What is Python Used For? Popular Use Cases Explained
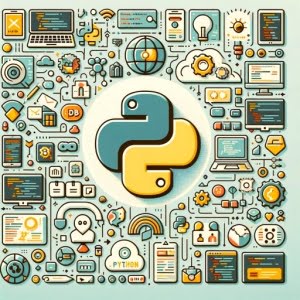
Ever wondered what Python is used for? Like a Swiss Army knife, Python is a versatile tool that can be used for a wide range of tasks.
This article will explore the many uses of Python, from web development to data analysis. Python, a high-level programming language, has become a go-to for many programmers and developers due to its readability and efficiency.
Whether you’re a seasoned developer or a beginner in the world of programming, understanding what Python is used for can open up new avenues for your coding journey.
So, let’s dive in and uncover the power and versatility of Python.
TL;DR: What is Python Used For?
Python is a high-level programming language used for a variety of purposes including web development, data analysis, machine learning, artificial intelligence, automation, and more. It’s known for its simplicity and versatility, making it a popular choice among developers worldwide.
Here’s a simple example of Python code:
print('Hello, Python!')
# Output:
# 'Hello, Python!'
This simple line of code demonstrates Python’s ease of use and readability. It’s just a glimpse of what Python can do. Read on to discover the power and versatility of Python in various fields.
Table of Contents
- Python in Web Development: A Powerful Tool
- Python in Data Analysis: A Reliable Companion
- Python in Machine Learning and AI: A Preferred Choice
- Python in Automation: The Ultimate Tool
- Python vs Other Languages: A Comparative Analysis
- Python: Beyond the Conventional Uses
- Wrapping Up: Python’s Versatility Uncovered
Python in Web Development: A Powerful Tool
Python’s simplicity and readability make it a popular choice in web development. Its vast array of frameworks like Django and Flask enable developers to create sophisticated web applications with ease.
Python Frameworks: Django and Flask
Django, a high-level Python web framework, encourages rapid development and clean, pragmatic design. It takes care of much of the hassle of web development, so you can focus on writing your app without needing to reinvent the wheel. Here’s a simple Django view:
from django.http import HttpResponse
def hello(request):
return HttpResponse("Hello, World!")
# Output:
# When you navigate to the appropriate page in your web browser, you will see 'Hello, World!'
Flask, on the other hand, is a lightweight and more flexible framework that is great for smaller projects and microservices. It is easy to use and lets you get a simple web application up and running in no time:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
# Output:
# When you navigate to the appropriate page in your web browser, you will see 'Hello, World!'
Pros and Cons of Using Python for Web Development
Python’s clear syntax and readability make it a great language for beginners. Its extensive libraries and frameworks save time and effort on project development. However, Python’s execution speed may be slower compared to languages like C or Java. But for most web applications, this difference is negligible and can be outweighed by Python’s advantages in productivity and ease of maintenance.
Python in Data Analysis: A Reliable Companion
Python’s extensive libraries and simplicity make it a popular choice in the realm of data analysis. Libraries like Pandas and NumPy provide powerful tools that simplify the process of manipulating, visualizing, and analyzing data.
Python Libraries: Pandas and NumPy
Pandas, short for ‘Python Data Analysis Library’, is a flexible and powerful tool that provides data structures for efficiently storing, manipulating, and analyzing data. Here’s a simple example of how you can use Pandas to create a data frame and perform operations on it:
import pandas as pd
data = {'Name': ['John', 'Anna', 'Peter'], 'Age': [28, 24, 35]}
df = pd.DataFrame(data)
print(df)
# Output:
# Name Age
# 0 John 28
# 1 Anna 24
# 2 Peter 35
In the above example, we first import the pandas library. We then create a dictionary of data, which we pass to the pd.DataFrame()
function to create a data frame. Finally, we print the data frame, which displays a neatly formatted table of our data.
NumPy, or ‘Numerical Python’, on the other hand, is a library used for numerical computations in Python. It provides a high-performance multidimensional array object and tools for working with these arrays. Here’s an example of creating a NumPy array and performing a mathematical operation on it:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
print(arr * 2)
# Output:
# [ 2 4 6 8 10]
In the above example, we first import the NumPy library. We then create a NumPy array and multiply it by 2, which doubles each element in the array. This showcases NumPy’s ability to perform operations on entire arrays, a feature that is incredibly useful in data analysis.
Python’s role in data analysis extends beyond these libraries, but Pandas and NumPy serve as a solid foundation for any data analyst or scientist working with Python.
Python in Machine Learning and AI: A Preferred Choice
Python’s versatility extends to the fields of machine learning (ML) and artificial intelligence (AI), where it is widely used due to its simplicity and the availability of ML and AI libraries such as TensorFlow and PyTorch.
TensorFlow and PyTorch: Python’s ML and AI Libraries
TensorFlow, developed by Google, is a powerful library for dataflow programming, a type of programming model used across a range of tasks. It’s particularly well-suited for machine learning and deep learning, and it’s capable of running on multiple CPUs and GPUs which makes it valuable for computationally complex applications.
Here’s an example of how you can use TensorFlow to create a simple neural network:
import tensorflow as tf
model = tf.keras.models.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(10)
])
# The model is now ready to be compiled and trained
In the above example, we first import the TensorFlow library. We then create a simple neural network model with one flatten layer and two dense layers.
PyTorch, on the other hand, is a library developed by Facebook’s AI Research lab. It’s known for its simplicity and ease of use, as well as its seamless transition between CPUs and GPUs. PyTorch is also dynamic, meaning that it allows you to change the way your neural network behaves on the fly.
