Python Import: Using The Module Import Statement
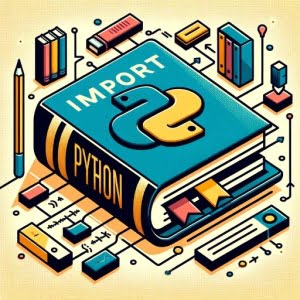
Ever felt like you’re playing a game of fetch when importing modules and packages in Python? You’re not alone. Many developers find themselves in a similar situation.
Think of Python’s import statement as a diligent retriever – always ready to fetch and incorporate external modules into your code.
In this guide, we’ll walk you through the process of using Python’s import statement, from the basics to more advanced techniques. We’ll cover everything from the simple import
statement, advanced techniques like from...import
, import...as
, and even delve into dynamic imports using importlib
and __import__()
function.
Let’s get started!
TL;DR: How Do I Use the Import Statement in Python?
To import a module in Python, you use the
import
statement. This command allows you to access the functions and classes defined in that module.
Here’s a simple example:
import math
print(math.pi)
# Output:
# 3.141592653589793
In this example, we’ve imported the math
module using the import
statement. We then print the value of pi
which is a constant defined in the math
module. The output is the numerical value of pi up to 15 decimal places.
But there’s so much more to Python’s import system than just the basic
import
statement. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
Understanding Python’s Import Statement
Python’s import
statement is a built-in function that allows you to include external modules in your program. It’s an essential tool for every Python developer, as it provides access to a wealth of functions, classes, and methods that can simplify your code and increase its functionality.
The Syntax of Import Statement
The syntax of the import
statement is straightforward. All you need to do is type import
followed by the name of the module you want to import.
Here’s a basic example:
import random
# Let's use a function from the 'random' module
random_number = random.randint(1, 10)
print(random_number)
# Output:
# (A random number between 1 and 10)
In this code block, we first import the random
module. Then, we call the randint
function from the random
module to generate a random number between 1 and 10. The output will be a random number within the specified range.
Advantages and Potential Pitfalls
The main advantage of the import
statement is that it allows you to use pre-existing code from Python’s vast library of modules, saving you from having to write everything from scratch.
However, there are potential pitfalls to be aware of. One of the most common issues is the risk of namespace conflicts. This happens when a function or class in the imported module has the same name as a function or class in your own code. To avoid this, Python provides several ways to handle imports, which we will cover in the ‘Advanced Use’ section.
In conclusion, Python’s import
statement is a powerful tool that, when used correctly, can greatly enhance your coding efficiency and program functionality.
Advanced Python Import Techniques
As you grow more comfortable with Python’s import
statement, you’ll find that there are several advanced techniques that can provide you with more control and flexibility.
Importing Specific Functions or Classes
Instead of importing an entire module, you can choose to import only specific functions or classes. This can help prevent namespace conflicts and reduce memory usage. Here’s how you can do it:
from math import pi
print(pi)
# Output:
# 3.141592653589793
In this example, we’re importing only the pi
constant from the math
module. As a result, we can use pi
directly in our code without having to precede it with math.
.
Using from
with import
The from...import
statement is a more precise tool that allows you to import only what you need from a module. This can help keep your code clean and efficient. Here’s an example:
from datetime import datetime
print(datetime.now())
# Output:
# (Current date and time)
In the code above, we’re importing only the datetime
class from the datetime
module. We then use it to print the current date and time.
Aliasing with as
Aliasing is a technique that allows you to give a different name to the module you’re importing. This can be particularly useful when dealing with modules that have long or complex names. Here’s how to do it:
import datetime as dt
print(dt.datetime.now())
# Output:
# (Current date and time)
In this example, we’re importing the datetime
module but giving it the alias dt
. This means we can refer to it as dt
in our code, which can make our code easier to read and write.
These advanced techniques can give you more control over your code and help you write more efficient and effective programs. But remember, with great power comes great responsibility. Use these techniques wisely to avoid confusion and ensure your code remains clean and readable.
