Packages in Java: The Ultimate Guide
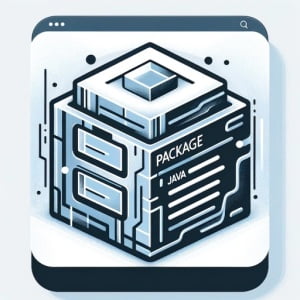
Ever found yourself puzzled over Java packages? You’re not alone. Many developers find the concept of Java packages a bit overwhelming. But, think of a Java package as a filing cabinet for related classes and interfaces. It’s a simple concept that can make your coding life much easier.
Java packages are a powerful way to organize your code, making it easier to manage and maintain. They are especially useful when you are working on large projects with a lot of classes and interfaces.
This guide will walk you through everything you need to know about Java packages, from creating your first package to organizing complex projects. We’ll cover everything from the basics of creating and using packages to more advanced techniques, as well as troubleshooting common issues.
So, let’s dive in and start mastering Java packages!
TL;DR: What is a Java Package and How Do I Use It?
You can use a java package by calling
package packageName
. A package is a namespace that organizes a set of related classes and interfaces. To create a package, you simply need to include a package command in the source file. Here’s a quick example:
package newPackage;
This line of code creates a package named newPackage
. All the classes and interfaces you define in this file will belong to this package.
This is just the tip of the iceberg when it comes to Java packages. Continue reading for a comprehensive guide that will provide a deeper understanding and advanced usage scenarios.
Table of Contents
- Creating Your First Java Package
- Adding Classes to Your Package
- Using Your Package
- Advantages and Potential Pitfalls
- Organizing Packages in Larger Projects
- Using Sub-Packages
- Importing Classes from Other Packages
- The Advent of Java Modules
- Modules vs Packages: When to Use Which?
- Troubleshooting Common Java Package Issues
- Understanding Java’s Namespace Support
- The Significance of Code Organization
- Relevance of Java Packages in Real-World Applications
- Exploring Related Concepts
- Wrapping Up: Mastering Java Packages
Creating Your First Java Package
Creating a Java package is a straightforward process. Let’s start with a simple example. Consider you’re building an application for your company, mycompany
, and the application is named myapp
. Here’s how you can create a package for it:
package com.mycompany.myapp;
This line of code creates a package named com.mycompany.myapp
. Any classes or interfaces that you define in this file will belong to this package.
Adding Classes to Your Package
Once you’ve created a package, you can start adding classes to it. Here’s an example of how to add a HelloWorld
class to your package:
package com.mycompany.myapp;
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
In this example, the HelloWorld
class is part of the com.mycompany.myapp
package. When you run this program, it will print Hello, World!
.
Using Your Package
To use a class from a package, you need to import it first. Here’s how you can import and use the HelloWorld
class from the com.mycompany.myapp
package:
import com.mycompany.myapp.HelloWorld;
public class Test {
public static void main(String[] args) {
HelloWorld.main(new String[0]);
}
}
# Output:
# Hello, World!
In this example, the Test
class imports the HelloWorld
class from the com.mycompany.myapp
package and calls its main
method. The output of this program is Hello, World!
.
Advantages and Potential Pitfalls
Using Java packages has several advantages. It helps you organize your code, avoid naming conflicts, and control access to your classes and interfaces. However, there are also potential pitfalls. For example, if you forget to import a class from a package, you’ll get a compilation error. Also, you need to be careful about access control, as some classes and interfaces may not be accessible from other packages.
Organizing Packages in Larger Projects
As your project grows, organizing your code becomes crucial. Java packages can help you structure your code in a logical and manageable way. For instance, you could have a package for each major feature of your application.
Let’s say you’re building a blogging platform. You might have a package for user management, another for blog post management, and another for comments. Here’s how you might structure your packages:
com.mycompany.myapp.user
com.mycompany.myapp.post
com.mycompany.myapp.comment
Each of these packages would contain the classes and interfaces related to that feature.
Using Sub-Packages
Sub-packages can further refine your code organization. For instance, within the user
package, you might have sub-packages for authentication
, profile
, and settings
:
com.mycompany.myapp.user.authentication
com.mycompany.myapp.user.profile
com.mycompany.myapp.user.settings
This way, you can keep related classes close together, making your code easier to navigate and maintain.
Importing Classes from Other Packages
To use a class from another package, you need to import it. The import
statement allows you to use classes from other packages without having to use their fully qualified names. Here’s an example:
import com.mycompany.myapp.user.User;
public class Test {
public static void main(String[] args) {
User user = new User();
user.sayHello();
}
}
# Output:
# Hello, User!
In this example, the Test
class imports the User
class from the com.mycompany.myapp.user
package and uses it to create a new User
object and call its sayHello
method. The output of this program is Hello, User!
.
Remember, organizing your packages properly and importing classes as needed can significantly improve the maintainability and readability of your code.
The Advent of Java Modules
Java 9 introduced a powerful new feature: modules. A Java module is a mechanism that provides a higher level of code organization than packages. It’s like a container for packages and includes features for encapsulation and dependency management.
Here’s an example of a module descriptor, which is a module-info.java
file at the root of your module:
module com.mycompany.myapp {
requires java.base;
exports com.mycompany.myapp;
}
This descriptor creates a module named com.mycompany.myapp
, requires the java.base
module (which is implicitly required by all modules), and exports the com.mycompany.myapp
package.
Modules vs Packages: When to Use Which?
While packages are suitable for organizing classes and interfaces within a module, modules are designed to organize packages and manage dependencies between them at a higher level.