Here’s an example of how you can use PyTorch to create a tensor (a multi-dimensional array):
import torch
x = torch.tensor([1, 2, 3])
print(x)
# Output:
# tensor([1, 2, 3])
In the above example, we first import the PyTorch library. We then create a tensor and print it.
In conclusion, Python’s readability, coupled with its powerful libraries, makes it a preferred choice in the fields of machine learning and AI. Python provides the flexibility and tools to build everything from simple machine learning models to complex neural networks.
Python in Automation: The Ultimate Tool
Python’s simplicity and extensive library support make it a prime choice for automation tasks. From automating mundane tasks like file organization to complex system operations, Python has got you covered.
Python Scripts for Automation
Python’s built-in libraries and third-party modules enable you to automate a wide range of tasks. For instance, you can use the os
and shutil
modules to automate file and directory management tasks. Here’s an example of a simple Python script that renames a file:
import os
os.rename('old_filename.txt', 'new_filename.txt')
# Output:
# The file 'old_filename.txt' will be renamed to 'new_filename.txt'
In the above example, we first import the os
module. We then use the os.rename()
function to rename a file from ‘old_filename.txt’ to ‘new_filename.txt’.
Pros and Cons of Using Python for Automation
Python’s clear syntax and rich library ecosystem make it a great language for automation. It enables developers to write scripts that can automate a variety of tasks, reducing the amount of manual work required. However, like any tool, Python is not without its drawbacks. Its execution speed may be slower compared to languages like C or Java. But for most automation tasks, this difference is negligible and is outweighed by Python’s advantages in ease of use and development speed.
Python vs Other Languages: A Comparative Analysis
Python’s versatility and simplicity make it a popular choice among programmers. However, how does it stack up against other popular programming languages?
Python vs Java
Java, a statically-typed, object-oriented language, is widely used in enterprise-scale applications. It’s known for its speed and scalability. However, Python’s syntax is simpler and more readable. For instance, the classic ‘Hello, World!’ program in Java requires multiple lines of code:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# 'Hello, World!'
In Python, it’s just one line:
print('Hello, World!')
# Output:
# 'Hello, World!'
Python vs JavaScript
JavaScript is primarily used for enhancing web interactivity. It’s the language of the web, running in the browser. Python, on the other hand, is a general-purpose language that can be used for a variety of tasks beyond web development. However, Python’s syntax is simpler and easier to read than JavaScript’s.
Here’s a JavaScript function to compute the factorial of a number:
function factorial(n) {
if (n === 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
console.log(factorial(5));
# Output:
# 120
In Python, the equivalent function is more concise:
def factorial(n):
return 1 if n == 0 else n * factorial(n - 1)
print(factorial(5))
# Output:
# 120
In conclusion, while Python is an excellent language for beginners and for applications such as data analysis, web development, and automation, the choice of language depends on the specific requirements of the project. Each language has its strengths and is best suited to certain types of tasks.
Python: Beyond the Conventional Uses
Python’s versatility extends beyond the common uses we’ve discussed so far. It’s also used in fields like network programming, game development, and even in digital forensics! Python’s simplicity and vast library support make it a tool that can adapt to almost any task.
Python in Network Programming
Python is widely used in network programming due to its simple syntax and socket support. Libraries like Scapy allow for packet manipulation, while frameworks like Twisted support asynchronous network programming.
Python in Game Development
Python isn’t the first language that comes to mind when thinking about game development, but it’s been used in the development of games like Civilization IV. Libraries like Pygame provide modules for game development.
Python in Digital Forensics
Python is used in digital forensics for tasks like creating scripts to automate data analysis. Libraries like Volatility provide frameworks for incident response and digital forensics.
Further Resources for Python Proficiency
If you’re interested in delving deeper into Python, here are some resources to explore:
- The Python Documentation: A comprehensive resource covering all aspects of Python.
- Real Python: Offers Python tutorials, articles, and other resources.
- Python for Beginners: Provides a variety of tutorials and examples for Python beginners.
Remember, Python is a tool, and like any tool, its effectiveness depends on how well you know how to use it. So, keep exploring, keep learning, and you’ll uncover even more uses for Python.
Wrapping Up: Python’s Versatility Uncovered
In this exploration of what is Python used for
, we’ve seen the diverse applications of this versatile language.
From web development with frameworks like Django and Flask to data analysis with libraries such as Pandas and NumPy, Python proves to be a powerful tool. Its role in machine learning and AI with TensorFlow and PyTorch, and its capacity for automation further testify to its utility.
But Python’s versatility doesn’t stop there. It extends to network programming, game development, digital forensics, and beyond. Python’s simplicity, readability, and extensive libraries make it a popular choice among programmers, regardless of their experience level.
Use Case | Python Libraries/Frameworks | Advantages of Python |
---|---|---|
Web Development | Django, Flask | Simplicity, readability, vast array of frameworks |
Data Analysis | Pandas, NumPy | Powerful data manipulation and analysis tools |
Machine Learning & AI | TensorFlow, PyTorch | Extensive ML and AI libraries |
Automation | Built-in and third-party modules | Ease of scripting |
Remember, Python is a tool and its effectiveness depends on how well you know how to use it. Whether you’re a seasoned developer or a beginner in the world of programming, Python has something for everyone. So, keep exploring, keep learning, and you’ll uncover even more uses for Python.