Spring Boot Actuator: Monitoring Your Spring Applications
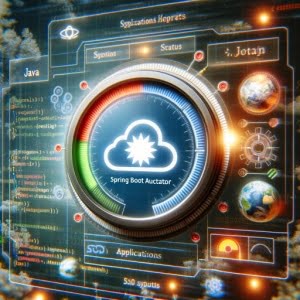
Are you finding it challenging to manage and monitor your Spring Boot application? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a car’s dashboard, Spring Boot Actuator provides essential features to keep your application running smoothly. These features allow you to monitor and manage your application, just as a car’s dashboard allows you to monitor and control the vehicle.
In this guide, we’ll walk you through the ins and outs of using Spring Boot Actuator, from basic use to advanced techniques. We’ll explore Spring Boot Actuator’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Spring Boot Actuator!
TL;DR: What is Spring Boot Actuator and How Do I Use It?
Spring Boot Actuator
is a sub-project of Spring Boot that provides built-in endpoints to monitor and manage your application. To use it, you simply need to add thespring-boot-starter-actuator
dependency to your project.
Here’s a simple example of how to add the dependency in your pom.xml
file:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
</dependencies>
In this example, we’ve added the spring-boot-starter-actuator
dependency to our Spring Boot project. This enables the Actuator’s features, allowing us to monitor and manage our application.
This is just a basic way to use Spring Boot Actuator, but there’s much more to learn about managing and monitoring your Spring Boot applications. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started with Spring Boot Actuator
- Customizing and Securing Actuator Endpoints
- Exploring Alternatives: JMX and Remote Shell Access
- Troubleshooting Spring Boot Actuator
- Spring Boot: Embracing Convention over Configuration
- The Role of Spring Boot Actuator
- Spring Boot Actuator in Larger Projects
- Wrapping Up:
Getting Started with Spring Boot Actuator
Spring Boot Actuator is easy to integrate into your project. Let’s go through a step-by-step guide on how to add Spring Boot Actuator to your project and how to use its built-in endpoints to monitor your application.
Step 1: Adding the Actuator Dependency
Firstly, you need to add the spring-boot-starter-actuator
dependency to your pom.xml
file. The code block from the example adds the necessary dependency to your Spring Boot project. Once you’ve done this, Spring Boot Actuator’s features become available to your application.
Step 2: Accessing the Endpoints
Once the Actuator is added, you can access various built-in endpoints to monitor your application. For instance, you can use the /health
endpoint to check the health status of your application.
curl localhost:8080/actuator/health
# Output:
# {
# "status": "UP"
# }
In the example above, we used the curl
command to send a GET request to the /health
endpoint. The UP
status indicates that our application is running smoothly.
The Spring Boot Actuator offers many benefits, such as easy monitoring and management of your application. It provides a wide range of endpoints that offer insights into your application’s health, metrics, info, and more. However, a potential pitfall is that exposing all Actuator’s endpoints might reveal sensitive information. Therefore, it’s crucial to secure sensitive endpoints, which we will cover in the advanced use section.
Customizing and Securing Actuator Endpoints
As you gain more experience with Spring Boot Actuator, you may want to customize and secure the Actuator endpoints. This allows you to tailor the Actuator’s functionality to your specific needs and protect sensitive information.
Customizing Actuator Endpoints
Spring Boot Actuator allows you to customize its endpoints. For instance, you can change the ID of an endpoint, disable an endpoint, or even create your own endpoints. Let’s see how you can change the ID of the health
endpoint to status
.
You can do this in your application.properties
file:
management.endpoints.web.path-mapping.health=status
In this example, we’ve changed the ID of the health
endpoint to status
. Now, you can access the health status of your application at the /status
path.
Securing Actuator Endpoints
Securing your Actuator endpoints is crucial to protect sensitive information. You can use Spring Security to secure your endpoints. Here’s an example of how to allow only authenticated users to access the health
endpoint.
First, add the Spring Security dependency to your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
Then, configure the security settings in your application.properties
file:
management.endpoint.health.enabled=true
management.endpoints.web.exposure.include=*
management.endpoints.web.exposure.exclude=health
In the example above, we’ve allowed all endpoints to be exposed except for the health
endpoint. Now, only authenticated users can access the health
endpoint.
