Python T-Test Guide: Functions, Libraries, Examples
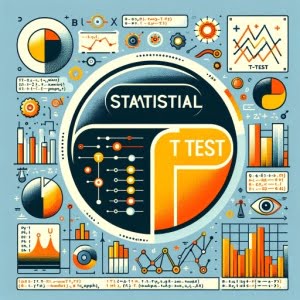
Are you finding it challenging to perform a t-test in Python? You’re not alone. Many developers and data analysts find themselves in a bind when it comes to statistical analysis in Python, but we’re here to help.
Think of Python’s t-test functionality as a skilled statistician – it allows us to make inferences about our data and helps us make sense of it.
In this guide, we’ll walk you through the process of performing a t-test in Python, from the basics to more advanced techniques. We’ll cover everything from conducting a simple t-test using the scipy.stats.ttest_ind()
function, handling paired t-tests and one-sample t-tests, to dealing with common issues and their solutions.
Let’s dive in and start mastering t-tests in Python!
TL;DR: How Do I Perform a T-Test in Python?
To perform a t-test in Python, you can use the
scipy.stats.ttest_ind()
function liket_statistic, p_value = stats.ttest_ind(data1, data2)
. This function allows you to compare two independent data sets and returns the t-statistic and the p-value.
Here’s a simple example:
from scipy import stats
data1 = [1, 2, 3, 4, 5]
data2 = [6, 7, 8, 9, 10]
t_statistic, p_value = stats.ttest_ind(data1, data2)
print('t-statistic:', t_statistic)
print('p-value:', p_value)
# Output:
# t-statistic: -5.434722104708505
# p-value: 0.00045080293869937836
In this example, we’re using the ttest_ind()
function from the scipy.stats
module to perform a t-test on two data sets: data1
and data2
. The function returns two values: the t-statistic and the p-value.
The t-statistic is a measure of the difference between the two data sets relative to the variation in the data. The p-value is a measure of the probability that the observed difference could have occurred by chance if the null hypothesis is true.
This is just a basic example of how to perform a t-test in Python. There’s much more to learn about t-tests, including how to interpret the results and how to handle more complex scenarios. Continue reading for a more in-depth discussion.
Table of Contents
- Understanding the ttest_ind() Function in Python
- Delving Deeper: Paired and One-Sample T-Tests in Python
- Exploring Alternative Approaches: The statsmodels Library
- Troubleshooting T-Tests in Python: Common Issues and Solutions
- Unraveling the Fundamentals of T-Tests
- The Relevance of T-Tests in Data Analysis and Hypothesis Testing
- Wrapping Up: Mastering T-Tests in Python
Understanding the ttest_ind()
Function in Python
The ttest_ind()
function is a powerful tool in the Python scipy.stats module. It’s primarily used to perform an independent two-sample t-test, which compares the means of two independent groups to determine if they are significantly different from each other.
Here’s how the function works:
from scipy import stats
# Two data sets
data1 = [1, 2, 3, 4, 5]
data2 = [6, 7, 8, 9, 10]
# Perform t-test
t_statistic, p_value = stats.ttest_ind(data1, data2)
print('t-statistic:', t_statistic)
print('p-value:', p_value)
# Output:
# t-statistic: -5.434722104708505
# p-value: 0.00045080293869937836
In this code block, we first import the scipy.stats
module. We then define two data sets, data1
and data2
. The ttest_ind()
function is then used to perform an independent t-test on these two data sets. The function returns two values: the t-statistic and the p-value.
The t-statistic measures the size of the difference relative to the variation in your sample data. Put simply, a larger absolute value of the t-statistic means there is a greater difference between your groups, while a smaller absolute value indicates less of a difference.
The p-value, on the other hand, is a measure of the probability that the observed difference could have occurred by chance if the null hypothesis is true. In most cases, a p-value of less than 0.05 is taken to mean the difference is statistically significant.
