Tkinter: Python GUI Application Development Guide
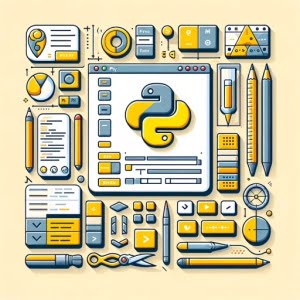
Are you on a quest to create a Graphical User Interface (GUI) in Python? Look no further. Tkinter, Python’s standard GUI package, is like a skilled architect at your disposal, allowing you to design and build user interfaces with ease and precision.
This comprehensive guide will walk you through the process of creating a GUI using Tkinter in Python. Whether you’re a beginner just starting out or an intermediate looking to level up your skills, this guide has got you covered.
So, let’s embark on this exciting journey of mastering Tkinter and creating impressive Python GUIs.
TL;DR: How Do I Create a GUI in Python Using Tkinter?
To create a GUI in Python using Tkinter, you first import the Tkinter module, create a window, and then add widgets to it. Here’s a simple example:
import tkinter as tk
window = tk.Tk()
label = tk.Label(window, text='Hello, Tkinter')
label.pack()
window.mainloop()
# Output:
# A window pops up with a label that says 'Hello, Tkinter'
This code creates a window with a label that says ‘Hello, Tkinter’. The import tkinter as tk
line brings in the Tkinter module. window = tk.Tk()
creates a new window. label = tk.Label(window, text='Hello, Tkinter')
creates a new label widget, and label.pack()
adds it to the window. Finally, window.mainloop()
starts the GUI event loop, which waits for user interaction.
This is just the tip of the iceberg when it comes to creating GUIs with Tkinter. Continue reading for a more detailed guide that will take you from a beginner to an expert in Python GUI creation with Tkinter.
Table of Contents
- Crafting Your First GUI with Tkinter
- Exploring Tkinter’s Advanced Features
- Exploring Alternative Libraries for Python GUIs
- Common Tkinter Pitfalls and Solutions
- Uncovering Tkinter: Python’s Standard GUI Package
- Understanding GUI Design Principles
- Embracing Event-Driven Programming
- Tkinter GUIs: Impact in Real-World Applications
- Exploring Related Concepts: Multithreading and Network Programming
- Tkinter Python GUI: A Comprehensive Recap
Crafting Your First GUI with Tkinter
Tkinter simplifies the process of creating a GUI in Python. Let’s start with the basics: creating windows and adding widgets like labels, buttons, and text boxes.
Creating a Window
The first step in creating a GUI with Tkinter is to make a window. This is done by creating an instance of the Tk
class from the Tkinter module.
import tkinter as tk
window = tk.Tk()
window.mainloop()
# Output:
# A blank window pops up
The Tk()
function creates a top-level window. mainloop()
is an infinite loop used to run the application, wait for an event to occur, and process the event till the window is not closed.
Adding Widgets: Labels, Buttons, and Text Boxes
Widgets are the elements that give your application functionality. Tkinter provides various controls, such as buttons, labels, and text boxes used in a GUI application. These controls are commonly called widgets.
Labels
Labels are used to display texts or images. The text displayed by this widget can be updated at any time you want.
import tkinter as tk
window = tk.Tk()
label = tk.Label(window, text='Hello, Tkinter')
label.pack()
window.mainloop()
# Output:
# A window pops up with a label that says 'Hello, Tkinter'
The Label()
widget is a standard Tkinter widget. An instance of this class is created by passing the parent window and the text you want to display as parameters.
Buttons
Buttons in Tkinter are pretty straightforward and are created using the Button
class.
import tkinter as tk
def click():
print('Button clicked!')
window = tk.Tk()
button = tk.Button(window, text='Click me', command=click)
button.pack()
window.mainloop()
# Output:
# A window pops up with a button. When clicked, 'Button clicked!' is printed in the console.
The Button()
widget creates a button that can contain text and can perform an action when clicked. The command
parameter is the function to be executed when the button is clicked.
