Python YAML Parser Guide | PyYAML, ruamel.yaml And More
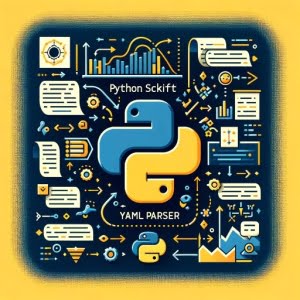
Are you finding it challenging to parse YAML files in Python? You’re not alone. Many developers struggle with this task, but Python, like a skilled interpreter, can easily translate YAML files into a format that you can work with.
YAML, being a human-friendly data serialization standard, is often used for writing configuration files and in applications where data is being stored or transmitted. Python, with its powerful libraries, makes it easy to parse these YAML files and work with them in a more Pythonic manner.
In this guide, we’ll walk you through the process of parsing YAML files using Python, from basic usage to advanced techniques. We’ll cover everything from using the PyYAML library for simple YAML parsing tasks to handling more complex YAML files with custom tags or complex data structures. We’ll also introduce alternative libraries for parsing YAML files in Python, such as ruamel.yaml.
So, let’s dive in and start mastering YAML parsing in Python!
TL;DR: How Do I Parse a YAML File in Python?
To parse a YAML file in Python, you can use the PyYAML library, like
data = yaml.safe_load(file)
. This library allows you to load YAML files and convert them into Python data structures such as dictionaries and lists.
Here’s a simple example:
import yaml
with open('example.yaml', 'r') as file:
data = yaml.safe_load(file)
print(data)
# Output:
# {'example': 'data'}
In this example, we import the yaml module and use the yaml.safe_load()
function to parse the YAML file. The safe_load()
function converts the YAML document into a Python dictionary, which we then print to the console.
This is a basic way to parse YAML files in Python, but there’s much more to learn about handling more complex YAML files and using alternative libraries. Continue reading for a more detailed guide on parsing YAML files with Python.
Table of Contents
- Getting Started with PyYAML: The Basics
- Advanced PyYAML Parsing: Custom Tags and Complex Structures
- Exploring Alternative Libraries: ruamel.yaml
- Troubleshooting Python YAML Parsing
- Understanding YAML and Parsing Concepts
- YAML Parsing in Real-World Applications
- Expanding Your Parsing Skills: JSON and XML
- Further Resources for Mastering YAML Parsing
- Wrapping Up: Mastering Python YAML Parsing
Getting Started with PyYAML: The Basics
PyYAML is a Python library that provides a set of tools for parsing YAML files. It’s widely used due to its simplicity and effectiveness. Let’s dive into how we can use PyYAML to parse YAML files.
Parsing YAML with PyYAML: A Simple Example
Let’s start with a basic example. Suppose we have a YAML file named ‘example.yaml’ with the following content:
name: John Doe
age: 30
We can parse this YAML file into a Python dictionary using PyYAML as follows:
import yaml
with open('example.yaml', 'r') as file:
data = yaml.safe_load(file)
print(data)
# Output:
# {'name': 'John Doe', 'age': 30}
In this example, we first import the yaml module. Then, we open the YAML file using Python’s built-in open()
function and pass it to yaml.safe_load()
. The safe_load()
function reads the YAML file and converts it into a Python dictionary. Finally, we print the dictionary to the console.
Understanding PyYAML: Advantages and Pitfalls
One of the main advantages of PyYAML is its simplicity. As seen in the example above, you can parse a YAML file with just a few lines of code. PyYAML also supports all YAML 1.1 constructs, so it can handle most YAML files you’ll encounter.
However, PyYAML has its pitfalls. For example, it doesn’t support YAML 1.2, the latest version of YAML. Also, while yaml.load()
can handle any YAML file, it’s not safe to use because it can execute arbitrary Python code contained in the YAML file. Therefore, you should always use yaml.safe_load()
instead.
Advanced PyYAML Parsing: Custom Tags and Complex Structures
As your YAML parsing needs become more complex, PyYAML continues to offer solutions. Let’s explore how you can handle custom tags and complex data structures.
PyYAML and Custom Tags
In YAML, tags are a way to specify the data type of a node. PyYAML allows you to define custom tags to handle specific data types.
