Python Async: Master Asynchronous Programming
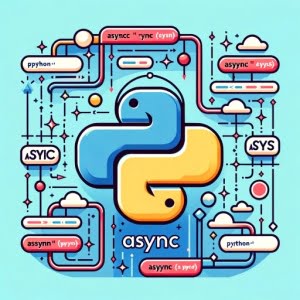
Are you finding it difficult to understand Python’s async feature? You’re not alone. Many developers find themselves puzzled when it comes to handling async in Python, but we’re here to help.
Think of Python’s async feature as a well-oiled machine – allowing us to write efficient, non-blocking code, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of working with async in Python, from async() to await(), as well as asynchronous I/O. We’ll cover everything from the basics of asynchronous programming to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Use Python’s Async Feature?
Python’s async feature is used to write asynchronous code, enabling you to write non-blocking code. Running a function asynchronously is as simple as
asyncio.run(function())
. Here’s a simple example:
async def main():
print('Hello, World!')
import asyncio
asyncio.run(main())
# Output:
# 'Hello, World!'
In this example, we define an asynchronous function main()
using the async def
syntax. Inside this function, we print ‘Hello, World!’. We then import the asyncio
module and use asyncio.run(main())
to execute our asynchronous function.
This is just a basic usage of Python’s async feature. In the rest of this guide, we’ll dive deeper into how async works and how to use it effectively in different scenarios.
Table of Contents
- Getting Started with Python Async
- Python Async in Depth: I/O-bound Tasks and Task Management
- Exploring Alternatives to Async in Python
- Troubleshooting Python’s Async: Common Issues and Solutions
- Understanding Python Async: Event Loop and Coroutines
- Python Async Beyond the Basics: Real-World Applications
- Wrapping Up: Mastering Python Async for Asynchronous Programming
Getting Started with Python Async
Understanding Python’s async feature starts with getting a grasp of two essential keywords: async
and await
. These keywords are the building blocks of asynchronous programming in Python.
Async and Await: The Basics
The async
keyword in Python is used to declare a function as a “coroutine.” A coroutine is a special kind of function that can be paused and resumed, allowing Python to handle other tasks in the meantime.
The await
keyword is used inside an async function to call another async function and wait for it to finish. The function being called with await
is also a coroutine.
Let’s see this in action with a simple example:
import asyncio
async def say_hello():
await asyncio.sleep(1)
print('Hello, World!')
asyncio.run(say_hello())
# Output:
# (After a one-second pause) 'Hello, World!'
In this code, we define a coroutine say_hello()
using the async def
syntax. Inside this function, we use the await
keyword to call asyncio.sleep(1)
, which is a coroutine that pauses execution for one second. After the pause, we print ‘Hello, World!’. Finally, we use asyncio.run(say_hello())
to execute our coroutine.
This is the basic usage of Python’s async feature. The power of async becomes more evident when we start working with multiple coroutines and I/O-bound tasks, which we’ll dive into in the next sections.
Python Async in Depth: I/O-bound Tasks and Task Management
As we dive deeper into Python’s async feature, we’ll see its true power in dealing with I/O-bound tasks and managing multiple async tasks simultaneously.
Async for I/O-bound Tasks
Async is particularly useful when dealing with I/O-bound tasks – operations that spend most of their time waiting for input/output operations to complete, such as reading from or writing to a file, making network requests, or querying a database.
When an I/O-bound task is executed in a traditional synchronous manner, the entire program waits for the task to complete before moving onto the next task. With async, however, the program can move on to other tasks while waiting for the I/O-bound task to complete.
Let’s see this in action:
import asyncio
import time
async def count():
print('Counting...')
await asyncio.sleep(1)
print('Done!')
start = time.time()
asyncio.run(count())
asyncio.run(count())
end = time.time()
print(f'Total time: {end - start}')
# Output:
# 'Counting...'
# (After a one-second pause) 'Done!'
# 'Counting...'
# (After a one-second pause) 'Done!'
# 'Total time: 2.0'
In this example, we define a coroutine count()
that prints ‘Counting…’, waits for one second, then prints ‘Done!’. We then run this coroutine twice. Since the coroutines are run one after the other, the total time taken is approximately 2 seconds.
Managing Multiple Async Tasks
Async in Python also allows us to manage multiple tasks at once. This is achieved using the asyncio.gather()
function, which runs multiple coroutines concurrently and waits for all of them to finish.
