Java Swing: The GUI Widget Toolkit Guide
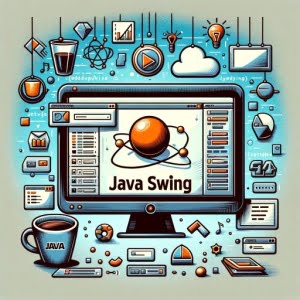
Are you finding it challenging to create GUIs in Java? You’re not alone. Many developers find themselves in a similar situation, but there’s a tool that can make this process a breeze.
Think of Java Swing as a skilled architect – allowing you to construct intuitive and interactive graphical user interfaces. These interfaces can run on any system, even those without Java installed.
This guide will walk you through using Java Swing to create GUIs, from the basics to more advanced techniques. We’ll explore Java Swing’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Java Swing!
TL;DR: How Do I Create a Simple GUI Using Java Swing?
To create a simple GUI in Java, you can use the
JFrame
class, imported withimport javax.swing.JFrame;
. Once imported, you can create a new customized instance by setting the properties, like the size,.setSize([dimensions]);
, and visibility with,.setVisible([true or false]);
. This class provides the basic functionality to create a window on your screen.
Here’s a basic example:
import javax.swing.JFrame;
public class Main {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setSize(400, 400);
frame.setVisible(true);
}
}
// Output:
// A simple window of size 400x400 is displayed on the screen.
In this example, we import the JFrame
class from the javax.swing package
. We then create a new JFrame object, set its size to 400×400 pixels, and make it visible. This results in a simple window being displayed on the screen.
This is just a basic way to use Java Swing to create a GUI, but there’s much more to learn about creating and manipulating GUIs in Java Swing. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Basic Use of Java Swing
Java Swing is a powerful library for creating GUIs in Java. For beginners, understanding the basics is the first step to mastering its use. Let’s start by creating a simple GUI using Swing.
Step 1: Importing Swing Libraries
The first step is to import the necessary Swing libraries into your Java code. For a basic GUI, we need the JFrame class, which is part of the javax.swing package.
import javax.swing.JFrame;
Step 2: Creating a New JFrame
Next, we create a new JFrame object. This represents a window in our GUI.
JFrame frame = new JFrame();
Step 3: Setting the Frame Size
We then set the size of the frame using the setSize
method. This method takes two parameters: the width and the height of the window in pixels.
frame.setSize(600, 600);
Step 4: Making the Frame Visible
Finally, we make the frame visible using the setVisible
method. This method takes a boolean value. When set to true, the frame is displayed.
frame.setVisible(true);
Putting it all together, we have:
import javax.swing.JFrame;
public class Main {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setSize(600, 600);
frame.setVisible(true);
}
}
// Output:
// A simple window of size 600x600 is displayed on the screen.
This is a simple example of using Java Swing to create a GUI. However, a real-world application will have more complex requirements. In the next section, we’ll look at some more advanced uses of Swing.
Advanced Java Swing: Crafting Complex GUIs
As you become more comfortable with Java Swing, you can start to explore more complex GUI elements. Let’s dive into some of these components and how to use them.
Adding Buttons with JButton
Buttons are a staple in GUI design, and Java Swing provides a simple way to add them using the JButton
class.
import javax.swing.JButton;
import javax.swing.JFrame;
public class Main {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setSize(400, 400);
JButton button = new JButton("Click Me!");
frame.add(button);
frame.setVisible(true);
}
}
// Output:
// A window of size 400x400 is displayed with a button labeled 'Click Me!'.
In this example, we create a JButton
object, label it ‘Click Me!’, and add it to our frame. When you run this code, you’ll see a button in the middle of the window.
Creating Menus with JMenuItem
Menus are a common element in GUI applications. Let’s see how to create a simple menu in JFrame:
JMenuBar menuBar = new JMenuBar();
JMenu fileMenu = new JMenu("File");
JMenuItem openItem = new JMenuItem("Open");
fileMenu.add(openItem);
menuBar.add(fileMenu);
frame.setJMenuBar(menuBar);
This block of code creates a menu bar, a ‘File’ menu, and an ‘Open’ menu item. The ‘Open’ item is added to the ‘File’ menu, which is then added to the menu bar. Finally, the menu bar is set as the JFrame’s menu bar.
These are just a few examples of what you can do with JFrame. There are many more features and components to explore, so don’t hesitate to experiment and learn more!
Incorporating Text Fields with JTextField
Text fields are another common GUI component. They allow users to input text. The JTextField
class is used to create text fields in Java Swing.
import javax.swing.JFrame;
import javax.swing.JTextField;
public class Main {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setSize(400, 400);
JTextField textField = new JTextField();
frame.add(textField);
frame.setVisible(true);
}
}
# Output:
# A window of size 400x400 is displayed with an empty text field.
In this code, we create a JTextField object and add it to our frame. This results in a window with an empty text field.
Organizing Components with JPanel
JPanel is a versatile container that can group other components. It’s especially useful when you want to manage multiple components like buttons and text fields.