Text Boxes
Text boxes (also called entry widgets) allow the user to input a single line of text.
import tkinter as tk
window = tk.Tk()
text_box = tk.Entry(window)
text_box.pack()
window.mainloop()
# Output:
# A window pops up with a text box.
The Entry()
widget allows the user to enter a single line of text that can be retrieved with the get()
method.
Tkinter makes it easy to create and manage GUIs in Python, but it’s important to understand the underlying principles to avoid potential pitfalls. For example, forgetting to call mainloop()
will result in a window that closes immediately. Also, remember that widget methods like pack()
are needed to organize widgets in the window.
Exploring Tkinter’s Advanced Features
As you get more comfortable with Tkinter, you’ll find it offers more than just basic widgets. It provides a set of powerful tools for creating menus, handling events, and using more complex widgets like lists and canvases.
Creating Menus
Menus are an integral part of most GUI applications. They provide a simple way for users to access various commands. Here’s how you can create a menu in Tkinter:
import tkinter as tk
def show_about():
print('This is a Tkinter GUI')
window = tk.Tk()
menubar = tk.Menu(window)
window.config(menu=menubar)
file_menu = tk.Menu(menubar, tearoff=0)
menubar.add_cascade(label='File', menu=file_menu)
file_menu.add_command(label='About', command=show_about)
window.mainloop()
# Output:
# A window pops up with a 'File' menu. When 'About' is clicked, 'This is a Tkinter GUI' is printed in the console.
In this example, we first create a Menu
widget. We then associate this menu with our window using the config
method. Next, we create a submenu (in this case, a ‘File’ menu), and add it to the main menu using the add_cascade
method. Finally, we add a command to the ‘File’ menu using the add_command
method.
Handling Events
Event handling is a key part of any GUI application. In Tkinter, events like button clicks or key presses can be handled using command callbacks or event bindings.
import tkinter as tk
def on_keypress(event):
print(f'You pressed {event.char}')
window = tk.Tk()
window.bind('<KeyPress>', on_keypress)
window.mainloop()
# Output:
# When a key is pressed in the window, 'You pressed [key]' is printed in the console.
In this example, we use the bind
method to bind a key press event to a callback function. Whenever a key is pressed, the on_keypress
function is called, and the pressed key is printed.
Using Complex Widgets: Lists and Canvases
Tkinter provides several complex widgets, such as lists and canvases, which allow for more advanced GUI designs.
import tkinter as tk
window = tk.Tk()
listbox = tk.Listbox(window)
listbox.insert(1, 'Python')
listbox.insert(2, 'Java')
listbox.insert(3, 'C++')
listbox.pack()
canvas = tk.Canvas(window, width=100, height=100)
canvas.create_rectangle(20, 20, 80, 80, fill='blue')
canvas.pack()
window.mainloop()
# Output:
# A window pops up with a list box containing 'Python', 'Java', and 'C++' and a canvas with a blue square.
In this example, we first create a Listbox
widget and insert a few items into it. We then create a Canvas
widget and draw a blue square on it.
These examples showcase some of the more advanced features of Tkinter. By understanding and utilizing these features, you can create more complex and interactive GUIs in Python.
Exploring Alternative Libraries for Python GUIs
While Tkinter is a powerful tool for creating GUIs in Python, it’s not the only game in town. Other libraries such as PyQt and wxPython also offer unique features and capabilities.
PyQt: A Step Up in Complexity
PyQt is a set of Python bindings for The Qt Company’s Qt application framework. It supports a variety of features, including advanced graphics, networking, and database functionality.
from PyQt5.QtWidgets import QApplication, QLabel
app = QApplication([])
label = QLabel('Hello, PyQt')
label.show()
app.exec_()
# Output:
# A window pops up with a label that says 'Hello, PyQt'
In this example, we create a QLabel widget (similar to a Tkinter Label) and display it. PyQt’s main advantage over Tkinter is its breadth of features and capabilities, but it’s also more complex and has a steeper learning curve.
wxPython: Native Look and Feel
wxPython is a wrapper for the wxWidgets C++ library, which allows Python programs to create a native look and feel for their GUIs.
import wx
app = wx.App()
frame = wx.Frame(None, -1, 'Hello, wxPython')
frame.Show()
app.MainLoop()
# Output:
# A window pops up with a title that says 'Hello, wxPython'
In this example, we create a wx.Frame (similar to a Tkinter window) and display it. wxPython’s main advantage is its ability to create GUIs that look and feel native on a variety of platforms.
