How To Run a Python Script: Step-by-Step Guide
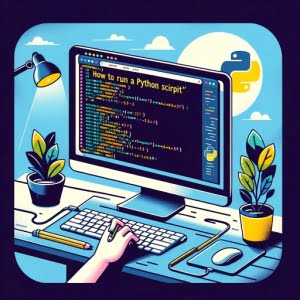
Ever wondered how to bring your Python scripts to life? Like a director calling ‘action’ on a movie set, running a Python script is where your code starts its performance.
This guide will walk you through the process of running Python scripts, from the basics to more advanced techniques. Whether you’re a beginner just starting out, or an intermediate user looking to expand your knowledge, this guide has something for everyone.
So, let’s dive in and start exploring the world of Python scripts!
TL;DR: How Do I Run a Python Script?
To run a Python script, you use the
python
orpython3
command followed by the name of your script. Here’s a simple example:
python my_script.py
# Output:
# [Expected output from your script]
In this example, python
is the command that starts the Python interpreter, and my_script.py
is the name of the Python script you want to run. The output will vary depending on what your script is designed to do.
Stay tuned for more detailed instructions and advanced usage scenarios. Whether you’re a beginner just starting out, or an intermediate user looking to expand your knowledge, this guide has something for everyone.
Table of Contents
- Running Python Scripts: The Basics
- Potential Issues and Best Practices
- Running Python Scripts with Command Line Arguments
- Running Python Scripts: Advanced Techniques
- Common Issues and Fixes When Running Python Scripts
- Understanding Python Scripts
- The Python Interpreter: Bringing Scripts to Life
- Python Scripts in Larger Projects
- Exploring Related Concepts
- Recap: Running Python Scripts Made Easy
Running Python Scripts: The Basics
Running a Python script is a straightforward process. You start by opening a command line interface like Terminal on macOS or Command Prompt on Windows. Navigate to the directory where your Python script is located using the cd
command. Once you’re in the right directory, you can run your script.
Here’s a simple example:
# Navigate to the directory where your script is located
cd /path/to/your/script
# Run your Python script
python my_script.py
# Output:
# [Expected output from your script]
In this example, cd /path/to/your/script
is the command that changes the current directory to the one where your script is located. python my_script.py
is the command that starts the Python interpreter and runs your script. The output will vary depending on what your script is designed to do.
It’s important to note that if you’re using Python 3, you might need to use the python3
command instead of python
. The exact command depends on how Python is installed on your system.
Potential Issues and Best Practices
One common issue beginners face is running scripts from the wrong directory. If you try to run a script from a directory where it doesn’t exist, you’ll get a No such file or directory
error. Make sure you’re in the right directory before you try to run your script.
Another common issue is syntax errors in your script. If your script has a syntax error, the Python interpreter will show an error message and stop running the script. Make sure your script is free of syntax errors before you try to run it.
As a best practice, always include a shebang line at the top of your Python scripts. A shebang line is a line that starts with #!
followed by the path to the Python interpreter. This tells the system that the script is a Python script and should be run with the Python interpreter. Here’s an example of a shebang line:
#!/usr/bin/env python3
This shebang line tells the system to run the script with the Python 3 interpreter.
Running Python Scripts with Command Line Arguments
As you become more comfortable with running Python scripts, you might find yourself needing to pass arguments from the command line to your script. Python makes this easy with the sys
module, which has a argv
list that holds the command-line arguments passed to the script.
Here’s a simple example of a script that accepts command line arguments:
# script.py
import sys
print('Number of arguments:', len(sys.argv), 'arguments.')
print('Argument List:', str(sys.argv))
# To run the script, you would use the following command:
python script.py arg1 arg2 arg3
# Output:
# Number of arguments: 4 arguments.
# Argument List: ['script.py', 'arg1', 'arg2', 'arg3']
In this example, sys.argv
is a list in Python, which contains the command-line arguments passed to the script. With the len(sys.argv) function you can count the number of arguments. If the length of sys.argv is less than 2, you know that no command line options were passed to Python. sys.argv[0] is the script name (it is operating system dependent whether this is a full pathname or not). If the command was executed using the -c command line option to the interpreter, sys.argv[0] is set to the string ‘-c’. If no script name was passed to the Python interpreter, sys.argv[0] is the empty string.
