Python strip() Function Guide (With Examples)
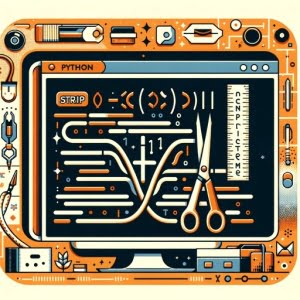
Struggling with unwanted white spaces in your Python strings? Just like a skilled barber, Python’s strip()
method can trim off the excess, leaving you with a clean, well-groomed string.
Whether you are a beginner or an expert, this guide will walk you through the ins and outs of the strip()
method. We’ll cover everything from the fundamentals of Python strings, to how the strip()
method works, and even delve into some alternative approaches.
So, let’s dive in and start mastering Python’s strip()
method together!
TL;DR: How do I use the strip() method in Python?
The
strip()
method in Python is used to remove leading and trailing spaces from a string. Here’s a simple example:
text = ' Hello, World! '
trimmed_text = text.strip()
print(trimmed_text)
# Output:
# 'Hello, World!'
In this example, we have a string ‘ Hello, World! ‘ with leading and trailing spaces. We use the strip()
method to remove these spaces, and the result is a clean string ‘Hello, World!’.
Keep reading for more detailed explanations, advanced usage scenarios, and to explore the power of Python’s
strip()
method.
Table of Contents
- Trimming Strings with Python’s strip() Method
- Advanced Trimming with Python’s strip() Method
- Exploring Alternatives: lstrip() and rstrip() Methods
- Troubleshooting Python’s strip() Method
- Understanding Python Strings and Whitespace
- Real-World Applications of Python’s strip() Method
- Recap: Mastering Python’s strip() Method
Trimming Strings with Python’s strip() Method
The strip()
method in Python is straightforward to use. It’s a built-in function that operates on string data types. Its primary purpose is to remove leading (spaces at the start) and trailing (spaces at the end) whitespace from a string.
Here’s a simple example:
text = ' Python strip '
trimmed_text = text.strip()
print(trimmed_text)
# Output:
# 'Python strip'
In the code above, we started with a string ‘ Python strip ‘ that had leading and trailing spaces. After calling the strip()
method on it, we got a clean string ‘Python strip’.
The strip()
method can be incredibly useful when dealing with user input or data cleaning where extra spaces can cause issues. For instance, when comparing two strings, ‘Python’ and ‘ Python ‘, Python would consider these as different due to the extra space, but with strip()
, we can ensure accurate comparisons.
However, it’s essential to remember that strip()
only removes leading and trailing spaces, not spaces in between words. For example:
text = ' Python strip '
trimmed_text = text.strip()
print(trimmed_text)
# Output:
# 'Python strip'
In this case, the strip()
method will not remove the extra spaces between ‘Python’ and ‘strip’.
Advanced Trimming with Python’s strip() Method
The strip()
method in Python can do more than just removing leading and trailing spaces. It can also be used to remove specific characters from the start or end of a string. This is done by passing the character or characters you want to remove as an argument to the strip()
method.
Here’s an example:
text = '###Python strip###'
trimmed_text = text.strip('#')
print(trimmed_text)
# Output:
# 'Python strip'
In this example, we have a string ‘###Python strip###’ with leading and trailing hash symbols. When we pass ‘#’ as an argument to the strip()
method, it removes these characters, leaving us with a clean string ‘Python strip’.
This advanced use of strip()
comes in handy when dealing with strings that may have unwanted characters. It’s a common scenario in data cleaning tasks where you might be dealing with strings wrapped in quotes, brackets, or other characters.
It’s important to note that strip()
will remove the characters in the order they appear in the argument. For instance, if you pass ‘ab’ as an argument, it will first remove ‘a’ and then ‘b’ from the start and end of the string:
text = 'abPython stripba'
trimmed_text = text.strip('ab')
print(trimmed_text)
# Output:
# 'Python strip'
In the above code, the strip('ab')
method removes both ‘a’ and ‘b’ from the start and end, resulting in ‘Python strip’. However, it will not remove the ‘b’ before ‘a’ or ‘a’ before ‘b’ if they appear in that order.
Exploring Alternatives: lstrip() and rstrip() Methods
Python provides more precise control over string trimming with the lstrip()
and rstrip()
methods. These methods work similarly to strip()
, but lstrip()
only removes characters from the left (start) of the string, and rstrip()
only from the right (end).
Here’s an example of using lstrip()
and rstrip()
:
text = ' Python strip '
left_trimmed_text = text.lstrip()
right_trimmed_text = text.rstrip()
print(left_trimmed_text)
print(right_trimmed_text)
# Output:
# 'Python strip '
# ' Python strip'
In the above code, lstrip()
removes spaces only from the start of the string, while rstrip()
removes spaces only from the end. This can be useful when you want to maintain the spaces at one end of the string.
Similarly to strip()
, lstrip()
and rstrip()
can also remove specific characters:
text = '###Python strip###'
left_trimmed_text = text.lstrip('#')
right_trimmed_text = text.rstrip('#')
print(left_trimmed_text)
print(right_trimmed_text)
# Output:
# 'Python strip###'
# '###Python strip'
In this case, lstrip('#')
removes hash symbols from the start, and rstrip('#')
removes them from the end.
These alternative methods give you more control over string trimming in Python. However, they are more specific in their use, and it’s important to choose the right method based on the needs of your task.
Troubleshooting Python’s strip() Method
While Python’s strip()
method is generally straightforward, there are some common pitfalls that can lead to unexpected results. Understanding these issues can help you use the strip()
method more effectively.
