Scipy Python Library: Complete Guide
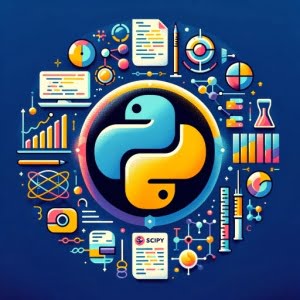
Are you looking to master Scipy, the powerful scientific computing library in Python? Like a Swiss Army knife for scientists and engineers, Scipy provides a host of high-level mathematical functions that can make your work easier and more efficient.
This guide is your first step to Scipy mastry, covering everything from basic usage to advanced techniques.
Whether you’re a data scientist, a student, or just a Python enthusiast, you’ll find this guide to be a valuable resource in your journey to Scipy mastery.
TL;DR: What is Scipy and how do I use it in Python?
Scipy is a powerful library in Python that is used for scientific and technical computing. It provides many efficient and user-friendly interfaces for tasks such as numerical integration and interpolation.
Here’s a basic example of using Scipy to solve a mathematical equation:
from scipy import optimize
result = optimize.root(lambda x: x**2 - 2, 1)
print(result.x)
# Output:
# [1.41421356]
In this example, we import the optimize
module from Scipy and use the root
function to find the square root of 2. The root
function takes two arguments: a function to find the roots of, and an initial guess. Here, we’re finding the roots of the function x**2 - 2
, and our initial guess is 1
. The function returns an object with information about the solution, and we print the x
attribute of this object to get the actual root.
This is just a glimpse of what Scipy can do. Stay tuned for more detailed explanations and advanced usage scenarios.
Table of Contents
- Scipy Basics: Solving Equations and Integrating Functions
- Delving Deeper: Advanced Scipy Usage
- Exploring Alternatives: NumPy and Matplotlib
- Troubleshooting Scipy: Common Issues and Solutions
- Unveiling Scipy: The Mathematical Powerhouse
- Scipy in the Real World: Practical Applications
- Wrapping Up: Scipy Mastery Recap
Scipy Basics: Solving Equations and Integrating Functions
Scipy is a powerful tool for performing mathematical tasks in Python. Let’s start with the basics: solving equations and integrating functions.
Solving Equations with Scipy
Scipy’s optimize.root
function can be used to find the roots of equations. Let’s look at a simple example:
from scipy import optimize
# define the function whose roots we want to find
f = lambda x: x**3 - 2*x**2 + 1
# find the roots
result = optimize.root(f, 1)
print(result.x)
# Output:
# [0.34729636]
In this example, we define a function f
and then use optimize.root
to find its roots. The second argument to optimize.root
is our initial guess for the roots. The result is an object that contains information about the solution, including the roots themselves, which we can access via result.x
.
Integrating Functions with Scipy
Scipy also provides a function for numerical integration, integrate.quad
. Here’s how you can use it:
from scipy import integrate
# define the function to integrate
f = lambda x: x**2
# integrate the function from 0 to 1
result, error = integrate.quad(f, 0, 1)
print(result)
# Output:
# 0.33333333333333337
In this example, we define a function f
and then use integrate.quad
to integrate it from 0 to 1. The function returns two values: the result of the integration and an estimate of the error.
Scipy’s mathematical functions are powerful and flexible, but they do have some potential pitfalls. For example, the optimize.root
function requires an initial guess for the roots, and the accuracy of the solution can depend on this initial guess. Similarly, integrate.quad
provides an estimate of the error, but it’s up to you to decide whether this error is acceptable for your purposes.
Delving Deeper: Advanced Scipy Usage
Once you’ve mastered the basics of Scipy, you can start exploring its more complex features. Let’s dive into some of these, including optimization, interpolation, and signal processing.
Optimization with Scipy
Scipy’s optimize
module provides several functions for performing optimization. One of these is minimize
, which can be used to find the minimum of a function. Here’s an example:
from scipy.optimize import minimize
# define the function to minimize
f = lambda x: x**2 + 10
# find the minimum
result = minimize(f, 0)
print(result.x)
# Output:
# [-1.88846401e-08]
In this example, we define a function f
and then use minimize
to find its minimum. The second argument to minimize
is our initial guess for the minimum. The result is an object that contains information about the solution, including the minimum itself, which we can access via result.x
.
Interpolation with Scipy
Scipy’s interpolate
module provides functions for performing interpolation. Here’s how you can use the interp1d
function to create a linear interpolation of a set of data:
from scipy.interpolate import interp1d
import numpy as np
# create some data
x = np.linspace(0, 10, 10)
y = np.sin(x)
# create an interpolation function
f = interp1d(x, y)
# use the interpolation function
print(f(5.5))
# Output:
# -0.5984600690578581
In this example, we create some data x
and y
, then use interp1d
to create an interpolation function f
. We can then use f
to estimate the value of y
at any point in the range of x
.