Let’s modify our previous example to run the coroutines concurrently:
start = time.time()
asyncio.run(asyncio.gather(count(), count()))
end = time.time()
print(f'Total time: {end - start}')
# Output:
# 'Counting...'
# 'Counting...'
# (After a one-second pause) 'Done!'
# 'Done!'
# 'Total time: 1.0'
In this modified example, we use asyncio.gather(count(), count())
to run the count()
coroutines concurrently. As a result, the total time taken is approximately 1 second, even though we’re running the same number of coroutines as before. This is the power of async in Python!
Exploring Alternatives to Async in Python
While Python’s async feature is powerful and versatile, it’s not the only tool in Python’s arsenal for handling concurrent tasks. There are alternative approaches to asynchronous programming in Python, such as threading and multiprocessing.
Python Threading for Concurrent Tasks
Threading in Python can be used to run multiple tasks concurrently. However, due to Python’s Global Interpreter Lock (GIL), threads in Python are not truly concurrent and may not provide a significant speedup for CPU-bound tasks.
Here’s an example of using threading in Python:
import threading
import time
def count():
print('Counting...')
time.sleep(1)
print('Done!')
start = time.time()
thread1 = threading.Thread(target=count)
thread2 = threading.Thread(target=count)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
end = time.time()
print(f'Total time: {end - start}')
# Output:
# 'Counting...'
# 'Counting...'
# (After a one-second pause) 'Done!'
# 'Done!'
# 'Total time: 1.0'
In this example, we use Python’s threading
module to create two threads that run the count()
function concurrently. The total time taken is approximately 1 second, similar to using asyncio.gather()
.
Python Multiprocessing for Parallel Tasks
Multiprocessing in Python allows for the creation of separate processes, each with its own Python interpreter and memory space, thus bypassing the GIL and allowing for true parallelism.
Here’s an example of using multiprocessing in Python:
import multiprocessing
import time
def count():
print('Counting...')
time.sleep(1)
print('Done!')
start = time.time()
process1 = multiprocessing.Process(target=count)
process2 = multiprocessing.Process(target=count)
process1.start()
process2.start()
process1.join()
process2.join()
end = time.time()
print(f'Total time: {end - start}')
# Output:
# 'Counting...'
# 'Counting...'
# (After a one-second pause) 'Done!'
# 'Done!'
# 'Total time: 1.0'
In this example, we use Python’s multiprocessing
module to create two processes that run the count()
function in parallel. The total time taken is approximately 1 second, similar to using asyncio.gather()
and threading.
While threading and multiprocessing provide alternatives to async, they come with their own trade-offs. Threading does not provide true concurrency due to the GIL, and multiprocessing involves more overhead due to the creation of separate processes. Therefore, the choice between async, threading, and multiprocessing will depend on the specific requirements of your task.
Troubleshooting Python’s Async: Common Issues and Solutions
As with any programming concept, using Python’s async feature can sometimes lead to unforeseen issues. Let’s discuss some common problems you may encounter while working with async in Python, and how to troubleshoot them.
Handling Exceptions in Async Code
Exceptions in async code can be handled just like in synchronous code, using try
/except
blocks. However, if an exception is not caught within the async function, it will be propagated to the event loop, which will terminate the program.
Here’s an example of handling exceptions in async code:
import asyncio
async def raise_exception():
raise Exception('An exception occurred!')
async def main():
try:
await raise_exception()
except Exception as e:
print(f'Caught exception: {e}')
asyncio.run(main())
# Output:
# 'Caught exception: An exception occurred!'
In this example, the raise_exception()
coroutine raises an exception. In the main()
coroutine, we use a try
/except
block to catch and handle the exception.
Debugging Async Code
Debugging async code can be a bit tricky, as errors might not manifest until the event loop is running. Python’s built-in pdb
debugger might not be very helpful in this case. However, you can use the asyncio
module’s debugging mode to get more information about where things are going wrong.
You can enable debugging mode by setting the PYTHONASYNCIODEBUG
environment variable, or by calling asyncio.get_event_loop().set_debug(True)
.
These are just a few of the issues you might encounter while working with Python’s async feature. Always remember to handle exceptions properly and use debugging tools effectively to ensure your async code runs smoothly.
Understanding Python Async: Event Loop and Coroutines
To truly master Python’s async feature, it’s crucial to understand the concepts that underlie it. Two key concepts are the event loop and coroutines.
The Event Loop: Python’s Task Scheduler
At the heart of every asyncio program is the event loop. Think of it as the control center of asyncio – it’s responsible for executing coroutines and scheduling callbacks.
