Dependency Injection in Java: A Guide to Managed Objects
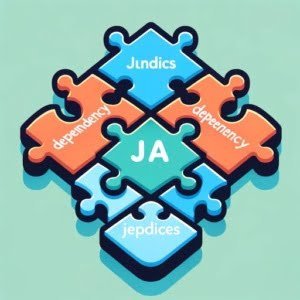
Are you finding it challenging to manage dependencies in your Java applications? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a skilled architect, you can use dependency injection in Java to build flexible and maintainable software. These techniques can help you manage dependencies in your code, making it more modular, testable, and easy to maintain.
This guide will walk you through the process of using dependency injection in Java, from basic use to advanced techniques. We’ll explore everything from the core concepts of dependency injection, how to use it in your Java applications, to more advanced techniques and alternative approaches.
So, let’s dive in and start mastering dependency injection in Java!
TL;DR: How Do I Implement Dependency Injection in Java?
Dependency injection in Java can be implemented using frameworks like Spring or Guice. The syntax will depend on the framewok being used, however dependencies can normally be injected by preceding an
@
before the annotation, for example@Autowired
. It’s a technique that helps manage dependencies within your code, making it more modular, testable, and maintainable.
Here’s a simple example using Spring:
@Autowired
private MyService myService;
In this example, we’re using the @Autowired
annotation provided by Spring. This annotation is used to automatically inject dependencies into your Java classes. In this case, an instance of MyService
is automatically created and injected where it’s needed.
This is just a basic way to implement dependency injection in Java, but there’s much more to learn about this powerful technique. Continue reading for a deeper understanding and more advanced usage scenarios.
Table of Contents
- Getting Started with Dependency Injection in Java
- Diving Deeper: Advanced Dependency Injection in Java
- Exploring Alternatives: Beyond Spring Framework
- Troubleshooting Dependency Injection in Java
- Unpacking Dependency Injection: The Fundamentals
- Taking it Further: Dependency Injection in Larger Java Projects
- Wrapping Up: Dependency Injection in Java
Getting Started with Dependency Injection in Java
Dependency injection may seem complex at first, but once you understand the basics, it’s a powerful tool to have in your Java toolkit. Let’s start by implementing dependency injection using the Spring framework.
Step-by-Step Guide to Dependency Injection in Java
- Create a new Java class: Let’s start by creating a simple service class. This class will have a method that we’ll use to demonstrate dependency injection.
public class MyService {
public void serve() {
System.out.println("Service is being provided");
}
}
- Inject the dependency using Spring: Now, let’s inject an instance of
MyService
into another class using the@Autowired
annotation. The@Autowired
annotation tells Spring to automatically create an instance ofMyService
and use it wherever it’s needed.
public class MyController {
@Autowired
private MyService myService;
public void executeService() {
myService.serve();
}
}
In the MyController
class, we’re using the @Autowired
annotation to automatically inject an instance of MyService
. Then, we’re using this instance in the executeService
method.
- Run the application: When we run the application and call the
executeService
method, we’ll see the following output:
// Output:
// Service is being provided
This output shows that the serve
method of MyService
is being called, demonstrating that the dependency was successfully injected.
This is a basic example of how to implement dependency injection in Java using the Spring framework. It’s a simple yet powerful technique that can make your code more flexible and maintainable.
Diving Deeper: Advanced Dependency Injection in Java
After getting a grip on the basics, it’s time to explore more complex uses of dependency injection in Java. We’ll focus on three advanced techniques: constructor injection, setter injection, and field injection.
Constructor Injection in Java
Constructor injection is a type of dependency injection where the container invokes a class constructor with arguments, each representing a dependency.
public class MyController {
private MyService myService;
public MyController(MyService myService) {
this.myService = myService;
}
public void executeService() {
myService.serve();
}
}
In this example, we’re injecting MyService
into MyController
through the constructor. When Spring creates an instance of MyController
, it automatically provides an instance of MyService
.
Setter Injection in Java
Setter injection is when the container calls setter methods on your class, after invoking a no-argument constructor or no-argument static factory method to instantiate your bean.
public class MyController {
private MyService myService;
public void setMyService(MyService myService) {
this.myService = myService;
}
public void executeService() {
myService.serve();
}
}
In this example, we’re using a setter method to inject MyService
. Spring calls the setMyService
method and provides an instance of MyService
.