Tkinter, PyQt, or wxPython?
When deciding which library to use, it’s important to consider your specific needs. Tkinter is a great choice for beginners and for simple applications due to its simplicity and ease of use. PyQt, with its extensive features, is suitable for more complex applications. wxPython, on the other hand, is ideal if you need your application to have a native look and feel on multiple platforms.
Common Tkinter Pitfalls and Solutions
While Tkinter is a robust tool for creating Python GUIs, you may encounter some common issues, especially when dealing with layout management and event handling. Let’s discuss these challenges and provide some solutions.
Layout Management Woes
One common issue is the misplacement or overlapping of widgets. This typically occurs when you’re using the pack
geometry manager, which places widgets in a block, one after the other.
import tkinter as tk
window = tk.Tk()
label1 = tk.Label(window, text='Hello, Tkinter').pack()
label2 = tk.Label(window, text='Goodbye, Tkinter').pack()
window.mainloop()
# Output:
# A window pops up with 'Hello, Tkinter' and 'Goodbye, Tkinter' labels stacked vertically.
In this example, the labels are stacked vertically, which may not be what you intended. To have more control over widget placement, use the grid
geometry manager instead.
import tkinter as tk
window = tk.Tk()
label1 = tk.Label(window, text='Hello, Tkinter')
label1.grid(row=0, column=0)
label2 = tk.Label(window, text='Goodbye, Tkinter')
label2.grid(row=0, column=1)
window.mainloop()
# Output:
# A window pops up with 'Hello, Tkinter' and 'Goodbye, Tkinter' labels placed side by side.
With grid
, you can specify the row and column of each widget, allowing for more complex layouts.
Event Handling Hurdles
Another common issue is unresponsive widgets, often caused by misconfigured event handlers.
import tkinter as tk
def click():
print('Button clicked!')
window = tk.Tk()
button = tk.Button(window, text='Click me')
button.pack()
window.mainloop()
# Output:
# A window pops up with a button. When clicked, nothing happens.
In this example, the button doesn’t do anything when clicked because we forgot to attach the click
function to the command
parameter. Here’s the corrected code:
import tkinter as tk
def click():
print('Button clicked!')
window = tk.Tk()
button = tk.Button(window, text='Click me', command=click)
button.pack()
window.mainloop()
# Output:
# A window pops up with a button. When clicked, 'Button clicked!' is printed in the console.
Remember to always attach a function to the command
parameter of a button or a similar widget to ensure it performs an action when interacted with.
By understanding these common issues and their solutions, you can avoid many headaches when creating GUIs with Tkinter.
Uncovering Tkinter: Python’s Standard GUI Package
Tkinter is Python’s standard GUI (Graphical User Interface) package. It’s an interface to the Tk GUI toolkit, which is why it’s often referred to as ‘Tk interface,’ or ‘Tkinter’ for short.
Tkinter is a cross-platform library, meaning it can run on various operating systems, including Windows, Mac OS, and Linux. It’s included with standard Python distributions, so there’s no need for additional downloads or installations.
import tkinter as tk
# Output:
# No error means Tkinter is correctly installed.
In this example, we import the Tkinter module. If Tkinter is correctly installed, this will run without errors.
Understanding GUI Design Principles
GUI design revolves around widgets, which are the building blocks of a GUI application. Widgets include elements like buttons, text boxes, labels, menus, and more. In Tkinter, each widget is an instance of a class, and you create a GUI by creating instances of these classes.
