Mastering Design Patterns in Java: Your Ultimate Guide
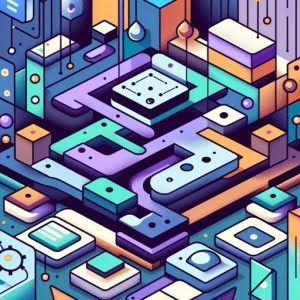
Are you finding it challenging to master design patterns in Java? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Think of Java’s design patterns as a blueprint – a blueprint that simplifies the software development process, making it more manageable and efficient.
This guide will walk you through the most common design patterns in Java, from basic to advanced levels. We’ll explore their core functionality, delve into their advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering design patterns in Java!
TL;DR: What are Design Patterns in Java?
Design patterns in Java provide solutions to common software design problems and enhance efficient communication among developers. There are three main types:
Creational, Structural, and Behavioral
. Key examples include:Singleton pattern, Factory pattern, Builder pattern, Adapter pattern, Decorator pattern, and Strategy pattern
.
Here’s a simple example:
public class Singleton {
private static Singleton single_instance = null;
public String s;
private Singleton() {
s = "Hello I am a singleton!";
}
public static Singleton getInstance()
{
if (single_instance == null)
single_instance = new Singleton();
return single_instance;
}
}
# Output:
# 'Hello I am a singleton!'
In this example, we’ve created a Singleton class. The Singleton class maintains a static reference to the lone singleton instance and returns that reference from the static getInstance() method.
This is just a basic example of a design pattern in Java. There’s a lot more to learn about design patterns and their implementation in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding Singleton and Factory Design Patterns
- Delving into Observer and Decorator Patterns
- Exploring Composite and State Patterns
- Common Issues with Design Patterns in Java
- Understanding the Fundamentals of Design Patterns
- The Relevance of Design Patterns in Large-Scale Software Development
- Design Patterns in Microservices Architecture
- Exploring Related Concepts: SOLID Principles and Clean Code Practices
- Wrapping Up: Java Design Patterns
Understanding Singleton and Factory Design Patterns
Singleton Pattern: The One and Only
The Singleton pattern is a design pattern that restricts the instantiation of a class to a single instance. This is useful when exactly one object is needed to coordinate actions across the system.
Here’s a simple Singleton pattern implementation in Java:
public class Singleton {
private static Singleton instance = null;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
In this code, we’ve created a Singleton class. The Singleton class maintains a static reference to the lone singleton instance and returns that reference from the static getInstance() method.
The Singleton pattern has several advantages, such as controlled access to the sole instance, reduced namespace, and the ability to permit a variable number of instances. However, it can be difficult to unit test due to its global access nature, and it may mask bad design, such as not defining proper objects for the job.
Factory Pattern: Creating Objects Made Easy
The Factory pattern is another common design pattern in Java. This pattern involves a single class (the factory) which is responsible for creating instances of other classes.
Here’s a simple Factory pattern implementation in Java:
public class AnimalFactory {
public Animal getAnimal(String animalType) {
if (animalType == null) {
return null;
}
if (animalType.equalsIgnoreCase("DOG")) {
return new Dog();
} else if (animalType.equalsIgnoreCase("CAT")) {
return new Cat();
}
return null;
}
}
In this code, we’ve created an AnimalFactory class. This factory class creates different types of Animal objects based on the input it receives.
The Factory pattern provides a way to encapsulate a group of individual factories that have a common theme without specifying their concrete classes. However, the complexity of the code increases as the number of classes increases, and it can become difficult to manage and maintain.
Delving into Observer and Decorator Patterns
Observer Pattern: Keeping Tabs
The Observer pattern is a design pattern where an object (known as a subject) maintains a list of objects depending on it (observers), automatically notifying them of any changes to state.
Here’s a simple Observer pattern implementation in Java:
import java.util.Observable;
import java.util.Observer;
public class JobPost extends Observable {
private String title;
public JobPost(String title) {
this.title = title;
}
public String getTitle() {
return title;
}
public void addJob(Observer o) {
this.addObserver(o);
this.setChanged();
this.notifyObservers(this);
}
}
In this code, we’ve created a JobPost class. When a new job is added, all registered job seekers will get notified.
The Observer pattern is a great way to achieve loose coupling between objects. It allows for a highly modular structure, where the subject and observers can be reused independently. However, observers are not aware of each other and can lead to unexpected updates.
