Python Heatmaps | Seaborn heatmap() Function and more
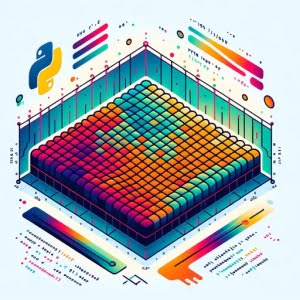
Are you finding it challenging to visualize your data in Python? You’re not alone. Many developers find themselves in a similar situation, but there’s a tool in Python that can make this process a breeze.
Like a painter bringing a canvas to life, Python can transform your raw data into vibrant heatmaps. These heatmaps can help you understand patterns, correlations, and trends in your data.
This guide will walk you through the process of creating heatmaps in Python, from simple to advanced. We’ll explore Python’s seaborn library, delve into its core functionality, discuss its advanced features, and even troubleshoot common issues.
So, let’s dive in and start mastering Python heatmaps!
TL;DR: How Do I Create a Heatmap in Python?
To create a heatmap in Python, you can use the seaborn library’s
heatmap()
function. This function takes a DataFrame as input and generates a heatmap as output.
Here’s a simple example:
import seaborn as sns
import pandas as pd
data = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
sns.heatmap(data)
# Output:
# A heatmap visualization of the data
In this example, we first import the seaborn and pandas libraries. We then create a DataFrame using pandas. The DataFrame is a 3×3 grid with values ranging from 1 to 9. We then pass this DataFrame to the heatmap()
function from seaborn, which generates a heatmap visualization of the data.
This is a basic way to create a heatmap in Python, but there’s much more to learn about creating and customizing heatmaps. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the Basics of Python Heatmaps
- Diving Deeper: Advanced Heatmap Techniques
- Exploring Alternatives: Matplotlib and Plotly
- Troubleshooting Python Heatmaps: Common Issues and Solutions
- Unraveling the Theory: Heatmaps and Data Visualization
- Heatmaps in Data Analysis and Machine Learning: The Bigger Picture
- Wrapping Up: Mastering Python Heatmaps
Understanding the Basics of Python Heatmaps
Seaborn’s Heatmap Function: A Primer
At the heart of creating heatmaps in Python is the seaborn library’s heatmap()
function. Seaborn is a Python data visualization library based on matplotlib. It provides a high-level interface for drawing attractive and informative statistical graphics, including heatmaps.
The heatmap()
function takes a data matrix as input and plots that matrix as a heatmap. Each cell in the heatmap corresponds to a data point in the matrix, and the color of the cell represents the value of that data point.
Here’s a basic example of how to use the heatmap()
function:
import seaborn as sns
import pandas as pd
data = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
sns.heatmap(data)
# Output:
# A heatmap visualization of the data
In this example, we first import the seaborn and pandas libraries. We then create a DataFrame using pandas. The DataFrame is a 3×3 grid with values ranging from 1 to 9. We then pass this DataFrame to the heatmap()
function from seaborn, which generates a heatmap visualization of the data.
One of the key advantages of seaborn’s heatmap()
function is its simplicity. With just a few lines of code, you can generate a meaningful visualization of your data. However, it’s important to note that the heatmap()
function has its limitations. It works best with numerical data, and it may not be suitable for categorical data or data with a large number of unique values.
In the following sections, we’ll delve deeper into the heatmap()
function and explore how to use it to create more complex heatmaps.
Diving Deeper: Advanced Heatmap Techniques
Customizing Heatmaps: Colors, Annotations, and Correlations
Once you’ve mastered the basics of creating heatmaps in Python using seaborn’s heatmap()
function, you can start exploring more advanced features. These features allow you to customize your heatmaps and make them more informative and visually appealing.
Changing Color Schemes
One of the simplest ways to customize your heatmap is by changing the color scheme. The heatmap()
function allows you to specify a color map using the cmap
parameter. Here’s how you can create a heatmap with a different color scheme:
import seaborn as sns
import pandas as pd
data = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
sns.heatmap(data, cmap='coolwarm')
# Output:
# A heatmap visualization of the data with a cool-warm color scheme
In this example, we use the ‘coolwarm’ color map, which ranges from cool to warm colors. This can be particularly useful when you want to emphasize differences between high and low values.