The strip() Method Doesn’t Affect Middle Spaces
One common misunderstanding is thinking that strip()
removes all spaces in a string. However, strip()
only removes leading and trailing spaces, not spaces in between words:
text = ' Python strip '
trimmed_text = text.strip()
print(trimmed_text)
# Output:
# 'Python strip'
As you can see, the strip()
method leaves the spaces between ‘Python’ and ‘strip’. If you need to remove all spaces in a string, consider using the replace()
method instead:
text = ' Python strip '
trimmed_text = text.replace(' ', '')
print(trimmed_text)
# Output:
# 'Pythonstrip'
The strip() Method Removes Characters Sequentially
When you pass multiple characters to the strip()
method, it removes them in the order they appear in the argument. This can lead to unexpected results if you’re not aware of it:
text = 'abPython stripba'
trimmed_text = text.strip('ab')
print(trimmed_text)
# Output:
# 'Python strip'
In this case, strip('ab')
removes both ‘a’ and ‘b’ from the start and end, but it will not remove the ‘b’ before ‘a’ or ‘a’ before ‘b’ if they appear in that order.
Understanding these considerations can help you avoid common issues and use Python’s strip()
method more effectively.
Understanding Python Strings and Whitespace
Python strings are sequences of characters enclosed in quotes. They can contain any characters: letters, numbers, symbols, and even spaces. These spaces, also known as whitespace, are just as much a part of the string as any other character.
text = ' Python strip '
print(text)
# Output:
# ' Python strip '
In the above example, the spaces at the start, end, and between ‘Python’ and ‘strip’ are all part of the string. They can affect how the string is processed and displayed, which can lead to unexpected results.
For instance, consider a program that compares two strings:
text1 = 'Python'
text2 = ' Python '
print(text1 == text2)
# Output:
# False
Even though ‘Python’ and ‘ Python ‘ look similar to us, Python considers them different because of the extra spaces in ‘ Python ‘. This can be problematic when comparing user input or processing data where extra spaces might be present.
This is where string manipulation methods like strip()
come in. They allow us to control and modify the whitespace in our strings, ensuring that it doesn’t interfere with our program’s operation. Understanding how to manipulate strings is a critical skill in Python, whether you’re working with user input, data analysis, or any task that involves processing text.
Real-World Applications of Python’s strip() Method
Python’s strip()
method is not just a theoretical concept, but a practical tool used in various real-world applications. One of the most common uses of strip()
is in data cleaning. Raw data often comes with extra spaces that can interfere with data processing, and strip()
helps in removing these unwanted characters.
Consider the following example where we have a list of names with extra spaces, and we want to clean it:
names = [' Alice ', ' Bob ', ' Charlie ']
cleaned_names = [name.strip() for name in names]
print(cleaned_names)
# Output:
# ['Alice', 'Bob', 'Charlie']
In this example, we used strip()
in a list comprehension to clean each name in the list. The cleaned list doesn’t contain any extra spaces.
Another common use of strip()
is in file handling. When reading lines from a file, we often get extra newline characters at the end of each line. strip()
can help remove these:
with open('file.txt', 'r') as file:
lines = file.readlines()
cleaned_lines = [line.strip() for line in lines]
In this code, strip()
is used to remove the newline characters from each line in the file, leaving us with clean lines of text.
Python’s strip()
method is a versatile tool that can be applied in many different scenarios. If you’re interested in further exploring Python’s string manipulation methods, consider looking into split()
, join()
, and replace()
. These methods offer more ways to control and manipulate strings in Python, and understanding them can significantly enhance your Python skills.
Further Resources for Python Strings
If you’re interested in learning more ways to handle strings in Python, here are a few resources that you might find helpful:
- Crafting Efficient Python Code with String Techniques: Craft more efficient Python code by utilizing advanced string techniques, optimizing your approach to handling and processing text.
Python String to Int Conversion Guide with Examples: This guide provides examples and explanations on how to convert a string to an integer in Python, including different scenarios and error handling.
Python Substring Quick Reference: This quick reference guide provides an overview of slicing and substring techniques in Python, along with examples and explanations for extracting substrings from strings using different methods.
Python strip() Method: A Comprehensive Guide: A comprehensive guide on w3schools.com that explains the strip() method in Python, used to remove leading and trailing characters from a string.
Python Standard Library Documentation: The official Python documentation for the strip() method, providing detailed information on its usage in the standard library.
Python String strip() Method: Simplilearn Tutorial: A tutorial on Simplilearn that presents the strip() method in Python and demonstrates how to use it to remove leading and trailing characters from a string.
Recap: Mastering Python’s strip() Method
Python’s strip()
method is a powerful tool for string manipulation. Its primary function is to remove leading and trailing spaces from a string, ensuring clean and well-formatted text. This is particularly useful in data cleaning and user input validation, where extra spaces can lead to unexpected results.
We also explored advanced usage of strip()
, where it can remove specific characters from the start or end of a string. This adds another layer of flexibility to the method, allowing it to handle a wider range of string cleaning tasks.
Python also provides lstrip()
and rstrip()
methods as alternatives to strip()
. These methods only remove characters from the left or right of the string, giving you more precise control over string trimming.
However, it’s important to remember that strip()
and its variants have their quirks. They don’t affect spaces in between words and remove characters in the order they appear in the argument. Understanding these considerations can help you avoid common issues and use these methods more effectively.
In conclusion, Python’s strip()
method and its variants are versatile tools for string manipulation. They can handle a wide range of tasks, from basic string cleaning to more complex text processing requirements. By mastering these methods, you can significantly enhance your Python skills and write more efficient, cleaner code.