Java Abstract Class vs Interface: A Detailed Comparison
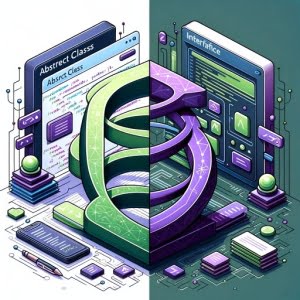
Ever found yourself puzzled about when to use an abstract class and when to use an interface in Java? You’re not alone. Many developers find themselves in a quandary when it comes to choosing between abstract classes and interfaces in Java.
Think of Java’s abstract classes and interfaces as different tools in your coding toolbox. Knowing when to use which tool can make your code more efficient and readable.
This guide will help you understand the differences, similarities, and use cases for both abstract classes and interfaces in Java. We’ll delve into the basics, explore advanced use cases, and even discuss alternative approaches.
So, let’s dive in and start mastering the use of Java abstract classes and interfaces!
TL;DR: What is the Difference Between an Abstract Class and an Interface in Java?
The key difference between an abstract class,
abstract class AbstractClass
, and an interface,interface Interface
, in Java lies in their structure and usage. An interface can only declare methods (which are implicitly abstract) and constants. On the other hand, an abstract class can have members, methods (abstract or not), and constructors. However, the choice between the two often depends on the design requirements.
Here’s a simple example:
// Abstract Class
abstract class AbstractClass {
abstract void abstractMethod();
void nonAbstractMethod() {
System.out.println("This is a non-abstract method");
}
}
// Interface
interface Interface {
void interfaceMethod();
}
// Output:
// This is a non-abstract method
In this example, the abstract class AbstractClass
has an abstract method abstractMethod()
and a non-abstract method nonAbstractMethod()
. The interface Interface
only has one method interfaceMethod()
. The abstract class can have a method with an implementation (nonAbstractMethod()
), but the interface cannot.
This is just a basic comparison between abstract classes and interfaces in Java. There’s much more to learn about when to use each one and their specific use cases. Continue reading for a more detailed comparison and examples.
Table of Contents
- Understanding Abstract Classes and Interfaces in Java
- Delving Deeper: Abstract Classes vs Interfaces in Java
- Choosing Between Abstract Class and Interface: Scenario-Based Discussions
- Troubleshooting: Common Issues with Abstract Classes and Interfaces
- Best Practices for Using Abstract Classes and Interfaces
- Understanding Abstraction and Interfaces in Java
- Advancing Your Java Skills: Abstract Classes and Interfaces
- Wrapping Up: Abstract Classes vs Interfaces
Understanding Abstract Classes and Interfaces in Java
In Java, both abstract classes and interfaces are used to achieve abstraction, a key concept in object-oriented programming. However, they are used in different scenarios and come with their own set of rules and characteristics.
Abstract Classes in Java
An abstract class in Java is a class that cannot be instantiated, meaning you cannot create an object of an abstract class. It can contain abstract (method without a body) as well as non-abstract methods (methods with a body).
Here’s a basic example of an abstract class:
abstract class Vehicle {
abstract void run();
}
class Car extends Vehicle {
void run() {
System.out.println("Car is running");
}
}
public class Main {
public static void main(String[] args) {
Vehicle vehicle = new Car();
vehicle.run();
}
}
// Output:
// Car is running
In this example, Vehicle
is an abstract class with an abstract method run()
. Car
is a class that extends Vehicle
and provides the implementation for the run()
method. When we run the Main
class, it prints ‘Car is running’.
Interfaces in Java
An interface in Java is a completely abstract class that can only have abstract methods. It allows us to achieve full abstraction and multiple inheritance.
Here’s a basic example of an interface:
interface Animal {
void sound();
}
class Dog implements Animal {
public void sound() {
System.out.println("Dog barks");
}
}
public class Main {
public static void main(String[] args) {
Animal animal = new Dog();
animal.sound();
}
}
// Output:
// Dog barks
In this example, Animal
is an interface with an abstract method sound()
. Dog
is a class that implements Animal
and provides the implementation for the sound()
method. When we run the Main
class, it prints ‘Dog barks’.
Delving Deeper: Abstract Classes vs Interfaces in Java
As we move beyond the basics, let’s discuss some of the more nuanced differences between abstract classes and interfaces in Java, such as multiple inheritance, type definition, and implementation.
Multiple Inheritance
One of the key differences between abstract classes and interfaces involves multiple inheritance. In Java, a class can inherit from only one superclass, but it can implement multiple interfaces.
Here’s an example illustrating this difference:
interface Interface1 {
void method1();
}
interface Interface2 {
void method2();
}
class MyClass implements Interface1, Interface2 {
public void method1() {
System.out.println("Implemented method1");
}
public void method2() {
System.out.println("Implemented method2");
}
}
public class Main {
public static void main(String[] args) {
MyClass myClass = new MyClass();
myClass.method1();
myClass.method2();
}
}
// Output:
// Implemented method1
// Implemented method2
In this example, MyClass
implements two interfaces, Interface1
and Interface2
, demonstrating multiple inheritance. This would not be possible with abstract classes.
