Git Bash Commands: A Bash Scripting Guide
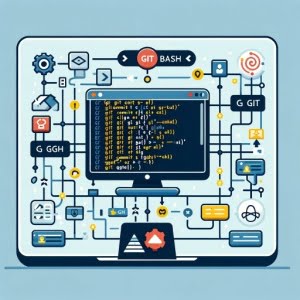
Are you finding it difficult to navigate the world of Git Bash commands? You’re not alone. Many developers, both beginners and experienced, often find themselves lost in the dense forest of commands.
Think of Git Bash commands as a compass – a tool that guides you through the intricate world of software development, helping you track changes, collaborate with others, and manage your code. Git Bash commands are an essential tool for any developer, providing a powerful way to leverage Git, the most widely used version control system.
In this guide, we’ll walk you through the process of using Git Bash commands, from the basics to more advanced techniques. We’ll cover everything from initializing a repository with git init
, staging changes with git add
, saving changes with git commit
, to sending changes to a remote repository with git push
. We’ll also delve into more advanced topics like branching, merging, and resolving conflicts.
So, let’s embark on this journey and start mastering Git Bash commands!
TL;DR: What are some basic Git Bash commands?
Some basic Git Bash commands include
git init
to initialize a new repository,git add
to stage changes,git commit
to save changes, andgit push
to send changes to a remote repository.
Here’s a simple example:
# Initialize a new Git repository
git init
# Stage changes
git add .
# Commit changes
git commit -m 'Initial commit'
# Push changes to a remote repository
git push origin master
# Output:
# Initialized empty Git repository in /path/to/directory/.git/
# [master (root-commit) 0a0b0c0] Initial commit
# 1 file changed, 0 insertions(+), 0 deletions(-)
# create mode 100644 file.txt
# Counting objects: 3, done.
# Writing objects: 100% (3/3), 212 bytes | 212.00 KiB/s, done.
# Total 3 (delta 0), reused 0 (delta 0)
# To github.com:username/repository.git
# * [new branch] master -> master
In this example, we first initialize a new Git repository with git init
. Next, we stage our changes with git add .
, which adds all the files in the current directory. We then commit our changes with git commit -m 'Initial commit'
, creating a new commit with the message ‘Initial commit’. Finally, we push our changes to a remote repository with git push origin master
.
This is just a basic introduction to Git Bash commands. Continue reading for a more comprehensive list of commands and their uses, along with detailed explanations and examples.
Table of Contents
Git Bash Basics: The Essential Commands
Git Bash is a powerful tool, and understanding its basic commands is the first step towards mastering it. Let’s explore some of the fundamental Git Bash commands and their uses.
Initializing a Repository with git init
The git init
command is used to create a new, empty repository in Git. It’s the first command you use when starting a new project. Here’s how it works:
# Create a new directory
mkdir my_project
cd my_project
# Initialize a new Git repository
git init
# Output:
# Initialized empty Git repository in /path/to/my_project/.git/
In this example, we first create a new directory with the mkdir
command, then navigate into it with the cd
command. Finally, we initialize a new Git repository with git init
. The output confirms that we’ve successfully created an empty Git repository.
Staging Changes with git add
The git add
command is used to add changes to the staging area in Git. This is where you prepare your changes before committing them. Let’s see it in action:
# Create a new file
echo 'Hello, Git!' > hello.txt
# Add the file to the staging area
git add hello.txt
# Check the status of the repository
git status
# Output:
# On branch master
# Changes to be committed:
# (use "git restore --staged <file>..." to unstage)
# new file: hello.txt
In this example, we first create a new file hello.txt
with the echo
command. We then add this file to the staging area with git add
. The git status
command shows that our file is ready to be committed.
Committing Changes with git commit
The git commit
command is used to save your changes to the local repository. Each commit creates a unique ID that allows you to keep track of your modifications. Here’s an example:
# Commit the changes
git commit -m 'Add hello.txt'
# Output:
# [master (root-commit) 1a2b3c4] Add hello.txt
# 1 file changed, 1 insertion(+)
# create mode 100644 hello.txt
In this example, we commit our changes with git commit -m 'Add hello.txt'
. The -m
option allows us to add a meaningful message to our commit. The output shows that we’ve successfully committed our changes.
