Bash ‘Eval’ Command: Command Execution in Shell Script
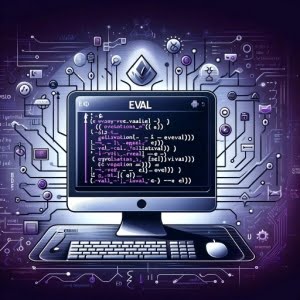
Are you finding the bash eval command a bit perplexing? You’re not alone. Many developers find themselves entangled when it comes to using the bash eval command, but we’re here to untangle the knots.
Think of the bash eval command as a master puppeteer, allowing you to manipulate and execute commands in bash with precision and flexibility. It’s a powerful tool in your bash scripting arsenal, providing you with greater control over command execution.
This guide will walk you through the ins and outs of the bash eval command, from basic usage to advanced techniques. We’ll cover everything from the fundamentals of bash scripting to more complex scenarios, as well as alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering the bash eval command!
TL;DR: What is the Bash Eval Command and How Do I Use It?
The bash
eval
command is a built-in function that allows you to execute arguments as a bash command. To use it, you must first define a command such asgreeting='echo Hello World
. Then you can call the new command witheval $greeeting
. It’s a powerful tool that can help you manipulate and execute commands in bash more efficiently.
Here’s a simple example:
command='ls -l'
eval $command
# Output:
# total 0
# drwxr-xr-x 2 user staff 64 Feb 2 10:24 Desktop
# drwxr-xr-x 3 user staff 96 Feb 2 10:24 Documents
# drwxr-xr-x 3 user staff 96 Feb 2 10:24 Downloads
# drwxr-xr-x 5 user staff 160 Feb 2 10:24 Library
# drwxr-xr-x 2 user staff 64 Feb 2 10:24 Movies
# drwxr-xr-x 2 user staff 64 Feb 2 10:24 Music
# drwxr-xr-x 2 user staff 64 Feb 2 10:24 Pictures
# drwxr-xr-x 5 user staff 160 Feb 2 10:24 Public
In this example, we’ve assigned the ls -l
command to a variable named command
. We then use eval $command
to execute the command stored in the variable. The output is a long listing (-l
) of the current directory.
This is just a basic way to use the bash eval command, but there’s much more to learn about command execution in bash. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding Bash Eval: A Beginner’s Guide
- Leveraging Bash Eval for Complex Scenarios
- Exploring Alternatives to Bash Eval
- Troubleshooting Bash Eval: Common Issues and Solutions
- Bash Scripting and Command Execution Fundamentals
- The Relevance of Bash Eval in Shell Scripting and Automation
- Wrapping Up: Mastering Bash Eval for Command Execution
Understanding Bash Eval: A Beginner’s Guide
The bash eval command is a built-in function that takes arguments and executes them as a bash command. It’s a powerful tool that can make your bash scripts more dynamic and adaptable. But with great power comes great responsibility, so it’s important to understand how it works and how to use it safely.
Let’s start with a simple example:
name='Alice'
greeting='echo Hello, $name'
eval $greeting
# Output:
# Hello, Alice
In this example, we first assign the string ‘Alice’ to a variable named name
. Then, we create a second variable greeting
that contains a command as a string, which is ‘echo Hello, $name’. Notice that we’re using the $name
variable inside the string. When we use eval $greeting
, the eval command first expands $greeting
into the string ‘echo Hello, $name’, then it executes this string as a command. As a result, it prints ‘Hello, Alice’ to the console.
This is a basic example of how eval works. It shows that eval can be used to execute commands stored in variables, which can be very useful in bash scripting. However, it’s important to remember that eval is a powerful command that should be used with caution. It executes whatever it’s given, which can lead to unexpected behavior if you’re not careful. It’s crucial to ensure that the input to eval is controlled and safe to avoid potential security issues.
Leveraging Bash Eval for Complex Scenarios
As you become more comfortable with the bash eval command, you can start to leverage it for more complex scenarios. One such scenario involves using eval with variable substitution.
Consider the following example:
var1='Hello'
var2='World'
command='echo $var1, $var2'
eval $command
# Output:
# Hello, World
In this code block, we have two variables var1
and var2
holding the strings ‘Hello’ and ‘World’ respectively. The command
variable holds a string that references these two variables. When we use eval $command
, the bash eval command first substitutes $var1
and $var2
with their respective values, and then executes the resulting command, printing ‘Hello, World’ to the console.
This example illustrates how you can use eval to dynamically construct and execute commands in bash. This technique can be particularly useful when you need to construct commands based on the state of your script or the environment in which it’s running.
However, while powerful, the bash eval command should be used judiciously. Because eval will execute any string it’s given, it can lead to unexpected behavior and potential security risks if not used carefully. Always ensure that the input to eval is safe and controlled, and consider alternative approaches where possible.
Exploring Alternatives to Bash Eval
While the bash eval command is a powerful tool for executing commands stored in variables, it’s not the only method available. As you advance in your bash scripting journey, you may find it useful to explore alternative approaches like command substitution or process substitution.
Command Substitution
Command substitution allows you to execute a command and substitute its output in place. It’s done by enclosing the command in $( )
or backticks ``
.
Here’s an example:
files=$(ls)
echo $files
# Output:
# file1.txt file2.txt file3.txt
In this code block, we use command substitution to assign the output of the ls
command to the files
variable. When we echo $files
, it prints the names of all the files in the current directory.
Command substitution is a powerful technique, but it’s not a direct substitute for eval. It’s best used when you want to capture the output of a command, rather than execute a command stored in a string.
Process Substitution
Process substitution is another technique that allows you to execute a command and use its output as an input file for another command. It’s done by enclosing the command in ()
.