Customizing and securing Actuator endpoints can greatly enhance your application’s monitoring capabilities and security. However, remember that improper configuration can lead to unauthorized access or exposure of sensitive information. Therefore, always be cautious when configuring your Actuator endpoints.
Exploring Alternatives: JMX and Remote Shell Access
While Spring Boot Actuator is a powerful tool for monitoring and managing your Spring Boot applications, it’s not the only tool in the toolbox. There are other approaches, such as JMX (Java Management Extensions) and remote shell access. Let’s delve into these alternatives and discuss their benefits, drawbacks, and when you might choose to use them.
JMX: In-depth Application Monitoring
JMX is a Java technology that supplies tools for managing and monitoring applications. You can monitor resource consumption, track system performance, and even perform operations on your application at runtime.
To enable JMX monitoring for your Spring Boot application, you need to add the following line to your application.properties
file:
spring.jmx.enabled=true
With this setting, your application will expose MBeans that JMX clients can interact with. Here’s a simple JConsole view of a Spring Boot application:
# Output:
# [A screenshot of JConsole showing various MBeans exposed by a Spring Boot application]
JMX provides a wealth of information and control over your application. However, it can be complex to use and may not be suitable for all use-cases or teams.
Remote Shell Access: Direct Control
Remote shell access allows you to interact with your application directly via a shell, providing a high level of control. However, it requires careful security considerations.
You can enable remote shell access by adding the spring-shell-starter
dependency to your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-shell-starter</artifactId>
</dependency>
Once enabled, you can connect to your application using SSH and perform various operations.
ssh -p 2000 user@localhost
In this example, we’re connecting to our application via SSH on port 2000. The shell provides a range of commands for interacting with the application.
While powerful, remote shell access can pose a significant security risk if not properly secured. It should only be used when necessary and with appropriate security measures in place.
When deciding between Spring Boot Actuator, JMX, and remote shell access, consider your team’s needs, skills, and the specific requirements of your application. Each tool has its strengths and weaknesses, and the best choice depends on your particular situation.
Troubleshooting Spring Boot Actuator
Like any tool, Spring Boot Actuator might present some challenges. Let’s go through some common issues and their solutions. We’ll also share some tips for best practices and optimization.
Issue: Endpoints Not Exposed
One common issue is finding that your Actuator endpoints are not exposed after adding the Actuator dependency. This might be due to security configurations or properties settings.
Here’s an example of a curl
command that results in a 404 error:
curl localhost:8080/actuator/health
# Output:
# {"timestamp":"2022-01-01T00:00:00.000+00:00","status":404,"error":"Not Found","message":"No message available","path":"/actuator/health"}
In this example, the health
endpoint is not exposed, leading to a 404 error. To solve this, you need to ensure that the management.endpoints.web.exposure.include
property in your application.properties
file includes health
.
management.endpoints.web.exposure.include=health,info
This property configuration exposes the health
and info
endpoints.
Issue: Unauthorized Access
Another common issue is receiving a 401 Unauthorized error when trying to access an endpoint. This is typically due to Spring Security configurations.
Here’s an example of a curl
command that results in a 401 error:
curl localhost:8080/actuator/health
# Output:
# {"timestamp":"2022-01-01T00:00:00.000+00:00","status":401,"error":"Unauthorized","message":"Unauthorized","path":"/actuator/health"}
In this example, the health
endpoint is secured, and the request does not include the necessary authentication, leading to a 401 error. To solve this, you need to include the necessary authentication in your request or adjust your security configurations.
Best Practices and Optimization
When using Spring Boot Actuator, it’s important to follow best practices to optimize your application’s performance and security. Here are some tips:
- Secure Your Endpoints: Always secure sensitive Actuator endpoints to prevent unauthorized access.
- Limit Exposed Endpoints: Only expose the endpoints that you need to minimize the surface area for potential attacks.
- Customize Endpoint Paths: Customize the paths of your endpoints to avoid conflicts with your application’s other routes.
- Use the
info
Endpoint: Use theinfo
endpoint to expose useful information about your application, such as build version or git commit information.
Spring Boot: Embracing Convention over Configuration
To fully appreciate the Spring Boot Actuator, it’s crucial to understand the underlying philosophy of the Spring Boot framework. Spring Boot embraces the concept of ‘convention over configuration.’ This approach minimizes the decisions that developers need to make, reducing the complexity of application development.
