Python Unzip | ZipFile Module Usage Guide
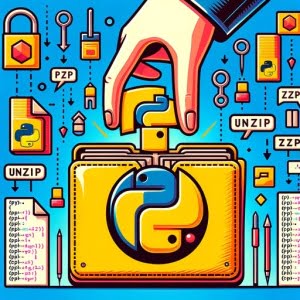
Ever found yourself stuck with a zipped file in Python? You’re not alone. Many developers find themselves in a similar situation, but Python, just like a skilled locksmith, can unlock the contents of zipped files with ease.
Python’s built-in libraries offer a robust set of tools to handle file compression and decompression, making it a go-to language for such tasks. From handling simple .zip files to dealing with more complex formats like .tar, .gz, and .rar, Python has got you covered.
In this guide, we’ll walk you through the process of unzipping files in Python, from the basics to more advanced techniques. We’ll cover everything from using the zipfile
module, handling different types of zipped files, to troubleshooting common issues and exploring alternative approaches.
So, let’s dive in and start mastering Python unzip!
TL;DR: How Do I Unzip Files in Python?
To unzip files in Python, you can use the built-in
zipfile
module’sextractall()
function. This module provides a simple and efficient way to handle zip files in Python.
Here’s a basic example:
import zipfile
with zipfile.ZipFile('file.zip', 'r') as zip_ref:
zip_ref.extractall()
# Output:
# This will extract all files from 'file.zip'
In this example, we import the zipfile
module and use the ZipFile
class to open the zip file in read mode (‘r’). We then call the extractall()
method to extract all files from the zip file.
This is a simple way to unzip files in Python, but there’s much more to learn about handling zip files and other compressed file formats in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Python Unzip: Basic Usage
Python’s zipfile
module is a versatile tool for working with zip files. It provides classes and methods that allow you to create, read, write, append, and list a ZIP file. For the purpose of this guide, we’ll focus on how to use it to unzip files.
Unzipping a File with zipfile
Here’s a simple example of how to use the zipfile
module to unzip a file:
import zipfile
with zipfile.ZipFile('file.zip', 'r') as zip_ref:
zip_ref.extractall()
# Output:
# This will extract all files from 'file.zip' to the current working directory
In this code block, we first import the zipfile
module. We then use the ZipFile
class from the zipfile
module to open the zip file in read mode (‘r’). The with
statement ensures that the file is properly closed after it is no longer needed. The extractall()
method is used to extract all files from the zip file to the current working directory.
Advantages and Potential Pitfalls
The main advantage of using the zipfile
module is that it is part of Python’s standard library. This means you don’t have to install any additional packages to use it. It’s also easy to use and provides a high level of control over zip file operations.
However, the zipfile
module has its limitations. For one, it can only handle zip files. If you need to work with other types of compressed files (like .tar or .gz), you’ll need to use other modules or third-party libraries. Additionally, while the zipfile
module can handle large files, it can be slow if you’re working with very large zip files.
Handling Different Zipped Files
Python’s built-in modules can handle a variety of compressed file formats. Beyond the standard .zip files, Python can also handle .tar, .gz, and .rar files. Let’s take a look at how to handle these different types of zipped files.
Unzipping .tar and .gz Files
For .tar and .gz files, Python provides the tarfile
module. Here’s an example of how to use it:
import tarfile
with tarfile.open('file.tar.gz', 'r:gz') as tar_ref:
tar_ref.extractall()
# Output:
# This will extract all files from 'file.tar.gz' to the current working directory
In this example, we use the tarfile
module’s open
method to open the .tar.gz file in read mode (‘r:gz’). The extractall()
method is then used to extract all files from the .tar.gz file.
