json.loads() Python Function | Convert JSON to dict
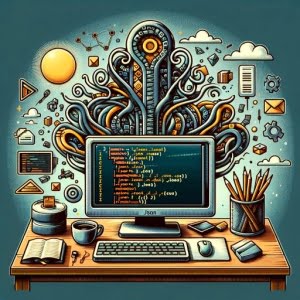
Are you struggling with json.loads in Python? Think of it as a magical key that unlocks the treasure trove of data stored in JSON format.
This guide is your map to that treasure, providing a step-by-step walkthrough on how to use json.loads to decode JSON data in Python.
We’ll start with the basics, then move on to more advanced techniques, ensuring you have a comprehensive understanding of json.loads in Python.
So, let’s embark on this journey to master json.loads together!
TL;DR: How Do I Use json.loads to convert JSON to a dict in Python?
The
json.loads()
function can be simply used likedata = json.loads(json_string)
to parse JSON data into a Python dictionary. It’s like a translator, converting JSON data into a Python-readable format. Here’s a simple example:
import json
json_string = '{"name": "John", "age": 30}'
data = json.loads(json_string)
print(data)
# Output:
# {'name': 'John', 'age': 30}
In this example, we have a JSON string json_string
that represents a person named John who is 30 years old. The json.loads
function is used to convert this JSON string into a Python dictionary, which is then printed out. The output shows the Python dictionary with the same data as the original JSON string.
This is just the tip of the iceberg! Stay tuned for a more detailed understanding and advanced usage scenarios of json.loads in Python.
Table of Contents
Unveiling Json.loads: The Basics
The json.loads function is a part of Python’s json module, and it’s used to decode JSON data. JSON, or JavaScript Object Notation, is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. It’s often used when data is sent from a server to a web page.
The json.loads function takes a JSON formatted string and converts, or ‘loads’, it into a Python object. Let’s break down how it works with a simple example.
import json
# a JSON formatted string
json_string = '{"fruit": "Apple", "size": "Large", "color": "Red"}'
# using json.loads to decode the JSON data
python_obj = json.loads(json_string)
print(python_obj)
# Output:
# {'fruit': 'Apple', 'size': 'Large', 'color': 'Red'}
In this example, we have a JSON string json_string
that describes an apple. The json.loads
function is used to convert this JSON string into a Python dictionary, which is then printed out. The output shows the Python dictionary with the same data as the original JSON string.
One of the key advantages of using json.loads is its simplicity and efficiency in converting JSON data into Python objects. However, one potential pitfall to keep in mind is that json.loads will raise a json.decoder.JSONDecodeError
if the input is not a correctly formatted JSON string. Therefore, it’s important to ensure your JSON data is correctly formatted before using json.loads.
Json.loads: Dealing with Complex JSON Data
As you become more comfortable with json.loads, you’ll likely encounter more complex JSON data. This data might include nested objects or arrays. This is where json.loads truly shines – it can handle this complexity with ease.
Let’s take a look at an example of using json.loads with nested JSON data.
import json
# a JSON formatted string with nested data
json_string = '{"person": {"name": "John", "age": 30, "city": "New York"}}'
# using json.loads to decode the JSON data
python_obj = json.loads(json_string)
print(python_obj)
# Output:
# {'person': {'name': 'John', 'age': 30, 'city': 'New York'}}
In this example, the JSON string json_string
has a nested object person
. The json.loads
function is used to convert this nested JSON string into a nested Python dictionary. The output shows the nested Python dictionary with the same data structure as the original JSON string.
One of the best practices when dealing with complex JSON data is to always print out the converted Python object. This allows you to visually verify the data structure and ensure it matches the original JSON data. Remember, json.loads will raise a json.decoder.JSONDecodeError
if the JSON data is not correctly formatted. So it’s always a good idea to double-check your data.