Adding Annotations
Another useful feature is the ability to add annotations to the heatmap. Annotations can help you quickly identify the value represented by each cell. You can add annotations using the annot
parameter:
sns.heatmap(data, cmap='coolwarm', annot=True)
# Output:
# A heatmap visualization of the data with annotations
In this example, the annot=True
parameter adds the value of each cell as an annotation on the heatmap.
Creating a Correlation Heatmap
One of the most common uses of heatmaps is to visualize correlations between different variables. You can create a correlation heatmap by first calculating the correlation matrix of your data and then passing this matrix to the heatmap()
function:
correlation_matrix = data.corr()
sns.heatmap(correlation_matrix, cmap='coolwarm', annot=True)
# Output:
# A correlation heatmap visualization of the data
In this example, we first calculate the correlation matrix of the data using the corr()
function from pandas. We then pass this correlation matrix to the heatmap()
function to create a correlation heatmap. This can be particularly useful when you’re working with large datasets and you want to identify relationships between different variables.
By mastering these advanced features, you can create more complex and informative heatmaps using Python and seaborn.
Exploring Alternatives: Matplotlib and Plotly
While seaborn is a popular library for creating heatmaps in Python, it’s not the only option. Other libraries such as matplotlib and plotly also offer functions for creating heatmaps. These alternative approaches come with their own set of advantages and disadvantages.
Matplotlib: Python’s Fundamental Plotting Library
Matplotlib is a comprehensive library for creating static, animated, and interactive visualizations in Python. You can create a heatmap in matplotlib using the imshow()
function:
import matplotlib.pyplot as plt
import numpy as np
# Creating a 10x10 array
data = np.random.rand(10,10)
# Creating a heatmap using imshow()
plt.imshow(data, cmap='hot', interpolation='nearest')
plt.show()
# Output:
# A heatmap visualization of the data using Matplotlib
In this example, we first generate a 10×10 array of random values. We then pass this array to the imshow()
function, which displays the array as a heatmap.
One of the main advantages of using matplotlib is its flexibility. You can customize almost every aspect of your heatmap, from the color map to the interpolation method. However, matplotlib’s syntax can be more complex and less intuitive than seaborn’s, especially for beginners.
Plotly: Interactive Data Visualization
Plotly is another powerful Python library for data visualization. It’s particularly known for its interactive plots. Here’s how you can create a heatmap in plotly:
import plotly.figure_factory as ff
# Create a random 10x10 dataframe
data = np.random.rand(10,10)
# Create a heatmap with plotly
heatmap = ff.create_annotated_heatmap(data, colorscale='Viridis')
heatmap.show()
# Output:
# An interactive heatmap visualization of the data using Plotly
In this example, we generate a 10×10 array of random values and pass this array to the create_annotated_heatmap()
function. This function returns an interactive heatmap that you can hover over to see the value of each cell.
One of the main advantages of using plotly is its interactivity. Interactive heatmaps can be particularly useful for exploratory data analysis, as they allow you to examine the value of each cell more closely. However, plotly’s syntax can be more complex than seaborn’s, and its interactivity may not be necessary for all use cases.
When choosing a library for creating heatmaps in Python, consider your specific needs and preferences. If you prefer a simple and intuitive syntax, seaborn may be the best choice. If you need more customization options, consider using matplotlib. If you need interactive plots, plotly may be the best option.
Troubleshooting Python Heatmaps: Common Issues and Solutions
Handling Missing Data
When working with real-world data, you might encounter missing values. Seaborn’s heatmap()
function does not handle missing data by default. If your dataset contains NaN values, the heatmap function will raise an error. To handle this, you can use the dropna()
function from pandas to remove missing values before creating the heatmap:
import seaborn as sns
import pandas as pd
import numpy as np
data = pd.DataFrame({'A': [1, np.nan, 3], 'B': [4, 5, np.nan], 'C': [7, 8, 9]})
data = data.dropna()
sns.heatmap(data)
# Output:
# A heatmap visualization of the data with missing values removed
In this example, the DataFrame contains NaN values. We use the dropna()
function to remove these values before passing the DataFrame to the heatmap()
function.
