Python XML Handling | Guide (With Examples)
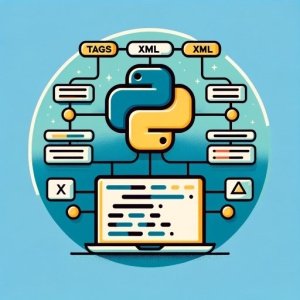
Are you finding it challenging to navigate the labyrinth of XML data in Python? You’re not alone. Many developers find themselves in a maze when it comes to handling XML files in Python, but we’re here to help.
Think of Python’s XML handling as a skilled librarian – it can help you navigate through a maze of XML data, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of parsing and generating XML files using Python, from their creation, manipulation, and usage. We’ll cover everything from the basics of XML parsing to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Work with XML Files in Python?
Python provides several libraries for parsing and generating XML files, including the built-in
xml.etree.ElementTree
module. Here’s a simple example of parsing an XML file:
import xml.etree.ElementTree as ET
tree = ET.parse('example.xml')
root = tree.getroot()
print(root.tag)
# Output:
# 'root_element'
In this example, we import the xml.etree.ElementTree
module as ET
. We then parse the ‘example.xml’ file and get the root of the XML tree. Finally, we print the tag of the root element, which in this case is ‘root_element’.
This is a basic way to parse XML files in Python, but there’s much more to learn about XML parsing and generation. Continue reading for more detailed explanations and advanced usage examples.
Table of Contents
- Parsing XML Files in Python: A Beginner’s Guide
- Advanced XML Parsing Techniques with Python
- Exploring Alternative Libraries for XML in Python
- Troubleshooting XML Handling in Python
- Understanding XML and Its Comparison to Other Data Formats
- Exploring DOM and SAX Parsing Models
- Integrating XML Parsing into Larger Python Projects
- Wrapping Up: Mastering XML Parsing and Generation in Python
Parsing XML Files in Python: A Beginner’s Guide
Python offers a variety of libraries for parsing XML files, but one of the most straightforward and beginner-friendly is the built-in xml.etree.ElementTree
module. This module treats an XML document as a tree of elements, making it intuitive to navigate and manipulate the data.
Here’s a simple step-by-step guide on how to parse an XML file using xml.etree.ElementTree
:
# Import the required module
import xml.etree.ElementTree as ET
# Parse the XML file
tree = ET.parse('example.xml')
# Get the root element
root = tree.getroot()
# Print the tag of the root element
print(root.tag)
# Output:
# 'root_element'
In this example, we first import the xml.etree.ElementTree
module as ET
. We then parse the ‘example.xml’ file and get the root of the XML tree. Finally, we print the tag of the root element, which in this case is ‘root_element’.
Pros and Cons of Using xml.etree.ElementTree
Like all tools, xml.etree.ElementTree
has its strengths and weaknesses. On the plus side, it’s built into Python, so you don’t need to install any additional packages. It also treats the XML document as a tree, which can be more intuitive than other models of XML parsing.
On the downside, xml.etree.ElementTree
isn’t as powerful or flexible as some other XML parsing libraries. For example, it doesn’t support XPath expressions, which can be a more efficient way to navigate an XML document. We’ll explore some of these more advanced techniques in the next section.
Advanced XML Parsing Techniques with Python
As you become more comfortable with XML parsing in Python, you might find yourself needing more powerful tools. One such tool is XPath, a language for navigating XML documents.
XPath expressions can be used with the xml.etree.ElementTree
module to find specific elements or attributes. Here’s an example:
# Import the required module
import xml.etree.ElementTree as ET
# Parse the XML file
tree = ET.parse('example.xml')
# Use an XPath expression to find all 'child' elements
children = tree.findall('.//child')
# Print the tags and text of each child element
for child in children:
print(child.tag, child.text)
# Output:
# 'child' 'Child text'
In this example, the XPath expression ‘.//child’ finds all ‘child’ elements in the XML document. We then print the tag and text of each ‘child’ element.
Generating XML Files with Python
Python’s xml.etree.ElementTree
module can also be used to generate XML files. Here’s a simple example:
# Import the required module
import xml.etree.ElementTree as ET
# Create the root element
root = ET.Element('root')
# Create a child element
child = ET.SubElement(root, 'child')
# Set the text of the child element
child.text = 'This is a child element'
# Create an ElementTree object
tree = ET.ElementTree(root)
# Write the XML document to a file
tree.write('output.xml')
# Output:
# A new XML file named 'output.xml' is created.
In this example, we first create the root element and a child element. We then set the text of the child element, create an ElementTree object, and write the XML document to a file.
Pros and Cons of Advanced XML Parsing Techniques
Advanced XML parsing techniques like XPath can provide more efficient and flexible ways to navigate XML documents. However, they also have a steeper learning curve and can be overkill for simple tasks. Similarly, generating XML files with Python can be a powerful tool, but it requires a good understanding of both the XML format and Python’s XML libraries.
