Master Sklearn Logistic Regression: Step-by-Step Guide
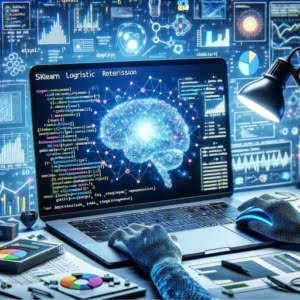
Are you finding it challenging to implement logistic regression with sklearn in Python? You’re not alone. Many developers find this task daunting, but sklearn, like a skilled craftsman, provides you with the tools to build a logistic regression model with ease.
Whether you’re predicting customer churn, diagnosing diseases, or solving a myriad of other classification problems, understanding how to implement logistic regression in sklearn can significantly streamline your machine learning projects.
In this guide, we’ll walk you through the process of implementing logistic regression with sklearn, from the basics to more advanced techniques. We’ll cover everything from the LogisticRegression
class, different solvers, regularization techniques, as well as alternative approaches.
So, whether you’re a beginner just starting out or an experienced data scientist looking to brush up your skills, there’s something in this guide for you.
Let’s dive in and start mastering sklearn logistic regression!
TL;DR: How Do I Implement Logistic Regression with Sklearn?
To implement logistic regression with sklearn, you use the
LogisticRegression
class from the sklearn.linear_model module. Here’s a simple example:
from sklearn.linear_model import LogisticRegression
# Create a Logistic Regression model
model = LogisticRegression()
# Fit the model with training data
model.fit(X_train, y_train)
# Output:
# LogisticRegression()
In this example, we first import the LogisticRegression
class from the sklearn.linear_model module. Then, we create an instance of the LogisticRegression
class. Finally, we fit the model with the training data using the fit
method. The output shows that a LogisticRegression model has been successfully created and fitted with the training data.
This is a basic way to implement logistic regression with sklearn, but there’s much more to learn about fine-tuning your model and handling more complex scenarios. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Sklearn Logistic Regression: The Basics
- Advanced Logistic Regression with Sklearn
- Exploring Alternative Approaches to Logistic Regression
- Navigating Common Hurdles in Sklearn Logistic Regression
- Unpacking Logistic Regression and Sklearn
- Expanding Your Sklearn Logistic Regression Skills
- Wrapping Up: Mastering Sklearn Logistic Regression
Sklearn Logistic Regression: The Basics
The LogisticRegression
class in sklearn.linear_model is the starting point for implementing logistic regression. Let’s take a closer look at how to use it to fit a model.
Creating and Fitting a Logistic Regression Model
The first step is to import the LogisticRegression
class from the sklearn.linear_model module. You then create an instance of this class, which represents your logistic regression model. Here’s a simple example:
from sklearn.linear_model import LogisticRegression
# Create a Logistic Regression model
model = LogisticRegression()
# Output:
# LogisticRegression()
In this code block, we have successfully imported the LogisticRegression
class and created an instance of it. This instance, model
, is our logistic regression model.
The next step is to fit the model with your training data. This is done using the fit
method. Here’s how:
# Assume X_train and y_train are your training data and target values
# Fit the model with training data
model.fit(X_train, y_train)
# Output:
# LogisticRegression()
In this block, we call the fit
method on our model
instance, passing in our training data X_train
and target values y_train
. The fit
method adjusts the model’s parameters to best predict the target values from the training data.
Advantages and Potential Pitfalls
Using the LogisticRegression
class in sklearn has several advantages. It’s simple and straightforward, making it a great starting point for beginners. It also includes several options for customization, such as different solvers and regularization techniques, which we’ll explore later.
However, as with any tool, there are potential pitfalls. One common issue is failing to properly prepare your data before fitting the model. For example, sklearn’s LogisticRegression
expects numerical input, so you’ll need to convert any categorical data into numerical form before fitting your model. Furthermore, it’s important to handle missing data appropriately, as the fit
method cannot handle missing values.
Stay tuned for our discussion on troubleshooting, where we’ll delve into these issues and their solutions in more detail.
Advanced Logistic Regression with Sklearn
As you become more comfortable with the basics of sklearn’s logistic regression, you can start exploring its more advanced features. Let’s dive into some of them.
Different Solvers
The LogisticRegression
class in sklearn allows you to choose from various solvers. A solver is the algorithm that sklearn uses to find the model parameters that minimize the cost function. Sklearn offers several solvers, including ‘newton-cg’, ‘lbfgs’, ‘liblinear’, ‘sag’, and ‘saga’. Here’s an example of using the ‘saga’ solver:
from sklearn.linear_model import LogisticRegression
# Create a Logistic Regression model with the 'saga' solver
model = LogisticRegression(solver='saga')
model.fit(X_train, y_train)
# Output:
# LogisticRegression(solver='saga')
In this code block, we specify the solver as ‘saga’ when creating our logistic regression model. The ‘saga’ solver is a variant of the ‘sag’ solver that also supports the ‘elasticnet’ penalty, and thus is suitable for large datasets.