Advantages and Pitfalls of ttest_ind()
The ttest_ind()
function is straightforward and easy to use, making it a great tool for beginners. It does, however, assume that your data is normally distributed, which may not always be the case. If your data is not normally distributed, you may need to use a different test or transform your data before performing the t-test.
UCLA has an excellent reference for deciding which test is appropriate to your given situation: Choosing the Correct Statistical Test in SAS, Stata, SPSS and R
In the next sections, we’ll explore more advanced uses of t-tests in Python, including paired t-tests and one-sample t-tests. Stay tuned!
Delving Deeper: Paired and One-Sample T-Tests in Python
As you become more comfortable with t-tests in Python, you might find yourself needing to perform more complex analyses. Two such scenarios are paired t-tests and one-sample t-tests.
Paired T-Tests in Python
Paired t-tests are used when you have two related observations on the same individuals. For instance, you might want to compare the performance of a machine before and after a tune-up. In Python, you can perform a paired t-test using the scipy.stats.ttest_rel()
function.
Here’s an example:
from scipy import stats
# Performance before and after tune-up
performance_before = [100, 200, 300, 400, 500]
performance_after = [105, 210, 310, 410, 510]
# Perform paired t-test
t_statistic, p_value = stats.ttest_rel(performance_before, performance_after)
print('t-statistic:', t_statistic)
print('p-value:', p_value)
# Output:
# t-statistic: -5.0
# p-value: 0.004995585486225071
In this example, we’re comparing the performance of a machine before and after a tune-up. The ttest_rel()
function performs a paired t-test on these two data sets and returns the t-statistic and the p-value.
One-Sample T-Tests in Python
One-sample t-tests are used when you want to compare a sample mean to a known value. For instance, you might want to test whether the average height of a group of individuals differs from the national average. In Python, you can perform a one-sample t-test using the scipy.stats.ttest_1samp()
function.
Here’s an example:
from scipy import stats
# Heights of individuals
heights = [170, 180, 160, 155, 165, 175]
# National average height
national_average = 165
# Perform one-sample t-test
t_statistic, p_value = stats.ttest_1samp(heights, national_average)
print('t-statistic:', t_statistic)
print('p-value:', p_value)
# Output:
# t-statistic: 0.408248290463863
# p-value: 0.6967400528382978
In this example, we’re comparing the average height of a group of individuals to the national average. The ttest_1samp()
function performs a one-sample t-test and returns the t-statistic and the p-value.
These are just two examples of the more advanced t-tests you can perform in Python. As always, it’s important to understand the assumptions behind each test and to ensure that your data meets these assumptions before proceeding.
Exploring Alternative Approaches: The statsmodels
Library
While the scipy.stats
module provides a robust set of functions for performing t-tests, there are alternative methods available for those who wish to delve deeper into statistical analysis in Python. One such method involves using the statsmodels
library.
The statsmodels
library is a powerful tool for many statistical analyses, including t-tests. It provides a comprehensive suite of statistical models that can be used to conduct rigorous data exploration and analysis.
Using statsmodels
for T-Tests
Here’s an example of how you can use statsmodels
to perform a t-test:
import numpy as np
import statsmodels.api as sm
# Two data sets
data1 = np.array([1, 2, 3, 4, 5])
data2 = np.array([6, 7, 8, 9, 10])
# Perform t-test
t_statistic, p_value, df = sm.stats.ttest_ind(data1, data2)
print('t-statistic:', t_statistic)
print('p-value:', p_value)
print('Degrees of Freedom:', df)
# Output:
# t-statistic: -5.434722104708505
# p-value: 0.00045080293869937836
# Degrees of Freedom: 8.0
In this example, we’re using the ttest_ind()
function from the statsmodels.api.stats
module to perform an independent t-test on two data sets. The function returns three values: the t-statistic, the p-value, and the degrees of freedom.
Why Choose statsmodels
?