Pushing Changes with git push
The git push
command is used to send your committed changes to a remote repository. It’s how you share your work with others. Here’s how you use it:
# Add a remote repository
git remote add origin https://github.com/username/my_project.git
# Push changes to the remote repository
git push -u origin master
# Output:
# Counting objects: 3, done.
# Writing objects: 100% (3/3), 212 bytes | 212.00 KiB/s, done.
# Total 3 (delta 0), reused 0 (delta 0)
# To https://github.com/username/my_project.git
# * [new branch] master -> master
In this example, we first add a remote repository with git remote add
. We then push our changes to this remote repository with git push
. The -u
option sets the upstream, which means Git will remember your preferences for future pushes to this repository.
These are some of the most essential Git Bash commands. Mastering these will help you manage your code effectively and collaborate with others seamlessly.
Diving Deeper: Intermediate Git Bash Commands
As you grow more comfortable with Git Bash, you’ll find that the basic commands are just the tip of the iceberg. Let’s explore some of the more advanced commands and workflows that can streamline your development process.
Branching with git branch
and git checkout
Branching is a powerful feature in Git that allows you to work on different versions of your project simultaneously. Here’s how you can create a new branch and switch to it using git branch
and git checkout
:
# Create a new branch
git branch feature
# Switch to the new branch
git checkout feature
# Output:
# Switched to branch 'feature'
In this example, we first create a new branch called ‘feature’ with git branch
. We then switch to this branch with git checkout
. The output confirms that we’ve successfully switched to our new branch.
Merging with git merge
Once you’ve completed your work on a branch, you can integrate it back into your main codebase with git merge
. Here’s an example:
# Switch back to the master branch
git checkout master
# Merge the feature branch into master
git merge feature
# Output:
# Updating a1b2c3d..e4f5g6h
# Fast-forward
# hello.txt | 2 +-
# 1 file changed, 1 insertion(+), 1 deletion(-)
In this example, we first switch back to the master branch with git checkout
. We then merge our ‘feature’ branch into ‘master’ with git merge
. The output shows that our changes have been successfully integrated.
Resolving Conflicts
Sometimes, when you try to merge branches, you might encounter conflicts. These occur when the same part of your code has been modified in two different branches. Here’s how you can resolve conflicts:
# Output when a conflict occurs:
# Auto-merging hello.txt
# CONFLICT (content): Merge conflict in hello.txt
# Automatic merge failed; fix conflicts and then commit the result.
# Open the file with conflicts and resolve them
# Then add the resolved file to the staging area
git add hello.txt
# Commit the resolved changes
git commit -m 'Resolved merge conflict'
# Output:
# [master 1a2b3c4] Resolved merge conflict
In this example, we first see the output when a conflict occurs. To resolve the conflict, we open the file with conflicts and manually fix them. We then add the resolved file to the staging area with git add
and commit our changes with git commit
.
These advanced commands and workflows can greatly enhance your productivity and collaboration in Git. By mastering them, you’ll be well on your way to becoming a Git Bash expert.
Exploring Further: Expert-level Git Bash Commands
Once you’ve mastered the basics and intermediate Git Bash commands, it’s time to explore some expert-level techniques. These techniques offer alternative ways to use commands, allowing you to optimize your workflows and scripts.
Using Flags and Options
Many Git Bash commands have flags and options that provide additional functionalities. Let’s look at some examples:
Viewing Git Log in One Line
The git log
command shows the commit history. By adding --oneline
flag, you can view each commit on a single line, which is much easier to read.
# View the commit history in one line
git log --oneline
# Output:
# 1a2b3c4 (HEAD -> master) Add feature
# e4f5g6h Initial commit
In this example, the git log --oneline
command shows each commit on a single line. The output includes the commit hash and the commit message.
Staging and Committing in One Step
Instead of using git add
and git commit
separately, you can stage and commit changes in one step with the -a
flag.
# Make some changes to hello.txt
echo 'Hello, Git Bash!' >> hello.txt
# Stage and commit changes in one step
git commit -a -m 'Update hello.txt'
# Output:
# [master 1a2b3c4] Update hello.txt
# 1 file changed, 1 insertion(+)
In this example, we first make some changes to hello.txt
. We then stage and commit these changes in one step with git commit -a -m 'Update hello.txt'
. The -a
flag automatically stages all modified and deleted files, and the -m
option adds a commit message.
