Using Java Abstract Classes: A Detailed Usage Guide
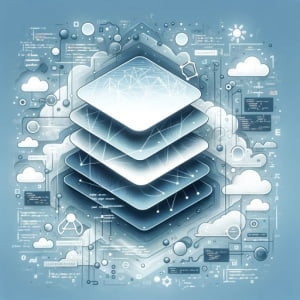
Ever found yourself grappling with abstract classes in Java? You’re not alone. Many developers find the concept of abstract classes a bit challenging. Think of Java’s abstract classes as blueprints – they lay out a foundation for other classes to build upon, providing a versatile and handy tool for various tasks.
Abstract classes are a powerful way to extend the functionality of your Java programs, making them extremely popular for creating complex software architectures.
In this guide, we’ll walk you through the process of using abstract classes in Java, from the basics to more advanced techniques. We’ll cover everything from declaring an abstract class and its methods, subclassing it, to dealing with common issues and their solutions.
Let’s kick things off and learn to master abstract classes in Java!
TL;DR: What is an Abstract Class in Java and How Do I Use It?
An abstract class in Java is a class that cannot be instantiated and is meant to be subclassed by other classes, declared by using the
abstract
keyword before theclass
keyword:abstract class className {}
. It serves as a blueprint for other classes to define methods that must be created within any child classes built from the abstract class.
Here’s a simple example:
abstract class Animal {
abstract void makeSound();
}
class Dog extends Animal {
void makeSound() {
System.out.println('Woof!');
}
}
// Output:
// 'Woof!'
In this example, we’ve defined an abstract class Animal
with an abstract method makeSound()
. Then, we’ve created a Dog
class that extends Animal
and provides an implementation for the makeSound()
method. When we call makeSound()
on an instance of Dog
, it prints ‘Woof!’.
This is a basic way to use abstract classes in Java, but there’s much more to learn about using them effectively in your code. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Declaring and Using Abstract Classes in Java
- Advanced Usage of Abstract Classes in Java
- Exploring Alternatives to Abstract Classes
- Troubleshooting Common Issues with Abstract Classes
- Understanding Abstraction in Object-Oriented Programming
- Abstract Classes in Larger Java Projects
- Wrapping Up: Abstract Classes
Declaring and Using Abstract Classes in Java
Abstract classes are a fundamental part of Java’s object-oriented programming. They act as a template for other classes and can’t be instantiated themselves. To declare an abstract class in Java, you use the abstract
keyword. Let’s dive into how to declare an abstract class and its methods, and how to subclass it.
Declaring an Abstract Class
To declare an abstract class, you simply use the abstract
keyword before the class
keyword. Here’s a basic example:
abstract class Vehicle {
abstract void run();
}
In this code snippet, we’ve declared an abstract class Vehicle
with an abstract method run()
. This method doesn’t have a body – it’s just a declaration. That’s because the implementation of this method is provided by the class that extends this abstract class.
Subclassing an Abstract Class
Now, let’s create a subclass of our Vehicle
abstract class:
class Car extends Vehicle {
void run() {
System.out.println('The car is running.');
}
}
In the Car
class, we’ve provided an implementation for the run()
method declared in the Vehicle
abstract class. When we call run()
on an instance of Car
, it will print ‘The car is running.’.
Here’s how you can use it in your code:
Car myCar = new Car();
myCar.run();
// Output:
// 'The car is running.'
Advantages and Potential Pitfalls
Using abstract classes in Java has several advantages. They allow you to define a common interface for multiple subclasses, increasing code reusability and reducing redundancy. They also allow you to restrict the user from creating an object of the parent class.
However, there are potential pitfalls to be aware of. For instance, if a class includes even one abstract method, the entire class must be declared abstract. Also, an abstract class can’t be instantiated, which means you can’t create an object of an abstract class. It can only be subclassed.
Understanding these basics will set a solid foundation for you to explore more complex uses of abstract classes in Java.
Advanced Usage of Abstract Classes in Java
As we dive deeper into the realm of Java abstract classes, you’ll find that they can be used in conjunction with interfaces for more complex tasks. Here, we’ll explore these advanced techniques and discuss their pros and cons.
Abstract Classes and Interfaces
In Java, an interface is a reference type, similar to an abstract class, that can contain only constants, method signatures, default methods, static methods, and nested types. They give us a way to define contracts that must be fulfilled by classes implementing them.
Abstract classes and interfaces can work together. For instance, an abstract class can implement an interface and provide a default implementation for some or all of its methods. Subclasses of the abstract class are then only responsible for implementing methods that the abstract class hasn’t already.