Field Injection in Java
Field injection is when the container directly assigns the dependencies to fields.
public class MyController {
@Autowired
private MyService myService;
public void executeService() {
myService.serve();
}
}
In this example, we’re using field injection. Spring automatically assigns an instance of MyService
to the myService
field.
Each of these methods has its own advantages and use cases. It’s important to understand the differences and choose the best method for your specific needs. As you continue to work with dependency injection in Java, you’ll develop a sense of which method to use in different situations.
Exploring Alternatives: Beyond Spring Framework
While the Spring framework is a popular choice for implementing dependency injection in Java, it’s not the only option. Let’s explore some alternative approaches, including using the Guice framework and manual dependency injection.
Dependency Injection with Guice
Guice, developed by Google, is a lightweight dependency injection framework for Java. It uses annotations to configure dependencies, similar to Spring, but it’s more lightweight and focused solely on dependency injection.
Here’s a simple example of how to use Guice:
public class MyController {
@Inject
private MyService myService;
public void executeService() {
myService.serve();
}
}
In this example, we’re using the @Inject
annotation provided by Guice. Similar to the @Autowired
annotation in Spring, @Inject
tells Guice to automatically create an instance of MyService
and inject it into MyController
.
Manual Dependency Injection
Manual dependency injection is another alternative. It involves creating and passing dependencies manually, without using a specific framework. This can give you more control over your code, but it can also make your code more complex and harder to manage.
Here’s a simple example of manual dependency injection:
public class MyController {
private MyService myService;
public MyController() {
this.myService = new MyService();
}
public void executeService() {
myService.serve();
}
}
In this example, we’re creating an instance of MyService
manually in the MyController
constructor. This is a simple form of manual dependency injection, but it can get more complex as your application grows.
Each of these alternative approaches has its own benefits and drawbacks. Guice can be a good choice if you’re looking for a lightweight, focused dependency injection framework, while manual dependency injection can give you more control over your code. However, manual dependency injection can also make your code more complex and harder to manage, especially in larger applications. It’s important to consider these factors when deciding which approach to use.
Troubleshooting Dependency Injection in Java
While dependency injection in Java is a powerful tool, it’s not without its challenges. Let’s discuss some common issues you may encounter and how to resolve them.
Handling Null and Optional Dependencies
One common issue when implementing dependency injection in Java is handling null or optional dependencies. For instance, you might have a situation where a dependency isn’t always required.
In Spring, you can handle this by using the @Autowired(required = false)
annotation. This tells Spring that the dependency is optional and it’s okay if no matching bean is found.
@Autowired(required = false)
private MyService myService;
In this example, Spring will inject MyService
if a matching bean is found. If not, myService
will be null.
Dealing with Multiple Beans of the Same Type
Another common issue is dealing with multiple beans of the same type. If you have more than one bean of the same type, Spring might not know which one to inject.
You can handle this by using the @Qualifier
annotation to specify which bean to inject.
@Autowired
@Qualifier("myServiceOne")
private MyService myService;
In this example, Spring will inject the bean named myServiceOne
.
Managing Circular Dependencies
Circular dependencies can be a tricky issue. This happens when two beans depend on each other, creating a circular dependency.
Spring can handle circular dependencies for setter and field injection, but not for constructor injection. If you encounter a circular dependency with constructor injection, you might need to redesign your classes to avoid the circular dependency.
These are just a few of the common issues you might encounter when implementing dependency injection in Java. By understanding these issues and how to resolve them, you can use dependency injection more effectively in your Java applications.
Unpacking Dependency Injection: The Fundamentals
Dependency injection is more than just a coding technique; it’s a fundamental concept in software design that promotes loose coupling and code modularity. To fully grasp the power of dependency injection in Java, we need to delve into its conceptual underpinnings.
Understanding Dependency Injection
At its core, dependency injection is about managing dependencies between objects. In a complex application, objects often depend on each other to perform their tasks. Dependency injection is a way to provide an object with the other objects it depends on.