Remember that the name of the script running is always the first argument in the sys.argv
list. That’s why our output shows four arguments even though we only passed three: the script name is included as the first argument.
This opens up a wide range of possibilities for using command line arguments to customize the behavior of your scripts. For example, you could write a script that performs different actions based on the arguments it receives, or a script that processes a list of files specified as arguments.
Running Python Scripts: Advanced Techniques
As you progress on your Python journey, you’ll find that there are several ways to run Python scripts beyond the basic command line approach. Let’s explore some of these alternative methods.
Running Scripts from an IDE
Integrated Development Environments (IDEs) like PyCharm or Visual Studio Code offer built-in tools to run Python scripts. This can simplify the process and provide additional features like debugging and code completion.
Here’s how you can run a Python script in PyCharm:
- Open your Python script in PyCharm.
- Right-click anywhere in the script, and select
Run '[Script Name]'
.
Your script will run in a dedicated Python console within PyCharm, and the output will be displayed there.
Using Shebang Lines on Unix-based Systems
On Unix-based systems like Linux or macOS, you can use shebang lines to specify the interpreter for your script. Here’s an example:
#!/usr/bin/env python3
print('Hello, World!')
# To run the script, you would use the following command:
./script.py
# Output:
# Hello, World!
In this example, the shebang line #!/usr/bin/env python3
tells the system to run the script with the Python 3 interpreter. The ./
before the script name is a Unix convention that means ‘run this file in the current directory’.
Making Python Scripts Executable
You can also make your Python scripts executable, which allows you to run them just like any other program. To do this, you need to add a shebang line to your script and change its permissions to allow execution. Here’s how:
# Add a shebang line to your script
echo '#!/usr/bin/env python3' > script.py
# Change the script's permissions to allow execution
chmod +x script.py
# Now you can run your script like this:
./script.py
# Output:
# [Expected output from your script]
In this example, the chmod +x script.py
command changes the permissions of the script to allow it to be executed. The ./script.py
command then runs the script.
Each of these methods has its own advantages and disadvantages. Running scripts from an IDE can be more convenient and offers additional features, but it also requires you to have the IDE installed and can be slower than running scripts from the command line. Using shebang lines or making scripts executable allows you to run scripts more easily, but these methods are specific to Unix-based systems and won’t work on Windows.
Common Issues and Fixes When Running Python Scripts
Running Python scripts can sometimes result in errors. Let’s discuss some common issues and how to resolve them.
Syntax Errors
Syntax errors occur when the Python interpreter encounters code it doesn’t understand. This could be due to a typo, incorrect indentation, or forgetting to close a parenthesis or quotation mark.
# An example of a syntax error
print('Hello, World!
# Output:
# SyntaxError: EOL while scanning string literal
In this example, we forgot to close the quotation marks around the string. The Python interpreter gives us a SyntaxError
with a message indicating what it thinks went wrong.
To fix syntax errors, carefully check your code for typos or missing symbols. An IDE with syntax highlighting can be very helpful for this.
Indentation Errors
Python uses indentation to determine the structure of code. If your code is not properly indented, you’ll get an IndentationError
.
# An example of an indentation error
def greet(name):
print(f'Hello, {name}!')
# Output:
# IndentationError: expected an indented block
In this example, the print
statement should be indented to indicate that it’s part of the greet
function. The Python interpreter gives us an IndentationError
because it expects an indented block after the function definition.
To fix indentation errors, make sure that each block of code is properly indented. In Python, it’s standard to use four spaces for each level of indentation.
Module Not Found Errors
If you try to import a module that isn’t installed or doesn’t exist, you’ll get a ModuleNotFoundError
.
# An example of a module not found error
import non_existent_module
# Output:
# ModuleNotFoundError: No module named 'non_existent_module'
In this example, we’re trying to import a module that doesn’t exist. The Python interpreter gives us a ModuleNotFoundError
with the name of the module that it couldn’t find.
To fix module not found errors, make sure that the module you’re trying to import is installed and that you’ve spelled its name correctly. You can install modules using pip, Python’s package installer.
