Python ord & chr | 2 Reliable Python ASCII to Char Tools
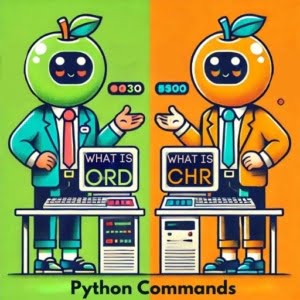
To help with character conversion in our scripts at IOFLOOD, we wondered what is chr and ord in Python, and how could they help? During our testing, we found that these straightforward tools can convert characters to and from their ASCII values. To help our dedicated server hosting customers streamline their data processing needs, we have shared our findings in today’s article.
In this guide, we aim to provide an overview of the ord
Python function and the Python chr
function, their purpose, and practical usages in Python programming.
Stay tuned as we navigate the universe of Unicode characters together!
TL;DR: What are the Python ord and Python chr functions?
Python ord is used with the syntax,
print(ord('A'))
and takes a single character as an argument and returns its Unicode code point. Python chr is used with the syntax,print(chr(65))
and does the reverse of the ord python function.
For Example:
print(ord('A')) # Output: 65
print(chr(65)) # Output: 'A'
Table of Contents
Understanding Python ord and chr
If you’d like to understand Unicode before you dive into the ord() and chr() functions, skip ahead to Unicode: Revolutionizing Character Encoding
One of Python’s key functions for managing Unicode characters is the ord()
function. In this section, we’ll delve into this function and its application in your code.
The Role of ord Python
Python’s ord()
function is a built-in function that accepts a string containing a single Unicode character and returns an integer representing the Unicode point of that character. For instance, ord('A')
would return 65, the Unicode point for the character ‘A’.
print(ord('A')) # Output: 65
Converting Characters with Python ord()
The ord()
function proves incredibly useful when you need to convert characters to their corresponding Unicode points. This comes in handy in several situations, such as comparing characters, implementing encryption algorithms, or working with data in diverse languages.
print(ord('€')) # Output: 8364
print(ord('字')) # Output: 23383
What is ASCII vs What is Unicode
Although the Unicode standard can represent over a million unique characters, the first 128 Unicode points align with the ASCII standard. This means that for characters in the ASCII range (0-127), the ord()
function will return the ASCII value of the character.
print(ord('Z')) # Output: 90
Python ord() Function and Single Characters
As previously mentioned, the ord()
function can only process a single character. If you need to convert a string with multiple characters to Unicode, you would need to iterate over the string and call ord()
for each character.
s = 'Hello'
for char in s:
print(ord(char))
# Output:
# 72
# 101
# 108
# 108
# 111
Next, we’ll explore Python’s chr()
function, the counterpart of ord()
, which converts Unicode points back to characters.
Handling Errors with ord Python
It’s crucial to note that the ord()
function expects a single character. If you pass a string with more than one character or an empty string, Python will raise a TypeError
.
print(ord('AB')) # Output: TypeError: ord() expected a character, but string of length 2 found
print(ord('')) # Output: TypeError: ord() expected a character, but string of length 0 found
Decoding Unicode with Python chr
Having explored Python’s ord()
function, it’s time to shift our focus to its counterpart: the chr()
function. This function performs the inverse operation of ord()
: it accepts a Unicode point (an integer) and returns the corresponding character.
What is chr in Python?
In Python, the chr()
function is a built-in function that accepts an integer as its argument and returns a string representing a character at that Unicode point. For example, chr(65)
would return ‘A’, the character for the Unicode point 65.
print(chr(65)) # Output: 'A'
Conversions with chr Function Python
The chr()
function proves extremely useful when you need to convert Unicode points to their corresponding characters. This can be beneficial in various situations, such as decoding encrypted data or displaying characters from different languages.
print(chr(8364)) # Output: '€'
print(chr(23383)) # Output: '字'
The Scope of Python chr Function
The chr()
function can convert any valid Unicode point to a character. This means it can handle any integer in the range of 0 through 1,114,111, covering all Unicode characters, including language scripts, special symbols, and emojis.
