OOPs Concepts in Java: Structuring Code for Scalability
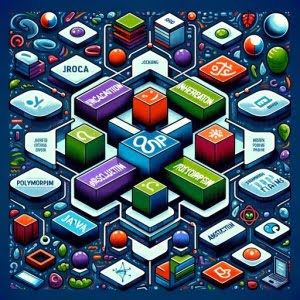
Are you finding it challenging to grasp Java’s OOP (Object-Oriented Programming) concepts? You’re not alone. Many developers find themselves puzzled when it comes to understanding and applying these fundamental principles in their Java applications.
Think of Java’s OOP concepts as the building blocks of your software – they provide a structure that makes your code reusable, scalable, and easy to maintain. These concepts are the backbone of most Java applications, making them a crucial aspect to master.
In this guide, we’ll walk you through the process of understanding and applying the four key OOP concepts in Java, from their basic definitions to their implementation with practical examples. We’ll cover everything from encapsulation, inheritance, polymorphism, to abstraction.
So, let’s dive in and start mastering Java OOP concepts!
TL;DR: What are the OOP Concepts in Java?
The four main OOP (Object-Oriented Programming) concepts in Java are
Encapsulation, Inheritance, Polymorphism, and Abstraction
. These principles help to structure Java code in a way that is reusable, scalable, and easy to maintain.
Here’s a simple example of how these concepts might be used in a Java class:
public class Animal {
// Encapsulation: private variables can only be accessed through public methods
private String name;
// Inheritance: subclasses can inherit properties and methods from this class
public void eat() {
System.out.println(name + ' is eating.');
}
// Polymorphism: subclasses can provide their own implementation of this method
public void makeSound() {
System.out.println('Animal sound');
}
// Abstraction: this class can be used as a blueprint for creating more specific animal classes
}
# Output:
# (This is a blueprint class and does not produce output on its own)
In this example, we’ve created a basic Animal
class that demonstrates each of the four OOP concepts. This class is abstract in nature and serves as a blueprint for creating more specific animal classes. It encapsulates the name
property, allows for inheritance by other classes, and provides a method (makeSound()
) that can be overridden by subclasses, demonstrating polymorphism.
This is just a basic introduction to OOP concepts in Java, but there’s much more to learn about each concept and how to use them effectively. Continue reading for more detailed explanations and examples of each concept.
Table of Contents
Understanding Encapsulation in Java
Encapsulation is one of the four fundamental OOP concepts in Java. It’s all about wrapping data (variables) and code acting on the data (methods) into a single unit known as a ‘class’. This mechanism is used to hide the values or state of a structured data object inside a class, preventing unauthorized parties’ direct access to them.
The primary benefit of encapsulation is the ability to modify our implemented code without breaking the code of others who use our code. With this feature, a class can change its internal implementation without hurting the overall functioning of the system.
Here’s a simple example of encapsulation in Java:
public class Employee {
private String name;
// Getter method
public String getName() {
return name;
}
// Setter method
public void setName(String newName) {
name = newName;
}
}
Employee emp = new Employee();
emp.setName('John Doe');
System.out.println(emp.getName());
# Output:
# 'John Doe'
In this example, the Employee
class encapsulates the name
variable. It’s marked as private, which means it can’t be accessed directly from outside the Employee
class. Instead, we use getter and setter methods (getName()
and setName()
) to read and modify the name
variable.
The main advantage of encapsulation is increased security – our data will be hidden from users, and can be accessed only through the methods we provide. However, it might make the code slightly longer since we need to create getter and setter methods for every data member. But the pros of encapsulation often outweigh this minor con.
Delving into Inheritance in Java
Inheritance is another fundamental concept of Java’s OOP paradigm. It’s a mechanism where one class acquires, or inherits, the properties (fields) and behaviors (methods) of another class. In Java, the class which inherits the properties of other is known as subclass (derived or child class), and the class whose properties are inherited is known as superclass (base or parent class).
Inheritance offers a way to promote code reuse and a way to define relationships between your classes, leading to a well-organized, hierarchical structure of code.
Here’s an example of how inheritance works in Java:
// Superclass
public class Animal {
public void eat() {
System.out.println('Eating...');
}
}
// Subclass
public class Dog extends Animal {
public void bark() {
System.out.println('Barking...');
}
}
Dog dog = new Dog();
dog.eat(); // method from Animal class
dog.bark(); // method from Dog class
# Output:
# 'Eating...'
# 'Barking...'
In this example, we have a superclass Animal
and a subclass Dog
. The Dog
class extends the Animal
class, which means it inherits the eat()
method from Animal
. So, an object of Dog
can call both eat()
and bark()
methods.