Decorator Pattern: Adding Flair
The Decorator pattern is a design pattern that allows behavior to be added to an individual object, either statically or dynamically, without affecting the behavior of other objects from the same class.
Here’s a simple Decorator pattern implementation in Java:
public abstract class Coffee {
public abstract double getCost();
}
public class SimpleCoffee extends Coffee {
@Override
public double getCost() {
return 1;
}
}
public class MilkCoffee extends Coffee {
protected Coffee coffee;
public MilkCoffee(Coffee coffee) {
this.coffee = coffee;
}
@Override
public double getCost() {
return this.coffee.getCost() + 0.5;
}
}
In this code, we’ve created a Coffee class and a SimpleCoffee class that extends Coffee. We then created a MilkCoffee class that also extends Coffee and adds a cost to it.
The Decorator pattern is useful for adding and removing responsibilities from objects at runtime. It provides a flexible alternative to subclassing for extending functionality. However, it can result in many small objects that can be hard to track and debug.
Exploring Composite and State Patterns
Composite Pattern: Building Blocks
The Composite pattern is a structural design pattern that allows you to compose objects into tree structures and then work with these structures as if they were individual objects.
Here’s a simple Composite pattern implementation in Java:
interface Component {
void showPrice();
}
class Leaf implements Component {
private int price;
Leaf(int price) {
this.price = price;
}
public void showPrice() {
System.out.println(price);
}
}
class Composite implements Component {
List<Component> components = new ArrayList<Component>();
void addComponent(Component component) {
components.add(component);
}
public void showPrice() {
for (Component c : components) {
c.showPrice();
}
}
}
In this example, we’ve created a Component interface, a Leaf class that implements Component, and a Composite class that also implements Component. The Composite class can contain both Leaf and Composite objects, allowing for complex, tree-like structures.
The Composite pattern is great for representing part-whole hierarchies. It makes it easier to add new kinds of components. However, it can make design overly general and harder to restrict components.
State Pattern: Adapting to Change
The State pattern is a behavioral design pattern that lets an object alter its behavior when its internal state changes. The object will appear to change its class.
Here’s a simple State pattern implementation in Java:
interface State {
void handleRequest();
}
class ConcreteStateA implements State {
public void handleRequest() {
System.out.println("Handling request by turning into ConcreteStateB");
}
}
class ConcreteStateB implements State {
public void handleRequest() {
System.out.println("Handling request by turning into ConcreteStateA");
}
}
class Context {
private State state;
public void setState(State state) {
this.state = state;
}
public void request() {
state.handleRequest();
this.setState(new ConcreteStateA());
}
}
In this code, we’ve created a State interface and two concrete states that implement the State interface. We also have a Context class that uses the State interface to change its behavior based on its current state.
The State pattern allows a class to change its behavior at runtime. It also encapsulates state-specific behavior and divides the behavior of a class. However, the State pattern can be overkill if a state machine has only a few states or rarely changes.
Common Issues with Design Patterns in Java
When implementing design patterns in Java, developers often run into a few common issues. Let’s explore these problems and provide some solutions and workarounds.
Misuse of Patterns
One of the most common problems is the misuse of patterns. Sometimes, developers force the use of design patterns where they’re not needed, leading to unnecessary complexity.
For example, using the Singleton pattern unnecessarily can lead to problems with unit testing and hiding dependencies.
Overuse of Patterns
Another common issue is the overuse of patterns. While design patterns can be powerful tools, using them excessively can lead to overly complex and hard-to-maintain code.
For instance, overusing the Factory pattern can lead to an explosion of factory classes, making the code harder to understand and manage.
Solutions and Workarounds
To avoid these issues, it’s important to understand the purpose and use case of each design pattern. Use design patterns only when they solve a specific problem and simplify the code, not just for the sake of using them.
Additionally, remember that design patterns are not concrete designs. They can be modified and adapted to fit the specific needs of your project. Don’t be afraid to adapt a pattern or create your own if it serves your project better.
In conclusion, while design patterns in Java are powerful tools, they must be used judiciously and appropriately. Understanding the potential issues and how to avoid them will help you use design patterns more effectively in your Java projects.
Understanding the Fundamentals of Design Patterns
Design patterns are fundamental to software development, providing a standardized and optimized approach to solving common problems. They are essentially well-proven solutions to recurring issues in software design.
What are Design Patterns?
