Openpyxl: The Python Excel Library Guide
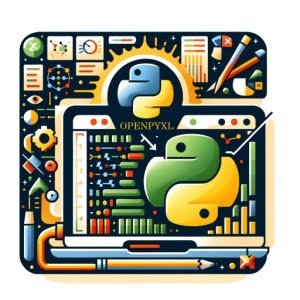
Struggling with handling Excel files in Python? You’re not alone. Many Python developers find it challenging to work with Excel files due to their complex structure and unique data handling requirements. But don’t worry, just like a skilled librarian, openpyxl can help you manage your Excel files efficiently.
This guide will take you through the ins and outs of the openpyxl library, from basic usage to advanced techniques. Whether you’re a beginner just starting out with Python and Excel, or an experienced developer looking to streamline your data processing tasks, this guide has something for you.
So let’s dive in and start mastering openpyxl!
TL;DR: How Do I Use Openpyxl in Python?
Openpyxl is a Python library for reading and writing Excel files. It allows you to create, modify, and manage Excel files in a simple and efficient way.
Here’s a simple example of how to use it:
from openpyxl import Workbook
wb = Workbook()
ws = wb.active
ws['A1'] = 'Hello'
wb.save('sample.xlsx')
# Output:
# A new Excel file named 'sample.xlsx' is created with 'Hello' in cell A1.
In this example, we first import the Workbook class from the openpyxl library. We then create a new workbook and get the active worksheet. We assign the value ‘Hello’ to cell A1 and finally save the workbook as ‘sample.xlsx’.
This is a basic way to use openpyxl in Python, but there’s much more to learn about handling Excel files in Python. Continue reading for more detailed information and advanced usage examples.
Table of Contents
Getting Started with Openpyxl
Openpyxl is a Python library that allows you to read and write Excel files. Here’s how you can get started with it.
Installation
First, you need to install the library. You can do this using pip, the Python package installer. Open your command line and type the following command:
pip install openpyxl
# Output:
# Successfully installed openpyxl
This command will download and install the openpyxl library on your system.
Importing the Library
Once installed, you can import the library into your Python script using the following line of code:
from openpyxl import Workbook
Creating a Workbook
Creating a new workbook is as simple as instantiating a new Workbook object. Here’s how:
wb = Workbook()
Adding Data to Cells
You can add data to a cell by accessing it through its coordinate and assigning a value. For example, to add the text ‘Hello’ to cell A1, you would do:
ws = wb.active
ws['A1'] = 'Hello'
Saving the Workbook
Finally, to save your workbook, you can use the save method and provide a filename. For example:
wb.save('sample.xlsx')
# Output:
# The file 'sample.xlsx' is saved with 'Hello' in cell A1.
And that’s it! You’ve created a new Excel file, added some data, and saved it, all using Python and openpyxl. But this is just the beginning. Openpyxl offers much more functionality, which we’ll explore in the next sections.
Advanced Openpyxl Techniques
As you become more comfortable with openpyxl, you can start to explore its more advanced features. These include reading data from existing files, using formulas, formatting cells, and handling multiple worksheets.
Reading Data from Existing Files
To read data from an existing Excel file, you can use the load_workbook function. Here’s how:
from openpyxl import load_workbook
wb = load_workbook('sample.xlsx')
ws = wb.active
print(ws['A1'].value)
# Output:
# 'Hello'
This code opens the ‘sample.xlsx’ file we created earlier, accesses the active worksheet, and prints the value of cell A1.
Using Formulas
Openpyxl also supports Excel formulas. You can assign a formula to a cell just like you would assign a value. For example:
ws['A2'] = '=SUM(1, 1)'
wb.save('sample.xlsx')
# Output:
# The file 'sample.xlsx' is saved with the formula '=SUM(1, 1)' in cell A2.
This code assigns the formula ‘=SUM(1, 1)’ to cell A2 and saves the workbook.
Formatting Cells
You can format cells using the openpyxl.styles module. For example, you can change the font color of a cell like this:
from openpyxl.styles import Font, Color
red_font = Font(color='00FF0000')
ws['A1'].font = red_font
wb.save('sample.xlsx')
# Output:
# The file 'sample.xlsx' is saved with cell A1's font color changed to red.
This code creates a new Font object with the color set to red (in RGB format), assigns this font to cell A1, and saves the workbook.
Handling Multiple Worksheets
You can create, access, and manipulate multiple worksheets using openpyxl. Here’s how you can create a new worksheet:
ws1 = wb.create_sheet('NewSheet')
ws1['A1'] = 'Hello from NewSheet'
wb.save('sample.xlsx')
# Output:
# The file 'sample.xlsx' is saved with a new worksheet 'NewSheet' and 'Hello from NewSheet' in cell A1 of 'NewSheet'.
This code creates a new worksheet named ‘NewSheet’, adds some data to cell A1, and saves the workbook.