Consider the following YAML document with a custom !Person
tag:
- !Person
name: John Doe
age: 30
To parse this YAML document, you need to define a Python class for the Person
data type and a constructor that tells PyYAML how to convert !Person
nodes into Person
objects:
import yaml
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def person_constructor(loader, node):
values = loader.construct_mapping(node)
return Person(values['name'], values['age'])
yaml.SafeLoader.add_constructor('!Person', person_constructor)
data = yaml.safe_load(yaml_string)
for person in data:
print(f'{person.name} is {person.age} years old.')
# Output:
# John Doe is 30 years old.
In this example, we define a Person
class and a person_constructor
function. We then tell PyYAML to use person_constructor
to convert !Person
nodes into Person
objects by calling yaml.SafeLoader.add_constructor()
.
Handling Complex Data Structures
PyYAML can also handle more complex data structures, such as nested dictionaries and lists. Consider the following YAML document:
employees:
- name: John Doe
age: 30
- name: Jane Doe
age: 25
You can parse this YAML document into a Python dictionary containing a list of dictionaries as follows:
import yaml
with open('example.yaml', 'r') as file:
data = yaml.safe_load(file)
for employee in data['employees']:
print(f'{employee['name']} is {employee['age']} years old.')
# Output:
# John Doe is 30 years old.
# Jane Doe is 25 years old.
In this example, yaml.safe_load()
converts the YAML document into a Python dictionary where the value of the ’employees’ key is a list of dictionaries. Each dictionary represents an employee and contains ‘name’ and ‘age’ keys.
Exploring Alternative Libraries: ruamel.yaml
While PyYAML is a popular choice for parsing YAML files in Python, there are alternative libraries that you might find useful, such as ruamel.yaml. This library is a YAML 1.2 loader/dumper package for Python and can handle edge cases that PyYAML cannot.
Parsing YAML with ruamel.yaml
Let’s illustrate the usage of ruamel.yaml with a simple example. Suppose we have the same ‘example.yaml’ file we used earlier:
name: John Doe
age: 30
Here’s how you can parse this YAML file using ruamel.yaml:
from ruamel.yaml import YAML
yaml = YAML()
with open('example.yaml', 'r') as file:
data = yaml.load(file)
print(data)
# Output:
# {'name': 'John Doe', 'age': 30}
In this example, we first import the YAML
class from the ruamel.yaml
module. We then create an instance of the YAML
class and use its load()
method to parse the YAML file. The load()
method returns a dictionary that we print to the console.
Advantages and Disadvantages of ruamel.yaml
One of the main advantages of ruamel.yaml is its support for YAML 1.2, the latest version of YAML. It also preserves the order of dictionaries and the formatting of the original YAML file, which can be useful in certain scenarios.
On the downside, ruamel.yaml is more complex than PyYAML and has a steeper learning curve. It’s also not as widely used as PyYAML, so you might find fewer resources and community support.
Choosing the Right Library for Your Project
In conclusion, while PyYAML is a great choice for most YAML parsing tasks due to its simplicity and wide usage, ruamel.yaml is a powerful alternative that you might consider for more complex or specific needs. Always evaluate the needs of your project and choose the library that best fits those needs.
Troubleshooting Python YAML Parsing
Even with the best tools and techniques, you might encounter some challenges when parsing YAML files with Python. Let’s discuss some common issues and their solutions.
Dealing with Parsing Errors
One common issue is parsing errors, which occur when the YAML file contains syntax errors. PyYAML and ruamel.yaml will raise a YAMLError
if they can’t parse the YAML file.
Here’s an example of how you can handle parsing errors:
import yaml
try:
with open('example.yaml', 'r') as file:
data = yaml.safe_load(file)
except yaml.YAMLError as error:
print(f'Error parsing YAML file: {error}')
In this example, we use a try/except block to catch YAMLError
exceptions. If a YAMLError
is raised, we print an error message to the console.
Handling Specific Data Structures
Another common issue is dealing with specific data structures, such as nested dictionaries or lists. Both PyYAML and ruamel.yaml can handle these data structures, but you need to understand how they convert YAML nodes into Python data structures.
For example, consider the following YAML document:
employees:
- name: John Doe
age: 30
- name: Jane Doe
age: 25
Both PyYAML and ruamel.yaml will parse this YAML document into a Python dictionary where the value of the ’employees’ key is a list of dictionaries. Each dictionary represents an employee and contains ‘name’ and ‘age’ keys.