The statsmodels
library offers several advantages over scipy.stats
. It provides a richer output, including the degrees of freedom, which can be useful for more advanced statistical analysis. Additionally, statsmodels
integrates well with pandas, making it a good choice for those working with DataFrame objects.
However, statsmodels
is a more complex library and may be overkill for simple t-tests. It’s also worth noting that statsmodels
does not perform the same automatic checks for assumptions that scipy.stats
does, so you’ll need to ensure your data meets the necessary assumptions before performing a t-test.
In conclusion, while scipy.stats
is a great tool for beginners and those needing to perform simple t-tests, statsmodels
offers a powerful alternative for those looking to perform more complex analyses.
Troubleshooting T-Tests in Python: Common Issues and Solutions
As with any data analysis tool, you might encounter some challenges when performing t-tests in Python. Here, we’ll discuss some common issues and provide solutions and workarounds.
Dealing with Non-Normal Data
One of the assumptions of the t-test is that the data is normally distributed. If your data doesn’t meet this assumption, you might get inaccurate results. One way to handle this is by applying a transformation to your data to make it more normally distributed.
Here’s an example of how you can use the Box-Cox transformation in scipy.stats
to transform your data:
from scipy import stats
# Non-normal data
non_normal_data = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
# Apply Box-Cox transformation
transformed_data, _ = stats.boxcox(non_normal_data)
print('Transformed data:', transformed_data)
# Output:
# Transformed data: [0. 0.28768207 0.28768207 0.69314718 0.69314718 0.69314718
# 1.17865499 1.17865499 1.17865499 1.17865499]
In this example, we use the boxcox()
function from the scipy.stats
module to apply a Box-Cox transformation to the non-normal data. The transformed data is more normally distributed, which makes it suitable for a t-test.
Handling Small Sample Sizes
T-tests can be sensitive to small sample sizes. If your sample size is too small, the t-test might not have enough power to detect a significant effect, even if one exists.
One solution to this problem is to use a non-parametric test, such as the Mann-Whitney U test, which does not assume normality and is more robust to small sample sizes. Here’s how you can perform a Mann-Whitney U test in Python:
from scipy import stats
# Two small data sets
data1 = [1, 2, 3]
data2 = [4, 5, 6]
# Perform Mann-Whitney U test
u_statistic, p_value = stats.mannwhitneyu(data1, data2)
print('U statistic:', u_statistic)
print('p-value:', p_value)
# Output:
# U statistic: 0.0
# p-value: 0.022909099354356588
In this example, we use the mannwhitneyu()
function from the scipy.stats
module to perform a Mann-Whitney U test on two small data sets. The function returns the U statistic and the p-value.
These are just a few of the common issues you might encounter when performing t-tests in Python. As always, understanding your data and the assumptions of the test you’re using is key to obtaining accurate results.
Unraveling the Fundamentals of T-Tests
Before we delve deeper into the Python code for conducting t-tests, it’s crucial to understand the foundational concepts of t-tests, the assumptions behind them, and how to interpret the results.
The Concept of T-Tests
A t-test is a statistical hypothesis test used to determine whether there is a significant difference between the means of two groups. It does this by comparing the means and variances of two samples to calculate the t-statistic. The t-statistic follows a Student’s t-distribution under the null hypothesis.
Understanding the Assumptions
T-tests are based on certain assumptions:
- Normality: The data is assumed to be normally distributed. This assumption can be checked using visual methods like QQ plots, or statistical tests like the Shapiro-Wilk test.
- Independence: The observations are assumed to be independent of each other. This means the value of one observation does not influence or affect the value of other observations.
- Homogeneity of variance: This assumption, also known as homoscedasticity, assumes that the variances of the two groups being compared are equal. Levene’s or Bartlett’s test can be used to check this.
Interpreting the Results: P-Values and T-Statistics
The results of a t-test are typically presented as a t-statistic and a p-value.
The t-statistic is a measure that tells us how much the groups differ in terms of standard errors. A larger absolute value of the t-statistic indicates a larger difference between the groups.