Dealing with Large Datasets
Another common issue when creating heatmaps is dealing with large datasets. If your dataset is too large, the heatmap can become cluttered and difficult to interpret. One solution is to use clustering techniques to group similar data points together. Seaborn’s clustermap()
function can help with this:
import seaborn as sns
import pandas as pd
import numpy as np
data = pd.DataFrame(np.random.rand(100,100))
sns.clustermap(data)
# Output:
# A clustered heatmap visualization of the data
In this example, we create a 100×100 DataFrame of random values. We then pass this DataFrame to the clustermap()
function, which creates a clustered heatmap. This can make it easier to identify patterns in the data.
Addressing Color Perception Issues
Finally, color perception can be an issue when creating heatmaps. Different people perceive colors differently, and this can affect how they interpret a heatmap. One solution is to use a colorblind-friendly color map, such as ‘viridis’. You can specify this color map using the cmap
parameter:
sns.heatmap(data, cmap='viridis')
# Output:
# A heatmap visualization of the data with a colorblind-friendly color map
In this example, we use the ‘viridis’ color map, which is designed to be perceptually uniform and colorblind-friendly. This can make your heatmap more accessible to a wider audience.
Unraveling the Theory: Heatmaps and Data Visualization
The Science behind Heatmaps
Heatmaps are a powerful tool for visualizing complex data. They display values represented as colors, with different colors corresponding to different ranges of values. This allows us to perceive data patterns, correlations, and trends at a glance.
The theory behind heatmaps is rooted in the concept of color perception. Our brains are wired to perceive different colors and associate them with different meanings. For instance, we often associate warm colors like red and orange with high values, and cool colors like blue and green with low values. Heatmaps leverage this innate color perception to represent data.
In the context of Python, a heatmap is essentially a two-dimensional graphical representation of data where the individual values contained in a matrix are represented as colors. The seaborn library’s heatmap()
function is a perfect tool for this, as it automatically maps data values to a color scale and displays the result as an image.
import seaborn as sns
import pandas as pd
data = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
sns.heatmap(data)
# Output:
# A heatmap visualization of the data
In this code block, we create a DataFrame and pass it to seaborn’s heatmap()
function. The function then maps the data values to a color scale, with different colors representing different ranges of values. The result is a heatmap that provides a visual representation of the data.
Color Perception and Heatmap Effectiveness
The effectiveness of a heatmap largely depends on the chosen color scale. The right color scale can make patterns and correlations stand out, while the wrong one can make them difficult to perceive. It’s important to choose a color scale that matches the nature of your data and the message you want to convey.
For instance, if you’re working with data that has a clear low-to-high progression (like temperatures), you might choose a color scale that goes from cool to warm colors. On the other hand, if you’re working with categorical data, you might choose a color scale with distinctly different colors to represent different categories.
Furthermore, it’s essential to consider color blindness when choosing a color scale for your heatmap. Color blindness affects a significant portion of the population, and certain color scales can be difficult for color-blind people to interpret. Fortunately, libraries like seaborn provide color-blind-friendly color scales, such as ‘viridis’.
sns.heatmap(data, cmap='viridis')
# Output:
# A heatmap visualization of the data with a colorblind-friendly color map
In this example, we use the ‘viridis’ color map, which is designed to be perceptually uniform and colorblind-friendly. This ensures that our heatmap is accessible to a wider audience.
Understanding the theory behind heatmaps and the principles of color perception can help you create more effective heatmaps. It allows you to choose the right color scale for your data and ensures that your heatmap is accessible and meaningful to your audience.