In the context of software development, a design pattern is a reusable solution that can be applied to common problems encountered in software design. They represent best practices evolved over a period of time and are named in a way that can convey their intent.
public class Singleton {
private static Singleton instance = null;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
In the above example, we’ve implemented a Singleton pattern. This pattern ensures that a class only has one instance and provides a global point of access to it.
Why are Design Patterns Important?
Design patterns provide a standard terminology and are fundamental to efficient communication among developers. They allow developers to discuss possible solutions efficiently, as they provide a common language for certain complex structures. Moreover, design patterns encourage code reuse, reducing the overall development effort.
The Role of Design Patterns in Java Programming
In Java programming, design patterns play a crucial role in helping developers write flexible and reusable code. They provide solutions to common programming challenges and follow the ‘Gang of Four’ design patterns, which are divided into three categories: Creational, Structural, and Behavioral. Each pattern has a specific role and use-case in the Java programming language.
In summary, understanding design patterns and their role in Java programming is crucial for writing efficient, reusable, and understandable code.
The Relevance of Design Patterns in Large-Scale Software Development
Design patterns are not just for small projects or simple programs. They play a critical role in large-scale software development as well. When dealing with complex systems with numerous interacting parts, design patterns can help manage this complexity by providing a clear, standardized structure.
For instance, the Factory pattern can be used to manage and control the creation of objects in a large application, while the Observer pattern can help maintain consistency in a system with many interconnected parts.
Design Patterns in Microservices Architecture
In the world of microservices architecture, design patterns still hold a significant place. Microservices architecture is about developing a single application as a suite of small services, each running its own process and communicating with lightweight mechanisms. Here, patterns like the Aggregator, Proxy, and Chained Microservices patterns can be incredibly useful.
Exploring Related Concepts: SOLID Principles and Clean Code Practices
Design patterns are just one tool in a developer’s toolkit. Other concepts, like the SOLID principles and clean code practices, are also crucial for writing efficient, maintainable code.
The SOLID principles are a set of five principles for designing software that is easy to manage and maintain. They include principles like Single Responsibility, Open-Closed, and Liskov Substitution, which all aim to make software more understandable, flexible, and maintainable.
Clean code practices, on the other hand, are about making the code as clear, simple, and readable as possible. This includes practices like choosing descriptive names, keeping functions and classes small, and using comments sparingly and effectively.
Further Resources for Mastering Design Patterns in Java
Java Design patterns are an inherent part of Java Object Oriented Programming. To delve further into the benefits of design in Java OOP, and other concepts, Click Here!
To further your understanding of design patterns in Java, here are some additional resources:
- Java Abstract Class Basics – Learn how to declare abstract methods and classes, and extend abstract classes.
Lambda Expressions in Java – Explore lambda expressions in Java for writing concise and expressive code.
Java Design Patterns – A complete guide to all the classic design patterns in Java.
Design Patterns – A great resource for learning about design patterns in various programming languages.
Design Patterns Series – A series of articles explaining various design patterns and their implementation in Java.
Wrapping Up: Java Design Patterns
In this comprehensive guide, we’ve delved into the world of design patterns in Java, a powerful tool for efficient and reusable code. We’ve explored the most common design patterns, from basic to advanced, and discussed their implementation, advantages, and potential pitfalls.
We began with the basics, introducing the Singleton and Factory patterns, which are fundamental to many Java applications. We then moved on to more complex patterns, such as the Observer and Decorator patterns, providing code examples and discussing their use cases and benefits.
Next, we ventured into expert-level territory, introducing alternative approaches such as the Composite and State patterns. We provided code examples for these patterns, discussed their advantages and disadvantages, and provided recommendations for their use.
We also touched on common issues that developers may encounter when implementing design patterns, such as misuse and overuse, and provided solutions and workarounds for these issues.
Here’s a quick comparison of the design patterns we’ve discussed:
Design Pattern | Level | Use Case |
---|---|---|
Singleton | Basic | Ensuring a class has only one instance |
Factory | Basic | Creating objects |
Observer | Intermediate | Maintaining consistency in systems |
Decorator | Intermediate | Adding responsibilities to objects |
Composite | Expert | Managing tree-like structures |
State | Expert | Allowing an object to change its behavior |
Whether you’re a beginner just starting out with Java, or an experienced developer looking to deepen your understanding of design patterns, we hope this guide has been a valuable resource.
Mastering design patterns in Java can significantly improve your coding efficiency and the quality of your software. So keep practicing, keep learning, and happy coding!