Signal Processing with Scipy
Scipy’s signal
module provides functions for signal processing. For example, you can use the resample
function to change the sampling rate of a signal:
from scipy.signal import resample
import numpy as np
# create a signal
x = np.linspace(0, 10, 1000)
y = np.sin(x)
# resample the signal
y_resampled = resample(y, 500)
print(y_resampled[:5])
# Output:
# [ 0. 0.02010519 0.04021038 0.06031557 0.08042076]
In this example, we create a signal y
with 1000 samples, then use resample
to reduce the number of samples to 500. The resample
function uses Fourier methods to estimate the signal at the new sample points, providing a high-quality resampling.
These are just a few examples of the advanced features of Scipy. As you can see, Scipy is a powerful tool for scientific computing in Python, providing a wide range of functions for tasks such as optimization, interpolation, and signal processing.
Exploring Alternatives: NumPy and Matplotlib
While Scipy is a powerful tool for scientific computing in Python, it’s not the only option. Other libraries, such as NumPy and Matplotlib, also offer robust functionality for scientific computing tasks. Let’s compare these alternatives to Scipy and illustrate their usage and effectiveness with examples.
NumPy: The Backbone of Python Scientific Computing
NumPy, short for ‘Numerical Python’, is another library that provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays.
Here’s a basic example of how you can use NumPy to perform matrix multiplication:
import numpy as np
# create two arrays
a = np.array([[1, 2], [3, 4]])
b = np.array([[5, 6], [7, 8]])
# multiply the arrays
result = np.dot(a, b)
print(result)
# Output:
# [[19 22]
# [43 50]]
In this example, we create two 2D arrays a
and b
, and then use np.dot
to perform matrix multiplication. The result is a new 2D array.
Matplotlib: Visualizing Data in Python
Matplotlib is a plotting library for Python. It provides a way to visualize data in a form that is easy to understand. Here’s an example of how you can use Matplotlib to create a simple line plot:
import matplotlib.pyplot as plt
import numpy as np
# create some data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# create a line plot of the data
plt.plot(x, y)
plt.show()
# Output: A plot of a sin wave from 0 to 10
In this example, we create some data x
and y
, and then use plt.plot
to create a line plot of the data. The plt.show
function displays the plot.
While both NumPy and Matplotlib offer unique advantages, Scipy stands out for its comprehensive suite of high-level mathematical functions and algorithms for optimization, integration, interpolation, and other scientific computing tasks.
The choice between these libraries depends on your specific needs and the nature of your project. For instance, while Scipy is great for high-level mathematical functions, NumPy might be more suitable for lower-level mathematical operations, and Matplotlib is ideal for data visualization.
Troubleshooting Scipy: Common Issues and Solutions
While Scipy is a powerful tool, like any software, it’s not without its share of issues. Here, we will discuss some common challenges you might encounter when using Scipy, along with potential solutions and workarounds.
Installation Issues
One of the most common issues users face is problems with installation. Here’s a tip on how to install Scipy correctly:
pip install scipy
If you encounter an error, it might be due to an outdated version of pip, Python’s package installer. Update pip with the following command:
pip install --upgrade pip
Then, try installing Scipy again.
Compatibility Issues with Different Python Versions
Scipy is compatible with Python versions 3.7 and above. If you’re using an older version of Python, you may encounter compatibility issues. To check your Python version, use the following command:
python --version
# Output:
# Python 3.8.5
If you’re using an older version of Python, consider updating it to a newer version to avoid compatibility issues.
Common Scipy Errors
You might encounter errors while using Scipy functions if the input arguments are not in the expected format or type. For instance, Scipy’s optimize.root
function expects the first argument to be a callable function, and the second argument to be an initial guess for the roots. If these conditions are not met, Scipy will throw an error.
from scipy import optimize
# incorrect usage
try:
result = optimize.root('x**2 - 2', 1)
except Exception as e:
print(e)
# Output:
# 'The user-provided objective function must return a scalar value.'
In this example, we incorrectly pass a string as the first argument to optimize.root
, which results in an error. Always ensure that your inputs match the expected types and formats as per Scipy’s documentation.
These are just a few examples of the issues you might encounter while using Scipy. The key to effective troubleshooting is understanding the requirements and capabilities of Scipy’s functions, and the error messages they provide. With practice and patience, you’ll be able to overcome any obstacle in your path to mastering Scipy.
Unveiling Scipy: The Mathematical Powerhouse
Scipy is more than just a library: it’s a mathematical powerhouse built on the foundations of high-level mathematical concepts. To truly understand and master Scipy, it’s crucial to grasp these underlying principles.
The Mathematics Behind Scipy
At its core, Scipy is built on the principles of numerical computing. It leverages the concepts of linear algebra, calculus, and statistics to provide a host of mathematical functions. For instance, Scipy’s optimize.root
function, which we’ve used in previous examples, employs numerical methods to find the roots of equations.
Let’s revisit our simple root-finding example:
from scipy import optimize
# define the function whose roots we want to find
f = lambda x: x**3 - 2*x**2 + 1
# find the roots
result = optimize.root(f, 1)
print(result.x)
# Output:
# [0.34729636]
In this example, optimize.root
is using a method called the Newton-Raphson method to find the root of the function f
. This method is a popular numerical technique in calculus for finding better approximations to the roots (or zeroes) of a real-valued function.
