Python Range() Function Guide | Examples, syntax, and advanced uses
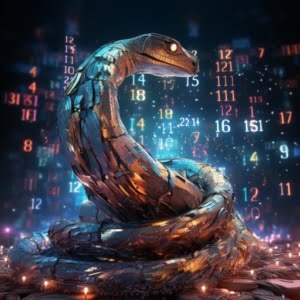
Python’s built-in functions are a powerful aspect of the language, and today, we’re going to explore one of the most versatile among them – the range()
function. This function is like a diligent worker, generating a sequence of numbers within a specified range with ease.
The range()
function is an essential tool whether you’re looping over a sequence or need a specific series of numbers. It embodies Python’s user-friendly design, allowing you to generate diverse number sequences with minimal effort.
TL;DR: What is the range()
function in Python?
The
range()
function is a built-in Python function used to generate a sequence of numbers within a specified range.
In this article, we’re going to delve into the depths of the range()
function, exploring its different uses in Python. So, whether you’re a seasoned Pythonista or just starting your coding journey, buckle up and get ready to enhance your programming prowess with the adaptable and practical range()
function!
Table of Contents
Basics of Python’s range() Function
Starting with the basics, the range()
function in Python is a built-in tool primarily used to generate sequences of numbers. While this may sound simple, the range()
function is a core element in Python, especially when it comes to the common task of sequence generation.
The syntax of the range()
function is quite straightforward. It accepts three parameters – start, stop, and step. Each of these plays a vital role in defining the sequence of numbers that the function generates.
By default, the range()
function returns a sequence of numbers that starts from 0, increments by 1, and stops before a specified number. For instance, range(3)
would generate a sequence of 0, 1, and 2.
range(3)
Beyond sequence generation, the range()
function is also a potent tool for simplifying iteration in Python. It’s often used in ‘for’ loops to iterate a specific number of times. For example, for i in range(3):
would loop three times, showcasing the diverse usage of the range()
function in Python programming.
for i in range(3):
pass
Whether you’re generating a sequence of numbers or controlling the number of loop iterations, the range()
function is a reliable ally. It stands as a testament to the adaptability and practicality of Python’s built-in functions.
Performance
The range
command, from Python 3 onwards, operates as a generator. A Python generator is a special type of function that returns an iterable sequence of items. Instead of returning all items at once, it yields one item at a time, which can be more memory-efficient, espeically for large lists of items.
Exploring Different Initializations of range() Function
Having covered the basics, let’s now explore the various ways you can initialize the range()
function in Python, which truly exemplifies its flexibility and adaptability.
To begin with, you can initialize the range()
function with just one argument. This argument specifies the stop point for the sequence. So, if you were to write range(3)
, Python would interpret this as a sequence that starts at 0 and stops before 3, resulting in a sequence of 0, 1, 2.
range(3)
But what if you want to start your sequence from a number other than 0? This is where initializing the range()
function with two arguments comes into play. The first argument specifies the start point, and the second argument defines the stop point. So, range(1, 4)
would generate a sequence of 1, 2, 3.
range(1, 4)
Taking it a notch higher, you can also initialize the range()
function with three arguments. This allows you to define the start, stop, and step values for the sequence. The step value determines the increment between each number in the sequence. For example, range(1, 10, 2)
would generate a sequence of 1, 3, 5, 7, 9.
range(1, 10, 2)
These different initializations of the range()
function provide immense flexibility in creating tailored number sequences. Whether you need a sequence that starts at a specific number, stops at a specific number, or increments by a specific number, the range()
function is all you need.
But the flexibility doesn’t end there. You can even use the reversed()
function in combination with the range()
function to generate a sequence of numbers in reverse order. So, reversed(range(1, 5))
would give you a sequence of 4, 3, 2, 1, further exhibiting the power of Python’s range()
function.
reversed(range(1, 5))
Initialization | Description | Resulting Sequence |
---|---|---|
range(3) | Sequence starts at 0 and stops before 3 | 0, 1, 2 |
range(1, 4) | Sequence starts at 1 and stops before 4 | 1, 2, 3 |
range(1, 10, 2) | Sequence starts at 1, stops before 10, increments by 2 | 1, 3, 5, 7, 9 |
reversed(range(1, 5)) | Sequence starts at 4 and decrements to 1 | 4, 3, 2, 1 |
Thinking of the range()
function as a conveyor belt in a factory, producing a series of products (numbers) according to specified parameters (start, stop, step) can make the concept more relatable. This analogy encapsulates the function’s ability to deliver a range of outcomes based on the input parameters, much like a production line.
Working with Positive and Negative Steps in range() Function
We’ve already seen how Python’s range()
function can generate sequences with a positive step value. But it’s also capable of handling negative step values, further showcasing its adaptability.
When you define a positive step value, the range()
function increments the sequence. For instance, range(1, 10, 2)
yields a sequence of 1, 3, 5, 7, 9. Here, the sequence starts at 1, stops before 10, and increments by 2.
range(1, 10, 2)
Conversely, a negative step value can be used to decrement the sequence. This means the sequence will start from a higher number and end at a lower number. For example, range(10, 1, -2)
will generate a sequence of 10, 8, 6, 4, 2. In this case, the sequence starts at 10, stops before 1, and decrements by 2.
range(10, 1, -2)
The step value has a significant role in controlling the sequence generation, allowing for a custom interval between generated numbers. This gives you complete control over the number sequences you generate, making the range()
function a potent tool in Python.
Moreover, the range()
function can also be used with the len()
function to dynamically iterate over lists of varying lengths. This means you can use the range()
function to iterate over a list, regardless of its length. For example, for i in range(len(my_list)):
would iterate over each element in the list my_list
, regardless of the number of elements it contains.
my_list = [1, 2, 3, 4, 5]
for i in range(len(my_list)):
pass
Furthermore, the range()
function can generate negative and reverse sequences by accepting negative integer values. This adds another layer of flexibility to this already versatile function. Whether you need to generate a sequence of numbers in ascending, descending, or even reverse order, the range()
function is up to the task!
Further Resources for Python Functions
For those looking to deepen their knowledge of Python functions, we have compiled a list of valuable resources:
- Python Built-In Functions Tips for Productivity – Explore functions for working with iterable objects and comprehensions.
Ordinal Value and Character Conversions in Python – Dive into character-to-integer conversions and Unicode support.
Python round() Function: Rounding Numbers with Precision – Discover Python’s “round” function for rounding numbers.
Python’s Official Documentation on Math Module delves into Python’s math module capabilities.
W3Schools’ Python Functions Reference is a detailed look at Python’s built-in functions and their usage.
Understanding Python Range Function on Coursera explains how the range function is used in Python.
These resources are designed to enrich your understanding of Python functions, taking your coding capabilities to the next level.
Conclusion
The range()
function’s adaptability allows you to generate diverse number sequences, control loop iterations, and even iterate over lists dynamically. Whether you’re generating sequences in ascending, descending, or reverse order, the range()
function stands ready to assist.
But the true strength of the range()
function lies in its practical usage. It’s not just about what it can do, but how it can enhance your scripting capabilities. By understanding and leveraging the functionalities of the range()
function, you can write more efficient, flexible, and powerful code.
Hone your Python skills further by exploring our extensive guide on Python syntax.
And with that, we’ve come to the end of our deep dive into the range()
function. Think of it as a conveyor belt in a factory, producing a series of products (numbers) according to specified parameters (start, stop, step). Just like in a factory, you have the power to control the production line, tailoring it to your needs. Keep exploring, keep coding, and most importantly, have fun while doing it!