Type Definition
Another difference lies in how abstract classes and interfaces are used for type definition. An abstract class defines a base type for its subclasses, while an interface defines a contract that implementing classes need to follow.
Implementation
Finally, abstract classes can provide a default implementation for some methods, while interfaces can’t (until Java 8 introduced default methods). This means you can create a method with a body in an abstract class, but not in an interface.
abstract class AbstractClass {
void implementedMethod() {
System.out.println("This method is implemented");
}
abstract void abstractMethod();
}
class MyClass extends AbstractClass {
void abstractMethod() {
System.out.println("Implemented abstract method");
}
}
public class Main {
public static void main(String[] args) {
MyClass myClass = new MyClass();
myClass.implementedMethod();
myClass.abstractMethod();
}
}
// Output:
// This method is implemented
// Implemented abstract method
In this example, AbstractClass
provides a default implementation for implementedMethod()
, which can be used by any class that extends it. abstractMethod()
, on the other hand, is abstract and must be implemented by any class that extends AbstractClass
.
Choosing Between Abstract Class and Interface: Scenario-Based Discussions
As we delve deeper into the world of Java, it’s crucial to understand when to use an abstract class vs an interface based on different scenarios and requirements. Let’s explore this with some examples.
Scenario 1: Need for Multiple Inheritance
In Java, if your class needs to inherit behavior from multiple sources, interfaces are your best bet since Java doesn’t support multiple inheritance with classes.
interface Flyable {
void fly();
}
interface Driveable {
void drive();
}
class FlyingCar implements Flyable, Driveable {
public void fly() {
System.out.println("FlyingCar is flying");
}
public void drive() {
System.out.println("FlyingCar is driving");
}
}
public class Main {
public static void main(String[] args) {
FlyingCar flyingCar = new FlyingCar();
flyingCar.fly();
flyingCar.drive();
}
}
// Output:
// FlyingCar is flying
// FlyingCar is driving
In this example, FlyingCar
implements two interfaces, Flyable
and Driveable
, thus inheriting behavior from both.
Scenario 2: Shared Constants
If you need to share constants (final variables) among several classes, using an interface can be beneficial.
interface Constants {
int MAX_SPEED = 120;
}
class Car implements Constants {
void drive() {
System.out.println("Driving at max speed: " + MAX_SPEED);
}
}
public class Main {
public static void main(String[] args) {
Car car = new Car();
car.drive();
}
}
// Output:
// Driving at max speed: 120
In this example, the Car
class implements the Constants
interface and uses the MAX_SPEED
constant.
Scenario 3: Base Class with Common Implementation
If you have a base class with a common implementation that other classes can extend, an abstract class is a good choice.
abstract class Animal {
abstract void sound();
void breathe() {
System.out.println("Breathing...");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Dog barks");
}
}
public class Main {
public static void main(String[] args) {
Dog dog = new Dog();
dog.sound();
dog.breathe();
}
}
// Output:
// Dog barks
// Breathing...
In this example, Dog
extends the Animal
abstract class, inheriting the breathe()
method and providing its own implementation of the sound()
method.
Troubleshooting: Common Issues with Abstract Classes and Interfaces
As with any aspect of programming, working with abstract classes and interfaces in Java can sometimes lead to unexpected issues. Let’s discuss some common problems you might encounter and how to resolve them.
Issue 1: Overriding Abstract Methods
One common mistake is forgetting to provide an implementation for all abstract methods in a class that extends an abstract class or implements an interface.
abstract class AbstractClass {
abstract void abstractMethod();
}
class MyClass extends AbstractClass {
// No implementation for abstractMethod
}
// Output:
// Error: MyClass is not abstract and does not override abstract method abstractMethod() in AbstractClass
In this example, MyClass
extends AbstractClass
but does not provide an implementation for abstractMethod()
, leading to a compile-time error. The solution is to ensure that all abstract methods are implemented in the subclass.
Issue 2: Implementing Multiple Interfaces with Default Methods
Another issue arises when a class implements multiple interfaces, each with default methods of the same name. This leads to a ‘diamond problem’.
interface Interface1 {
default void defaultMethod() {
System.out.println("Interface1's default method");
}
}
interface Interface2 {
default void defaultMethod() {
System.out.println("Interface2's default method");
}
}
class MyClass implements Interface1, Interface2 {
// Which defaultMethod to use?
}
// Output:
// Error: class MyClass inherits unrelated defaults for defaultMethod() from types Interface1 and Interface2
In this example, MyClass
implements Interface1
and Interface2
, both of which have a defaultMethod()
. This leads to a compile-time error. The solution is to override the conflicting method in the class that implements the interfaces.
Best Practices for Using Abstract Classes and Interfaces
- Use interfaces for multiple inheritance: Since Java doesn’t support multiple inheritance with classes, use interfaces when you need to inherit behavior from multiple sources.