Here’s an example:
interface Movable {
void move();
}
abstract class Vehicle implements Movable {
abstract void fuelUp();
}
class Car extends Vehicle {
void move() {
System.out.println('The car is moving.');
}
void fuelUp() {
System.out.println('The car is fuelling up.');
}
}
Car myCar = new Car();
myCar.move();
myCar.fuelUp();
// Output:
// 'The car is moving.'
// 'The car is fuelling up.'
In this example, the Vehicle
abstract class is implementing the Movable
interface and declaring an abstract method fuelUp()
. The Car
class, which extends Vehicle
, provides implementations for both move()
and fuelUp()
. When we create an instance of Car
and call these methods, it prints the corresponding messages.
Pros and Cons
Using abstract classes in conjunction with interfaces has its advantages. It allows you to define a contract with the interface and provide a partial implementation with the abstract class, making your code more flexible and reducing duplication.
However, this approach also has its downsides. Java does not support multiple inheritances of classes. This means a class can only extend one abstract class, but it can implement multiple interfaces. So, it’s crucial to carefully design your class hierarchy to avoid potential issues.
Understanding these advanced techniques will take your Java programming skills to the next level and allow you to leverage the full power of abstract classes in your projects.
Exploring Alternatives to Abstract Classes
While abstract classes are a powerful tool in Java, they are not always the best solution. Depending on the situation, you might find that interfaces or regular classes are more suitable. Let’s explore these alternatives and discuss their benefits, drawbacks, and when to use them.
Using Interfaces as an Alternative
Interfaces in Java can often serve as an effective alternative to abstract classes. An interface is a completely “abstract class” that is used to group related methods with empty bodies. Here’s a simple example:
interface Animal {
void makeSound();
}
class Dog implements Animal {
public void makeSound() {
System.out.println('Woof!');
}
}
Dog myDog = new Dog();
myDog.makeSound();
// Output:
// 'Woof!'
In this example, we’ve created an Animal
interface with a makeSound()
method. The Dog
class implements this interface and provides an implementation for this method. When we create an instance of Dog
and call makeSound()
, it prints ‘Woof!’.
Interfaces are particularly useful when different classes share a common behavior, but don’t necessarily constitute a ‘type’ of a certain class — a situation where inheritance might not be the best solution.
Regular Classes as an Alternative
In certain scenarios, a regular class might be more appropriate than an abstract class. If a class doesn’t contain any abstract methods and we need to create instances of this class, a regular class is a better choice.
Making the Right Choice
Deciding whether to use an abstract class, interface, or regular class depends on your specific needs. If you need to create a template for future classes, an abstract class is a good option. If you want to define a contract for other classes to follow, consider using an interface. If you need to instantiate a class and it doesn’t contain any abstract methods, a regular class is the way to go.
Remember, good software design often involves a combination of these elements. Knowing when to use each one is a key skill for any Java programmer.
Troubleshooting Common Issues with Abstract Classes
While working with abstract classes in Java, you may encounter some common issues. Let’s discuss these problems, their solutions, and some best practices for optimization.
Problem: Instantiating an Abstract Class
One common mistake is trying to instantiate an abstract class. Since an abstract class is a blueprint for other classes, it can’t be instantiated. Attempting to do so will result in a compile-time error.
abstract class Vehicle {
abstract void run();
}
Vehicle myVehicle = new Vehicle(); // This will throw a compile-time error
The comment in the code snippet above is trying to instantiate an abstract class, which is not allowed in Java. This will result in a compile-time error.
Solution: Use a Subclass
The correct way to use an abstract class is to create a subclass and use that instead.
class Car extends Vehicle {
void run() {
System.out.println('The car is running.');
}
}
Car myCar = new Car();
myCar.run();
// Output:
// 'The car is running.'
In this example, we’ve created a Car
class that extends the Vehicle
abstract class and provides an implementation for the run()
method. We then create an instance of Car
and call run()
, which prints ‘The car is running.’.
Best Practices and Optimization
When working with abstract classes in Java, here are some best practices to keep in mind:
- Use abstract classes when you want to provide a common interface for different subclasses.
- Avoid adding too many abstract methods in an abstract class. This could make the class difficult to use and maintain.
- Remember that a class can only extend one abstract class, but it can implement multiple interfaces. Choose your class hierarchy wisely to take advantage of this.
By understanding these common issues and their solutions, you can avoid potential pitfalls and use abstract classes effectively in your Java programs.