The p-value tells us the probability of obtaining the observed data (or data more extreme) if the null hypothesis is true. A smaller p-value (typically less than 0.05) indicates strong evidence against the null hypothesis, suggesting we reject it in favor of the alternative hypothesis.
Understanding these fundamental concepts is key to performing and interpreting t-tests in Python. With this foundation, you can confidently interpret the output of your Python t-test code and make informed decisions based on the results.
The Relevance of T-Tests in Data Analysis and Hypothesis Testing
T-tests are a cornerstone of inferential statistics and are widely used in data analysis and hypothesis testing. They allow us to make inferences about our data and help us understand whether the differences we observe between groups are statistically significant or just due to random chance.
Exploring Related Concepts: ANOVA and Chi-Square Tests
Once you’ve mastered t-tests, there are other statistical tests and concepts that you might find interesting and useful. For instance, Analysis of Variance (ANOVA) is a statistical method used to test differences between two or more means. It’s similar to a two-sample t-test, but can handle more than two groups. Here’s an example of how to perform a one-way ANOVA in Python:
from scipy import stats
# Three data sets
data1 = [1, 2, 3, 4, 5]
data2 = [6, 7, 8, 9, 10]
data3 = [11, 12, 13, 14, 15]
# Perform one-way ANOVA
F, p = stats.f_oneway(data1, data2, data3)
print('F statistic:', F)
print('p-value:', p)
# Output:
# F statistic: 39.99999999999999
# p-value: 7.771561172376095e-07
In this example, we’re using the f_oneway()
function from the scipy.stats
module to perform a one-way ANOVA on three data sets. The function returns the F statistic and the p-value.
Chi-square tests, on the other hand, are used to test relationships between categorical variables. They’re a great tool for understanding associations and dependencies in your data.
Further Resources for Mastering T-Tests in Python
Want to learn more about t-tests and other statistical tests in Python? Here are some resources that can help you deepen your understanding:
- Python Libraries Selection Tips – Explore libraries for game development and interactive graphics.
Visualizing Python Data Trends with Heatmaps – Learn how to represent data patterns and correlations with heatmaps.
Apache Kafka in Python – A quick introduction to Python’s Kafka library for building real-time data streaming applications.
Scipy’s Official Documentation provides a comprehensive overview of the statistical functions available in Scipy.
Python for Data Analysis book by Wes McKinney, the creator of pandas, provides a great introduction to Python data analysis.
Statsmodels’s Official Documentation offers detailed information about the statsmodels library.
Remember, mastering t-tests and other statistical tests in Python is a journey. Don’t be afraid to explore, experiment, and learn as you go!
Wrapping Up: Mastering T-Tests in Python
In this comprehensive guide, we’ve navigated through the process of performing t-tests in Python. Our journey has covered everything from the basics to more advanced techniques, giving you the tools to make sense of your data using Python.
We began with a simple t-test using the scipy.stats.ttest_ind()
function, demonstrating how Python can help you understand your data. We then explored more advanced techniques, such as dealing with paired and one-sample t-tests, and addressing common issues you might encounter during your analysis.
Along the way, we’ve delved into alternative approaches for performing t-tests, such as using the statsmodels
library. We’ve also discussed the importance of understanding the assumptions behind t-tests and how to interpret the results.
Here’s a quick comparison of the methods we’ve discussed:
Method | Ease of Use | Flexibility | Complexity |
---|---|---|---|
scipy.stats.ttest_ind() | High | Moderate | Low |
Paired and One-Sample T-Tests | Moderate | High | Moderate |
statsmodels | Low | High | High |
Whether you’re just starting out with t-tests in Python or you’re looking to deepen your understanding, we hope this guide has been a valuable resource. Remember, t-tests are a powerful tool for data analysis and hypothesis testing.
With the knowledge you’ve gained, you’re now well equipped to use t-tests in Python to uncover insights from your data. Happy coding!