By exploring these advanced features, you can start to leverage the full power of openpyxl and handle Excel files with ease.
Exploring Alternative Python Libraries for Excel
While openpyxl is a powerful library for handling Excel files in Python, it’s not the only one. There are other libraries, such as pandas and xlrd/xlwt, that offer different approaches to working with Excel files. Let’s take a look at these alternatives and consider their benefits and drawbacks.
Pandas: Data Analysis Powerhouse
Pandas is a popular data analysis library in Python that provides powerful data structures and data analysis tools. It has built-in functions for reading and writing Excel files.
Here’s an example of how you can read an Excel file using pandas:
import pandas as pd
data = pd.read_excel('sample.xlsx')
print(data)
# Output:
# Prints the content of the 'sample.xlsx' file.
In this example, we use the read_excel function to read the ‘sample.xlsx’ file and print its content. Pandas is especially useful when dealing with large datasets, as it provides efficient data structures and operations for data manipulation.
However, pandas might be overkill if you only need to perform simple operations on Excel files. It also has a steep learning curve compared to openpyxl.
Xlrd/Xlwt: Reading and Writing Excel Files
Xlrd and xlwt are two libraries that allow you to read and write Excel files, respectively. They are older than openpyxl and don’t support the newer .xlsx file format, but they are still widely used due to their simplicity and efficiency.
Here’s an example of how you can read an Excel file using xlrd:
import xlrd
book = xlrd.open_workbook('sample.xls')
sheet = book.sheet_by_index(0)
print(sheet.cell_value(0, 0))
# Output:
# Prints the value of cell A1 in the 'sample.xls' file.
In this example, we use xlrd to open the ‘sample.xls’ file, access the first worksheet, and print the value of cell A1. Xlrd and xlwt are simple and efficient, but their lack of support for .xlsx files and some advanced Excel features make them less versatile than openpyxl.
In conclusion, while openpyxl is a comprehensive and powerful library for handling Excel files in Python, there are alternatives like pandas and xlrd/xlwt that might be more suitable depending on your specific needs and circumstances. It’s important to understand the strengths and weaknesses of each library and choose the one that best fits your project.
Troubleshooting Openpyxl: Common Issues and Solutions
As you work with openpyxl, you may encounter certain issues. These can range from handling large files, dealing with different Excel versions, or troubleshooting common errors. Here, we’ll discuss these common problems and how to resolve them.
Handling Large Files
Working with large Excel files can be challenging due to memory constraints. Openpyxl has a read_only
mode that allows you to read large Excel files efficiently.
from openpyxl import load_workbook
wb = load_workbook('large_file.xlsx', read_only=True)
ws = wb.active
for row in ws.rows:
for cell in row:
print(cell.value)
# Output:
# Prints the values of all cells in 'large_file.xlsx'.
In this example, we open a large Excel file in read_only
mode and print the values of all cells. This mode allows openpyxl to read the file without loading the entire workbook into memory, making it much more memory-efficient.
Dealing with Different Excel Versions
Openpyxl supports the .xlsx file format, which is used by Excel 2007 and later. If you need to work with the older .xls format, you might encounter compatibility issues. In this case, you can use libraries like xlrd
and xlwt
, as we discussed in the previous section.
Troubleshooting Common Errors
You might encounter errors while using openpyxl, such as InvalidFileException
when trying to open a non-Excel file, or TypeError
when trying to assign a non-string value to a cell. Understanding these errors and how to fix them is crucial for efficient work.
For example, if you encounter an InvalidFileException
, make sure the file you’re trying to open is an Excel file and that its path is correct. If you encounter a TypeError
, check the type of the value you’re trying to assign to a cell and make sure it’s a string, number, or date.
By understanding these common issues and their solutions, you can make your work with openpyxl smoother and more efficient.
Understanding Excel Files and the .xlsx Format
Before diving deeper into the openpyxl library, it’s important to understand the basics of Excel files and the .xlsx format.
Excel File Structure
An Excel file, or more specifically a .xlsx file, is a package of XML files. This package includes files that represent worksheets, charts, and other elements of an Excel workbook. Each worksheet is represented by a separate XML file, which contains the data of the cells in the worksheet.
The .xlsx Format
The .xlsx format is a Microsoft Excel Open XML Spreadsheet file format. This format is based on the Open XML standard, which allows for the creation of documents that can be opened by a wide variety of software applications. The ‘x’ in .xlsx stands for XML, indicating that this file format is based on XML.