Here’s an example:
diff <(ls dir1) <(ls dir2)
# Output:
# Only in dir1: file1.txt
# Only in dir2: file2.txt
In this code block, we use process substitution to compare the contents of two directories using the diff
command. The output lists the files that are unique to each directory.
Like command substitution, process substitution is a powerful technique, but it’s not a direct substitute for eval. It’s best used when you want to use the output of a command as an input file for another command.
While these alternatives can be useful in certain scenarios, it’s important to choose the right tool for the job. Remember, the best method depends on your specific needs and the nature of your bash script.
Troubleshooting Bash Eval: Common Issues and Solutions
Like any powerful tool, the bash eval command has its quirks and potential pitfalls. One of the most common issues is command injection vulnerabilities, which can occur if you’re not careful with the input you pass to eval.
Command Injection Vulnerabilities
Command injection is a type of vulnerability that allows an attacker to execute arbitrary commands on a system. This can occur when you use eval to execute a command that includes user-supplied input.
Here’s an example:
read -p "Enter a directory: " dir
eval ls $dir
In this code block, we’re prompting the user to enter a directory, and then using eval to list the contents of that directory. The problem is, if the user enters something like ; rm -rf *
, the eval command will execute ls ; rm -rf *
, which will list the contents of the current directory and then delete all files in it!
To avoid command injection vulnerabilities, never use eval with user-supplied input. If you need to execute a command based on user input, consider safer alternatives like command substitution.
Safe Usage of Bash Eval
While eval is a powerful command, it should be used judiciously. Always ensure that the input to eval is safe and controlled. If you’re dealing with user-supplied input, consider using safer alternatives like command substitution or process substitution.
Remember, with great power comes great responsibility. Use the bash eval command wisely, and you’ll find it to be a versatile tool in your bash scripting arsenal.
Bash Scripting and Command Execution Fundamentals
Before diving deeper into the bash eval command, it’s crucial to have a solid understanding of the fundamentals of bash scripting and command execution. This knowledge will provide a solid foundation for understanding the underlying concepts of the eval command.
Bash Scripting Basics
Bash (Bourne Again SHell) is a popular command-line interpreter or shell. A shell is a program that takes commands from the keyboard and gives them to the operating system to perform.
Bash scripting is writing a series of commands for the bash interpreter to execute. These commands are stored in a file (known as a shell script) and can be run just like any other command.
Here’s a simple bash script example:
#!/bin/bash
echo 'Hello, world!'
# Output:
# Hello, world!
In this script, the first line #!/bin/bash
is called a shebang. It tells the system that this script should be executed using the bash interpreter. The echo
command is used to print ‘Hello, world!’ to the console.
Command Execution in Bash
When you type a command in a bash shell, the shell performs several processes before the command is actually executed. These processes include splitting the command into words, performing variable substitution, and command substitution.
Understanding these processes is key to understanding how the bash eval command works. The eval command takes a string as an argument, performs all the usual bash processes on that string, and then executes the resulting command.
By understanding the basics of bash scripting and command execution, you’ll be better equipped to understand and use the bash eval command effectively.
The Relevance of Bash Eval in Shell Scripting and Automation
The bash eval command is more than just a command execution tool. Its power and flexibility make it relevant in a wide range of scenarios, from shell scripting to automation and beyond.
In shell scripting, eval can be used to execute commands stored in variables, making your scripts more dynamic and adaptable. It can also be used to construct and execute commands based on the state of your script or the environment in which it’s running.
In automation, eval can be used to execute commands generated by your scripts, allowing you to automate complex tasks and processes. It can also be used to evaluate and execute commands received from external sources, such as configuration files or user input.
While the bash eval command is a powerful tool, it’s not the only method for executing commands in bash. As you continue to hone your bash scripting skills, we encourage you to explore related concepts like command substitution and process substitution. These techniques offer alternative ways to execute commands and can be more appropriate in certain scenarios.
Further Resources for Bash Eval Mastery
If you’re interested in learning more about the bash eval command and related concepts, here are some resources that you might find helpful:
- GNU Bash Reference Manual: This is the official documentation for the bash shell. It’s a comprehensive resource that covers all aspects of bash, including the eval command.
Bash Guide for Beginners: This guide provides a gentle introduction to bash scripting. It’s a great resource for beginners looking to get started with bash.
Advanced Bash-Scripting Guide: This guide is a comprehensive resource for bash scripting. It covers advanced topics and includes a detailed section on the eval command.
Remember, the best way to master the bash eval command is through practice. The more you use it, the more comfortable you’ll become with its quirks and potential pitfalls. So, don’t be afraid to roll up your sleeves and start scripting!
Wrapping Up: Mastering Bash Eval for Command Execution
In this comprehensive guide, we’ve explored the bash eval command, a powerful tool for executing commands in bash.
We began with the basics, learning how to use the bash eval command to execute commands stored in variables. We then delved into more advanced usage, illustrating how eval can be used for more complex scenarios involving variable substitution. We also discussed potential pitfalls and common issues, such as command injection vulnerabilities, and provided solutions and precautions to ensure safe usage.
Along the way, we’ve considered alternative approaches to command execution in bash, such as command substitution and process substitution. These techniques offer different ways to execute commands and can be more appropriate in certain scenarios.
Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Bash Eval | Executes commands stored in variables | Potential for command injection vulnerabilities |
Command Substitution | Captures the output of a command | Not suitable for executing commands stored in strings |
Process Substitution | Uses the output of a command as an input file for another command | Not a direct substitute for eval |
Whether you’re just starting out with bash scripting or you’re looking to level up your command execution skills, we hope this guide has given you a deeper understanding of the bash eval command and its alternatives.
With its power and flexibility, the bash eval command is a valuable tool in your bash scripting arsenal. Use it wisely, and it can greatly enhance your scripting capabilities. Happy scripting!