Understanding these conversions is crucial for working with complex YAML files. Always refer to the PyYAML or ruamel.yaml documentation for more information about these conversions.
Understanding YAML and Parsing Concepts
To fully grasp the process of parsing YAML files with Python, it’s essential to understand what YAML is and the basic theory behind parsing.
YAML: A Human-Friendly Data Serialization Standard
YAML, which stands for ‘YAML Ain’t Markup Language’, is a human-friendly data serialization standard. It’s often used for configuration files and in applications where data is being stored or transmitted. YAML files are easy to read and write, making them a popular choice among developers.
Here’s an example of a simple YAML document:
name: John Doe
age: 30
In this example, the YAML document consists of two key-value pairs: ‘name’ and ‘age’. Each key-value pair is separated by a colon, and each pair is on a new line.
Parsing: Translating Data into a Usable Format
Parsing is the process of analyzing a string of symbols, either in natural language or in computer languages, according to the rules of a formal grammar. In the context of YAML files, parsing is the process of converting the YAML document into a data structure that Python can work with, such as a dictionary or a list.
When you parse a YAML file in Python using a library like PyYAML or ruamel.yaml, the library reads the YAML file and converts it into a Python data structure. This conversion process is based on the YAML specification, which defines how different YAML constructs should be represented in different data structures.
By understanding YAML and the theory behind parsing, you can better understand how Python libraries like PyYAML and ruamel.yaml parse YAML files and how you can use these libraries to effectively work with YAML files in Python.
YAML Parsing in Real-World Applications
Parsing YAML files in Python is not just an academic exercise; it has significant real-world applications. Let’s explore some of them.
YAML in Configuration Management
YAML files are often used for configuration management. They provide a human-friendly way to specify configuration settings and can be easily parsed by Python, making them a popular choice for configuring Python applications.
Data Serialization with YAML
YAML is also used for data serialization. When you need to store or transmit data, you can serialize it into a YAML document using Python. When you need to use the data, you can parse the YAML document back into a Python data structure.
Expanding Your Parsing Skills: JSON and XML
Once you’ve mastered YAML parsing in Python, consider exploring related concepts like JSON parsing or XML parsing. JSON and XML are other popular data formats that you might encounter, and Python provides libraries for parsing them, such as json and xml.etree.ElementTree.
Further Resources for Mastering YAML Parsing
To continue your journey towards mastering YAML parsing in Python, here are some additional resources you might find helpful:
- Python JSON Fundamentals Covered – Dive deep into JSON manipulation and data modification using Python.
XML Handling in Python: A Quick Introduction – Explore Python’s XML processing usave for data manipulation.
Python json.dumps() Explained – Explore JSON serialization techniques for data interchange in Python.
Official YAML Website – Learn more about the YAML specification and its features.
PyYAML Documentation – Dive deeper into the PyYAML library and its capabilities.
ruamel.yaml Documentation – Explore the advanced features of ruamel.yaml.
Wrapping Up: Mastering Python YAML Parsing
In this comprehensive guide, we’ve delved into the art of parsing YAML files using Python, a skill that’s vital in handling configuration files and data serialization.
We began with the basics, understanding how to use PyYAML, a simple yet powerful library for parsing YAML files. We explored how to parse simple YAML files into Python dictionaries and discussed the advantages and pitfalls of using PyYAML.
We then ventured into more advanced territory, exploring how PyYAML can handle custom tags and complex data structures. We also introduced an alternative library, ruamel.yaml, which offers advanced features and supports the latest version of YAML.
Along the way, we tackled common challenges that you might face when parsing YAML files with Python, such as parsing errors and handling specific data structures, providing you with solutions and workarounds for each issue.
Here’s a quick comparison of the libraries we’ve discussed:
Library | YAML Support | Complexity | Use Case |
---|---|---|---|
PyYAML | YAML 1.1 | Low to Medium | Basic to Intermediate YAML files |
ruamel.yaml | YAML 1.2 | Medium to High | Complex YAML files with custom tags |
Whether you’re just starting out with parsing YAML files in Python or you’re looking to expand your skills, we hope this guide has given you a deeper understanding of the process and the tools available to you.
With the knowledge you’ve gained, you’re now equipped to handle YAML files in Python with confidence. Happy coding!