Consider using modules when you need to:
- Encapsulate internal packages and expose only the public ones.
- Explicitly define dependencies between modules.
On the other hand, continue using packages for organizing classes and interfaces within a module.
Remember, modules are a more advanced feature. If you’re just starting out or working on a small project, packages might be all you need. As your project grows and you start facing challenges with package organization and dependency management, that’s when you should consider using modules.
Troubleshooting Common Java Package Issues
Working with Java packages can sometimes lead to unexpected issues. Let’s discuss some common problems and their solutions.
Resolving Naming Conflicts
One common issue is naming conflicts. This happens when two classes in different packages have the same name. Here’s an example:
import com.mycompany.myapp.ClassA;
import com.othercompany.theirapp.ClassA;
In this case, Java won’t know which ClassA
you’re referring to when you use it in your code. The solution is to use the fully qualified name of the class in your code:
com.mycompany.myapp.ClassA classA = new com.mycompany.myapp.ClassA();
com.othercompany.theirapp.ClassA classB = new com.othercompany.theirapp.ClassA();
Overcoming Access Issues
Another common issue is access issues. This happens when you try to access a class, method, or field that’s not accessible from your current package. Here’s an example:
package com.mycompany.myapp;
class ClassA {
private int fieldA;
}
package com.mycompany.myapp2;
public class ClassB {
ClassA classA = new ClassA();
int fieldB = classA.fieldA; // Compilation error
}
In this case, fieldA
is private to ClassA
and can’t be accessed from ClassB
. The solution is to change the access level of fieldA
or provide a public method in ClassA
to access it.
Remember, understanding the common issues and their solutions can help you work more effectively with Java packages.
Understanding Java’s Namespace Support
Java offers robust support for namespaces, which are essential for organizing and managing your code effectively. A namespace is a container that holds a set of identifiers, or names, to ensure that these names are unique within this container. In Java, packages act as namespaces.
Here’s a simple analogy: think of your codebase as a city, classes and interfaces as buildings, and packages as streets. Streets (packages) group related buildings (classes and interfaces) together and give them unique addresses (names) in the city (codebase). This way, every building (class or interface) can be easily found.
Consider this code block:
package com.mycompany.myapp;
public class MyClass {...}
In this case, MyClass
is the name of the class, com.mycompany.myapp
is the package (or namespace), and com.mycompany.myapp.MyClass
is the fully qualified name of the class.
The Significance of Code Organization
Organizing code is crucial, especially in large projects. Without proper organization, your codebase can become a tangled mess that’s hard to navigate and maintain.
Java packages offer a way to organize your code logically. They group related classes and interfaces together, making your code easier to understand and manage.
For instance, in a blogging platform, you might have packages like com.mycompany.myapp.user
, com.mycompany.myapp.post
, and com.mycompany.myapp.comment
. Each package contains classes and interfaces related to a specific feature of the platform.
By organizing your code into packages, you can easily locate and update your classes and interfaces, making your development process more efficient and your codebase more maintainable.
Relevance of Java Packages in Real-World Applications
Java packages play a crucial role in real-world applications, especially in large projects. They help in logically grouping related classes and interfaces, which makes the codebase easier to navigate and maintain. Whether you’re building a web application, a mobile app, or a desktop software, you’ll likely find yourself using packages to structure your code.
Exploring Related Concepts
Beyond Java packages, there are other important concepts that you might want to explore, such as Java modules and access modifiers. Java modules, introduced in Java 9, provide a higher level of code organization than packages. They allow for encapsulation and dependency management at the module level.
Access modifiers, on the other hand, control the visibility of classes, methods, and variables. They play a crucial role in object-oriented programming and are closely tied to the concept of packages.
Further Resources for Mastering Java Packages
For those who wish to delve deeper into the world of Java packages, here are some helpful resources:
- Java Import Statements Reference explains accessing external classes and packages with import statements .
Jython Overview – Discover Jython, an implementation of the Python programming language written in Java.
Maven in Java Overview – Understand Maven, a build automation tool and project management tool for Java.
Oracle’s Java Tutorials provides info on how to create and manage Java packages.
Baeldung’s Guide on Java Packages provides a detailed explanation of Java packages.
GeeksforGeeks Java Packages offers an overview of Java packages, their advantages, and sub-packages.
These resources should give you a solid foundation in understanding and using Java packages, and help you take your Java programming skills to the next level.
Wrapping Up: Mastering Java Packages
In this comprehensive guide, we’ve delved deep into the world of Java packages, an essential tool for organizing and managing your code effectively. We’ve covered everything from the basics of creating and using packages to more advanced techniques, as well as troubleshooting common issues.
We began with the basics, learning how to create a Java package, how to add classes and interfaces to it, and how to use the package in other parts of your code. We then moved on to more advanced topics, discussing how to organize packages in larger projects, how to use sub-packages, and how to use the import statement to use classes from other packages.
We also explored alternative approaches, introducing the concept of Java modules introduced in Java 9 as an advanced way to organize code. We discussed when to use modules vs packages and provided examples and analysis for each. Finally, we tackled common issues one may encounter with Java packages, such as naming conflicts and access issues, offering solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Java Packages | Organizes code, avoids naming conflicts, controls access | May require troubleshooting for some programs |
Java Modules | Higher level of code organization, manages dependencies | More advanced, might be overkill for small projects |
Whether you’re just starting out with Java packages or you’re looking to level up your code organization skills, we hope this guide has given you a deeper understanding of Java packages and their capabilities.
With its balance of simplicity and power, Java packages are a fundamental tool for any Java developer. Happy coding!