Use abstract classes when subclasses share code: When several classes should share a common piece of code, consider using an abstract class and defining that shared code in the abstract class.
Use interfaces for constants: If several classes need to share constants, define them in an interface and implement that interface in the classes.
Use interfaces to define a contract: If you want to define a contract that several classes should follow, use an interface.
Understanding Abstraction and Interfaces in Java
To fully grasp the differences between abstract classes and interfaces in Java, it’s crucial to understand the fundamental concepts of abstraction and interfaces in the realm of object-oriented programming.
Abstraction in Java
Abstraction is a process of hiding the implementation details and showing only the functionality to the user. In other words, it deals with the outside view of an object (interface).
Abstraction can be achieved in two ways:
- Abstract Class (0 to 100% abstraction)
- Interface (100% abstraction)
An abstract class can have abstract and non-abstract methods (method with a body). But, in the case of the interface, it can have only abstract methods until Java 7. Since Java 8, it can have default and static methods too.
Interfaces in Java
An interface in Java is a blueprint of a class or you can say it is a collection of abstract methods and static constants. In an interface, each method is public and abstract but it does not contain any constructor. Along with abstraction, interface also helps to achieve multiple inheritance in Java.
interface Printable {
void print();
}
class A6 implements Printable {
public void print() {
System.out.println("Hello");
}
public static void main(String args[]) {
A6 obj = new A6();
obj.print();
}
}
// Output:
// Hello
In this example, we have created an interface named Printable
. That interface is implemented by class A6
. So, the print()
method of A6
class needs to provide the implementation for the print()
method of Printable
interface.
These fundamental concepts are the building blocks for understanding the usage and benefits of abstract classes and interfaces in Java.
Advancing Your Java Skills: Abstract Classes and Interfaces
Understanding the differences between abstract classes and interfaces in Java is more than just academic knowledge. It’s a practical skill that can significantly improve the efficiency of your code and your effectiveness as a Java programmer.
Enhanced Code Efficiency
Knowing when to use an abstract class or an interface can help you write more efficient code. For instance, using an interface allows you to achieve multiple inheritance, a feature not directly available in Java with classes. This can lead to more streamlined and efficient code structures.
Improved Programming Skills
Mastering the use of abstract classes and interfaces can also elevate your overall Java programming skills. These concepts are fundamental to object-oriented programming, and understanding them can provide a deeper insight into how Java works under the hood.
Exploring Related Concepts: Inheritance and Polymorphism
Once you’ve grasped abstract classes and interfaces, you can delve into related topics like inheritance and polymorphism. Inheritance is a mechanism whereby one class acquires the properties (fields and methods) of another. With the use of inheritance, information is made manageable in a hierarchical order.
Polymorphism, on the other hand, allows us to perform a single action in different ways. In Java, we use method overloading and method overriding to achieve polymorphism. Another example of polymorphism is an interface and its implementations.
Further Resources for Mastering Java Abstract Classes and Interfaces
To continue your learning journey, here are a few resources that provide a more in-depth look into abstract classes, interfaces, and related concepts in Java:
- Getting Started with Java Object Oriented Programming – Learn about constructors, their role in Java OOP, and more.
Java DI Basics – Understand dependency injection frameworks like Spring for managing object dependencies.
Using Abstract Classes in Java – Master abstract classes for achieving code reuse and flexibility in Java applications
Oracle’s Java Tutorials provides comprehensive tutorials on all aspects of the language.
Baeldung’s Guide on Java Interfaces dives deep into the concept of interfaces in Java.
GeeksforGeeks’ Java Programming Language section offers a wide range of tutorials and articles on Java.
Wrapping Up: Abstract Classes vs Interfaces
In this comprehensive guide, we’ve dissected the differences and similarities between Java’s abstract classes and interfaces, aiming to equip you with the knowledge to use these powerful tools effectively in your programming journey.
We began with the basics, explaining what abstract classes and interfaces are in Java, complete with code examples demonstrating their basic use. We then moved on to more advanced concepts, discussing the differences between abstract classes and interfaces, such as multiple inheritance, type definition, and implementation.
Next, we delved into alternative approaches based on different scenarios and requirements, providing you with a nuanced understanding of when to use an abstract class vs an interface. We also tackled common issues one might encounter when using abstract classes and interfaces, offering solutions and best practices to guide you.
Here’s a quick comparison of abstract classes and interfaces in Java:
Feature | Abstract Class | Interface |
---|---|---|
Multiple Inheritance | No | Yes |
Default Methods | Yes | No (before Java 8) |
Type Definition | Base type for subclasses | Contract for implementing classes |
Whether you’re a beginner just starting to explore Java, or an experienced programmer looking to deepen your understanding, we hope this guide has shed light on the intricacies of abstract classes and interfaces in Java. Remember, choosing between an abstract class and an interface is not about which is better overall, but which is better suited for your specific needs in a given situation.
With this knowledge, you’re now better equipped to write more efficient and effective Java code. Keep exploring, keep coding, and keep learning!