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class Main {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setSize(400, 400);
JButton button = new JButton("Click Me!");
JTextField textField = new JTextField();
JPanel panel = new JPanel();
panel.add(button);
panel.add(textField);
frame.add(panel);
frame.setVisible(true);
}
}
# Output:
# A window of size 400x400 is displayed with a button and a text field.
In this example, we create a JPanel object, add our button and text field to it, and then add the panel to our frame. This results in a window with a button and a text field.
These are just a few of the many components available in Java Swing. With them, you can create complex, interactive GUIs for your applications.
Exploring Different Layout Managers
Java provides several layout managers, each with its own unique way of arranging components. Let’s take a look at how to use the BorderLayout and GridLayout managers.
JFrame frame = new JFrame();
frame.setLayout(new BorderLayout());
JButton northButton = new JButton("North");
JButton southButton = new JButton("South");
frame.add(northButton, BorderLayout.NORTH);
frame.add(southButton, BorderLayout.SOUTH);
frame.setSize(300, 300);
frame.setVisible(true);
This block of code creates a JFrame and sets its layout manager to BorderLayout. We then create two buttons, one labeled ‘North’ and the other ‘South’. These buttons are added to the north and south regions of the BorderLayout, respectively.
Let’s try the GridLayout:
JFrame frame = new JFrame();
frame.setLayout(new GridLayout(2, 2));
for (int i = 1; i <= 4; i++) {
frame.add(new JButton("Button " + i));
}
frame.setSize(300, 300);
frame.setVisible(true);
In this example, we set the layout manager to GridLayout with 2 rows and 2 columns. We then create four buttons and add them to the JFrame. The GridLayout arranges these buttons in a 2×2 grid.
Exploring Alternatives: JavaFX and AWT
While Java Swing is a powerful tool for creating GUIs, it’s not the only option available. There are other libraries you can use, such as JavaFX and AWT, each with their unique strengths and weaknesses.
JavaFX: The Modern Approach
JavaFX is a modern, feature-rich library that is the successor to Swing. It offers advanced features like CSS styling, 3D graphics, and full-screen capabilities.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Hello World!");
Button btn = new Button();
btn.setText("Say 'Hello World'");
primaryStage.setScene(new Scene(btn, 300, 250));
primaryStage.show();
}
}
# Output:
# A window of size 300x250 is displayed with a button labeled 'Say 'Hello World''.
In this example, we create a simple JavaFX application with a button. Note how JavaFX uses a different structure compared to Swing, with the Application
class and the start
method.
AWT: The Original Java GUI Toolkit
AWT (Abstract Window Toolkit) is the original Java GUI toolkit. It’s less powerful than Swing or JavaFX, but it’s straightforward and doesn’t require any additional libraries.
import java.awt.Frame;
import java.awt.Button;
import java.awt.FlowLayout;
public class Main {
public static void main(String[] args) {
Frame frame = new Frame("Hello World!");
Button btn = new Button("Click me");
frame.setLayout(new FlowLayout());
frame.add(btn);
frame.setSize(300, 300);
frame.setVisible(true);
}
}
# Output:
# A window of size 300x300 is displayed with a button labeled 'Click me'.
In this code, we create a simple AWT application with a button. Note how AWT uses the Frame
class, which is similar to Swing’s JFrame
.
When choosing between Swing, JavaFX, and AWT, consider your application’s requirements and your team’s familiarity with the libraries. While Swing is a powerful and versatile choice, JavaFX and AWT can be more suitable in certain scenarios.
Troubleshooting Java Swing: Common Issues and Solutions
While Java Swing is a powerful tool for creating GUIs, it’s not without its challenges. Let’s discuss some common issues you might encounter when using Swing and how to solve them.
Handling Layout Issues
A common problem when working with Swing is dealing with layout managers. These are used to control how components are arranged in the GUI. However, they can sometimes behave unexpectedly.
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Main {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setSize(400, 400);
JPanel panel = new JPanel();
JButton button1 = new JButton("Button 1");
JButton button2 = new JButton("Button 2");
panel.add(button1);
panel.add(button2);
frame.add(panel);
frame.setVisible(true);
}
}
# Output:
# A window of size 400x400 is displayed with two buttons. However, the buttons are not sized or positioned as expected.
In this example, the buttons might not appear as expected because the JPanel uses a FlowLayout manager by default. To fix this, you can set a different layout manager, such as GridLayout or BorderLayout.
Managing Event Handling
Another common issue is handling events, like button clicks or text input. Swing uses an event-driven programming model, which can be a bit tricky to get the hang of.
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Main {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setSize(400, 400);
JPanel panel = new JPanel();
JButton button = new JButton("Click Me!");
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("Button clicked!");
}
});
panel.add(button);
frame.add(panel);
frame.setVisible(true);
}
}
# Output:
# A window of size 400x400 is displayed with a button. When the button is clicked, 'Button clicked!' is printed to the console.
In this code, we add an ActionListener to our button. When the button is clicked, the actionPerformed method is called, and ‘Button clicked!’ is printed to the console.
These are just a few of the issues you might encounter when working with Java Swing. With a bit of practice and patience, you’ll be able to create complex, interactive GUIs for your applications.
