Encapsulation in Java: A Guide to Securing Class Data
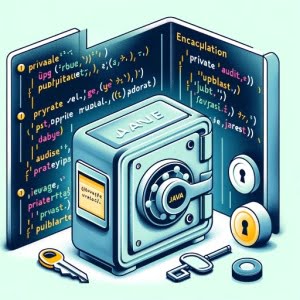
Are you finding it challenging to understand the concept of encapsulation in Java? You’re not alone. Many developers find themselves puzzled when it comes to mastering encapsulation in Java, but we’re here to help.
Think of encapsulation in Java as a protective shell – just like a capsule protects its contents, encapsulation in Java safeguards the data, ensuring it’s not mishandled or misused.
This guide will walk you through the concept of encapsulation in Java, from basic usage to advanced techniques. We’ll cover everything from the basics of encapsulation, its implementation, benefits, to more advanced techniques, as well as potential pitfalls.
So, let’s get started and master encapsulation in Java!
TL;DR: What is Encapsulation in Java?
Encapsulation in Java is a fundamental principle of object-oriented programming. It is defined within a class by assigning
private
to a class variable: Aprivate String name
inpublic class Employee
,. It binds together the data and functions that manipulate the data, hiding it within the class and preventing it from being accessed directly by other classes.
Here’s a simple example:
public class Employee {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
# Output:
# This code defines a class Employee with a private field 'name'.
# The 'getName' and 'setName' methods are the public interfaces to access and modify the 'name' field.
In this example, we’ve defined a class Employee
with a private field name
. The getName
and setName
methods are the public interfaces to access and modify the name
field. This is a basic implementation of encapsulation in Java.
But Java’s encapsulation capabilities go far beyond this. Continue reading for more detailed examples and advanced encapsulation techniques.
Table of Contents
- Understanding Encapsulation in Java
- Diving Deeper: Encapsulation with Inheritance and Polymorphism
- Exploring Alternatives: Abstraction and Interfaces
- Troubleshooting Encapsulation in Java
- Encapsulation in the Context of Object-Oriented Programming
- Applying Encapsulation in Large-Scale Java Projects
- Wrapping Up: Mastering Encapsulation in Java
Understanding Encapsulation in Java
Encapsulation is one of the four fundamental principles of object-oriented programming (OOP). In Java, encapsulation is implemented by combining the data and the methods that manipulate this data into a single unit, which we call a class. The data variables of a class are hidden from other classes, and can only be accessed through the methods of their current class. This is also known as data hiding.
Let’s take a look at a simple example of encapsulation in Java:
public class BankAccount {
private double balance;
public double getBalance() {
return balance;
}
public void deposit(double amount) {
if (amount > 0) {
balance += amount;
}
}
public void withdraw(double amount) {
if (amount <= balance) {
balance -= amount;
}
}
}
# Output:
# This code defines a class BankAccount with a private field 'balance'.
# The 'getBalance', 'deposit', and 'withdraw' methods are the public interfaces to access and modify the 'balance' field.
In this example, the BankAccount
class has a private field balance
. The getBalance
, deposit
, and withdraw
methods are the public interfaces to access and modify the balance
field. The balance
field is hidden and cannot be accessed directly, which is a key aspect of encapsulation.
Advantages of Encapsulation
Encapsulation provides several benefits:
- Data Hiding: The user will not be aware of the inner implementation of the class. It hides the data from the outside world.
- Increased Flexibility: We can make the variables of the class read-only or write-only according to our requirement.
- Reusability: Encapsulation also improves the reusability and easy to change with new requirements.
- Testing code is easy: Encapsulated code is easy to test for unit testing.
Potential Pitfalls
While encapsulation has many benefits, it’s important to be aware of potential pitfalls:
- Over-encapsulation: Too much encapsulation can lead to unnecessary complexity and can make the code harder to read.
- Misuse of Access Modifiers: Incorrect use of access modifiers (public, private, and protected) can lead to problems. For example, making a variable public can allow it to be changed from outside the class, breaking the encapsulation principle.
In conclusion, encapsulation is a powerful tool in Java, but like any tool, it must be used properly to be effective.
Diving Deeper: Encapsulation with Inheritance and Polymorphism
As you become more familiar with Java, you’ll encounter scenarios where encapsulation intersects with other OOP principles like inheritance and polymorphism. These advanced uses of encapsulation provide more flexibility and control over your code.
Encapsulation and Inheritance
Inheritance allows a new class to inherit the properties and methods of an existing class. But how does encapsulation play a role here?
public class Vehicle {
private int speed;
public int getSpeed() {
return speed;
}
public void setSpeed(int speed) {
this.speed = speed;
}
}
public class Car extends Vehicle {
public void increaseSpeed(int increment) {
setSpeed(getSpeed() + increment);
}
}
# Output:
# The Car class inherits from the Vehicle class. It uses the public methods of the Vehicle class to access and modify the 'speed' field.