Regularization Techniques
Regularization is a technique used to prevent overfitting by adding a penalty term to the loss function. Sklearn’s LogisticRegression
supports two types of regularization: L1 and L2. The type of regularization to use is specified using the ‘penalty’ parameter. Here’s an example of using L1 regularization:
from sklearn.linear_model import LogisticRegression
# Create a Logistic Regression model with L1 regularization
model = LogisticRegression(penalty='l1', solver='saga')
model.fit(X_train, y_train)
# Output:
# LogisticRegression(penalty='l1', solver='saga')
In this example, we specify the penalty as ‘l1’ to use L1 regularization. Note that not all solvers support all regularization types. In this case, the ‘saga’ solver supports both ‘l1’ and ‘l2’ penalties.
By taking advantage of these advanced features, you can fine-tune your logistic regression models to better fit your specific use case and improve their performance.
Exploring Alternative Approaches to Logistic Regression
While sklearn’s LogisticRegression
class is a powerful tool for implementing logistic regression, it’s not the only game in town. There are other classes within sklearn, as well as other Python libraries, that offer alternative ways to implement logistic regression. Let’s look at a couple of these alternatives.
SGDClassifier: An Alternative Within Sklearn
The SGDClassifier
class in sklearn.linear_model is another way to implement logistic regression. This class uses stochastic gradient descent (SGD) to find the model parameters that minimize the cost function. Here’s an example:
from sklearn.linear_model import SGDClassifier
# Create a SGDClassifier model with the 'log' loss function
model = SGDClassifier(loss='log')
model.fit(X_train, y_train)
# Output:
# SGDClassifier(loss='log')
In this code block, we create an instance of the SGDClassifier
class, specifying the loss function as ‘log’ to implement logistic regression. The SGDClassifier
class can be more efficient than LogisticRegression
for large datasets, but it may also be more sensitive to the choice of learning rate and may require more iterations to converge.
Statsmodels: An Alternative Library
Statsmodels is another Python library that you can use to implement logistic regression. It offers more statistical information about your model than sklearn, which can be useful for understanding your model in depth. Here’s an example:
import statsmodels.api as sm
# Add a constant to the training data
X_train = sm.add_constant(X_train)
# Create a logistic regression model and fit it
model = sm.Logit(y_train, X_train)
result = model.fit()
# Output:
# <statsmodels.discrete.discrete_model.BinaryResultsWrapper object at 0x...>
In this example, we use the Logit
class in statsmodels to implement logistic regression. Note that statsmodels does not add a constant to your data by default, so we do this manually using the add_constant
function. The fit
method returns a BinaryResultsWrapper
object that contains a wealth of statistical information about your model.
When deciding which approach to use, consider the size of your dataset, the computational resources available, and the level of statistical information you need about your model. Remember, the best tool is the one that suits your specific needs!
As you work with sklearn’s logistic regression, you might encounter some common issues. Let’s discuss these potential problems and how to address them.
Convergence Warnings
One common issue you might run into is a convergence warning. This warning is raised when the solver fails to find the optimal parameters within the given number of iterations. Here’s an example of what this might look like:
from sklearn.linear_model import LogisticRegression
# Create a Logistic Regression model
model = LogisticRegression(max_iter=10)
# Try to fit the model with training data
model.fit(X_train, y_train)
# Output:
# ConvergenceWarning: lbfgs failed to converge (status=1):
# STOP: TOTAL NO. of ITERATIONS REACHED LIMIT.
In this example, we set the maximum number of iterations to 10, which is not enough for the solver to converge. The output shows a ConvergenceWarning
.
The simplest solution to this issue is to increase the maximum number of iterations by setting the max_iter
parameter to a higher value. However, be aware that increasing max_iter
may increase the computation time.
Issues with Data Scaling
Another common issue is related to data scaling. Sklearn’s logistic regression works best when all features are on a similar scale. If your features have different scales, the solver might take longer to converge, or it might not converge at all. Here’s an example of how to scale your data using sklearn’s StandardScaler
:
from sklearn.preprocessing import StandardScaler
# Create a StandardScaler
scaler = StandardScaler()
# Fit the scaler and transform the training data
X_train_scaled = scaler.fit_transform(X_train)
# Now you can fit the model with the scaled data
model.fit(X_train_scaled, y_train)
# Output:
# LogisticRegression()
In this example, we first create a StandardScaler
, which standardizes features by removing the mean and scaling to unit variance. We then fit the scaler with our training data and transform the data. Finally, we fit the model with the scaled data.
By being aware of these common issues and knowing how to handle them, you can ensure a smoother journey in your sklearn logistic regression endeavors.
Unpacking Logistic Regression and Sklearn
To fully grasp the implementation of logistic regression with sklearn, it’s essential to understand the core concepts behind logistic regression and the sklearn library.
Logistic Regression: A Quick Recap
Logistic regression is a statistical model used for binary classification problems – where the outcome can be one of two possible categories. It uses the logistic function to model the probability of the ‘positive’ class. The logistic function, also known as the sigmoid function, takes any real-valued number and maps it into a value between 0 and 1.