Exploring Alternatives: json.load and More
While json.loads is a fantastic tool for decoding JSON data, Python offers other methods as well. One such alternative is the json.load function. Unlike json.loads, which works with JSON formatted strings, json.load is used to read JSON data from a file. Let’s take a look at how it works.
import json
# assuming 'data.json' contains: {"name": "John", "age": 30}
with open('data.json', 'r') as file:
data = json.load(file)
print(data)
# Output:
# {'name': 'John', 'age': 30}
In this example, we have a JSON file data.json
that contains JSON data. The json.load
function is used to read this data from the file and convert it into a Python dictionary. The output shows the Python dictionary with the same data as the original JSON file.
The advantage of using json.load is that it allows you to work directly with JSON files, which can be more efficient when dealing with large amounts of data. However, it requires the JSON data to be stored in a file, which might not always be the case. If your JSON data is stored as a string, json.loads would be the better choice.
Remember, the right tool depends on your specific needs. Both json.loads and json.load are powerful tools for parsing JSON data in Python, and understanding how to use both will make you a more versatile programmer.
Troubleshooting Json.loads: Common Issues
While json.loads is a powerful tool, it’s not without its quirks. You might encounter certain issues during JSON parsing, and understanding how to troubleshoot these can save you a lot of time and frustration.
Encountering the ‘json.decoder.JSONDecodeError’
One of the most common issues you might encounter is the ‘json.decoder.JSONDecodeError’. This error is raised when json.loads encounters a problem decoding a string. This is usually due to malformed JSON data. Here’s an example:
import json
# a malformed JSON string
json_string = '{"name" "John", "age": 30}'
try:
data = json.loads(json_string)
except json.decoder.JSONDecodeError as e:
print(f'Error decoding JSON: {e}')
# Output:
# Error decoding JSON: Expecting property name enclosed in double quotes: line 1 column 8 (char 7)
In this example, the JSON string json_string
is missing a colon between the key ‘name’ and its value ‘John’. When we try to decode this string with json.loads, it raises a json.decoder.JSONDecodeError
.
The solution to this problem is to ensure that your JSON data is correctly formatted. You can do this by manually checking your data, or by using a tool like a JSON validator. In this case, adding the missing colon will fix the problem.
Handling Malformed JSON Data
Another issue you might encounter is malformed JSON data. This can occur if there’s a problem with the structure of your JSON data, such as missing brackets, incorrect comma placement, or incorrect use of quotes. Here’s an example:
import json
# a malformed JSON string
json_string = '{"name": "John", "age": 30'
try:
data = json.loads(json_string)
except json.decoder.JSONDecodeError as e:
print(f'Error decoding JSON: {e}')
# Output:
# Error decoding JSON: Expecting property name enclosed in double quotes: line 1 column 29 (char 28)
In this example, the JSON string json_string
is missing a closing bracket. When we try to decode this string with json.loads, it raises a json.decoder.JSONDecodeError
.
The solution to this problem is similar to the previous one – ensure that your JSON data is correctly formatted. In this case, adding the missing closing bracket will fix the problem.
Understanding JSON and Python’s Json Module
Before we delve deeper into json.loads, let’s take a step back and understand the fundamentals – JSON and Python’s json module.
What is JSON?
JSON, or JavaScript Object Notation, is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. It’s a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others. These properties make JSON an ideal data-interchange language.
JSON is built on two structures:
- A collection of name/value pairs, which in various languages is realized as an object, record, struct, dictionary, hash table, keyed list, or associative array.
- An ordered list of values, which in most languages is realized as an array, vector, list, or sequence.
JSON’s basic data types are:
- Number: a signed decimal number that may contain a fractional part and may use exponential E notation, but cannot include non-numbers like NaN.
- String: a sequence of zero or more Unicode characters.
- Boolean: either of the values true or false.
- Array: an ordered list of zero or more values, each of which may be of any type.
- Object: an unordered collection of name/value pairs where the names (also called keys) are strings.
- null: an empty value, using the word null.
Python’s Json Module
Python’s json module contains functions to parse JSON from strings or files, as well as convert strings and Python data types to JSON. The two main functions are json.loads and json.dumps.
- json.loads: This function parses a JSON string and returns a Python object. It’s what we’ve been discussing in this guide.
json.dumps: This function takes a Python object, typically a dictionary or a list, and returns a JSON string. It’s the opposite of json.loads.
