Variables in Java | Understanding the Concepts and Syntax
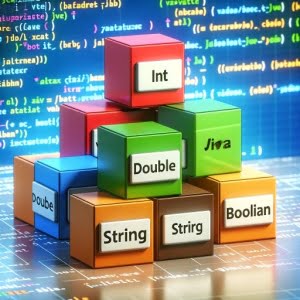
Are you finding it challenging to work with variables in Java? You’re not alone. Many developers, especially beginners, find variables in Java a bit complex. But, think of Java variables as the building blocks of your program – they’re fundamental to any coding task.
This guide will walk you through everything you need to know about variables in Java, from declaration to advanced usage. We’ll cover everything from the basics of declaring and initializing variables, to more advanced concepts like local, instance, and static variables, and even final, volatile, and transient variables.
So, let’s dive in and start mastering variables in Java!
TL;DR: How Do I Use Variables in Java?
In Java, you declare a
variable
by specifying its type and name, and you can initialize it by assigning a value, for exampleint myVar = 10;
. The type could be any Java data type, and the variable name is any valid identifier.
Here’s a simple example:
int myVar = 10;
System.out.println(myVar);
// Output:
// 10
In this example, we’ve declared a variable named myVar
of type int
and initialized it with the value 10
. We then print the value of myVar
to the console, which outputs 10
.
This is a basic way to use variables in Java, but there’s much more to learn about variables, including different types and advanced usage scenarios. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Declaring, Initializing, and Using Variables in Java
- Diving Deeper: Local, Instance, and Static Variables
- Advanced Variable Concepts: Final, Volatile, and Transient Variables
- Troubleshooting Common Issues with Java Variables
- Understanding Variables, Data Types, and Scope in Java
- The Bigger Picture: Variables in Object-Oriented Programming and Memory Management
- Wrapping Up: Variables in Java
Declaring, Initializing, and Using Variables in Java
In Java, the process of using a variable involves three steps: declaration, initialization, and usage.
Declaration
First, you need to declare a variable. When you declare a variable, you are telling Java its name and what type of data it will hold. The syntax for declaring a variable is:
<type> <variableName>;
Here’s an example of declaring an integer variable named myVar
:
int myVar;
Initialization
After declaring a variable, you can assign a value to it. This is known as initialization. The syntax for initializing a variable is:
<variableName> = <value>;
Here’s an example of initializing myVar
with the value 10
:
myVar = 10;
Usage
Once a variable is declared and initialized, you can use it in your code. For example, you can print the value of myVar
to the console:
System.out.println(myVar);
// Output:
// 10
Advantages of Variables
Variables in Java have several advantages. They allow you to store and manipulate data in your code. They also make your code more readable and maintainable, as you can assign meaningful names to your variables.
Potential Pitfalls
However, there are some potential pitfalls with using variables in Java. For example, if you try to use a variable before it’s been initialized, you’ll get a compiler error. Also, keep in mind that variables have scope – they only exist within the block of code where they’re declared. Trying to access a variable outside its scope will also result in a compiler error.
Diving Deeper: Local, Instance, and Static Variables
As you gain more experience in Java, you’ll come across different types of variables. Let’s discuss the three main types: local, instance, and static variables.
Local Variables
Local variables are declared within a block of code like methods and constructors. These variables are only accessible within the block they are declared.
Here’s an example of a local variable:
void myMethod() {
int localVariable = 10;
System.out.println(localVariable);
}
// Output:
// 10
In this example, localVariable
is a local variable. It’s only accessible within the myMethod
block.
Instance Variables
Instance variables are declared inside a class but outside a method. Each object of the class has its own copy of the instance variable.
Here’s an example of an instance variable:
public class MyClass {
int instanceVariable = 10;
}
In this example, instanceVariable
is an instance variable. Each object of MyClass
will have its own instanceVariable
.
Static Variables
Static variables are declared as static, which means they belong to the class, not the object. All instances of the class share the same static variable.
Here’s an example of a static variable:
public class MyClass {
static int staticVariable = 10;
}
In this example, staticVariable
is a static variable. It belongs to MyClass
and is shared among all its instances.
Understanding these different types of variables in Java and how to use them is crucial as you become more proficient in Java programming.
Advanced Variable Concepts: Final, Volatile, and Transient Variables
As you venture deeper into Java programming, you’ll encounter advanced concepts related to variables. Let’s delve into final, volatile, and transient variables.
Final Variables
Final variables in Java are constants. Once a final variable has been assigned, it cannot be changed. This feature is useful when you want to create a variable that should always hold the same value.
Here’s an example of a final variable:
final int MY_CONSTANT = 100;
System.out.println(MY_CONSTANT);
// Output:
// 100
In this example, MY_CONSTANT
is a final variable. Once it is assigned the value 100
, it cannot be changed.
Volatile Variables
Volatile variables are used in multi-threaded applications. The volatile keyword in Java is used to ensure that changes made in one thread are immediately visible to other threads.
Here’s an example of a volatile variable:
volatile boolean active = true;
In this example, active
is a volatile variable. If one thread changes the value of active
, other threads will see the new value immediately.
Transient Variables
Transient variables are not serialized. Serialization is the process of converting an object into a byte stream. If you don’t want to serialize some variables because they are not important or sensitive, you can declare them as transient.
Here’s an example of a transient variable:
transient int tempData;
In this example, tempData
is a transient variable. It will not be included in the serialization process.