Combining Commands in Scripts
You can combine multiple Git Bash commands into a script to automate repetitive tasks. Here’s an example of a script that creates a new branch, makes changes, and pushes the changes to a remote repository:
#!/bin/bash
# Create a new branch and switch to it
git checkout -b feature
# Make some changes
echo 'New feature' >> feature.txt
# Stage and commit changes
git commit -a -m 'Add feature'
# Push changes to the remote repository
git push origin feature
# Output:
# Switched to a new branch 'feature'
# [feature 1a2b3c4] Add feature
# 1 file changed, 1 insertion(+)
# create mode 100644 feature.txt
# Counting objects: 3, done.
# Writing objects: 100% (3/3), 230 bytes | 230.00 KiB/s, done.
# Total 3 (delta 0), reused 0 (delta 0)
# To https://github.com/username/my_project.git
# * [new branch] feature -> feature
In this script, we first create a new branch and switch to it with git checkout -b
. We then make some changes, stage and commit these changes, and finally push them to a remote repository. This script automates the entire workflow, saving you time and effort.
These expert-level techniques can help you use Git Bash commands more efficiently and effectively. By mastering these techniques, you’ll be able to handle even the most complex Git tasks with ease.
Troubleshooting Common Git Bash Issues
While Git Bash is an essential tool for developers, it can sometimes throw up errors that can be challenging to diagnose and resolve. Let’s explore some common issues you might encounter when using Git Bash commands and provide solutions to overcome them.
‘fatal: not a git repository’
This error typically occurs when you try to run a Git command outside of a Git repository. Here’s an example:
# Try to run a Git command outside of a Git repository
git status
# Output:
# fatal: not a git repository (or any of the parent directories): .git
In this example, we try to run git status
outside of a Git repository, which results in the ‘fatal: not a git repository’ error. The solution is to navigate to a Git repository or initialize a new one with git init
.
‘fatal: remote origin already exists’
This error occurs when you try to add a remote repository that already exists. Here’s an example:
# Try to add a remote repository that already exists
git remote add origin https://github.com/username/my_project.git
# Output:
# fatal: remote origin already exists.
In this example, we try to add a remote repository with git remote add
, which results in the ‘fatal: remote origin already exists’ error. The solution is to either use a different name for the remote repository or remove the existing remote repository with git remote remove
before adding the new one.
‘Your branch is ahead of ‘origin/master’ by X commits’
This message appears when you have committed changes to your local repository that have not been pushed to the remote repository. Here’s an example:
# Make some changes and commit them
echo 'More changes' >> hello.txt
git commit -a -m 'More changes'
# Check the status of the repository
git status
# Output:
# On branch master
# Your branch is ahead of 'origin/master' by 1 commit.
# (use "git push" to publish your local commits)
In this example, we make some changes and commit them with git commit
. When we check the status of the repository with git status
, we see the message ‘Your branch is ahead of ‘origin/master’ by 1 commit’. The solution is to push your changes to the remote repository with git push
.
These are just a few examples of the issues you might encounter when using Git Bash commands. By understanding these common errors and their solutions, you’ll be better equipped to troubleshoot issues and keep your development process running smoothly.
Understanding Git and the Bash Shell
Before we delve deeper into Git Bash commands, let’s take a moment to understand the fundamentals of Git and the Bash shell.
What is Git?
Git is a distributed version control system designed to handle everything from small to very large projects with speed and efficiency. It allows multiple developers to work on a project simultaneously, tracking and merging their changes efficiently.
# Clone a Git repository
git clone https://github.com/username/my_project.git
# Output:
# Cloning into 'my_project'...
# remote: Enumerating objects: 3, done.
# remote: Counting objects: 100% (3/3), done.
# remote: Total 3 (delta 0), reused 3 (delta 0), pack-reused 0
# Unpacking objects: 100% (3/3), done.
In this example, we use the git clone
command to clone a Git repository. This command downloads the repository and its entire version history, allowing you to work on the project locally.
Why is Git Useful for Version Control?
Git is incredibly useful for version control because it provides a detailed history of changes made to a project, making it easy to track progress, identify and fix issues, and coordinate work between multiple developers.
# View the commit history
git log
# Output:
# commit 1a2b3c4d5e6f7g8h9i0j1k2l3m4n5o6p7q8r9s0t
# Author: Your Name <[email protected]>
# Date: Mon Sep 24 00:00:00 2018 -0600
#
# Initial commit
In this example, we use the git log
command to view the commit history. Each commit shows the author, date, and a message describing the changes made.
What is the Bash Shell?
The Bash shell, or simply Bash, is a Unix shell and command language. It’s the default shell for most Unix systems, including Linux and macOS. When we talk about ‘Git Bash’, we’re referring to the application that provides Git command line features on Windows, which also includes Bash command line features.