These functions make it easy to work with JSON data in Python. Whether you’re reading data from a file, a website, or an API, the json module has the tools you need to parse and manipulate that data.
The Power of Json.loads Beyond Decoding
Understanding and mastering json.loads opens up a world of opportunities. It’s not just about decoding JSON data, but the applications of this process are vast and critical in today’s data-driven world.
Json.loads in Web Scraping
Web scraping is a method used to extract large amounts of data from websites. The data on the websites are unstructured, and json.loads is often used to parse the JSON data into a structured form.
import json
import requests
# Make a request
response = requests.get('https://api.example.com/data')
# Use json.loads to parse the response
data = json.loads(response.text)
print(data)
# Output:
# {'data': [{'id': 1, 'name': 'John'}, {'id': 2, 'name': 'Jane'}]}
In this example, we make a GET request to a hypothetical API and receive a response. The json.loads
function is used to parse the JSON data from the response, converting it into a Python object that we can work with.
Json.loads in API Interaction
APIs, or Application Programming Interfaces, are used to interact with other software components. They often return data in JSON format, and json.loads is used to parse this data.
import json
import requests
# Make a request to an API
response = requests.get('https://api.example.com/data')
# Use json.loads to parse the response
data = json.loads(response.text)
print(data)
# Output:
# {'data': [{'id': 1, 'name': 'John'}, {'id': 2, 'name': 'Jane'}]}
In this example, we make a GET request to a hypothetical API and receive a response. The json.loads
function is used to parse the JSON data from the response, converting it into a Python object that we can work with.
Json.loads in Data Analysis
Data analysis involves inspecting, cleansing, transforming, and modeling data to discover useful information, inform conclusions, and support decision-making. Json.loads plays a critical role in this process when the data is in JSON format.
import json
# a JSON formatted string
json_string = '[{"name": "John", "age": 30}, {"name": "Jane", "age": 25}]'
# using json.loads to decode the JSON data
data = json.loads(json_string)
# calculate the average age
average_age = sum(person['age'] for person in data) / len(data)
print(average_age)
# Output:
# 27.5
In this example, we have a JSON string json_string
that contains a list of people with their ages. The json.loads
function is used to convert this JSON string into a Python list of dictionaries, which we can then use to calculate the average age.
Further Resources for JSON and APIs in Python
The power of json.loads extends beyond these examples. It’s a fundamental tool in handling JSON data in files, working with APIs in Python, and much more. To explore these concepts further and more, consider using the following guides:
- Simplifying Python JSON – Dive into the world of structured data exchange using JSON in Python.
YAML Parsing in Python – A quick guide on Python’s YAML parsing capabilities for structured data representation.
JSON Data Conversion with json.dumps() offers tips on “json.dumps” for encoding Python dictionaries and lists into JSON strings.
Working with JSON in Python – Learn the mechanics of handling and processing JSON data in Python.
Python CSV Library Documentation – Access the official Python documentation for a comprehensive guide to the CSV module.
File Handling with XML in Python – Delve deep into XML file handling in Python with this informative guide.
Wrapping Up: json.loads Mastery
We’ve embarked on a comprehensive journey to understand the usage of json.loads in Python. We started with the basics, understanding what json.loads does – decoding JSON data into Python-readable format. We saw how a simple command like json.loads(json_string)
can convert a JSON string into a Python dictionary.
We also explored how json.loads handles complex and nested JSON data, providing us with the flexibility to work with a variety of data structures. We discussed potential issues one might face during JSON parsing, such as ‘json.decoder.JSONDecodeError’ and malformed JSON data, and how to troubleshoot them.
As we moved to advanced topics, we discovered alternative methods to parse JSON data in Python, like the json.load function. We learned that while json.loads works with JSON formatted strings, json.load reads JSON data from a file, offering a different approach to handle JSON parsing.
Finally, we delved into the relevance of JSON parsing in web scraping, API interaction, and data analysis, showing the vast applications of mastering json.loads. In essence, understanding json.loads is a key step in becoming proficient in handling JSON data in Python.
As you continue your journey in Python, remember that mastering tools like json.loads can significantly enhance your data handling capabilities.