Heatmaps in Data Analysis and Machine Learning: The Bigger Picture
The Role of Heatmaps in Data Analysis
Heatmaps play a crucial role in data analysis. They provide a visual representation of data that can reveal patterns, correlations, and trends that might not be apparent from raw data. This can be particularly useful when working with large datasets or complex data structures.
Heatmaps can also help to communicate findings in a clear and compelling way. They can make data more accessible to stakeholders who may not have a technical background, facilitating better decision-making.
Heatmaps in Machine Learning
In the field of machine learning, heatmaps can be used to visualize the weights, biases, and activations in neural networks. This can provide insights into how a model is learning and help to diagnose issues.
For instance, if a certain neuron in a neural network is always activated or always inactive, it might indicate a problem with the learning process. Visualizing these activations as a heatmap can make it easier to identify such issues.
Broadening Your Horizons: Other Types of Data Visualization
While heatmaps are a powerful tool for data visualization, they’re just one of the many tools available. Other types of data visualization include scatter plots, line graphs, bar charts, and pie charts. Each type of visualization has its strengths and weaknesses, and the best choice depends on the nature of your data and the message you want to convey.
For instance, scatter plots can be useful for visualizing the relationship between two variables, while line graphs are great for showing trends over time. Bar charts and pie charts, on the other hand, are ideal for comparing different categories of data.
Diving Deeper: Statistical Analysis
Data visualization is often used in conjunction with statistical analysis. Statistical analysis provides the tools to quantify patterns, correlations, and trends in data, while data visualization provides the tools to visualize these findings.
For instance, you might use statistical analysis to calculate the correlation between two variables, and then use a heatmap to visualize this correlation. This combination of statistical analysis and data visualization can provide a powerful tool for data analysis.
Further Resources for Python Heatmap Mastery
If you’re interested in learning more about creating heatmaps in Python, here are some resources that you might find helpful:
- IOFlood’s Python Libraries Guide explains how to create graphical user interfaces with Python libraries.
Python Scientific Computing with SciPy An overview of the SciPy library, a scientific computing tool in Python
Performing t-Tests in Python A quick tutorial on the t-test statistical analysis technique in Python for hypothesis testing.
Seaborn’s official documentation is a great resource for learning more about seaborn and its
heatmap()
function.Matplotlib’s official documentation is a guide to matplotlib, including its
imshow()
function for creating heatmaps.Plotly’s official documentation can help you learn more about plotly and its functions for creating interactive heatmaps.
Wrapping Up: Mastering Python Heatmaps
In this comprehensive guide, we’ve explored the process of creating heatmaps in Python, a powerful tool for visualizing complex data. We’ve seen how Python, with the help of libraries such as seaborn, matplotlib, and plotly, can transform raw data into vibrant, informative heatmaps.
We began with the basics, learning how to create simple heatmaps using seaborn’s heatmap()
function. We then ventured into more advanced territory, exploring how to customize heatmaps by changing color schemes, adding annotations, and creating correlation heatmaps. We also delved into the theory behind heatmaps and the principles of color perception, giving you a deeper understanding of how heatmaps work and how to make them more effective.
Along the way, we tackled common challenges you might face when creating heatmaps in Python, such as handling missing data, dealing with large datasets, and addressing color perception issues. For each challenge, we provided solutions and workarounds to help you overcome these obstacles and create effective heatmaps.
We also looked at alternative approaches to creating heatmaps, comparing seaborn with other libraries like matplotlib and plotly.
Here’s a quick comparison of these libraries:
Library | Ease of Use | Customization Options | Interactivity |
---|---|---|---|
Seaborn | High | Moderate | Low |
Matplotlib | Moderate | High | Low |
Plotly | Moderate | High | High |
Whether you’re just starting out with creating heatmaps in Python or you’re looking to level up your data visualization skills, we hope this guide has given you a deeper understanding of how to create effective heatmaps in Python.
With its balance of simplicity, customization options, and powerful visualization capabilities, Python is a fantastic tool for creating heatmaps. Now, you’re well equipped to take your data visualization skills to the next level. Happy coding!