Handling .rar Files
Handling .rar files is a bit more complex as Python’s standard library does not provide a way to extract .rar files. However, there are third-party libraries like rarfile
that can handle .rar files. Here’s how to use it:
import rarfile
with rarfile.RarFile('file.rar', 'r') as rar_ref:
rar_ref.extractall()
# Output:
# This will extract all files from 'file.rar' to the current working directory
In this example, we use the rarfile
module’s RarFile
class to open the .rar file in read mode (‘r’). The extractall()
method is then used to extract all files from the .rar file.
Handling Errors During Unzipping
When working with zip files in Python, you might encounter errors. One common error is the BadZipFile
error, which occurs when Python cannot open a file because it’s not a zip file or it is corrupted. Here’s how to handle this error:
import zipfile
try:
with zipfile.ZipFile('file.zip', 'r') as zip_ref:
zip_ref.extractall()
except zipfile.BadZipFile:
print('Not a zip file or a corrupted zip file')
# Output:
# 'Not a zip file or a corrupted zip file'
In this example, we use a try/except block to catch the BadZipFile
error. If Python encounters this error when trying to open or extract the zip file, it will print a message and continue with the rest of the program.
Exploring Alternative Methods for Unzipping Files
Python’s standard library offers a robust suite of modules for handling zip files, but there are also alternative approaches that can provide additional functionality or handle specific use cases.
Using the tarfile
and gzip
Modules
Python’s tarfile
and gzip
modules are great alternatives for handling .tar and .gz files, respectively. Here’s a quick example of how to use the gzip
module to unzip a .gz file:
import gzip
import shutil
with gzip.open('file.txt.gz', 'rb') as f_in:
with open('file.txt', 'wb') as f_out:
shutil.copyfileobj(f_in, f_out)
# Output:
# This will extract 'file.txt' from 'file.txt.gz'
In this example, we open the .gz file in read mode (‘rb’) with the gzip.open
method. We then open a new file in write mode (‘wb’) and use the shutil.copyfileobj
method to copy the contents of the .gz file to the new file.
Unzipping Files with pyunpack
pyunpack
is a third-party library that supports a wide variety of archive formats, including .zip, .tar, .rar, and .7z files. Here’s how to use it:
from pyunpack import Archive
Archive('file.zip').extractall('/path/to/destination')
# Output:
# This will extract all files from 'file.zip' to '/path/to/destination'
In this example, we use the Archive
class from pyunpack
to open the zip file, and then call the extractall
method to extract all files from the zip file to the specified destination.
While pyunpack
is a powerful tool, it’s worth noting that it depends on other tools like patool
, unrar
, and 7z
to handle different archive formats. This means you’ll need to install these tools on your system to use pyunpack
.
Troubleshooting Python Unzip Issues
As you work with Python to unzip files, you may encounter a few common issues. Here, we’ll discuss these potential pitfalls and provide tips on how to handle them effectively.
Dealing with ‘BadZipFile’ Errors
One of the most common issues when unzipping files in Python is the ‘BadZipFile’ error. This error typically occurs when Python cannot open a file because it’s not a zip file or it is corrupted.
You can handle this error using a try/except block. Here’s an example:
import zipfile
try:
with zipfile.ZipFile('file.zip', 'r') as zip_ref:
zip_ref.extractall()
except zipfile.BadZipFile:
print('Not a zip file or a corrupted zip file')
# Output:
# 'Not a zip file or a corrupted zip file'
In this code block, we attempt to open and extract a zip file. If Python encounters a ‘BadZipFile’ error, it will print a helpful message and continue with the rest of the program. This approach allows your program to continue running even if one zip file fails.
Handling Large Files
While Python’s zipfile
module can handle large files, it can be slow if you’re working with very large zip files. If speed is a concern, you may want to consider alternative approaches, such as using the tarfile
or gzip
modules for .tar and .gz files, respectively, or using third-party libraries like pyunpack
.
Understanding Python’s zipfile
Module
Python’s zipfile
module is a powerful tool for working with zip files. It provides a high level of control over zip file operations, including creating, reading, writing, appending, and listing a ZIP file.
How Does zipfile
Work?