In the above example, the Car
class inherits from the Vehicle
class. It uses the public methods of the Vehicle
class to access and modify the speed
field. This is a prime example of encapsulation working hand-in-hand with inheritance.
Encapsulation and Polymorphism
Polymorphism allows a child class to share the information and behaviors of a parent class. So how does encapsulation come into play?
public class Animal {
public void sound() {
System.out.println("The animal makes a sound");
}
}
public class Cat extends Animal {
@Override
public void sound() {
System.out.println("The cat meows");
}
}
# Output:
# The Cat class overrides the 'sound' method of the Animal class. This is an example of encapsulation with polymorphism.
In this example, the Cat
class overrides the sound
method of the Animal
class. This is an example of encapsulation with polymorphism.
Pros and Cons of Advanced Encapsulation
Advanced encapsulation techniques offer several benefits:
- Enhanced Code Reusability: Inheritance allows us to reuse code from a parent class, reducing redundancy.
- Code Flexibility: Polymorphism allows child classes to define their unique behaviors, increasing flexibility.
However, there can be potential pitfalls:
- Increased Complexity: The use of advanced encapsulation techniques can make the code more complex and harder to understand for beginners.
- Design Considerations: Incorrect use of inheritance and polymorphism can lead to design issues in your code.
Understanding these advanced encapsulation techniques will enable you to write more efficient and effective Java code.
Exploring Alternatives: Abstraction and Interfaces
While encapsulation is an effective way to achieve data hiding in Java, it’s not the only approach. Other concepts in Java, such as abstraction and interfaces, can also be used to hide data and increase code flexibility.
Abstraction in Java
Abstraction is a process where you show only relevant data and hide unnecessary details of an object from the user. Let’s look at an example:
abstract class Shape {
abstract void draw();
}
class Rectangle extends Shape {
void draw() {
System.out.println("Drawing rectangle");
}
}
# Output:
# The 'Shape' class is abstract and contains an abstract method 'draw'. The 'Rectangle' class extends 'Shape' and provides an implementation for the 'draw' method.
In this example, the Shape
class is abstract and contains an abstract method draw
. The Rectangle
class extends Shape
and provides an implementation for the draw
method. This is an example of data hiding through abstraction.
Interfaces in Java
An interface in Java is a blueprint of a class. It has static constants and abstract methods. The interface in Java is a mechanism to achieve abstraction.
interface Drawable {
void draw();
}
class Circle implements Drawable {
public void draw() {
System.out.println("Drawing circle");
}
}
# Output:
# The 'Drawable' interface contains a method 'draw'. The 'Circle' class implements 'Drawable' and provides an implementation for the 'draw' method.
In this example, the Drawable
interface contains a method draw
. The Circle
class implements Drawable
and provides an implementation for the draw
method. This is an example of data hiding through interfaces.
Weighing the Options
Abstraction and interfaces offer several benefits:
- Code Flexibility: They allow you to hide details and show only the functionality to the user.
- Multiple Inheritance: A class can implement multiple interfaces, overcoming Java’s restriction on multiple inheritance.
However, there can be potential drawbacks:
- Design Complexity: Incorrect use of abstraction and interfaces can lead to design issues in your code.
When deciding between encapsulation, abstraction, and interfaces, consider the needs of your code and which method will provide the most efficient and effective solution.
Troubleshooting Encapsulation in Java
As with any programming concept, implementing encapsulation in Java can sometimes lead to errors or obstacles. Let’s discuss some common issues and their solutions.
Common Errors
One common mistake is trying to access a private field directly from another class. This will lead to a compile-time error. For example:
public class Main {
public static void main(String[] args) {
BankAccount account = new BankAccount();
account.balance = 1000; // This will cause a compile-time error
}
}
# Output:
# Error: The field BankAccount.balance is not visible
In this example, trying to access the balance
field directly from the Main
class causes a compile-time error. The solution is to use the public methods provided by the BankAccount
class to interact with the balance
field.
public class Main {
public static void main(String[] args) {
BankAccount account = new BankAccount();
account.deposit(1000); // This is the correct way to modify the balance
}
}
# Output:
# No errors. The balance is successfully modified.
Best Practices and Optimization
When implementing encapsulation in Java, here are some best practices to follow:
- Use the ‘private’ keyword: Make the data members private so that they cannot be accessed directly from outside the class.
- Provide public setter and getter methods: To provide access to the data members, provide public setter and getter methods.
- Validate the data: In the setter methods, validate the data before setting the values.
By following these best practices, you can ensure that your encapsulation implementation is robust, secure, and efficient.
Encapsulation in the Context of Object-Oriented Programming
To truly understand encapsulation in Java, we need to delve into its roots in the broader context of object-oriented programming (OOP). At its core, encapsulation is one of the four fundamental principles of OOP, along with inheritance, polymorphism, and abstraction.
