Python UUID / GUID Object Generation Guide (With Examples)
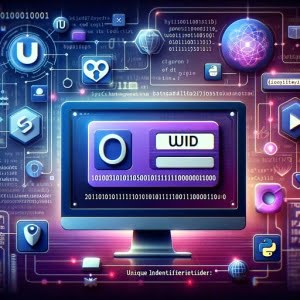
Have you ever marveled at the unique barcodes on products at a supermarket? Each product, even if it’s the same type, has a unique barcode that distinguishes it from the rest.
In the world of Python programming, we have something similar to these unique barcodes, and they’re called UUIDs (Universally Unique Identifiers). These identifiers are quintessential in various aspects of programming, from tagging objects to securing transactions, and much more.
These are generated using a built-in module known as uuid
. This powerful module is simpler to use than you might think.
In this comprehensive guide, we aim to help you use the uuid
module in Python. By the end of this guide, you’ll be generating unique identifiers like a pro. So, let’s dive right into the world of Python UUID!
TL;DR: How to generate a UUID in Python?
In Python, we can generate a Universally Unique Identifier (UUID) using the built-in
uuid
module. An UUID is a 128-bit number used to uniquely identify information in computer systems, often used interchangeably with ‘GUID’. With Python’suuid
module, you can generate a unique identifier in your code without requiring a central authority. Typically, a UUID is represented by 32 lowercase hexadecimal digits, displayed in five groups separated by hyphens.
Here’s how you can generate a UUID in Python:
import uuid
generated_uuid = uuid.uuid4()
print(generated_uuid)
Running this Python code will output a typical UUID like: 123e4567-e89b-12d3-a456-426614174000
Continue reading to learn more about Python’s
uuid
module, its functions and explore other methods to generate UUIDs in Python.
Table of Contents
How Python’s uuid Module Works
Python’s uuid
module is used to generate UUIDs. This module provides immutable UUID objects and functions for creating new UUIDs using various methods.
To generate a UUID, you first need to import the uuid
module, and then you can generate a basic UUID as follows:
import uuid
unique_id = uuid.uuid4()
print(unique_id)
This will print a unique UUID every time you run the code.
Different Versions of UUIDs
There are different versions of UUIDs, each with its own use case. Python’s uuid
module can generate UUIDs of versions 1, 3, 4, and 5.
- uuid1: This generates a UUID using a host ID, sequence number, and the current time.
- uuid3 and uuid5: These generate a UUID using the MD5 (for uuid3) or SHA1 (for uuid5) hash of a namespace identifier and a name.
- uuid4: This generates a random UUID.
Example of generating different versions of UUIDs:
import uuid
# uuid1
uuid1 = uuid.uuid1()
print(uuid1)
# uuid3
uuid3 = uuid.uuid3(uuid.NAMESPACE_DNS, 'example.com')
print(uuid3)
# uuid4
uuid4 = uuid.uuid4()
print(uuid4)
# uuid5
uuid5 = uuid.uuid5(uuid.NAMESPACE_DNS, 'example.com')
print(uuid5)
The choice of which version to use depends on the specific requirements of your project.
How to Validate a UUID
To validate a UUID, you can use the uuid
module’s UUID()
function. This function will raise a ValueError
if the input is not a valid UUID. Here’s an example:
try:
uuid.UUID('123e4567-e89b-12d3-a456-426614174000', version=4)
print('valid UUID')
except ValueError:
print('invalid UUID')
The Importance of UUIDs
UUIDs play a crucial role in ensuring data uniqueness and integrity. They allow you to assign a unique identifier to your data, reducing the chances of duplication.
This is particularly useful in distributed systems, where ensuring the uniqueness of data can be challenging. With UUIDs, you can generate identifiers without the need for a central authority, making them an excellent choice for a variety of applications.
Considerations When Using the Python UUID Module
While the Python uuid
module is powerful, there are some pitfalls and considerations to keep in mind.
For instance, while uuid1
and uuid4
are safe to use, they can potentially leak information about your software and hardware, respectively.
On the other hand, uuid3
and uuid5
can lead to collisions if the same namespace and name are used to generate the UUID.
Python UUIDs and Data Security
UUIDs play a vital role in data security and privacy. They allow for the creation of unique identifiers that can be used to tag and track data without exposing sensitive information. Additionally, because UUIDs are unique across space and time, they can be used to create secure tokens for authentication and authorization purposes.
Common Errors and Their Solutions
Like with any other module, you might encounter some errors while using the Python uuid
module. Here are a few common ones and how you can resolve them:
- AttributeError: module ‘uuid’ has no attribute ‘x’: This error occurs when you try to call a method that doesn’t exist in the
uuid
module. Make sure you’re calling a valid method and that you’ve spelled it correctly. TypeError: ‘x’ object is not callable: This error occurs when you try to call a method without the required parentheses. Ensure you’re including the parentheses, even if you’re not passing any arguments.
Example of these errors:
# AttributeError
try:
uuid.non_existent_method()
except AttributeError as e:
print(e)
# TypeError
try:
uuid4 = uuid.uuid4
uuid4()
except TypeError as e:
print(e)
Best Practices When Using UUIDs in Python
When using UUIDs in Python, there are a few best practices to keep in mind. Firstly, it’s essential to ensure you’re using the correct version of UUID for your specific use case. For instance, if you need a completely random UUID, uuid4()
would be your go-to choice. If a UUID based on a name and namespace is what you require, uuid3()
or uuid5()
would be suitable.