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
In the above code snippet, we have a simple Spring Boot application. The @SpringBootApplication
annotation is a convenience annotation that adds all of the following:
@Configuration
: Tags the class as a source of bean definitions for the application context.@EnableAutoConfiguration
: Tells Spring Boot to start adding beans based on classpath settings, other beans, and various property settings.@ComponentScan
: Tells Spring to look for other components, configurations, and services in thecom/example
package, allowing it to find the controllers.
The main method uses Spring Boot’s SpringApplication.run()
method to launch an application.
The Role of Spring Boot Actuator
Within the broader Spring Boot ecosystem, Spring Boot Actuator plays a vital role in application management and monitoring. It provides production-ready features out of the box without requiring extensive configuration, adhering to the ‘convention over configuration’ philosophy.
Spring Boot Actuator is designed to work with the Spring Boot framework, providing insights into the internals of your running application. It exposes various endpoints, allowing you to monitor application metrics, understand traffic, and even trace application errors.
These features make Spring Boot Actuator an invaluable tool in the Spring Boot ecosystem, offering deep insights into your application’s performance and behavior.
Spring Boot Actuator in Larger Projects
As your applications grow in complexity and scale, the role of Spring Boot Actuator becomes even more significant. It not only helps in monitoring and managing your application but also provides valuable insights that can guide your application’s scaling strategies.
Spring Boot’s Support for Building Web Applications
Spring Boot Actuator seamlessly integrates with Spring Boot’s support for building web applications. You can monitor various metrics related to your web application, such as HTTP request handling, session attributes, and more.
@RestController
public class HelloController {
@RequestMapping("/")
public String index() {
return "Hello, World!";
}
}
In the above code snippet, we have a simple Spring Boot web application that exposes a REST endpoint at /
. With Spring Boot Actuator, you can monitor the performance and usage of this endpoint.
Data Access and Messaging
Spring Boot Actuator also works well with Spring Boot’s support for data access and messaging. You can monitor database connections, query performance, message broker connections, and more.
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
}
In the above code snippet, we have a Spring Data JPA repository. With Spring Boot Actuator, you can monitor the performance of your data access layer.
Further Resources for Mastering Spring Boot Actuator
To delve deeper into Spring Boot Actuator and related topics, check out the following resources:
- IOFlood’s Java Spring Boot Guide – Integrate Spring Boot with other frameworks.
Spring Boot Starter: Overview – Explore Spring Boot starters for simplified dependency management.
Spring vs Spring Boot: Comparison – Understand differences between Spring and Spring Boot.
Spring Boot Actuator: Production-ready Features – The official documentation for Spring Boot Actuator. It provides a comprehensive overview of all the features.
Monitoring Spring Boot Apps with Micrometer, Prometheus, and Grafana – This tutorial guides you through setting up a monitoring dashboard for your Spring Boot application.
Spring Boot Actuator Endpoints – This article on Baeldung explains how to understand and configure Actuator endpoints.
Wrapping Up:
In this comprehensive guide, we’ve navigated the world of Spring Boot Actuator, an essential tool for managing and monitoring Spring Boot applications.
We kicked off with the basics, understanding how to integrate Spring Boot Actuator into your project and use its built-in endpoints for monitoring. We then ventured into advanced usage, exploring how to customize and secure Actuator endpoints, enhancing your application’s monitoring capabilities and security.
We also tackled common challenges you might face when using Spring Boot Actuator, such as endpoints not being exposed or unauthorized access, providing you with solutions and best practices to overcome these issues.
We took a look at alternative approaches to monitoring and managing Spring Boot applications, such as JMX and remote shell access, giving you a broader perspective on the available tools.
Method | Pros | Cons |
---|---|---|
Spring Boot Actuator | Easy integration, Comprehensive monitoring | Requires careful security configuration |
JMX | In-depth monitoring, Direct control over application | Can be complex to use |
Remote Shell Access | High level of control | Requires careful security measures |
Whether you’re just starting out with Spring Boot Actuator or you’re looking to enhance your application monitoring skills, we hope this guide has given you a deeper understanding of Spring Boot Actuator and its capabilities.
With its balance of ease of integration, comprehensive monitoring, and customizable endpoints, Spring Boot Actuator is a powerful tool for managing and monitoring your Spring Boot applications. Happy coding!