Understanding Abstraction in Object-Oriented Programming
Abstraction is a fundamental concept in object-oriented programming. It’s a process of hiding the implementation details and showing only the functionality to the user. In other words, it lets you focus on ‘what’ the object does instead of ‘how’ it does it.
Role of Abstract Classes in Abstraction
Abstract classes play a crucial role in implementing abstraction in Java. They allow you to declare methods without providing an implementation. The actual implementation is then provided by the subclass that extends the abstract class. This way, the abstract class can define a common interface for its subclasses.
Here’s a simple example:
abstract class Shape {
abstract void draw();
}
class Circle extends Shape {
void draw() {
System.out.println('Drawing a circle.');
}
}
class Square extends Shape {
void draw() {
System.out.println('Drawing a square.');
}
}
Shape myShape = new Circle();
myShape.draw();
myShape = new Square();
myShape.draw();
// Output:
// 'Drawing a circle.'
// 'Drawing a square.'
In this example, we’ve created an abstract Shape
class with an abstract draw()
method. We’ve then created Circle
and Square
classes that extend Shape
and provide their own implementations for draw()
. When we create instances of Circle
and Square
and call draw()
, it prints the corresponding messages.
This demonstrates how abstract classes can be used to implement abstraction in Java. By declaring methods in the abstract class, we’re defining a common interface for the subclasses. The actual implementation details are hidden in the subclasses, allowing us to focus on the functionality, not the implementation.
Abstract Classes in Larger Java Projects
Abstract classes are not just theoretical constructs, but practical tools used extensively in larger Java projects. They provide a structure and reduce the complexity when dealing with a large codebase. By defining a common interface for subclasses, they increase code reusability and maintainability.
Polymorphism and Abstract Classes
One of the key concepts that often accompany abstract classes is polymorphism. Polymorphism allows objects of different classes to be treated as objects of a common superclass. This is particularly useful when combined with abstract classes, as it allows us to write more general and reusable code.
Design Patterns Involving Abstract Classes
Abstract classes also play a crucial role in many design patterns in Java, such as the Template Method pattern. In this pattern, an abstract class defines a ‘template’ of methods, and concrete subclasses fill in the details. This allows you to define the skeleton of an algorithm in the abstract class and let subclasses redefine certain steps of the algorithm without changing its structure.
Further Resources for Mastering Abstract Classes
For those looking to delve deeper into the world of Java abstract classes, here are a few resources that offer more in-depth information:
- IOFlood’s Java OOPs Concepts Article – Understand how to achieve method overriding and method overloading in Java.
Abstract Class vs Interface in Java – Explore the differences between abstract classes and interfaces in Java.
Implementing Design Patterns in Java – Master design patterns for writing modular, maintainable, and scalable Java code
Java Documentation on Abstract Methods and Classes by Oracle details abstract classes and methods in Java.
Java Abstract Class and Interface Explained by Baeldung provides a comparison between abstract classes and interfaces.
Java Design Patterns is a useful resource for understanding various design patterns in Java.
By exploring these resources and understanding the practical applications of abstract classes, you can take your Java programming skills to the next level.
Wrapping Up: Abstract Classes
In this comprehensive guide, we’ve delved into the world of abstract classes in Java, a fundamental concept in object-oriented programming that provides a blueprint for creating more specific classes.
We started with the basics, learning how to declare an abstract class and its methods, and how to subclass it. We then explored more advanced topics, such as using abstract classes in conjunction with interfaces for more complex tasks. We also looked at alternative approaches, discussing when to use interfaces or regular classes instead of abstract classes.
Along the way, we tackled common issues that you might encounter when working with abstract classes, such as trying to instantiate an abstract class, and provided solutions to help you avoid these pitfalls. We also discussed the concept of abstraction in object-oriented programming and the role of abstract classes in implementing it.
For quick comparison, here’s a summary of the methods we’ve discussed:
Method | Use Case | Pros | Cons |
---|---|---|---|
Abstract Classes | When you want to provide a common interface for subclasses | Increases code reusability and maintainability | Can’t be instantiated; a class can only extend one abstract class |
Interfaces | When you want to define a contract for other classes to follow | Provides a way to ensure that a class adheres to a certain contract | Can’t contain any implementation details |
Regular Classes | When you need to instantiate a class and it doesn’t contain any abstract methods | Can be instantiated; easy to use | May lead to code duplication if not carefully designed |
Whether you’re just starting out with abstract classes in Java or you’re looking to deepen your understanding, we hope this guide has been a valuable resource. Abstract classes are a powerful tool in Java, enabling you to write more flexible and maintainable code. Happy coding!