Inheritance allows for code reuse, which can lead to a reduction in time and effort needed to develop software. It also represents real-world relationships well. However, the main disadvantage of inheritance is that the two classes (base and derived) get tightly coupled. This means one cannot be easily understood in isolation of the other, which can lead to complexity when scaling the codebase.
Unraveling Polymorphism in Java
Polymorphism is another core concept of Java’s OOP philosophy. The term ‘Polymorphism’ is derived from the Greek words ‘Poly’ (many) and ‘morphs’ (forms). In the context of object-oriented programming, Polymorphism allows objects of different classes to be treated as objects of a common superclass.
Polymorphism in Java has two types: Compile-time polymorphism (method overloading) and Runtime polymorphism (method overriding).
Here’s an example of how Polymorphism works in Java:
// Superclass
public class Animal {
public void makeSound() {
System.out.println('Animal sound');
}
}
// Subclass
public class Dog extends Animal {
// Method overriding
@Override
public void makeSound() {
System.out.println('Barking...');
}
}
Animal myDog = new Dog();
myDog.makeSound();
# Output:
# 'Barking...'
In this example, we have a superclass Animal
with a method makeSound()
. We also have a subclass Dog
that extends Animal
and overrides the makeSound()
method. When we create an Animal
object that refers to a Dog
object and call makeSound()
, the Dog
‘s makeSound()
method is called instead of the Animal
‘s makeSound()
method. This is polymorphism in action.
Polymorphism simplifies coding and improves the readability and maintainability of your code by allowing objects of different types to be processed as objects of a common superclass. However, it can lead to confusion when not used correctly, as it allows for multiple implementations of the same method.
Mastering Abstraction in Java
Abstraction in Java, the final pillar of OOP, is a process of hiding the implementation details and showing only the functionality. Abstraction lets you focus on what the object does instead of how it does it. It creates a simple, high-level view of an entity while hiding complex, low-level details.
Abstraction can be achieved in two ways: Abstract Classes (0-100% abstraction) and Interfaces (100% abstraction).
Here’s an example of how Abstraction works in Java using an abstract class:
// Abstract class
abstract class Animal {
// Abstract method (does not have a body)
public abstract void makeSound();
}
// Subclass (inherit from Animal)
class Dog extends Animal {
public void makeSound() {
// The body of makeSound() is provided here
System.out.println('Barking...');
}
}
Animal myDog = new Dog(); // Create a Dog object
myDog.makeSound();
# Output:
# 'Barking...'
In this example, we have an abstract class Animal
with an abstract method makeSound()
. We also have a Dog
class that extends Animal
and provides an implementation for the makeSound()
method. When we create a Dog
object and call makeSound()
, the Dog
‘s makeSound()
method is called.
Abstraction is a powerful concept that reduces code complexity by hiding the internal implementation and only showing the essential features of the object. However, it can also lead to incomplete or incorrect implementation if not handled properly. It’s important to use abstraction judiciously and in a way that aids understanding and simplifies your code.
Troubleshooting Common Java OOP Issues
While Java’s OOP concepts are powerful tools for structuring your code, you may encounter some common issues when implementing them. Below, we’ll discuss these issues and provide some tips for resolving them.
Misuse of Inheritance
One common issue is the misuse of inheritance. Inheritance should be used to model the ‘is-a’ relationship between classes. However, it’s often misused to achieve code reuse, which can lead to a confusing and tightly coupled codebase.
To avoid this, favor composition (the ‘has-a’ relationship) over inheritance for code reuse. Here’s a simple example:
// Instead of 'Dog extends Animal', use composition
public class Dog {
private Animal animal;
public Dog(Animal animal) {
this.animal = animal;
}
public void makeSound() {
animal.makeSound();
}
}
# Output:
# (This is a blueprint class and does not produce output on its own)
In this example, Dog
has an Animal
object, which promotes code reuse without the tight coupling of inheritance.
Overloading vs Overriding Confusion
Another common issue is confusing method overloading with method overriding, both of which are forms of polymorphism. Remember, method overloading occurs when two methods in the same class have the same name but different parameters. Method overriding occurs when a subclass provides a specific implementation of a method that is already provided by its superclass.
Improper Use of Abstraction
Abstraction is a powerful tool for hiding complexity, but it can also lead to incomplete or incorrect implementation if not properly used. Always provide complete implementation of abstract methods in the subclasses.
Encapsulation Overlooked
Lastly, forgetting to encapsulate your classes’ data can lead to unauthorized access and potential misuse. Always use private visibility for your data members and provide public getter and setter methods.
By being aware of these common issues and how to solve them, you can more effectively use Java’s OOP concepts to structure and organize your code.
The Theory Behind Java OOP Concepts
Before diving into the practical application of Java’s OOP concepts, let’s first understand the theory behind it. Object-Oriented Programming (OOP) is a programming paradigm that uses ‘objects’ – instances of classes – to design applications and computer programs. It’s based on several core concepts: namely, Encapsulation, Inheritance, Polymorphism, and Abstraction.