# Print the current working directory
pwd
# Output:
# /Users/username/my_project
In this example, we use the pwd
command, a common Bash command, to print the current working directory. This shows us where we are in the file system.
How Does Bash Interact with Git?
Bash interacts with Git by providing a command line interface to operate Git’s complex version control features. Through Bash, you can execute Git commands to initialize repositories, track changes, create branches, and more.
Understanding the fundamentals of Git and Bash is crucial to mastering Git Bash commands. With this knowledge, you can leverage the power of Git Bash to streamline your development workflow and collaborate effectively with others.
Expanding Horizons: Git Bash in Larger Projects
Git Bash commands are not just for simple projects. They can be scaled up to manage larger projects and complex workflows. Let’s explore how you can utilize Git Bash commands in broader contexts.
Collaborative Development with Git Bash
Git Bash is an excellent tool for collaborative development. With commands like git branch
, git checkout
, and git merge
, you can work on different features simultaneously, merge changes seamlessly, and resolve conflicts efficiently.
# Create a new branch for a feature
git checkout -b new-feature
# Make changes and commit them
echo 'New feature' >> feature.txt
git commit -a -m 'Add new feature'
# Switch back to the master branch and merge the feature branch
git checkout master
git merge new-feature
# Output:
# Switched to a new branch 'new-feature'
# [new-feature 1a2b3c4] Add new feature
# 1 file changed, 1 insertion(+)
# create mode 100644 feature.txt
# Switched to branch 'master'
# Updating a1b2c3d..e4f5g6h
# Fast-forward
# feature.txt | 1 +
# 1 file changed, 1 insertion(+)
# create mode 100644 feature.txt
In this example, we create a new branch for a feature, make changes and commit them, switch back to the master branch, and merge the feature branch. This workflow allows multiple developers to work on different features without interfering with each other’s work.
Git Bash with Other Command Line Tools
Git Bash can be combined with other command line tools to create powerful development environments. For example, you can use Git Bash with text editors like Vim or Emacs, or with build systems like Make or Ant.
# Open a file in Vim
vim hello.txt
# Make changes and save the file
# :wq
# Add and commit the changes with Git Bash
git commit -a -m 'Edit hello.txt'
# Output:
# [master 1a2b3c4] Edit hello.txt
# 1 file changed, 1 insertion(+), 1 deletion(-)
In this example, we open a file in Vim, make changes and save the file, and then add and commit the changes with Git Bash. This shows how Git Bash can be integrated with other command line tools to streamline your development workflow.
Further Resources for Mastering Git Bash
Want to learn more about Git Bash? Here are some resources that can help you deepen your understanding and hone your skills:
- Pro Git Book – This book, available for free online, is an in-depth guide to Git, covering everything from basics to advanced topics.
- Atlassian Git Tutorial – This comprehensive tutorial from Atlassian covers a wide range of Git topics, including branching and merging, resolving conflicts, and more.
- GeeksforGeeks’ Guide on Working with Git Bash: an introduction to working with Git Bash, including installation and basic Git commands.
Mastering Git Bash commands can significantly enhance your productivity and collaboration in software development. By understanding the basics and exploring advanced techniques, you can leverage the power of Git Bash in your projects.
Wrapping Up: Git Bash Commands
In this comprehensive guide, we’ve navigated through the intricate world of Git Bash commands, providing a roadmap from beginner to expert level usage.
We began with the essentials, exploring basic commands like git init
, git add
, git commit
, and git push
. We then ventured into intermediate territory, discussing branching with git branch
and git checkout
, merging with git merge
, and resolving conflicts. Our journey continued into expert-level techniques, exploring the use of flags and options and the power of combining commands in scripts.
Along the way, we tackled common challenges that you might encounter when using Git Bash, providing solutions to keep your development process running smoothly. We also delved into the fundamentals of Git and the Bash shell, providing a solid foundation for understanding Git Bash commands.
Here’s a quick comparison of the levels we’ve discussed:
Level | Commands | Use Cases |
---|---|---|
Beginner | git init , git add , git commit , git push | Initializing repositories, staging and committing changes |
Intermediate | git branch , git checkout , git merge | Branching, merging, resolving conflicts |
Expert | Flags, options, scripts | Optimizing workflows, automating tasks |
Whether you’re just starting out with Git Bash or you’re looking to level up your command line skills, we hope this guide has given you a deeper understanding of Git Bash commands and their capabilities.
With its balance of simplicity and power, Git Bash is a vital tool for any developer’s toolkit. Now, you’re well equipped to navigate the world of Git Bash commands. Happy coding!