Embracing Event-Driven Programming
Tkinter is event-driven, meaning it waits for events like button clicks or key presses and responds accordingly. This is done using an event loop, which is started with the mainloop
method.
import tkinter as tk
window = tk.Tk()
window.mainloop()
# Output:
# A blank window pops up and waits for user interaction.
In this example, we create a window and start the event loop. The window will stay open until the user closes it.
Understanding these background fundamentals of Tkinter and GUI design principles can help you better grasp how to create GUI applications in Python.
Tkinter GUIs: Impact in Real-World Applications
Creating GUIs with Tkinter isn’t just an academic exercise; it has real-world applications. From desktop apps to games, Python GUIs built with Tkinter are everywhere.
Desktop Applications
Tkinter is often used in desktop applications. It allows developers to create user-friendly interfaces that make their applications accessible to non-technical users.
import tkinter as tk
window = tk.Tk()
label = tk.Label(window, text='This could be a complex desktop application!')
label.pack()
window.mainloop()
# Output:
# A window pops up with a label that says 'This could be a complex desktop application!'
In this simple example, we create a window with a label. In a real-world desktop application, this could be a complex interface with menus, buttons, text boxes, and other widgets.
Games
Tkinter is also used in game development. While it’s not as powerful as dedicated game development libraries like Pygame or Panda3D, Tkinter is perfect for simple games and prototypes.
import tkinter as tk
window = tk.Tk()
canvas = tk.Canvas(window, width=400, height=400)
canvas.pack()
canvas.create_rectangle(50, 50, 350, 350, fill='blue')
window.mainloop()
# Output:
# A window pops up with a canvas. The canvas contains a blue square, which could be a simple game character.
In this example, we create a canvas and draw a blue square on it. In a real game, this could be a character, an enemy, a piece of terrain, or any other game element.
Exploring Related Concepts: Multithreading and Network Programming
Once you’re comfortable with Tkinter, you might want to explore related concepts like multithreading in GUI applications and network programming in Python. These advanced topics can help you create more complex and interactive applications.
Further Resources for Mastering Tkinter
If you’re interested in diving deeper into Tkinter and Python GUI development, here are some resources that can help:
- Beginner’s Guide to Python GUI – Discover the power of Python for creating interactive interfaces.
Django Framework Tutorial: Web Development Simplified – Learn Django for web development in Python.
Building Desktop Applications with PyQt – Explore PyQt’s rich set of widgets and tools.
Python’s official Tkinter documentation – A resource with detailed explanations of all Tkinter classes and methods.
TkDocs – A thorough guide to Tkinter (and Tk) that includes tutorials, examples, and more.
Python GUI Programming With Tkinter – A series of articles from Real Python that cover the various topics of Tkinter.
Tkinter Python GUI: A Comprehensive Recap
In this guide, we’ve journeyed through the process of creating a GUI in Python using Tkinter. We started with the basics, creating windows and adding simple widgets like labels and buttons. From there, we delved into more complex features, such as creating menus, handling events, and using advanced widgets like lists and canvases.
We also explored common issues that you might encounter when creating a GUI with Tkinter, such as layout management challenges and event handling hurdles. We provided solutions and workarounds for these issues, helping you to avoid potential pitfalls.
As we expanded our horizons, we looked at alternative libraries for creating Python GUIs. PyQt, with its extensive features, is suitable for complex applications, while wxPython allows for a native look and feel across multiple platforms. However, Tkinter’s simplicity and ease of use make it an excellent choice for beginners and for simple to intermediate applications.
Library | Use Case |
---|---|
Tkinter | Beginners, Simple to Intermediate Applications |
PyQt | Complex Applications |
wxPython | Native Look and Feel |
Finally, we delved into the background and fundamentals of Tkinter and GUI design, helping you to understand the principles that underpin your Python GUI creations. We also discussed the real-world applications of Tkinter GUIs, from desktop applications to simple games, and encouraged further exploration into related concepts like multithreading and network programming.
Remember, creating a GUI in Python using Tkinter is more than just writing code; it’s about designing an intuitive, user-friendly interface that makes your application accessible to others. So keep experimenting, keep learning, and most importantly, have fun with it!