Python and .xlsx Files
Python, being a versatile and powerful programming language, can interact with .xlsx files using libraries like openpyxl. These libraries provide classes and methods that allow you to read, write, and manipulate .xlsx files in a Pythonic way.
For example, openpyxl represents an Excel workbook as a Workbook object, an Excel worksheet as a Worksheet object, and an Excel cell as a Cell object. This allows you to work with Excel files in a way that’s consistent with Python’s object-oriented paradigm.
Here’s an example of how Python can interact with .xlsx files using openpyxl:
from openpyxl import load_workbook
# Load the workbook
wb = load_workbook('sample.xlsx')
# Access a worksheet
ws = wb['Sheet1']
# Access a cell
cell = ws['A1']
# Print the cell's value
print(cell.value)
# Output:
# Prints the value of cell A1 in 'Sheet1' of 'sample.xlsx'.
In this example, we load a workbook, access a worksheet, access a cell, and print the cell’s value. This demonstrates how Python can interact with the various elements of a .xlsx file using openpyxl.
Openpyxl: Beyond Excel Files Management
Openpyxl isn’t just a tool for reading and writing Excel files. Its capabilities extend far beyond, making it a valuable asset in data analysis, automation, and larger projects.
Openpyxl in Data Analysis
Data analysis often involves processing and manipulating large datasets, which are commonly stored in Excel files. Openpyxl provides a Pythonic and efficient way to handle these files, making it an essential tool for data analysts.
# Example: Calculating the average of a column of numbers in an Excel file
from openpyxl import load_workbook
wb = load_workbook('data.xlsx')
ws = wb.active
# Assume the numbers are in column A
numbers = [cell.value for cell in ws['A'] if isinstance(cell.value, (int, float))]
average = sum(numbers) / len(numbers)
print(average)
# Output:
# Prints the average of the numbers in column A of 'data.xlsx'.
In this example, we calculate the average of a column of numbers in an Excel file. This is a simple form of data analysis that can be performed using openpyxl.
Openpyxl in Automation
Automation often involves repetitive tasks, such as generating reports or updating data. Openpyxl can automate these tasks, saving time and reducing errors.
# Example: Automatically updating an Excel report
from openpyxl import load_workbook
import datetime
wb = load_workbook('report.xlsx')
ws = wb.active
# Update the date in cell A1
ws['A1'] = datetime.datetime.now()
wb.save('report.xlsx')
# Output:
# The file 'report.xlsx' is saved with the current date in cell A1.
In this example, we automatically update the date in an Excel report. This is a simple form of automation that can be performed using openpyxl.
Further Resources for Mastering Openpyxl
To continue your journey in mastering openpyxl, consider exploring these resources:
- Python JSON Techniques – Learn how to handle JSON data from web services and APIs in Python.
Excel Data Manipulation in Python – Discover how to read, write, and analyze Excel files using Python libraries.
Python and XML: Working with XML Data – Dive into the world of XML manipulation and parsing in Python.
Openpyxl’s Official Documentation – The official documentation of the library’s features and how to use them.
Openpyxl Tutorial by Real Python offers a detailed tutorial on openpyxl, complete with examples and explanations.
Tools for Working with Excel and Python – This guide provides practical examples of how to perform common tasks with Excel files in Python.
By exploring these resources and practicing with openpyxl, you can become proficient in handling Excel files in Python and leverage this skill in your data analysis, automation, or other larger projects.
Wrapping Up: Mastering Openpyxl in Python
Throughout this guide, we’ve explored how to use the openpyxl
library to handle Excel files in Python.
We’ve learned how to read and write data, use formulas, format cells, and work with multiple worksheets. We also discussed how to troubleshoot common issues, such as handling large files and dealing with different Excel versions.
We compared openpyxl
with alternative libraries like pandas
and xlrd/xlwt
, highlighting their strengths and weaknesses. Here’s a brief comparison:
Library | Strengths | Weaknesses |
---|---|---|
openpyxl | Comprehensive features, supports .xlsx format | Can be slow with large files |
pandas | Powerful data analysis tools, efficient with large datasets | Steep learning curve |
xlrd/xlwt | Simple and efficient | Doesn’t support .xlsx format or advanced Excel features |
While openpyxl
is a powerful tool for handling Excel files in Python, it’s important to choose the library that best fits your specific needs and circumstances. Whether it’s openpyxl for its comprehensive features, pandas for its data analysis capabilities, or xlrd/xlwt for their simplicity and efficiency, mastering these tools can greatly enhance your data handling skills in Python.
Remember, practice makes perfect. So keep exploring, keep coding, and you’ll become proficient in handling Excel files in Python with openpyxl in no time!