Exploring Dynamic Imports in Python
As you delve deeper into Python’s import system, you’ll encounter more advanced and flexible methods of importing modules. Two such methods are dynamic imports using importlib
and the __import__()
function.
Dynamic Imports Using importlib
Python’s importlib
module is a powerful tool that allows you to import modules dynamically. This means you can import modules based on variables, which can be particularly useful in large projects where the modules to import might not be known until runtime.
Here’s an example of how to use importlib
:
import importlib
# Let's say we have a variable that contains the name of the module we want to import
module_name = 'math'
# We can use importlib to import the module dynamically
module = importlib.import_module(module_name)
print(module.pi)
# Output:
# 3.141592653589793
In this example, we’re using importlib
to import the math
module dynamically. The module name is stored in a variable, and we use importlib.import_module()
to import it. We can then use the imported module just like we would with a regular import.
The __import__()
Function
The __import__()
function is another method for performing dynamic imports. It’s a built-in function in Python that’s invoked by the import
statement. However, it can also be called directly for more advanced import scenarios.
Here’s an example of how to use __import__()
:
# Again, we have a variable that contains the name of the module we want to import
module_name = 'math'
# We can use __import__() to import the module dynamically
module = __import__(module_name)
print(module.pi)
# Output:
# 3.141592653589793
In this code block, we’re using __import__()
to dynamically import the math
module. The usage is similar to importlib.import_module()
, but __import__()
is a lower-level function and it’s generally recommended to use importlib
for most use cases.
Both importlib
and __import__()
provide a high level of flexibility and control over your imports, but they also come with their own set of challenges. They can make your code more complex and harder to debug, so they should be used judiciously and only when necessary.
Troubleshooting Python Import Issues
While Python’s import system is powerful and flexible, it’s not without its challenges. In this section, we’ll explore some common errors and obstacles you might encounter when using the import
statement, along with their solutions.
The Dreaded ‘ModuleNotFoundError’
One of the most common issues you might face is the ModuleNotFoundError
. This error occurs when Python can’t locate the module you’re trying to import.
Here’s an example of what this might look like:
import non_existent_module
# Output:
# ModuleNotFoundError: No module named 'non_existent_module'
In this code block, we’re trying to import a module that doesn’t exist, which results in a ModuleNotFoundError
.
The solution to this error is usually straightforward: make sure the module you’re trying to import exists and is in the correct location. Check your spelling and capitalization, as Python is case-sensitive.
Navigating Circular Imports
Another common issue is circular imports. This occurs when two or more modules depend on each other, either directly or indirectly. This can lead to infinite loops and other unexpected behavior.
The solution to circular imports is often to refactor your code to remove the circular dependency. This might involve moving some code to a separate module or changing the order of your imports.
Best Practices and Optimization
When using the import
statement, it’s important to follow best practices to keep your code clean and efficient. Here are a few tips:
- Only import what you need. This can help reduce memory usage and prevent namespace conflicts.
- Use the
as
keyword to give your imports meaningful aliases. This can make your code easier to read and understand. - Avoid circular imports by carefully organizing your code and dependencies.
By understanding these common issues and how to solve them, you can use Python’s import system more effectively and write more robust and reliable code.
Python’s Module System and PYTHONPATH
Python’s module system is a core feature of the language. It’s what allows you to use the import
statement to include external code in your programs. But how exactly does Python locate and load these modules?
Understanding PYTHONPATH
When you import a module, Python searches for it in a list of directories defined by the PYTHONPATH environment variable. PYTHONPATH is like a map that tells Python where to look for modules.
You can view your current PYTHONPATH by running the following command in your Python interpreter:
import sys
print(sys.path)
# Output:
# ['(directory where your Python interpreter was invoked)', '(other directories in your PYTHONPATH)', '...']
In this code block, we’re importing the sys
module, which provides access to some variables used or maintained by the Python interpreter. We then print sys.path
, which is a list of directory names, with each string representing one directory.