Each of these variable concepts has its advantages and disadvantages, and their usage depends on the specific requirements of your Java program. Understanding them can significantly enhance your Java programming skills.
Troubleshooting Common Issues with Java Variables
While working with variables in Java, you may encounter a few common issues. Let’s discuss these problems and their solutions.
Type Mismatch
A type mismatch occurs when you try to assign a value of one type to a variable of a different type. Java is a statically-typed language, which means the type of variable is checked at compile-time.
Here’s an example of a type mismatch:
int myVar = "Hello";
// Error:
// incompatible types: String cannot be converted to int
In this example, we’re trying to assign a String value to an integer variable, which results in a type mismatch error. To solve this, ensure the value you’re assigning to a variable matches its type.
Uninitialized Variables
An uninitialized variable is a variable that’s been declared but not given a value. If you try to use an uninitialized variable, Java will throw a compiler error.
Here’s an example of an uninitialized variable:
int myVar;
System.out.println(myVar);
// Error:
// variable myVar might not have been initialized
In this example, we’re trying to print the value of myVar
, but we haven’t given myVar
a value. To solve this, always initialize your variables before you use them.
Understanding these common issues and their solutions can help you write more robust Java code and troubleshoot issues more efficiently.
Understanding Variables, Data Types, and Scope in Java
To effectively work with variables in Java, it’s essential to understand the underlying concepts: variables, data types, and scope.
What are Variables?
In Java, a variable is a container that holds values that are used in a Java program. Every variable is assigned a data type which designates the type and quantity of value it can hold.
int myNumber = 5;
In this example, myNumber
is a variable that holds the integer value 5
.
Understanding Data Types
Java is a statically-typed language, which means every variable must first be declared before it can be used. This involves stating the variable’s type and name.
Java supports several data types. For example, int
for integers, double
for floating point numbers, boolean
for true/false values, and String
for a sequence of characters.
int myNumber = 5;
double myDouble = 5.5;
boolean myBoolean = true;
String myString = "Hello";
In these examples, we declare variables of different data types and assign them appropriate values.
Grasping the Concept of Scope
In Java, the scope of a variable is the part of the code where the variable can be accessed. A variable’s scope is determined by where the variable is declared. For example, if a variable is declared inside a method, it can only be used within that method. This is known as a local variable.
void myMethod() {
int localVariable = 5;
System.out.println(localVariable);
}
// Output:
// 5
In this example, localVariable
is a local variable. It can only be accessed within the myMethod
block. Attempting to access it outside this block will result in a compiler error.
Understanding these fundamental concepts is crucial for mastering the use of variables in Java.
The Bigger Picture: Variables in Object-Oriented Programming and Memory Management
Understanding variables in Java isn’t just about knowing how to declare, initialize, and use them. It also involves understanding their relevance in broader concepts like object-oriented programming and memory management.
Variables in Object-Oriented Programming
In object-oriented programming (OOP), variables play a crucial role. They represent the state of an object. Each object can have different state but share the same structure, thanks to instance variables.
public class Car {
String color;
String model;
}
In this example, color
and model
are instance variables. They represent the state of a Car
object. Each Car
object will have its own color
and model
.
Variables and Memory Management
Understanding how variables work with memory can help you write more efficient Java code. When you declare a variable, Java allocates memory for it based on its data type. When a variable is no longer needed, Java’s garbage collector automatically reclaims its memory.
Exploring Related Concepts
Beyond variables, there are other fundamental concepts in Java worth exploring, such as classes, methods, and more. These concepts are interconnected with variables and offer a more comprehensive understanding of Java programming.
Further Resources for Mastering Java Variables
To further your understanding of Java variables, consider exploring the following resources:
- Exploring Java Methods – Explore the fundamentals of Java methods for structuring and organizing code efficiently.
Java Access Modifiers Explained – Explore Java’s access modifiers to enforce encapsulation and secure code.
Oracle’s Java Tutorials – Comprehensive tutorials from the creators of Java.
Java Variables and Data Types – A detailed guide by W3Schools.
Java Programming for Beginners – A Udemy course that covers Java variables and more.
Wrapping Up: Variables in Java
In this comprehensive guide, we’ve delved into the world of variables in Java, starting from the basics and moving on to more advanced concepts.
We began with the basics of declaring, initializing, and using variables in Java. We discussed the importance of variables, their advantages, and potential pitfalls. We then explored the different types of variables in Java, such as local, instance, and static variables, and provided code examples to illustrate their usage.
We also introduced advanced variable concepts, such as final, volatile, and transient variables, and discussed their usage and effectiveness. Along the way, we tackled common issues you might encounter when working with variables in Java, such as type mismatch and uninitialized variables, and provided solutions for each issue.
Here’s a quick comparison of the types of variables we’ve discussed:
Variable Type | Scope | Characteristics |
---|---|---|
Local Variables | Within the block they are declared | Accessible only within the block they are declared |
Instance Variables | Within the entire class | Each object of the class has its own copy |
Static Variables | Within the entire class | Shared among all instances of the class |
Final Variables | – | Cannot be changed once assigned |
Volatile Variables | – | Changes made in one thread are immediately visible to other threads |
Transient Variables | – | Not serialized |
Whether you’re just starting out with Java or you’re looking to level up your programming skills, we hope this guide has given you a deeper understanding of variables in Java and how to use them effectively.
With a firm grasp of variables, you’re now better equipped to write robust and efficient Java code. Happy coding!