For example, consider a Car
object that depends on an Engine
object to function. Instead of creating the Engine
inside the Car
class, we can inject it from the outside. This is the essence of dependency injection.
public class Car {
private Engine engine;
public Car(Engine engine) {
this.engine = engine;
}
public void drive() {
engine.start();
System.out.println("Car is driving");
}
}
// Output:
// Engine is starting
// Car is driving
In this example, the Car
class depends on the Engine
class. Instead of creating an Engine
inside Car
, we’re injecting it through the constructor. This makes our Car
class more flexible and easier to test.
The Principle of Inversion of Control (IoC)
Inversion of Control (IoC) is a key principle related to dependency injection. IoC is about inverting the control of creating and managing objects. Instead of an object creating and managing its dependencies, these responsibilities are handed over to an external entity (like a framework).
Dependency injection is one way to implement IoC. By injecting dependencies, we’re inverting the control of managing these dependencies. This makes our code more modular, flexible, and testable.
By understanding these fundamentals, you can better leverage the power of dependency injection in your Java applications. As you advance in your knowledge, you’ll begin to see how these principles apply in different scenarios and how they can help you write better, more maintainable code.
Taking it Further: Dependency Injection in Larger Java Projects
As your Java projects grow in size and complexity, dependency injection becomes increasingly valuable. It helps manage dependencies across different modules, making your code more modular, testable, and maintainable. But as you delve deeper into dependency injection, you might encounter related topics like Aspect-Oriented Programming (AOP).
Aspect-Oriented Programming (AOP) and Dependency Injection
AOP is a programming paradigm that aims to increase modularity by allowing the separation of cross-cutting concerns. It does so by adding additional behavior to existing code without modifying the code itself. Instead, you can declare which code is modified statically or dynamically.
Dependency injection works hand in hand with AOP, as it provides a way to inject these cross-cutting concerns into existing code. For instance, you might use AOP to handle logging or security concerns across your application, and then use dependency injection to inject these concerns where they’re needed.
@Component
@Aspect
public class LoggingAspect {
@Before("execution(* com.example.myapp.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Executing: " + joinPoint.getSignature());
}
}
In this example, we’re using AOP to log each method execution in our application. The @Aspect
annotation marks this class as an aspect, and the @Before
annotation tells Spring to execute this method before each method in our application. When we run our application, we’ll see a log message for each method execution.
// Output:
// Executing: com.example.myapp.MyService.serve()
// Executing: com.example.myapp.MyController.executeService()
This is just a simple example, but AOP and dependency injection can be used to handle more complex cross-cutting concerns in larger Java applications.
Further Resources for Dependency Injection in Java
If you’re interested in learning more about dependency injection in Java, here are a few resources that you might find helpful:
- Beginner’s Guide to Java OOPs Concepts – Discover the concept of classes and objects in Java programming.
Java Encapsulation Basics – Learn how to use access modifiers like private, public, and protected to control data access.
Java Abstract Class vs Interface – Understand when to use abstract classes and interfaces based on design requirements.
Java Dependency Injection design pattern example tutorial – A comprehensive tutorial on dependency injection in Java.
Introduction to Spring AOP – A detailed guide on using AOP with Spring, a common use case for dependency injection.
Google Guice – The official repository for Guice, Google’s lightweight dependency injection framework.
Wrapping Up: Dependency Injection in Java
In this comprehensive guide, we’ve journeyed through the world of dependency injection in Java, a powerful tool for managing dependencies and making your code more modular, testable, and maintainable.
We began with the basics, demonstrating how to implement dependency injection in Java using the Spring framework. We then ventured into more advanced territory, exploring techniques such as constructor injection, setter injection, and field injection. We also covered alternative approaches to dependency injection, including using the Guice framework and manual dependency injection.
Along the way, we tackled common issues you might encounter when implementing dependency injection in Java, such as handling null and optional dependencies, dealing with multiple beans of the same type, and managing circular dependencies. We provided solutions and workarounds for each issue, helping you navigate these challenges with confidence.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
Spring | High | Moderate | Large applications, wide variety of use cases |
Guice | Moderate | Low | Lightweight applications, focused on dependency injection |
Manual | High | High | Gives more control, suitable for smaller applications |
Whether you’re just starting out with dependency injection in Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of dependency injection and its capabilities.
With its balance of flexibility and control, dependency injection is a powerful tool for managing dependencies in your Java applications. Now, you’re well equipped to enjoy those benefits. Happy coding!