Why Encapsulation Matters
Encapsulation is often referred to as the ‘shield’ or ‘guardian’ of our data. It provides a way to protect our data from accidental corruption due to errors or bad code. By hiding our data, encapsulation ensures that it cannot be changed unpredictably or unknowingly by another part of the program.
public class DataShield {
private int secretData;
public int getSecretData() {
return secretData;
}
public void setSecretData(int secretData) {
this.secretData = secretData;
}
}
# Output:
# This code defines a class DataShield with a private field 'secretData'.
# The 'getSecretData' and 'setSecretData' methods are the public interfaces to access and modify the 'secretData' field.
In this example, the DataShield
class encapsulates the secretData
field. This field is hidden and can’t be accessed directly, protecting it from accidental corruption.
Encapsulation’s Role in Code Robustness and Maintainability
Encapsulation enhances the robustness and maintainability of code in several ways:
- Data Integrity: By controlling how data can be accessed or modified, encapsulation ensures the integrity of the data.
- Code Organization: Encapsulation helps in organizing the code into clear, manageable chunks. Each class is a self-contained unit that contains its own data and methods.
- Code Flexibility: With encapsulation, the specific implementation of a class can be changed without affecting other parts of the program that use the class.
In conclusion, encapsulation is a powerful concept in OOP that plays a critical role in creating robust, maintainable, and flexible code.
Applying Encapsulation in Large-Scale Java Projects
Once you’ve mastered the basics of encapsulation, it’s time to apply these principles in larger Java projects. In real-world applications, encapsulation is often used in conjunction with other OOP principles to create robust, flexible, and maintainable software.
Encapsulation in Action: A Real-World Scenario
Consider a large e-commerce application. Here, encapsulation could be used to protect critical data such as customer details, payment information, and order history. Each of these data sets could be encapsulated in their own classes, with specific methods to access and modify the data.
public class Customer {
private String name;
private String address;
// other private fields
// public getter and setter methods
}
public class Order {
private int orderId;
private Date orderDate;
// other private fields
// public getter and setter methods
}
# Output:
# The 'Customer' and 'Order' classes encapsulate their respective data fields, protecting them from unauthorized access.
In this example, the Customer
and Order
classes encapsulate their respective data fields, protecting them from unauthorized access. This not only ensures data integrity but also makes the code easier to manage and maintain.
Complementary Concepts to Encapsulation
In a typical Java project, encapsulation is often used along with other OOP principles like inheritance, polymorphism, and abstraction. These principles work together to create a cohesive, well-structured application.
For instance, inheritance allows you to create a general Product
class and then create specific product classes (like Book
, Electronics
, etc.) that inherit from the Product
class. Polymorphism allows these specific product classes to have their own implementations of the methods defined in the Product
class. Abstraction can be used to hide the complexity of these implementations from the user.
Further Resources for Mastering Java Encapsulation
To deepen your understanding of encapsulation and its applications in Java, here are a few resources that offer in-depth information:
- Strengthening Your Java Skills: OOPs Concepts Edition – Understand the role of Object Oriented Programming in Java.
Using Inheritance in Java – Master inheritance for building flexible and extensible Java applications.
Dependency Injection in Java – Learn about dependency injection in Java for achieving loose coupling and high cohesion.
Oracle’s Java Tutorials cover all aspects of Java programming, including encapsulation.
Geeks for Geeks’ Java Programming Language resources offers detailed guides on many Java topics.
Baeldung’s Guide to Java Classes provides a deep dive into Java classes and encapsulation.
Wrapping Up: Mastering Encapsulation in Java
In this comprehensive guide, we’ve delved deep into the world of encapsulation in Java, a fundamental principle of object-oriented programming that binds together data and the methods that manipulate it.
We began with the basics, explaining the concept of encapsulation and demonstrating its implementation through simple examples. We then progressed to more advanced usage, exploring how encapsulation interacts with other OOP principles like inheritance and polymorphism. We also discussed potential pitfalls and best practices to keep in mind when implementing encapsulation.
We tackled common issues that you might encounter when using encapsulation, providing practical solutions to these challenges. Furthermore, we explored alternative approaches to data hiding, such as abstraction and interfaces, giving you a broader perspective on data protection in Java.
Here’s a quick comparison of the methods we’ve discussed:
Method | Ease of Use | Flexibility | Complexity |
---|---|---|---|
Encapsulation | High | Moderate | Low |
Abstraction | Moderate | High | Moderate |
Interfaces | High | High | High |
Whether you’re a beginner just starting out with encapsulation or an intermediate programmer looking to hone your skills, we hope this guide has deepened your understanding of encapsulation in Java and its importance in creating robust, maintainable code.
Mastering encapsulation is a crucial step in your journey as a Java programmer. With this knowledge at your disposal, you’re well equipped to write efficient, secure, and organized code. Happy coding!