Exploring Alternative Libraries for XML in Python
While xml.etree.ElementTree
is a powerful tool for XML parsing and generation, Python offers other libraries that provide additional features and flexibility. Two such libraries are lxml
and xml.dom.minidom
.
The Power of lxml
lxml
is a library for processing XML and HTML in Python. It’s compatible with xml.etree.ElementTree
, but adds some powerful features like XPath 1.0 support, XSLT 1.0 support, and more.
Here’s an example of parsing an XML file with lxml
:
# Import the required module
from lxml import etree
# Parse the XML file
tree = etree.parse('example.xml')
# Use an XPath expression to find all 'child' elements
children = tree.xpath('//child')
# Print the tags and text of each child element
for child in children:
print(child.tag, child.text)
# Output:
# 'child' 'Child text'
In this example, we use the lxml.etree
module to parse an XML file and find all ‘child’ elements with an XPath expression.
Navigating XML with xml.dom.minidom
xml.dom.minidom
is another Python library for parsing XML documents. It implements the Document Object Model (DOM), a standard for navigating XML and HTML documents.
Here’s an example of parsing an XML file with xml.dom.minidom
:
# Import the required module
from xml.dom.minidom import parse
# Parse the XML file
dom = parse('example.xml')
# Use a DOM method to get all 'child' elements
children = dom.getElementsByTagName('child')
# Print the tags and text of each child element
for child in children:
print(child.tagName, child.firstChild.data)
# Output:
# 'child' 'Child text'
In this example, we use the xml.dom.minidom.parse
function to parse an XML file. We then use the getElementsByTagName
method to get all ‘child’ elements and print their tags and text.
Choosing the Right Library
Choosing the right library for XML parsing in Python depends on your needs. If you want a simple, intuitive interface, xml.etree.ElementTree
might be the right choice. If you need more powerful features like XPath and XSLT support, lxml
could be a better fit. If you prefer the DOM model for navigating XML documents, xml.dom.minidom
might be the best choice. Ultimately, the best tool is the one that fits your needs and workflow.
Troubleshooting XML Handling in Python
XML parsing and generation in Python is generally straightforward, but you may encounter some common issues. Two of the most common are handling namespaces and dealing with malformed XML files.
Handling Namespaces
Namespaces in XML are a way of avoiding element name conflicts. They can be tricky to handle, but Python’s XML libraries provide ways to manage them. Here’s an example using xml.etree.ElementTree
:
# Import the required module
import xml.etree.ElementTree as ET
# Parse the XML file with a namespace
root = ET.fromstring('<root xmlns:ns="http://example.com/ns"><ns:child>Child text</ns:child></root>')
# Define the namespace
ns = {'ns': 'http://example.com/ns'}
# Use an XPath expression with the namespace to find the 'child' element
child = root.find('ns:child', ns)
# Print the tag and text of the child element
print(child.tag, child.text)
# Output:
# '{http://example.com/ns}child' 'Child text'
In this example, we first parse an XML string with a namespace. We then define the namespace and use an XPath expression with the namespace to find the ‘child’ element.
Dealing with Malformed XML Files
Malformed XML files can cause errors when parsing. Python’s XML libraries raise specific exceptions for these cases, which can be caught and handled. Here’s an example:
# Import the required module
import xml.etree.ElementTree as ET
# Try to parse a malformed XML file
try:
ET.parse('malformed.xml')
except ET.ParseError:
print('Failed to parse XML file')
# Output:
# 'Failed to parse XML file'
In this example, we try to parse a malformed XML file. When the ET.parse
function raises a ParseError
, we catch it and print an error message.
Understanding XML and Its Comparison to Other Data Formats
XML, or Extensible Markup Language, is a widely used data format. It’s similar to HTML in its use of tags, but unlike HTML, XML allows you to define your own tags. This makes it a flexible and powerful tool for storing and exchanging data.
In comparison to other data formats like JSON or CSV, XML offers a few distinct advantages. It’s human-readable and machine-readable, supports complex nested data structures, and allows for the use of namespaces to avoid element name conflicts.
However, XML is also more verbose than JSON or CSV, which can lead to larger file sizes. It also requires a parser to read and write data, whereas JSON and CSV can be read and written with standard text editors.
Exploring DOM and SAX Parsing Models
When it comes to parsing XML files in Python, you’ll typically use one of two models: DOM (Document Object Model) or SAX (Simple API for XML).
Understanding the DOM Model
The DOM model treats an XML document as a tree of nodes or elements. This makes it easy to navigate and manipulate the document, but it also means that the entire document needs to be loaded into memory, which can be a problem for large files.
