeeksforGeeks simplifies Python socket programming with examples.
Each of these resources provides a wealth of information on Python sockets, including more in-depth explanations, examples, and advanced topics. Happy learning!
Wrapping Up: Python Socket Mastery
In this comprehensive guide, we’ve explored the world of Python sockets
, a critical tool for network communication in Python.
We’ve journeyed from the basics to advanced usage, delving into creating sockets, connecting to servers, and sending and receiving data. We’ve also highlighted the importance of error handling, particularly for connection errors and timeouts.
We’ve ventured into more complex uses, such as creating servers, handling multiple connections, and working with non-blocking sockets. We’ve also discovered alternative approaches to network communication using third-party libraries like Twisted
and asyncio
.
Here’s a quick comparison of these approaches:
Approach | Advantages | Disadvantages |
---|---|---|
Python Sockets | High degree of control, Built-in module | Can be tricky for beginners |
Twisted | Supports many protocols, Includes servers | Steep learning curve |
asyncio | Efficient use of resources, Built-in module | Complex to use correctly |
We’ve also discussed the underlying fundamentals of Python sockets, including TCP/IP
, UDP
, ports
, and IP addresses
. Additionally, we’ve looked at the real-world applications of Python sockets, such as in web servers and chat applications, and suggested further resources for deepening your understanding.
In mastering Python sockets, you’re not only gaining a powerful tool for your Python projects, but also stepping into the broader world of network communication. It’s a journey that can open up countless possibilities for your coding future.