Understanding GUIs and Event-Driven Programming
To fully grasp Java Swing, it’s essential to understand the fundamental concepts it is built upon: GUIs and event-driven programming.
What is a GUI?
GUI, or Graphical User Interface, is a type of user interface that allows users to interact with electronic devices through graphical icons and visual indicators, as opposed to text-based interfaces, typed command labels or text navigation.
# Example of a text-based interface
echo 'Hello, world!'
# Output:
# 'Hello, world!'
In the example above, we use a text-based interface to print ‘Hello, world!’. In a GUI, this could be accomplished by clicking a button.
Event-Driven Programming in Java Swing
Java Swing operates on an event-driven programming model. In this model, the flow of the program is determined by events such as user actions (mouse clicks, key presses), sensor outputs, or messages from other programs.
import javax.swing.JButton;
import javax.swing.JFrame;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Main {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setSize(400, 400);
JButton button = new JButton("Click Me!");
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("Button clicked!");
}
});
frame.add(button);
frame.setVisible(true);
}
}
# Output:
# A window of size 400x400 is displayed with a button. When the button is clicked, 'Button clicked!' is printed to the console.
In the example above, we create a button and add an ActionListener to it. When the button is clicked (an event), the actionPerformed method is called, and ‘Button clicked!’ is printed to the console.
Understanding these concepts is crucial for effectively using Java Swing to create interactive and intuitive GUIs.
Applying Java Swing in Larger Projects
Java Swing’s capabilities extend beyond creating simple GUIs for small-scale projects. It’s also a powerful tool for developing complex, feature-rich interfaces for larger software applications.
Multithreading in GUI Applications
Multithreading is a technique that can significantly improve the responsiveness of your Swing applications. By performing lengthy operations on a separate thread, you can prevent the GUI from becoming unresponsive.
import javax.swing.JButton;
import javax.swing.JFrame;
public class Main {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setSize(400, 400);
JButton button = new JButton("Start lengthy operation");
button.addActionListener(e -> new Thread(this::lengthyOperation).start());
frame.add(button);
frame.setVisible(true);
}
private void lengthyOperation() {
// Simulate a lengthy operation
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
# Output:
# A window of size 400x400 is displayed with a button. When the button is clicked, a lengthy operation is started on a separate thread, preventing the GUI from becoming unresponsive.
In this code, we create a button that starts a lengthy operation on a separate thread when clicked. This prevents the GUI from becoming unresponsive while the operation is in progress.
Network Programming with Swing
Java Swing can also be used in conjunction with Java’s networking capabilities to create networked applications with GUIs.
import javax.swing.JButton;
import javax.swing.JFrame;
import java.io.IOException;
import java.net.Socket;
public class Main {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setSize(400, 400);
JButton button = new JButton("Connect to server");
button.addActionListener(e -> {
try (Socket socket = new Socket("localhost", 1234)) {
System.out.println("Connected to server");
} catch (IOException ex) {
ex.printStackTrace();
}
});
frame.add(button);
frame.setVisible(true);
}
}
# Output:
# A window of size 400x400 is displayed with a button. When the button is clicked, a connection is attempted to a server on localhost:1234.
In this code, we create a button that attempts to connect to a server when clicked. This demonstrates how Swing can be used to create networked applications.
Further Resources for Java Swing
To continue your journey in mastering Java Swing, here are some useful resources:
- Getting Started Java Packages – Learn how packages achieve code reuse and maintenance in Java.
JavaFX GUI Development – Discover how to create interactive desktop and mobile applications with JavaFX.
Exploring Java Bean Usage – Explore the characteristics and usage of JavaBeans in various scenarios.
Oracle’s Java Swing Tutorial – In-depth guide for creating desktop applications with Java Swing.
Java Swing (GUI) Programming Udemy course takes you from novice to adept in Java Swing for GUI platforms.
Java Swing Tutorial by JavaTpoint focusing on core concepts for building graphical user interfaces.
Wrapping Up: Java Swing for GUI Creation
In this comprehensive guide, we’ve delved into the world of Java Swing, a powerful library for creating graphical user interfaces (GUIs) in Java.
We began with the basics, demonstrating how to create a simple GUI using Java Swing. We then explored more advanced uses, such as adding buttons, text fields, and organizing components using JPanel. Along the way, we provided practical code examples to help you understand and apply these concepts.
We also discussed common issues you might encounter when using Java Swing, such as layout management and event handling, and provided solutions to these challenges. Additionally, we looked at alternative libraries for GUI creation in Java, such as JavaFX and AWT, providing you with a broader perspective on Java GUI creation.
Here’s a quick comparison of these libraries:
Library | Complexity | Flexibility | Modernity |
---|---|---|---|
Java Swing | Moderate | High | Moderate |
JavaFX | High | High | High |
AWT | Low | Moderate | Low |
Whether you’re a beginner just starting out with Java Swing or an experienced developer looking to refine your skills, we hope this guide has provided you with a deeper understanding of Java Swing and its capabilities.
With its balance of flexibility and control, Java Swing is a powerful tool for creating interactive and intuitive GUIs in Java. Now, you’re well equipped to harness its power in your projects. Happy coding!