Here’s a simple example of an event loop in action:
import asyncio
async def main():
print('Hello, World!')
loop = asyncio.get_event_loop()
try:
loop.run_until_complete(main())
finally:
loop.close()
# Output:
# 'Hello, World!'
In this example, we create an event loop using asyncio.get_event_loop()
, then use loop.run_until_complete(main())
to execute the main()
coroutine. The event loop continues running until main()
is completed, after which it’s closed with loop.close()
.
Coroutines: The Building Blocks of Async
Coroutines are the building blocks of async in Python. A coroutine is a special kind of function that can be paused and resumed, allowing Python to handle other tasks in the meantime.
Coroutines are defined using the async def
syntax, and can be paused with the await
keyword. Here’s an example:
import asyncio
async def main():
await asyncio.sleep(1)
print('Hello, World!')
asyncio.run(main())
# Output:
# (After a one-second pause) 'Hello, World!'
In this example, we define a coroutine main()
that pauses for one second with await asyncio.sleep(1)
, then prints ‘Hello, World!’.
Understanding the event loop and coroutines is key to mastering Python’s async feature. With these concepts in mind, you’ll be able to write efficient, non-blocking code that can handle multiple tasks at once.
Python Async Beyond the Basics: Real-World Applications
Python’s async feature is not just a theoretical concept – it has practical applications in the real world, particularly in areas such as web scraping and web development.
Web Scraping with Python Async
Web scraping involves fetching data from websites, which often involves making multiple requests to a server. Python’s async feature can significantly speed up this process by handling multiple requests concurrently.
Here’s a simple example of an async web scraper:
import aiohttp
import asyncio
async def fetch(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
urls = ['http://example.com'] * 10 # a list of URLs to fetch
async with aiohttp.ClientSession() as session:
tasks = [fetch(session, url) for url in urls]
await asyncio.gather(*tasks)
asyncio.run(main())
In this example, we use the aiohttp
library to make HTTP requests. The fetch()
coroutine fetches the content of a URL, and the main()
coroutine fetches multiple URLs concurrently using asyncio.gather()
.
Web Development with Python Async
Python’s async feature can also be used in web development to handle multiple client requests concurrently. This can greatly improve the performance of your web server.
Here’s a simple example of an async web server using the aiohttp
library:
from aiohttp import web
async def handle(request):
return web.Response(text='Hello, World!')
app = web.Application()
app.router.add_get('/', handle)
web.run_app(app)
In this example, we define an async handler function that responds to HTTP GET requests with ‘Hello, World!’. We then create a web application, add our handler to the application’s router, and run the application.
Further Resources for Mastering Python Async
To further explore Python’s async feature, here are some resources that provide more in-depth information:
- Python Modules Selection Tips – Explore advanced module techniques like relative imports and submodules.
Python UUID Module: Universally Unique Identifiers – Dive into UUID creation, manipulation, and usage in Python.
Simplifying Module Import in Python – Dive into importing modules, packages, and external libraries in Python.
Python’s Official Asyncio Documentation – Learn about asyncio, Python’s library for writing single-threaded concurrent code using coroutines.
Asynchronous Programming with Asyncio – Real Python’s guide to understanding asynchronous programming using Python’s asyncio.
Python Async I/O Walkthrough – Gain insights into async and await keywords for asynchronous I/O handling in Python 3.7.
These resources provide comprehensive guides to Python’s async feature, from the basics to more advanced topics. They include detailed explanations, code examples, and practical applications of async in Python.
Wrapping Up: Mastering Python Async for Asynchronous Programming
In this comprehensive guide, we’ve journeyed through the world of Python’s async feature, a powerful tool for writing efficient, non-blocking code.
We began with the basics, understanding how to use Python’s async and await keywords to create and manage coroutines. We then dove into more advanced topics, such as using async with I/O-bound tasks and managing multiple async tasks at once.
Along the way, we explored alternative approaches to asynchronous programming in Python, such as threading and multiprocessing, and tackled common issues you might encounter when using async, such as handling exceptions and debugging async code.
Here’s a quick comparison of the approaches we’ve discussed:
Approach | Pros | Cons |
---|---|---|
Async | Efficient, non-blocking code | Can be complex for beginners |
Threading | Easy to use, can improve performance for I/O-bound tasks | Not truly concurrent due to Python’s GIL |
Multiprocessing | True parallelism, bypasses Python’s GIL | More overhead due to separate processes |
Whether you’re just starting out with Python’s async feature or you’re looking to level up your asynchronous programming skills, we hope this guide has given you a deeper understanding of async and its capabilities.
With its balance of efficiency and versatility, Python’s async feature is a powerful tool for handling multiple tasks simultaneously. Happy coding!