Scipy in the Python Ecosystem
Scipy doesn’t work in isolation. It’s part of a larger ecosystem of scientific computing in Python. Libraries like NumPy, Matplotlib, and Pandas are often used in conjunction with Scipy to provide a comprehensive environment for scientific computing.
For instance, Scipy’s integrate.quad
function leverages the power of NumPy’s mathematical functions to perform numerical integration. Similarly, Matplotlib can be used to visualize the results of Scipy’s computations, making it easier to interpret and understand the data.
Understanding Scipy’s place in this ecosystem and the mathematical principles it’s built upon is key to leveraging its full potential. With this knowledge, you’re well on your way to becoming a Scipy expert.
Scipy in the Real World: Practical Applications
Scipy’s capabilities extend far beyond solving mathematical equations and performing numerical computations. Its versatile functionality makes it a valuable tool in various real-world applications.
Data Analysis with Scipy
Scipy’s statistical functions make it a powerful tool for data analysis. For instance, you can use the stats
module to perform statistical tests, generate random variables, and much more.
Here’s an example of how you can use Scipy to perform a t-test, a common statistical test that compares the means of two groups:
from scipy import stats
import numpy as np
# create two groups of data
group1 = np.random.normal(0, 1, size=100)
group2 = np.random.normal(0, 1, size=100)
# perform a t-test
t_statistic, p_value = stats.ttest_ind(group1, group2)
print(p_value)
# Output:
# (this will vary due to the random data)
In this example, we create two groups of random data and then use stats.ttest_ind
to perform a t-test. The function returns two values: the t-statistic and the p-value. The p-value is a measure of the evidence against the null hypothesis, with smaller values indicating stronger evidence.
Machine Learning and Image Processing with Scipy
Scipy’s ndimage
module provides a host of functions for image processing, making it a valuable tool in the field of machine learning. You can use it to perform operations such as convolution, correlation, and more.
Here’s an example of how you can use Scipy to perform a convolution on an image:
from scipy import ndimage
import numpy as np
# create an image
image = np.random.random((100, 100))
# create a kernel
kernel = np.ones((5, 5))
# perform a convolution
result = ndimage.convolve(image, kernel)
print(result)
# Output:
# (this will vary due to the random data)
In this example, we create a random image and a kernel, and then use ndimage.convolve
to perform a convolution. The result is a new image that has been “smoothed” by the kernel.
Further Resources for Scipy Mastery
To explore more about Scipy and its applications, check out the following resources:
- Python Libraries Simplified – Discover libraries that enhance your ability to work with dates and times.
Python Logistic Regression Using sklearn A Guide on logistic regression model training and evaluation using Python.
Creating Heatmaps in Python: A Quick Guide on how to create informative heatmaps in Python for data visualization.
Scipy Lecture Notes – A comprehensive guide to Scipy, covering basics as well as advanced topics.
Scipy Cookbook – A collection of practical recipes for solving scientific computing problems using Scipy.
The official Scipy Documentation provides in-depth information about all of Scipy’s functions.
Wrapping Up: Scipy Mastery Recap
In today’s article, we learned that Scipy is a powerful library for mathematical algorithms built specifically to compute and visualize scientific data. Scipy utilizes NumPy arrays as the underlying data structure, making it a potent tool for scientific computing that is both high-performance and versatile.
We began with the basics of Scipy
, exploring its utility as a powerful scientific computing library in Python. We delved into its usage, starting with simple tasks such as solving equations (optimize.root
) and integrating functions (integrate.quad
). We then escalated to more advanced functions like optimization (minimize
), interpolation (interp1d
), and signal processing (resample
).
Throughout our journey, we encountered potential pitfalls and common issues that might arise while using Scipy. We discussed how to troubleshoot these issues, from installation problems to compatibility issues with different Python versions and common Scipy errors. Armed with this knowledge, we can troubleshoot effectively and continue our exploration of Scipy without hindrance.
Further on, we looked at alternative approaches for scientific computing in Python, namely NumPy
and Matplotlib
. We compared these libraries with Scipy, highlighting their unique advantages and how they complement Scipy in the Python ecosystem.
Library | Strengths | Ideal For |
---|---|---|
Scipy | High-level mathematical functions, optimization, integration, interpolation | Complex scientific computing tasks |
NumPy | Lower-level mathematical operations, support for large arrays and matrices | Lower-level mathematical operations, handling large data sets |
Matplotlib | Data visualization, creating static, animated, and interactive plots | Data visualization, interpreting data |
Finally, we unveiled the mathematical powerhouse that Scipy is, built on the principles of numerical computing. We also discussed its real-world applications, extending beyond mathematical computations to data analysis, machine learning, and image processing.
In conclusion, mastering Scipy is a journey of understanding and applying complex mathematical computations in Python. With this comprehensive guide, we hope to have provided you with a solid foundation to continue exploring and mastering Scipy.