print(chr(128512)) # Output: ''
Error Handling in Python chr
It’s crucial to understand that the chr()
function expects an integer as its argument. If you pass a non-integer or a negative integer, Python will raise a TypeError
or ValueError
respectively. Furthermore, the integer must be a valid Unicode point (0 through 1,114,111).
print(chr('65')) # Output: TypeError: an integer is required (got type str)
print(chr(-1)) # Output: ValueError: chr() arg not in range(0x110000)
Unique Uses of chr Python Function
A unique application of the chr()
function is its ability to convert a list of Unicode points into a Python string. This can be achieved by iterating over the list, calling chr()
for each number, and then joining the results.
numbers = [72, 101, 108, 108, 111]
string = ''.join(chr(num) for num in numbers)
print(string) # Output: 'Hello'
Core Concepts of Python Ord & Chr
Now that we understand Python’s ord()
and chr()
functions, let’s explore the universe of Unicode characters. But what is Unicode, and why is it crucial in today’s computing world?
The Language of Computers: Binary
At its core, a computer is a machine that understands binary – a system of 1s and 0s. Every piece of information processed by a computer, including text, is ultimately converted into binary. This conversion is done through character encoding, which assigns a unique number to each character.
The Chaos Before Unicode
In the early days of computing, multiple character encoding systems existed, each with its unique set of assigned numbers.
ASCII (American Standard Code for Information Interchange) was widely used. However, ASCII could only represent 128 characters, sufficient for English but inadequate for other languages.
This limitation led to various extended ASCII sets, creating a chaotic situation where the same number could represent different characters in different systems.
However limited, ASCII was able to be utilized for a variety of concepts and fun projects.
Unicode: A Universal Solution
Recognizing the need for a universal character encoding system, the Unicode Consortium introduced the Unicode Standard, capable of accommodating characters from different languages, special symbols, and even emojis.
Strings and Unicode in Programming
In programming, strings (a sequence of characters) are fundamental. They represent and manipulate text. With the advent of the Unicode Standard, handling strings became more versatile and inclusive.
Now, a single string can include characters from different languages, special symbols, and emojis, making our programs truly global. For example:
s = 'Hello, 你好, Bonjour, Hola, こんにちは'
print(s)
# Output: Hello, 你好, Bonjour, Hola, こんにちは
The Impact of Unicode
The introduction of Unicode was a game-changer. It brought order to the chaos of multiple character encoding systems. This has not only made programming more inclusive but also opened up new possibilities for digital communication.
Dive Deeper: Python Ord and More
To aid you in expanding your knowledge on Python functions, we’ve compiled a selection of informative resources for your perusal:
- Efficient Use of Python Built-In Functions – Learn about built-in functions, regular expressions and pattern matching.
Minimum Values in Python with min() – Dive into element selection, custom comparisons, and optional default values.
Python range() Usage Simplified – Dive into sequence creation, iteration, and slicing with “range.”
Comprehensive Info on Python chr Function – Understand how to use the chr method in Python through this guide by Reintech.
Effective Type Conversion in Python – Geeks for Geeks dives into the various ways to convert types in Python in this in-depth tutorial.
Consistent Int to Char Conversions – An easy-to-follow guide from TutorialsPoint on the ways to convert an integer to a character.
These additional resources will aid in deepening your understanding of Python functions, making you even more proficient in your Python development journey.
Recap: What is Chr and Python Ord?
As we’ve journeyed through the world of Unicode and Python’s ord()
and chr()
functions, we’ve seen the profound impact they’ve had on the realm of programming. These tools have unlocked a global digital communication experience, enabling our applications to support a vast array of languages, symbols, and emojis.
The ord()
function, with its ability to convert a character into its Unicode point, and the chr()
function, performing the inverse operation, are indispensable tools in any Python programmer’s toolkit. They offer a simple yet effective way to handle text in diverse languages, work with unique symbols, and even create your own emojis!
To learn more about Python, visit this page.
So, the next time you’re working with text in your Python code, remember the power of Unicode and the simplicity of Python’s ord()
and chr()
functions. Embrace the Unicode universe and experience the ease Python brings to your coding journey!