Understanding Python Scripts
Python scripts are essentially text files with the .py extension containing Python code that the Python interpreter can execute. They are the building blocks of any Python project, and they allow you to automate tasks, implement functionality, and even build entire applications.
# A simple Python script
print('Hello, World!')
# Output:
# Hello, World!
In this example, our script consists of a single line of code that prints ‘Hello, World!’ to the console. When we run this script, the Python interpreter reads the script, translates the print
function into a series of low-level instructions that your computer can understand, and then executes those instructions.
The Python Interpreter: Bringing Scripts to Life
The Python interpreter is the engine that runs Python scripts. When you type python my_script.py
into your command line, you’re telling the Python interpreter to read your script, translate it into machine code (the binary code that computers understand), and execute it.
The interpreter starts at the top of your script and executes each line of code in order, one at a time. If it encounters an error, it will stop and display an error message.
Python is an interpreted language, which means that it executes scripts line by line, as opposed to compiled languages that translate the entire program into machine code before running it. This makes Python ideal for scripting and rapid application development, but it also means that Python scripts can be slower than programs written in compiled languages.
Understanding these fundamentals of Python scripts and the Python interpreter is key to becoming proficient in Python and mastering the art of running Python scripts.
Python Scripts in Larger Projects
Running Python scripts is not just for small tasks or individual scripts. In larger projects and real-world applications, you will often find Python scripts working together, interacting with each other, and forming the backbone of the system.
For instance, in a web application built with Django (a Python web framework), you might have several Python scripts, each handling a different part of the application – one script for handling user requests, another for processing data, and so on. These scripts need to be run and managed effectively for the application to function correctly.
Exploring Related Concepts
To truly master running Python scripts, you should also explore related concepts like Python modules, packages, and virtual environments.
- Python Modules: A module is a file containing Python definitions and statements. It’s a way of organizing related code into a single file. You can import modules into your scripts to use the functions and classes they define.
# Importing the math module
import math
# Using a function from the math module
print(math.sqrt(16))
# Output:
# 4.0
In this example, we import the math
module and use its sqrt
function to calculate the square root of 16.
- Python Packages: A package is a way of organizing related modules into a directory hierarchy. It’s like a folder that contains multiple module files and a special
__init__.py
file to indicate that it’s a package. Virtual Environments: A virtual environment is an isolated environment where you can install packages without affecting your global Python installation. It’s a good practice to use virtual environments for your projects to avoid conflicts between package versions.
Further Resources for Python Script Mastery
For those looking to delve deeper into running Python scripts and related concepts, here are some valuable resources:
- Python’s official documentation: A comprehensive resource covering all aspects of Python, from running scripts to understanding advanced concepts.
Real Python: Offers a wealth of tutorials and articles on a wide range of Python topics, from beginner to advanced.
Python for Beginners: A helpful resource for those new to Python, with easy-to-understand tutorials and guides.
Recap: Running Python Scripts Made Easy
In this guide, we’ve explored the ins and outs of how to run a Python script.
We started with the basics, running a script from the command line using the python
or python3
command. We also discussed common issues such as syntax errors, indentation errors, and module not found errors, and provided solutions for each of these problems.
We then delved into more advanced techniques, like passing command line arguments to a script using the sys
module and running scripts from an Integrated Development Environment (IDE). We also covered Unix-specific methods like using shebang lines and making scripts executable.
Here’s a quick comparison of the methods we’ve discussed:
Method | Difficulty Level | Pros | Cons |
---|---|---|---|
Running scripts from the command line | Beginner | Easy to learn, doesn’t require additional software | Can be limited in functionality |
Passing command line arguments | Intermediate | Allows for more complex scripts | Can be complex for beginners |
Running scripts from an IDE | Intermediate | Offers additional features like debugging and code completion | Requires installation of the IDE |
Using shebang lines/making scripts executable | Advanced | Allows scripts to be run like any other program, doesn’t require the python command | Specific to Unix-based systems |
Lastly, we discussed the importance of understanding related concepts like Python modules, packages, and virtual environments. Mastering these concepts will allow you to write more complex scripts and manage larger Python projects effectively.
Remember, the key to mastering Python – or any programming language – is practice. Keep experimenting, keep learning, and don’t be afraid to make mistakes. Happy coding!