Built-in, Standard Library, and Third-Party Modules
There are three main types of modules in Python: built-in modules, standard library modules, and third-party modules.
Built-in modules are written in C and integrated with the Python interpreter. Examples include sys
, math
, and itertools
. These modules are always available, and you don’t need to install anything to use them.
Standard library modules are a part of Python’s standard distribution. They’re written in Python and provide a wide range of functionality, from file I/O and system calls to internet protocols and multimedia services. You can view a list of standard library modules by checking Python’s official documentation.
Third-party modules are modules developed by the Python community. They’re not included with Python’s standard distribution and need to be installed separately, typically using a package manager like pip. Examples include numpy
, requests
, and django
.
Understanding Python’s module system and PYTHONPATH is crucial for effective use of the import
statement. With this knowledge, you can better organize your code and avoid common import issues.
Expanding the Scope: Import in Larger Projects
As your Python projects grow in size and complexity, the way you handle imports can significantly impact your code’s readability and performance. In larger projects, it’s essential to organize your imports effectively to maintain clean, efficient code.
Organizing Imports in Python
In Python, it’s a common practice to place all import statements at the top of the file. This makes it easy to see all the dependencies at a glance. Additionally, it’s recommended to separate standard library imports, third-party imports, and local application/library specific imports with a blank line.
Here’s an example:
# Standard library imports
import math
import sys
# Third-party imports
import numpy as np
# Local application/library specific imports
import my_module
In this code block, the imports are organized into three separate groups, making it easy to identify the source of each module.
Creating Your Own Modules and Packages
Python’s import
statement isn’t just for using pre-existing modules. You can also create your own modules and packages, allowing you to reuse code across multiple projects. A module is simply a .py
file containing Python code, while a package is a directory of Python modules.
Here’s an example of how to create and use your own module:
# my_module.py
def hello_world():
return 'Hello, world!'
# main.py
import my_module
print(my_module.hello_world())
# Output:
# 'Hello, world!'
In this example, we’ve created a module called my_module
with a single function hello_world()
. We then import and use this module in main.py
.
Further Resources for Python Import Mastery
To delve deeper into Python’s import system, check out these resources:
- IOFlood’s Python Modules Guide – Discover how modules enhance code readability and maintainability.
Asynchronous Python Programming – Discover how to leverage ‘async’ and ‘await’ for concurrent operations in Python.
Unzipping Files in Python: A Quick Tutorial – Explore Python’s “zipfile” module and learn to extract compressed files.
Python’s official documentation on modules – An in-depth guide including how to create your own modules and packages.
Python Module of the Week – A series of articles covering the modules in Python’s standard library in detail.
Real Python’s guide to importing in Python includes how to handle errors and organize your imports effectively.
These resources provide a wealth of information for mastering Python’s import system and using it to write clean, efficient code.
Wrapping Up: Mastering Python’s Import System
In this comprehensive guide, we’ve journeyed through the intricacies of Python’s import system, from the basic import
statement to more advanced techniques and alternative approaches.
We began with the basics, understanding the import
statement’s syntax and usage. We then ventured into advanced territory, exploring techniques like from...import
, import...as
, and dynamic imports using importlib
and __import__()
function.
We tackled common challenges you might encounter when using the import
statement, such as ‘ModuleNotFoundError’ and circular imports, providing you with solutions and best practices for each issue.
We also delved into the background of Python’s module system, understanding the PYTHONPATH and the difference between built-in, standard library, and third-party modules. Finally, we explored how to organize imports in larger projects and the creation of your own modules and packages.
Here’s a quick recap of the methods we’ve discussed:
Method | Description | Use Case |
---|---|---|
Basic Import | Import the entire module | When you need all functions/classes from a module |
from...import | Import specific functions or classes | When you only need specific functions/classes |
import...as | Alias your imports | When the module names are long or complex |
importlib and __import__() | Dynamic imports | When you need to import modules during runtime |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of Python’s import system and its capabilities.
With its balance of simplicity and power, Python’s import system is a crucial tool for any Python programmer. Happy coding!