Here’s a simple example of parsing an XML file with the DOM model using the xml.dom.minidom
module:
# Import the required module
from xml.dom.minidom import parse
# Parse the XML file
dom = parse('example.xml')
# Get the root element
root = dom.documentElement
# Print the tag of the root element
print(root.tagName)
# Output:
# 'root_element'
Understanding the SAX Model
The SAX model, on the other hand, treats an XML document as a stream of elements. This makes it more memory-efficient than the DOM model, but it also makes the document harder to navigate and manipulate.
Here’s a simple example of parsing an XML file with the SAX model using the xml.sax
module:
# Import the required module
import xml.sax
# Define a handler class
class MyHandler(xml.sax.ContentHandler):
def startElement(self, name, attrs):
print('startElement', name)
def endElement(self, name):
print('endElement', name)
# Create a parser
parser = xml.sax.make_parser()
# Set the handlerparser.setContentHandler(MyHandler())
# Parse the XML file
parser.parse('example.xml')
# Output:
# 'startElement' 'root_element'
# 'endElement' 'root_element'
In this example, we define a handler class with methods for handling the start and end of elements. We then create a parser, set the handler, and parse the XML file.
Integrating XML Parsing into Larger Python Projects
The skills of parsing and generating XML files in Python can be applied to a wide range of larger projects. Two common applications are web scraping and data analysis.
XML Parsing in Web Scraping
Web scraping is the process of extracting data from websites. Many websites use XML (or HTML, which is a type of XML) to structure their data, so knowing how to parse XML can be a crucial skill for web scraping.
For example, you could write a Python script that uses the requests
library to download an XML sitemap from a website, then uses xml.etree.ElementTree
to parse the sitemap and extract the URLs of all the pages on the site.
XML Parsing in Data Analysis
XML is also a common format for data files, especially in fields like bioinformatics. If you’re analyzing data in Python, you might need to parse XML files to extract the data you need.
For example, you could write a Python script that uses xml.etree.ElementTree
to parse an XML file of gene expression data, then uses a library like pandas
or numpy
to analyze the data.
Exploring Related Topics
Once you’re comfortable with XML parsing and generation in Python, there are many related topics you could explore. Two suggestions are JSON parsing and web scraping with BeautifulSoup.
JSON (JavaScript Object Notation) is a lightweight data-interchange format that’s easy to read and write. It’s similar to XML, but less verbose and easier to work with in many cases. Python’s json
module provides tools for parsing and generating JSON data.
BeautifulSoup is a Python library for parsing HTML and XML documents. It’s often used for web scraping, and provides a more flexible and powerful interface than xml.etree.ElementTree
.
Further Resources for Mastering XML in Python
If you’re interested in learning more about XML parsing and generation in Python, here are some resources you might find helpful:
- Python JSON Mastery: Step-by-Step – Learn about JSON data validation and data structure integrity.
Excel Data Handling with Python’s openpyxl – Dive into the details of reading, writing, and modifying Excel spreadsheets.
Python YAML Processing: Techniques and Examples – Dive into the world of YAML parsing and data manipulation in Python.
Official Documentation for Python’s ‘xml.etree.ElementTree’ module for XML processing.
Official Documentation for ‘lxml’ Library – Consult ‘lxml’ library’s official documentation to learn about its features and applications in Python.
Also consider BeautifulSoup Library’s Official Documentation for comprehensive understanding of HTML and XML parsing in Python.
Wrapping Up: Mastering XML Parsing and Generation in Python
In this comprehensive guide, we’ve explored the ins and outs of working with XML files in Python, from parsing to generation, and everything in between.
We started with the basics, learning how to parse XML files using Python’s built-in xml.etree.ElementTree
module. We then dove deeper, exploring advanced techniques like XPath expressions and XML generation. We also ventured into alternative approaches, examining libraries like lxml
and xml.dom.minidom
and their unique strengths.
Along the way, we tackled common challenges you might encounter when working with XML in Python, such as handling namespaces and dealing with malformed XML files, providing you with practical solutions and examples for each issue.
We also delved into the fundamentals of XML, comparing it to other data formats and discussing the DOM and SAX parsing models. And we looked at how XML parsing and generation fit into larger Python projects, such as web scraping and data analysis.
Here’s a quick comparison of the methods and libraries we’ve discussed:
Method/Library | Pros | Cons |
---|---|---|
xml.etree.ElementTree | Built-in, intuitive | Less powerful than some alternatives |
XPath expressions | Efficient, flexible | Steeper learning curve |
lxml | Powerful features like XPath and XSLT support | Requires installation |
xml.dom.minidom | Implements the DOM model | Less intuitive than tree-based models |
Whether you’re just starting out with XML in Python or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of XML parsing and generation in Python.
With its balance of simplicity and power, Python is a fantastic tool for working with XML. Now, you’re well equipped to handle any XML tasks that come your way. Happy coding!