Here’s a simple visualization of the sigmoid function using Python and matplotlib:
import numpy as np
import matplotlib.pyplot as plt
# Define the sigmoid function
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# Generate a range of values from -10 to 10
x = np.linspace(-10, 10, 100)
# Apply the sigmoid function
y = sigmoid(x)
# Plot the result
plt.plot(x, y)
plt.title('Sigmoid Function')
plt.show()
# Output:
# A plot of the sigmoid function, showing how it maps any real-valued number into a value between 0 and 1.
Sklearn: Your Machine Learning Toolkit
Sklearn, or Scikit-learn, is a popular Python library for machine learning. It provides a range of supervised and unsupervised learning algorithms, as well as tools for model fitting, data preprocessing, model evaluation, and many other utilities.
Sklearn’s LogisticRegression
class is part of its linear_model module, which contains methods for regression, ridge regression, Lasso, and other linear models.
Solvers and Regularization: The Inner Workings
As we’ve discussed, sklearn’s LogisticRegression
class allows you to choose from various solvers. Each solver uses a different algorithm to find the model parameters that minimize the cost function. The choice of solver can impact the speed of convergence and the accuracy of the model, especially for large datasets.
Regularization is another key concept in logistic regression. It’s a technique used to prevent overfitting by adding a penalty to the loss function. Sklearn supports L1 and L2 regularization, which can be specified using the ‘penalty’ parameter.
By understanding these fundamentals, you’ll be better equipped to implement logistic regression with sklearn and troubleshoot any issues that arise.
Expanding Your Sklearn Logistic Regression Skills
Understanding how to implement logistic regression with sklearn is a crucial skill in machine learning and data science. However, the journey doesn’t end here. There are related concepts and tools that can further enhance your skill set.
Exploring Other Types of Regression
While logistic regression is used for binary classification problems, there are other types of regression for different kinds of problems. For instance, linear regression is used for predicting a continuous outcome, while multinomial logistic regression is used when the outcome can be one of more than two categories.
Diving Into Model Evaluation
Once you’ve built your logistic regression model, it’s crucial to evaluate its performance. Sklearn provides several tools for this, such as the accuracy_score
function for calculating the accuracy of your model, and the confusion_matrix
function for understanding the types of errors your model is making.
from sklearn.metrics import accuracy_score, confusion_matrix
# Assume y_test are the true target values and y_pred are the predicted values
# Calculate accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
# Calculate confusion matrix
conf_mat = confusion_matrix(y_test, y_pred)
print(f'Confusion matrix:
{conf_mat}')
# Output:
# Accuracy: 0.85
# Confusion matrix:
# [[50 10]
# [ 5 35]]
In this example, we first calculate the accuracy of our model, which is the proportion of correct predictions. Then, we calculate the confusion matrix, which shows the number of true positives, true negatives, false positives, and false negatives.
Further Resources for Mastering Sklearn Logistic Regression
If you’re eager to continue your journey in mastering sklearn logistic regression, here are some resources that can help:
- Python Libraries Fundamentals Unveiled – Learn how to access and utilize Python libraries for network programming.
Sklearn in Python Made Easy – Learn how to perform linear regression analysis in Python for data insights.
Scientific Analysis with SciPy Library – Learn how to solve complex scientific and engineering problems with SciPy.
Sklearn’s official documentation provides a thorough explanation of the
LogisticRegression
class and its methods.Python Data Science Handbook – This online book by Jake VanderPlas covers logistic regression and more.
Machine Learning Mastery – This website offers a wealth of articles on machine learning topics and logistic regression.
Remember, the key to mastering any skill is practice. Don’t be afraid to experiment with different settings and techniques, and always keep learning!
Wrapping Up: Mastering Sklearn Logistic Regression
In this comprehensive guide, we’ve delved deep into the world of logistic regression using sklearn, a powerful library for machine learning in Python.
We began with the basics, learning how to create and fit a logistic regression model using the LogisticRegression
class. We then ventured into more advanced territory, exploring different solvers and regularization techniques, and how to handle common issues such as convergence warnings and data scaling.
Along the way, we also looked at alternative approaches to logistic regression, including the SGDClassifier
class within sklearn and the Logit
class in the statsmodels library. Each of these approaches has its strengths and weaknesses, and the best choice depends on your specific needs and circumstances.
Here’s a quick comparison of these approaches:
Method | Pros | Cons |
---|---|---|
LogisticRegression | Robust, supports many solvers and regularization techniques | May require data scaling, can give convergence warnings |
SGDClassifier | Efficient for large datasets | Sensitive to learning rate, may require more iterations to converge |
Logit (statsmodels) | Provides rich statistical information | Does not add a constant by default, requires manual data preparation |
Whether you’re a beginner just starting out with sklearn logistic regression or an experienced data scientist looking to level up your skills, we hope this guide has given you a deeper understanding of how to implement logistic regression with sklearn and the power of this tool. Happy coding!