The zipfile
module works by providing a ZipFile
class that you can use to open and manipulate zip files. Here’s a quick example:
import zipfile
with zipfile.ZipFile('file.zip', 'r') as zip_ref:
zip_ref.extractall()
# Output:
# This will extract all files from 'file.zip' to the current working directory
In this example, we use the ZipFile
class to open the zip file in read mode (‘r’). We then call the extractall()
method to extract all files from the zip file.
The Concepts Behind File Compression and Decompression
File compression is the process of reducing the size of a file or a group of files. It’s a common technique for saving storage space and reducing the time it takes to transfer files over a network.
Decompression, on the other hand, is the process of restoring compressed files back to their original state. When you unzip a file, you’re decompressing it.
Python’s zipfile
module uses the ZIP file format for compression and decompression. The ZIP file format is a common file format that uses lossless data compression. A ZIP file may contain one or more files or directories that may have been compressed.
The zipfile
module provides a simple interface to compress and decompress files in Python. It provides methods for reading, writing, and extracting files from a zip file, making it a versatile tool for handling zip files in Python.
Unzipping Files in Python: Beyond the Basics
Unzipping files in Python is a fundamental skill, but its relevance extends far beyond just extracting data from compressed files. It plays a critical role in various applications such as data analysis, web scraping, and more.
The Role of File Decompression in Data Analysis
In data analysis, you often need to work with large datasets that are usually compressed to save storage space and bandwidth. Knowing how to unzip files in Python enables you to access and analyze this data efficiently.
Unzipping Files in Web Scraping
Web scraping often involves downloading and extracting data from the web. Some of this data may be in the form of compressed files. With Python’s zipfile
module, you can easily extract this data for further processing.
Exploring Related Concepts
While unzipping files is a crucial skill, it’s also worth exploring related concepts. For instance, understanding file I/O (input/output) in Python can help you manipulate the data you’ve extracted from a zip file. Additionally, learning how to work with binary data can be useful, especially when dealing with non-text files like images or audio files.
Further Resources for Mastering File Decompression in Python
To deepen your understanding of working with zip files and other compressed file formats in Python, check out the following resources:
- IOFlood’s Python Modules Article – Discover best practices for documenting and structuring modules.
Python Module Integration Made Easy – Discover how to organize and reuse code effectively with “import.”
Networking Made Easy with Python Socket – Discover how to create client-server interactions with Python sockets.
Python’s Official Documentation on The Zipfile Module is essential for handling zip archives.
Python’s Official Documentation on The Tarfile Module – Explore the tarfile module, fundamental to manage tar archive files.
Reading and Writing Files in Python – Informative guide from Real Python about reading and writing files in Python, critical to file manipulation.
Unzipping Files in Python: A Recap
In this comprehensive guide, we’ve explored the process of unzipping files in Python, delving into the usage of the zipfile
module, dealing with common issues, and providing solutions. We’ve also looked into alternative approaches to handle file decompression in Python, giving you a well-rounded understanding of the topic.
We began with the basics, learning how to use the zipfile
module to unzip .zip files. We then ventured into more advanced territory, discussing how to handle different types of zipped files such as .tar, .gz, and .rar.
Along the way, we tackled common challenges you might encounter when unzipping files in Python, such as ‘BadZipFile’ errors, and provided solutions for each issue.
We also explored alternative approaches to unzipping files in Python, discussing the use of other built-in modules like tarfile
and gzip
, as well as third-party libraries like pyunpack
.
Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
zipfile | Part of Python’s standard library, easy to use | Only handles .zip files |
tarfile & gzip | Handle .tar and .gz files, respectively | Each only handles one file format |
pyunpack | Supports a wide variety of archive formats | Depends on other tools like patool , unrar , and 7z |
Whether you’re just starting out with unzipping files in Python or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of the process and its related concepts.
With the ability to handle a wide variety of compressed file formats and deal with common issues, Python proves to be a powerful tool for file decompression. Happy unzipping!