Classes and Objects
In Java, a class is a blueprint from which individual objects are created. A class defines the properties (fields) and behaviors (methods) that an object can have. Here’s a simple example of a class and an object in Java:
// Class
public class Car {
// Field
private String color;
// Method
public void drive() {
System.out.println('Driving...');
}
}
// Object
Car myCar = new Car();
myCar.drive();
# Output:
# 'Driving...'
In this example, Car
is a class, and myCar
is an object of the Car
class. The Car
class has a field (color
) and a method (drive()
), and the myCar
object can access the drive()
method.
Methods and Attributes
Methods and attributes are key elements of a class. Methods define what type of functionality an object of the class has, and attributes are the properties the object possesses. In the Car
class example, drive()
is a method, and color
is an attribute.
Understanding these core concepts and the theory behind OOP is crucial to effectively using Java’s OOP concepts. They provide a solid foundation for organizing and structuring your code in a way that is both efficient and easy to understand.
Applying OOP Concepts in Large-Scale Java Projects
Java’s OOP concepts are not just theoretical constructs, but practical tools that can greatly simplify the process of developing large-scale projects. By structuring your code around objects and their interactions, you can create more flexible, modular programs that are easier to understand, debug, and maintain.
The Role of Design Patterns
One way to leverage the power of OOP in larger projects is through the use of design patterns. Design patterns are proven solutions to common software design problems. They represent best practices and can speed up the development process by providing tested, optimized solutions.
Some common OOP design patterns in Java include the Singleton pattern (which restricts a class to a single instance), the Factory pattern (which provides an interface for creating objects), and the Observer pattern (which defines a one-to-many dependency between objects).
OOP and Software Architecture
OOP concepts also play a crucial role in defining the architecture of a software system. For instance, the Model-View-Controller (MVC) architecture, commonly used in web applications, is built on OOP principles. The Model represents the application data, the View displays the data, and the Controller handles user input. Each component is an object with specific responsibilities, and they interact in well-defined ways.
Further Resources for Mastering Java OOP Concepts
To continue your journey in mastering Java’s OOP concepts, here are some resources that provide deeper insights and advanced topics:
- Java Basics – Dive into the foundational elements of Java programming with this comprehensive guide.
Using Encapsulation in Java – Master encapsulation techniques for achieving data hiding and code security in Java.
Design Patterns in Java – Dive into common design patterns in Java for solving recurring design problems.
Java OOPs Concepts – A detailed guide on OOP concepts in Java, with examples and quizzes for self-assessment.
Guru99’s Java OOPs Concepts Tutorial includes definitions, coding examples, and scenarios for practical application.
Design Patterns in Java Tutorial covers various design patterns and how they can be implemented in Java.
By understanding and applying Java’s OOP concepts, you can write more efficient, maintainable, and scalable code. So, keep learning and happy coding!
Wrapping Up: Mastering Java OOP Concepts
In this comprehensive guide, we’ve navigated through the world of Java’s Object-Oriented Programming (OOP) concepts. We’ve explored the four pillars of OOP in Java: Encapsulation, Inheritance, Polymorphism, and Abstraction, and how they form the foundation for writing efficient, scalable, and maintainable code in Java.
We started with the basics, understanding the theory behind OOP and the role of classes, objects, methods, and attributes. We then dove into each of the four key OOP concepts, starting with Encapsulation and its role in data protection. Next, we explored Inheritance and how it promotes code reuse and a hierarchical structure. We then ventured into Polymorphism, learning about its ability to allow objects of different types to be processed as a common type. Finally, we delved into Abstraction and how it hides complexity by showing only essential features.
Along the way, we tackled common issues that you might face when implementing these OOP concepts, providing solutions and workarounds for each. We also discussed the application of these concepts in larger projects and the role of design patterns.
Here’s a quick comparison of the OOP concepts we’ve discussed:
Concept | Pros | Cons |
---|---|---|
Encapsulation | Increases data security, promotes modularity | Can make code slightly longer |
Inheritance | Promotes code reuse, represents real-world relationships | Can lead to tightly coupled code |
Polymorphism | Simplifies coding, improves readability and maintainability | Can lead to confusion if not used correctly |
Abstraction | Reduces code complexity, promotes modularity | Can lead to incomplete or incorrect implementation if not handled properly |
Whether you’re new to Java or looking to deepen your understanding of its OOP concepts, we hope this guide has been a valuable resource. The journey of mastering Java’s OOP concepts is a continuous one, filled with learning and exploration. Keep practicing, keep coding, and soon you’ll see these concepts become second nature. Happy coding!