Secondly, while UUIDs are unique, they’re not inherently secure. If your application involves security-sensitive operations, it’s crucial to secure your UUIDs, for example, by implementing encryption.
Lastly, while UUIDs excel at ensuring uniqueness, they might not always be the optimal choice for performance. If performance is a critical concern for your application, other methods of generating unique identifiers might be worth considering.
UUIDs are longer than typical integer-based identifiers, which means they take up more storage space and are slower to compare. If performance is a critical concern for your application, you might want to consider other options.
Alternative Ways to Generate Unique Identifiers in Python
If UUIDs aren’t a good fit for your project, Python offers other ways to generate unique identifiers. For example, you could use the id()
function to get the memory address of an object, or the hash()
function to get a hash value of an object.
Example of generating unique identifiers using other Python functions:
# Using id()
object_id = id(object)
# Using hash()
object_hash = hash(object)
Keep in mind, though, that these methods have their own limitations and might not provide the same level of uniqueness as UUIDs.
Trade-offs Between Using UUIDs and Other Methods
While UUIDs are an excellent tool for generating unique identifiers, they’re not without their drawbacks.
As we mentioned earlier, UUIDs are larger and slower to compare than integer-based identifiers. They can also be more difficult to work with, as they’re not easily memorable or human-readable.
On the other hand, UUIDs offer a level of uniqueness and versatility that’s hard to match with other methods. Ultimately, the choice between using UUIDs or another method will depend on your specific needs and the trade-offs you’re willing to make.
Complementary Python Modules
UUIDs form an integral part of the larger Python ecosystem. They’re frequently used alongside other modules and frameworks.
For instance, many Python web development frameworks, such as Django and Flask, utilize UUIDs for tasks like session management. In the context of data analysis and machine learning, UUIDs can be used to uniquely identify datasets, models, and other resources.
Several Python modules complement the uuid
module. For example, the hashlib
module can be used to generate hash values, which can be used in conjunction with UUIDs for additional security. The random
module can be used to generate random numbers, which can be used as part of a custom unique identifier generation algorithm.
Example of using complementary Python modules:
# Using hashlib
import hashlib
hash_object = hashlib.sha256(b'Hello World')
hex_dig = hash_object.hexdigest()
print(hex_dig)
# Using random
import random
random_number = random.randint(0, 100)
print(random_number)
Further Resources for Python Modules
If you’re aiming to become proficient in Python Modules, here’s a curated list of resources to kick-start your learning journey:
- Python Modules for Efficient Coding – Discover how to handle module errors and exceptions effectively.
Python Subprocess Module: Executing External Processes – Explore Python’s “subprocess” module for running shell commands.
Python Async Await: Concurrent Execution – Dive into the world of asynchronous I/O and non-blocking code in Python.
Modules and Packages in Python Programming – Get a deep understanding of Python’s modules and packages with this book exerpt.
PyO3 Module – Learn about the PyO3 module in Python, a rust-wrapper interpreter that allows building native Python modules.
Python’s Official Documentation on Module – Delve into documentation on C-API Python modules, ideal for C and C++.
Mastering Python Modules, not only will you make your code cleaner and more efficient, but you’ll also unlock a greater depth of Python knowledge.
Using UUIDs with Popular Python Frameworks
Many popular Python frameworks, such as Django and Flask, offer built-in support for UUIDs. In Django, for instance, you can use UUIDs as primary keys for your models, ensuring that each record in your database is uniquely identifiable. Here’s an example of how you can do this:
from django.db import models
import uuid
class MyModel(models.Model):
id = models.UUIDField(primary_key=True, default=uuid.uuid4, editable=False)
Wrapping Up
Universally Unique Identifiers, or UUIDs, also known as Globally Unique Identifiers (GUIDs) are a fundamental tool in software development. They provide a reliable mechanism to generate unique identifiers, which are critical in various applications including database management, web development, and distributed systems.
Python’s uuid
module simplifies the process of generating UUIDs. It offers several methods to create different versions of UUIDs, each tailored to specific use cases. Whether you require a UUID based on the host ID and current time (uuid1
), a name and namespace (uuid3
and uuid5
), or a completely random one (uuid4
), the uuid
module is equipped to handle your needs.
Like any tool, the uuid
module has its own set of considerations. While it’s an efficient tool, it’s not always the optimal choice for every situation. UUIDs are larger and slower to compare than integer-based identifiers, and they can potentially expose information about your software and hardware. But with the right precautions, these pitfalls can be managed effectively.
Beyond Python, UUIDs play a pivotal role in the broader context of software development. They’re utilized in many other programming languages and are integral to many aspects of modern software development, including database management, web development, and particularly, distributed systems.
Just like the unique barcodes on supermarket products, UUIDs ensure uniqueness across multiple platforms and systems. They are a testament to the power and versatility of Python and its extensive library of modules.So, the next time you need to generate a unique identifier in Python, remember the uuid
module. It’s a hidden gem that’s more powerful than you might think.